Stop Overthinking and Start Writing: Why the First Draft of Your Code Doesn’t Matter
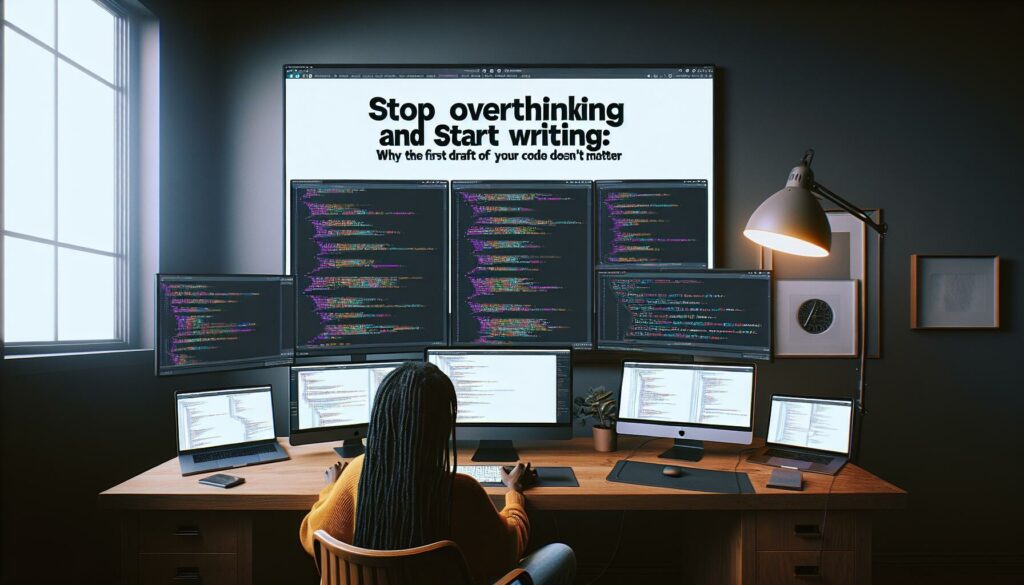
As aspiring programmers and even seasoned developers, we often find ourselves caught in the trap of perfectionism. We stare at the blank screen, cursor blinking, afraid to type that first line of code. Why? Because we’re convinced it needs to be perfect from the get-go. But here’s a revolutionary idea: the first draft of your code doesn’t matter. In fact, embracing imperfection in your initial coding attempts can be the key to unlocking your true potential as a programmer.
The Paralysis of Perfectionism
Perfectionism is a double-edged sword in the world of programming. While attention to detail and striving for excellence are admirable traits, they can also be crippling when taken to extremes. Many developers, especially those new to coding, fall into the trap of overthinking every line of code before they even begin typing.
This perfectionist mindset can lead to:
- Analysis paralysis: Spending hours planning without actually writing any code
- Fear of starting: Worrying that the code won’t be good enough from the beginning
- Procrastination: Putting off coding tasks because the perfect solution hasn’t been found yet
- Decreased productivity: Spending too much time on minor details instead of making progress
The truth is, no code is perfect on the first try. Even the most experienced developers write, rewrite, and refine their code multiple times before reaching a satisfactory result.
The Power of the First Draft
Instead of aiming for perfection, embrace the concept of the “first draft” in your coding process. Here’s why the initial version of your code is so valuable:
1. It Gets Ideas Flowing
Writing that first line of code, no matter how imperfect, breaks the ice and gets your creative juices flowing. Once you start typing, you’ll find that ideas come more easily, and you can build momentum.
2. It Provides a Starting Point
A rough first draft gives you something tangible to work with. It’s much easier to improve existing code than to create something from scratch. Having a foundation, even if it’s flawed, provides direction for your next steps.
3. It Helps Identify Problems Early
By writing a quick, imperfect version of your code, you can quickly identify potential issues or challenges that you might not have anticipated. This early problem detection can save you time and frustration in the long run.
4. It Encourages Experimentation
When you’re not focused on perfection, you’re more likely to try new approaches and experiment with different solutions. This experimentation is crucial for learning and growth as a programmer.
The Iterative Nature of Coding
Programming is an iterative process. The best code is rarely written in one go; it evolves through multiple revisions and refinements. Understanding this iterative nature can help alleviate the pressure to get everything right on the first try.
The Cycle of Improvement
A typical coding process might look something like this:
- Write a rough first draft
- Test the code and identify issues
- Refactor and optimize
- Repeat steps 2 and 3 until satisfied
Each iteration brings you closer to a better, more efficient solution. By embracing this cycle, you can focus on progress rather than perfection.
Strategies for Overcoming Perfectionism in Coding
If you find yourself struggling with perfectionism in your coding practice, try these strategies to help you get started and make progress:
1. Set Time Limits
Give yourself a specific amount of time to write the first draft of your code. This time pressure can help you focus on getting something done rather than getting it perfect.
2. Use Pseudocode
Start by writing pseudocode – a simplified, English-like description of your algorithm. This can help you outline your thoughts without worrying about syntax or implementation details.
3. Embrace the “Ugly” Solution
Allow yourself to write an “ugly” or inefficient solution first. The goal is to solve the problem; optimization can come later.
4. Comment Liberally
Use comments to explain your thought process and mark areas for improvement. This can help you move forward without getting stuck on perfecting every line.
5. Use Version Control
Utilize version control systems like Git to track changes and experiment freely. Knowing you can always revert to a previous version can give you the confidence to try new things.
Learning from Imperfection
Embracing imperfection in your first draft doesn’t mean settling for mediocrity. Instead, it’s about recognizing that imperfection is a natural part of the learning and development process. Here’s how you can learn from your imperfect code:
1. Analyze Your Mistakes
After writing your first draft, take time to analyze what worked and what didn’t. Understanding your mistakes is crucial for improvement.
2. Seek Feedback
Share your code with peers or mentors. Fresh eyes can provide valuable insights and alternative perspectives.
3. Compare Different Approaches
Once you have a working solution, research and compare it with other approaches. This can help you learn new techniques and best practices.
4. Reflect on Your Process
Consider how you approached the problem and what you might do differently next time. Continuous reflection leads to continuous improvement.
The Role of Practice in Improving Code Quality
While the first draft doesn’t need to be perfect, the goal is still to improve over time. Regular practice is key to enhancing your coding skills and producing better first drafts. Here are some ways to incorporate practice into your coding routine:
1. Daily Coding Challenges
Participate in daily coding challenges or exercises. Platforms like LeetCode, HackerRank, or AlgoCademy offer a variety of problems to solve.
2. Personal Projects
Work on personal projects that interest you. This self-motivated coding can help you apply your skills in practical scenarios.
3. Code Reviews
Participate in code reviews, either by reviewing others’ code or having your code reviewed. This process can expose you to different coding styles and best practices.
4. Pair Programming
Engage in pair programming sessions. Collaborating with others in real-time can help you learn new techniques and approaches.
Tools and Techniques for Improving Code Quality
While the first draft doesn’t need to be perfect, there are tools and techniques you can use to improve your code quality over time:
1. Linters and Code Formatters
Use tools like ESLint for JavaScript or Black for Python to automatically catch common errors and enforce consistent coding styles.
2. Integrated Development Environments (IDEs)
Utilize the features of modern IDEs, such as code completion, error highlighting, and refactoring tools, to improve your code as you write.
3. Testing Frameworks
Implement unit tests and integration tests to catch bugs early and ensure your code behaves as expected.
4. Code Documentation
Practice writing clear, concise documentation for your code. This helps you understand your own thought process and makes it easier for others to review and contribute to your code.
The Balance Between Speed and Quality
While we’ve emphasized the importance of not overthinking the first draft, it’s crucial to find a balance between speed and quality. The goal is to produce functional code quickly, but not at the expense of creating a mess that’s impossible to maintain or debug.
Tips for Balancing Speed and Quality:
- Write modular code: Break your code into smaller, reusable functions or classes.
- Follow naming conventions: Use clear, descriptive names for variables and functions.
- Keep it simple: Avoid unnecessary complexity. Aim for readability and simplicity in your first draft.
- Plan before you code: Spend a few minutes outlining your approach before diving in.
- Review as you go: Quickly review each function or module as you complete it.
Real-World Examples: From First Draft to Final Code
Let’s look at a real-world example of how code can evolve from a rough first draft to a more polished final version. We’ll use a simple problem: creating a function to find the maximum number in an array.
First Draft:
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
This first draft is functional but has room for improvement. It doesn’t handle edge cases, and the variable naming could be more descriptive.
Improved Version:
function findMaximum(numbers) {
if (!Array.isArray(numbers) || numbers.length === 0) {
throw new Error("Input must be a non-empty array of numbers");
}
let maximumNumber = numbers[0];
for (let i = 1; i < numbers.length; i++) {
if (typeof numbers[i] !== "number") {
throw new Error("All elements must be numbers");
}
if (numbers[i] > maximumNumber) {
maximumNumber = numbers[i];
}
}
return maximumNumber;
}
In this improved version, we’ve:
- Added input validation
- Used more descriptive variable names
- Included error handling for non-numeric elements
This example demonstrates how code can evolve from a basic implementation to a more robust and maintainable solution.
Overcoming Common Coding Challenges
As you work on your first drafts and subsequent iterations, you’re likely to encounter common coding challenges. Here are some strategies for overcoming them:
1. Debugging
When faced with bugs, don’t get discouraged. Use debugging tools provided by your IDE, add console logs, or use a step-by-step debugger to understand what’s happening in your code.
2. Optimizing Performance
If your code is slow, focus on identifying bottlenecks. Use profiling tools to find where your code spends the most time, and research more efficient algorithms or data structures.
3. Handling Edge Cases
As you refine your code, think about potential edge cases. What happens with empty inputs, extremely large numbers, or unexpected data types? Add tests and error handling for these scenarios.
4. Code Organization
If your code becomes unwieldy, consider refactoring. Break large functions into smaller, more manageable pieces. Use object-oriented principles or functional programming techniques to improve organization.
The Importance of Continuous Learning
Remember that becoming a proficient programmer is a journey of continuous learning. Each piece of code you write, whether it’s a rough first draft or a polished final version, is an opportunity to learn and improve.
Stay Updated
Keep up with the latest developments in your programming language and the broader tech industry. Follow blogs, attend webinars, or participate in coding communities to stay informed about new techniques and best practices.
Embrace New Challenges
Don’t shy away from tackling problems that seem difficult. Each challenge you overcome expands your skillset and builds your confidence as a developer.
Learn from Others
Study code written by experienced developers. Open-source projects can be a great resource for learning how to structure large codebases and seeing professional coding practices in action.
Conclusion: The Journey from First Draft to Mastery
In the world of programming, the journey from a rough first draft to polished, efficient code is where true learning and growth happen. By embracing imperfection in your initial attempts, you free yourself to explore, experiment, and ultimately improve as a developer.
Remember these key takeaways:
- The first draft is about getting your ideas down, not perfection.
- Iteration and refinement are natural parts of the coding process.
- Regular practice and continuous learning are crucial for improvement.
- Tools and techniques can help you enhance your code quality over time.
- Balance speed with maintainability in your coding approach.
So, the next time you find yourself staring at a blank screen, paralyzed by the desire for perfection, remember: the most important step is to start writing. Your first draft doesn’t have to be perfect; it just needs to exist. From there, you can refine, optimize, and transform your code into something truly remarkable.
Embrace the journey from first draft to mastery, and watch as your skills as a programmer grow with each line of code you write. Happy coding!