Strategies for Dynamic Connectivity Problems: Mastering Union-Find Algorithms
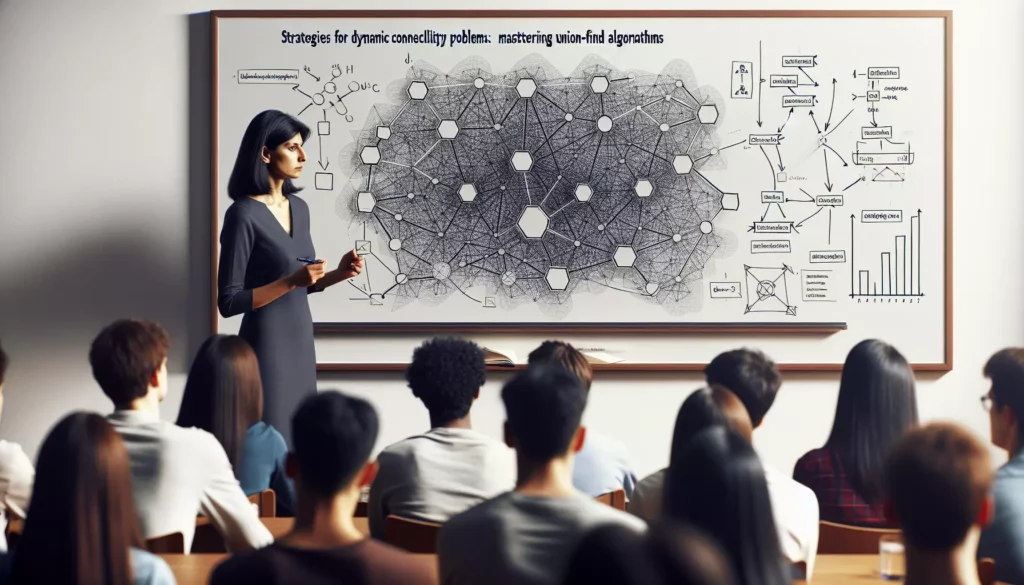
In the world of computer science and algorithmic problem-solving, dynamic connectivity problems play a crucial role. These problems involve maintaining information about the connectivity of elements in a set that changes over time. Understanding and implementing efficient solutions to these problems is essential for aspiring programmers and those preparing for technical interviews at top tech companies. In this comprehensive guide, we’ll explore various strategies for tackling dynamic connectivity problems, with a focus on Union-Find algorithms.
What are Dynamic Connectivity Problems?
Dynamic connectivity problems deal with a set of elements and connections between them. The goal is to efficiently perform two main operations:
- Union: Connect two elements
- Find: Determine if two elements are connected (directly or indirectly)
These problems are “dynamic” because the set of connections can change over time as we perform union operations. The challenge lies in implementing these operations efficiently, especially when dealing with large datasets.
The Importance of Union-Find Algorithms
Union-Find algorithms, also known as disjoint-set data structures, are the primary tools used to solve dynamic connectivity problems. They are fundamental in various applications, including:
- Network connectivity
- Image processing
- Social network analysis
- Kruskal’s algorithm for minimum spanning trees
- Percolation theory
Mastering Union-Find algorithms is crucial for coding interviews, especially when applying to FAANG (Facebook, Amazon, Apple, Netflix, Google) companies or other tech giants. Let’s dive into the different strategies and implementations of Union-Find algorithms, starting from the basic approach and progressing to more optimized versions.
1. Quick Find: The Eager Approach
The Quick Find algorithm is the most straightforward implementation of Union-Find. It’s called “eager” because it keeps track of connectivity information in a way that makes the find operation very fast.
Implementation
class QuickFind:
def __init__(self, n):
self.id = list(range(n))
def find(self, p):
return self.id[p]
def connected(self, p, q):
return self.find(p) == self.find(q)
def union(self, p, q):
pid = self.find(p)
qid = self.find(q)
if pid == qid:
return
for i in range(len(self.id)):
if self.id[i] == pid:
self.id[i] = qid
Analysis
- Initialization: O(n)
- Find: O(1)
- Union: O(n)
While Quick Find offers constant-time finds, its union operation is slow, making it inefficient for large datasets with many union operations.
2. Quick Union: The Lazy Approach
Quick Union takes a different approach by representing the connected components as trees. This method is “lazy” because it doesn’t update all elements during a union operation, potentially leading to tall trees.
Implementation
class QuickUnion:
def __init__(self, n):
self.parent = list(range(n))
def find(self, p):
while p != self.parent[p]:
p = self.parent[p]
return p
def connected(self, p, q):
return self.find(p) == self.find(q)
def union(self, p, q):
root_p = self.find(p)
root_q = self.find(q)
if root_p != root_q:
self.parent[root_p] = root_q
Analysis
- Initialization: O(n)
- Find: O(n) in the worst case
- Union: O(n) in the worst case (includes the cost of find)
Quick Union improves the union operation’s efficiency compared to Quick Find, but the find operation can be slow in the worst case of a tall tree.
3. Weighted Quick Union: Balancing the Trees
Weighted Quick Union is an improvement over Quick Union that helps keep the trees balanced. It does this by always attaching the smaller tree to the root of the larger tree during union operations.
Implementation
class WeightedQuickUnion:
def __init__(self, n):
self.parent = list(range(n))
self.size = [1] * n
def find(self, p):
while p != self.parent[p]:
p = self.parent[p]
return p
def connected(self, p, q):
return self.find(p) == self.find(q)
def union(self, p, q):
root_p = self.find(p)
root_q = self.find(q)
if root_p == root_q:
return
if self.size[root_p] < self.size[root_q]:
self.parent[root_p] = root_q
self.size[root_q] += self.size[root_p]
else:
self.parent[root_q] = root_p
self.size[root_p] += self.size[root_q]
Analysis
- Initialization: O(n)
- Find: O(log n)
- Union: O(log n)
Weighted Quick Union significantly improves both find and union operations, ensuring that the tree depth is always logarithmic in the number of nodes.
4. Path Compression: Flattening the Tree
Path Compression is an optimization technique that can be applied to both Quick Union and Weighted Quick Union. It works by making every node on the path to the root point directly to the root during find operations.
Implementation (with Weighted Quick Union)
class WeightedQuickUnionWithPathCompression:
def __init__(self, n):
self.parent = list(range(n))
self.size = [1] * n
def find(self, p):
root = p
while root != self.parent[root]:
root = self.parent[root]
while p != root:
next_p = self.parent[p]
self.parent[p] = root
p = next_p
return root
def connected(self, p, q):
return self.find(p) == self.find(q)
def union(self, p, q):
root_p = self.find(p)
root_q = self.find(q)
if root_p == root_q:
return
if self.size[root_p] < self.size[root_q]:
self.parent[root_p] = root_q
self.size[root_q] += self.size[root_p]
else:
self.parent[root_q] = root_p
self.size[root_p] += self.size[root_q]
Analysis
- Initialization: O(n)
- Find: O(α(n)), where α(n) is the inverse Ackermann function
- Union: O(α(n))
Path Compression, when combined with Weighted Quick Union, results in a nearly constant-time performance for both find and union operations. The inverse Ackermann function α(n) grows extremely slowly and is effectively constant for all practical values of n.
Comparing the Strategies
Let’s compare the time complexities of the different Union-Find implementations:
Algorithm | Initialize | Union | Find |
---|---|---|---|
Quick Find | O(n) | O(n) | O(1) |
Quick Union | O(n) | O(n) | O(n) |
Weighted Quick Union | O(n) | O(log n) | O(log n) |
Weighted QU with Path Compression | O(n) | O(α(n)) | O(α(n)) |
As we can see, the Weighted Quick Union with Path Compression provides the best overall performance, especially for large datasets.
Practical Applications and Interview Tips
Understanding and implementing Union-Find algorithms is crucial for solving various problems in coding interviews. Here are some common applications and tips:
1. Network Connectivity
Union-Find is often used to determine if two nodes in a network are connected. This can be applied to social networks, computer networks, or any system where connectivity needs to be tracked.
2. Detecting Cycles in Graphs
Union-Find can be used to detect cycles in undirected graphs. If you try to union two vertices that are already in the same set, you’ve found a cycle.
3. Kruskal’s Algorithm for Minimum Spanning Trees
Union-Find is a key component in Kruskal’s algorithm for finding the minimum spanning tree of a graph.
4. Image Processing
In image processing, Union-Find can be used for tasks like connected component labeling, where you need to identify distinct objects in an image.
Interview Tips
- Understand the trade-offs: Be prepared to explain why you chose a particular implementation based on the problem constraints.
- Implement from scratch: Practice implementing Union-Find from memory, as you may be asked to do so in an interview.
- Optimize step-by-step: Start with a basic implementation and be ready to optimize it if asked. This shows your problem-solving skills.
- Time and space complexity: Be prepared to analyze the time and space complexity of your implementation.
- Real-world applications: Discuss practical applications of Union-Find to demonstrate your understanding of its importance.
Advanced Topics and Further Optimizations
For those looking to dive deeper into Union-Find algorithms, there are several advanced topics and optimizations to explore:
1. Rank-Based Union
Instead of using size, we can use rank (an upper bound on the height of the tree) to decide which tree to attach to which. This can lead to slightly flatter trees in some cases.
2. Path Splitting and Halving
These are alternative path compression techniques that can be easier to implement in some scenarios:
- Path Splitting: Make every other node on the path point to its grandparent.
- Path Halving: Make every node on the path point to its grandparent.
3. Amortized Analysis
Understanding amortized analysis can help in proving the near-constant time complexity of operations in optimized Union-Find implementations.
4. Persistent Union-Find
This is a variation that allows for “undo” operations, maintaining the history of unions.
5. Dynamic Connectivity with Deletions
Extending Union-Find to support efficient deletions is a challenging problem with applications in dynamic graph algorithms.
Conclusion
Mastering strategies for dynamic connectivity problems, particularly Union-Find algorithms, is essential for any programmer aiming to excel in technical interviews and real-world problem-solving. From the basic Quick Find to the highly optimized Weighted Quick Union with Path Compression, each implementation offers unique trade-offs between simplicity and efficiency.
As you prepare for coding interviews, especially for top tech companies, make sure to:
- Understand the core principles behind each Union-Find implementation
- Practice implementing these algorithms from scratch
- Analyze the time and space complexities
- Explore real-world applications and be ready to discuss them
- Consider advanced optimizations and their use cases
Remember, the key to mastering these algorithms is not just understanding the theory but also applying them to solve diverse problems. Keep practicing, and you’ll be well-prepared to tackle dynamic connectivity challenges in your coding interviews and future projects.