Strategies for Converting O(n²) Solutions to O(n log n)
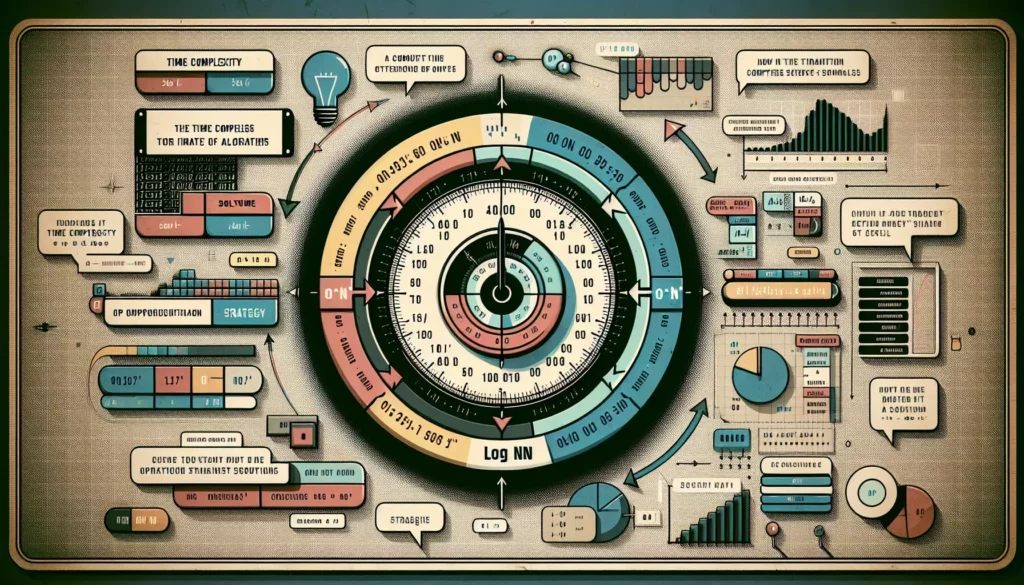
Understanding Time Complexity
Before diving into the conversion strategies, it’s crucial to understand what O(n²) and O(n log n) mean in terms of time complexity:
- O(n²): This quadratic time complexity means that the runtime grows quadratically with the input size. Common examples include nested loops iterating over an array.
- O(n log n): This linearithmic time complexity grows more slowly than quadratic time. It’s often seen in efficient sorting algorithms like mergesort and quicksort.
Converting from O(n²) to O(n log n) can make a substantial difference in runtime, especially as the input size increases.
Strategy 1: Divide and Conquer
The divide and conquer approach is a powerful technique for improving time complexity. It involves breaking down a problem into smaller subproblems, solving them independently, and then combining the results.
Example: Merge Sort
A classic example of using divide and conquer to achieve O(n log n) complexity is the merge sort algorithm. Here’s a basic implementation in Python:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
Merge sort achieves O(n log n) time complexity by repeatedly dividing the array in half and merging the sorted halves.
Strategy 2: Use Appropriate Data Structures
Sometimes, the key to improving time complexity lies in choosing the right data structure. Certain data structures can provide more efficient operations for specific tasks.
Example: Binary Search Tree
Consider a problem where you need to frequently search, insert, and delete elements. Using a balanced binary search tree (BST) like a Red-Black tree or AVL tree can provide O(log n) time complexity for these operations, compared to O(n) for an unsorted array.
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
def search(self, value):
return self._search_recursive(self.root, value)
def _search_recursive(self, node, value):
if node is None or node.value == value:
return node
if value < node.value:
return self._search_recursive(node.left, value)
return self._search_recursive(node.right, value)
Using a BST can reduce the time complexity of search operations from O(n) to O(log n) on average, assuming the tree remains balanced.
Strategy 3: Dynamic Programming
Dynamic programming can sometimes transform O(n²) solutions into more efficient ones by storing and reusing intermediate results.
Example: Longest Increasing Subsequence
The problem of finding the longest increasing subsequence (LIS) has a straightforward O(n²) solution, but it can be optimized to O(n log n) using dynamic programming with binary search.
def longest_increasing_subsequence(arr):
if not arr:
return 0
# Initialize dp array with the first element of arr
dp = [arr[0]]
for i in range(1, len(arr)):
if arr[i] > dp[-1]:
dp.append(arr[i])
else:
j = bisect_left(dp, arr[i])
dp[j] = arr[i]
return len(dp)
from bisect import bisect_left
# Example usage
arr = [10, 9, 2, 5, 3, 7, 101, 18]
print(longest_increasing_subsequence(arr)) # Output: 4
This approach uses binary search (via bisect_left
) to efficiently maintain a sorted list of potential candidates for the LIS, reducing the time complexity to O(n log n).
Strategy 4: Sorting and Binary Search
Many problems that initially seem to require O(n²) time can be solved more efficiently by first sorting the data and then using binary search.
Example: Two Sum Problem
The Two Sum problem asks to find two numbers in an array that sum to a target value. A naive O(n²) solution would check all pairs, but we can optimize it:
def two_sum(nums, target):
nums_sorted = sorted([(num, i) for i, num in enumerate(nums)])
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums_sorted[left][0] + nums_sorted[right][0]
if current_sum == target:
return [nums_sorted[left][1], nums_sorted[right][1]]
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
# Example usage
nums = [2, 7, 11, 15]
target = 9
print(two_sum(nums, target)) # Output: [0, 1]
This solution first sorts the array (O(n log n)) and then uses two pointers to find the pair, resulting in an overall O(n log n) time complexity.
Strategy 5: Preprocessing and Hashing
Sometimes, preprocessing the data and using hash tables can dramatically reduce time complexity.
Example: Counting Pairs with a Given Sum
Consider the problem of counting pairs in an array with a given sum. A naive approach would be O(n²), but we can optimize it:
from collections import defaultdict
def count_pairs_with_sum(arr, target_sum):
count = 0
freq_map = defaultdict(int)
for num in arr:
complement = target_sum - num
count += freq_map[complement]
freq_map[num] += 1
return count
# Example usage
arr = [1, 5, 7, -1, 5]
target_sum = 6
print(count_pairs_with_sum(arr, target_sum)) # Output: 3
This solution uses a hash map to store frequency counts, reducing the time complexity to O(n) at the cost of O(n) space complexity.
Strategy 6: Sliding Window Technique
The sliding window technique can be particularly useful for problems involving subarrays or substrings, often reducing O(n²) solutions to O(n).
Example: Maximum Sum Subarray of Size K
Consider finding the maximum sum of any contiguous subarray of size K in an array. A naive approach would be O(n*K), which is O(n²) when K is proportional to n. Here’s an optimized O(n) solution:
def max_sum_subarray(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
arr = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
print(max_sum_subarray(arr, k)) # Output: 39
This sliding window approach maintains a running sum of the current window, sliding it across the array in O(n) time.
Strategy 7: Prefix Sum and Difference Arrays
Prefix sums and difference arrays can transform certain range query and update operations from O(n) to O(1), effectively reducing O(n²) solutions to O(n).
Example: Range Sum Queries
Consider a problem where you need to perform multiple range sum queries on an array. A naive approach would sum the elements in the range for each query, resulting in O(n²) time complexity for n queries. Here’s an optimized solution using prefix sums:
class RangeSumQuery:
def __init__(self, nums):
self.prefix_sum = [0]
for num in nums:
self.prefix_sum.append(self.prefix_sum[-1] + num)
def sum_range(self, left, right):
return self.prefix_sum[right + 1] - self.prefix_sum[left]
# Example usage
nums = [1, 3, 5, 7, 9, 11]
rsq = RangeSumQuery(nums)
print(rsq.sum_range(1, 3)) # Output: 15
print(rsq.sum_range(2, 5)) # Output: 32
This approach precomputes prefix sums in O(n) time, allowing each subsequent range sum query to be answered in O(1) time.
Strategy 8: Bit Manipulation
In some cases, particularly for problems involving integers or sets, bit manipulation techniques can significantly reduce time complexity.
Example: Finding the Single Number
Consider a problem where you need to find a single number that appears only once in an array where every other number appears twice. A naive approach might use nested loops or sorting, resulting in O(n²) or O(n log n) time complexity. Here’s an O(n) solution using bit manipulation:
def find_single_number(nums):
result = 0
for num in nums:
result ^= num
return result
# Example usage
nums = [4, 1, 2, 1, 2]
print(find_single_number(nums)) # Output: 4
This solution uses the XOR operation’s properties to efficiently find the single number in O(n) time and O(1) space.
Strategy 9: Mathematical Approaches
Sometimes, mathematical insights can lead to dramatic improvements in time complexity.
Example: Counting Inversions
The problem of counting inversions in an array (pairs of elements where a[i] > a[j] for i
def count_inversions(arr):
def merge_and_count(left, right):
result = []
count = 0
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
count += len(left) - i
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result, count
def sort_and_count(arr):
if len(arr) <= 1:
return arr, 0
mid = len(arr) // 2
left, left_count = sort_and_count(arr[:mid])
right, right_count = sort_and_count(arr[mid:])
merged, merge_count = merge_and_count(left, right)
return merged, left_count + right_count + merge_count
_, inversion_count = sort_and_count(arr)
return inversion_count
# Example usage
arr = [8, 4, 2, 1]
print(count_inversions(arr)) # Output: 6
This approach leverages the merge step of merge sort to count inversions efficiently, reducing the time complexity from O(n²) to O(n log n).
Conclusion
Converting O(n²) solutions to O(n log n) or better is a crucial skill in algorithm design and optimization. The strategies we’ve explore divide and conquer, appropriate data structures, dynamic programming, sorting and binary search, preprocessing and hashing, sliding window technique, prefix sums, bit manipulation, and mathematical approaches provide a powerful toolkit for tackling complex problems efficiently.
Remember that the best approach often depends on the specific problem and constraints. Sometimes, a combination of these strategies might be necessary to achieve optimal performance. As you practice and apply these techniques, you’ll develop an intuition for recognizing patterns and choosing the most effective optimization strategy for each situation.
Continuous learning and practice are key to mastering these optimization techniques. Platforms like AlgoCademy offer a wealth of resources, interactive coding tutorials, and AI-powered assistance to help you hone your skills in algorithmic thinking and problem-solving. By applying these strategies and leveraging educational resources, you’ll be well-equipped to tackle complex coding challenges and excel in technical interviews at top tech companies.