Stop Focusing on Code and Start Thinking Like an Engineer
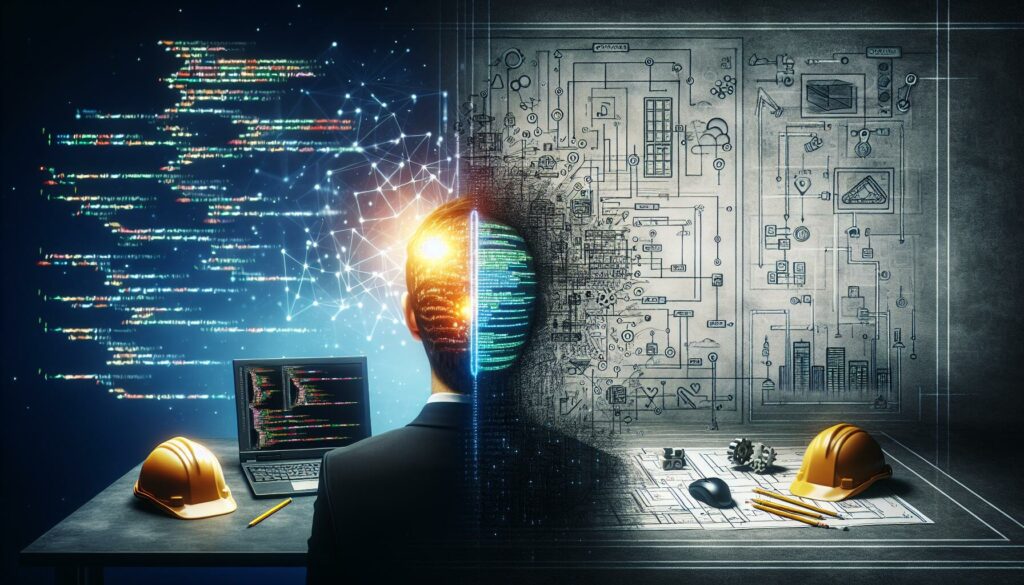
In the world of programming and software development, it’s easy to get caught up in the minutiae of coding. Syntax, language specifics, and the latest frameworks often dominate discussions and learning resources. However, true success in the field of software engineering requires much more than just writing code. It demands a shift in mindset – from that of a coder to that of an engineer. This article will explore why it’s crucial to stop focusing solely on code and start thinking like an engineer, especially for those aiming to excel in their careers and stand out in technical interviews at top tech companies.
The Limitations of a Code-Centric Approach
Before we delve into the engineering mindset, let’s consider the limitations of a purely code-focused approach:
- Tunnel Vision: Concentrating only on code can lead to missing the bigger picture of software development.
- Lack of Problem-Solving Skills: Coding without understanding the underlying problems can result in inefficient or inappropriate solutions.
- Limited Career Growth: While coding skills are important, they alone are not sufficient for advancing to senior roles in software engineering.
- Difficulty in Interviews: Top tech companies often look for problem-solving abilities and system design skills rather than just coding prowess.
What Does It Mean to Think Like an Engineer?
Engineering thinking encompasses a broader set of skills and approaches:
- Problem-Solving Orientation: Engineers focus on understanding and solving problems, not just implementing solutions.
- Systems Thinking: They consider how individual components interact within larger systems.
- Efficiency and Optimization: Engineers constantly seek ways to improve performance and reduce resource usage.
- Scalability Considerations: They design solutions that can grow and adapt to changing needs.
- Trade-off Analysis: Engineers weigh pros and cons to make informed decisions about design and implementation choices.
Key Aspects of Engineering Thinking
1. Problem Definition and Analysis
Before writing a single line of code, an engineer thoroughly analyzes the problem at hand. This involves:
- Clearly defining the problem statement
- Identifying constraints and requirements
- Breaking down complex problems into smaller, manageable parts
- Considering various approaches and their implications
For example, when faced with a task to implement a search functionality, an engineer wouldn’t immediately jump into coding. Instead, they would ask questions like:
- What type of data are we searching?
- How large is the dataset?
- What are the performance requirements?
- Are there any specific constraints (e.g., memory limitations)?
2. Algorithmic Thinking
While coding skills are important, the ability to think algorithmically is crucial. This involves:
- Understanding various algorithmic paradigms (e.g., divide and conquer, dynamic programming)
- Analyzing time and space complexity
- Choosing the most appropriate algorithm for a given problem
- Optimizing algorithms for specific use cases
Consider the following example of finding the nth Fibonacci number:
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
def fibonacci_dynamic(n):
if n <= 1:
return n
fib = [0] * (n+1)
fib[1] = 1
for i in range(2, n+1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
An engineer would not only implement these solutions but also analyze their efficiency:
- The recursive solution has a time complexity of O(2^n), which is highly inefficient for large n.
- The dynamic programming approach has a time complexity of O(n) and space complexity of O(n), making it much more efficient for larger values of n.
3. System Design and Architecture
Engineers think beyond individual components and consider the overall system architecture. This includes:
- Designing scalable and maintainable systems
- Choosing appropriate data structures and databases
- Considering distributed systems and microservices architectures
- Planning for future growth and changes
For instance, when designing a social media platform, an engineer would consider:
- How to store and retrieve user data efficiently
- Implementing a scalable newsfeed algorithm
- Designing a robust notification system
- Planning for data replication and sharding for increased user base
4. Performance Optimization
Optimizing performance is a key aspect of engineering thinking. This involves:
- Profiling and identifying bottlenecks
- Optimizing algorithms and data structures
- Implementing caching strategies
- Considering trade-offs between time and space complexity
Let’s look at an example of optimizing a function that checks if a number is prime:
def is_prime_naive(n):
if n < 2:
return False
for i in range(2, n):
if n % i == 0:
return False
return True
def is_prime_optimized(n):
if n < 2:
return False
if n == 2:
return True
if n % 2 == 0:
return False
for i in range(3, int(n**0.5) + 1, 2):
if n % i == 0:
return False
return True
An engineer would optimize this function by:
- Checking only up to the square root of n, reducing time complexity from O(n) to O(√n)
- Eliminating even numbers from the check, further reducing the number of iterations
5. Testing and Debugging
Engineering thinking emphasizes thorough testing and effective debugging:
- Implementing unit tests and integration tests
- Conducting edge case analysis
- Using debugging tools and techniques effectively
- Implementing logging and monitoring for production systems
For example, when implementing a sorting algorithm, an engineer would consider test cases such as:
- An already sorted array
- A reverse-sorted array
- An array with all identical elements
- An array with a single element
- An empty array
Applying Engineering Thinking in Technical Interviews
For those preparing for technical interviews, especially at top tech companies, adopting an engineering mindset is crucial. Here’s how to apply this thinking during interviews:
1. Problem Understanding
Before diving into coding:
- Ask clarifying questions about the problem
- Discuss potential edge cases and constraints
- Consider the scale of the problem (e.g., size of input, frequency of operations)
2. Solution Design
Before implementation:
- Discuss multiple approaches to solving the problem
- Analyze the time and space complexity of each approach
- Consider trade-offs between different solutions
- Choose the most appropriate solution based on the problem constraints
3. Implementation
While coding:
- Write clean, modular, and readable code
- Consider edge cases in your implementation
- Optimize your solution where possible
4. Testing and Refinement
After implementation:
- Walk through your code with test cases
- Discuss potential optimizations or improvements
- Consider how your solution would scale or adapt to changing requirements
Developing Engineering Thinking Skills
To cultivate an engineering mindset:
1. Practice Problem-Solving
Regularly engage with coding challenges and algorithmic problems. Platforms like AlgoCademy offer a wide range of problems to practice:
- Start with easier problems and gradually increase difficulty
- Focus on understanding the problem-solving approach rather than just the solution
- Analyze multiple solutions for each problem
2. Study System Design
Familiarize yourself with system design concepts:
- Read about architectures of popular systems and applications
- Practice designing systems on paper or whiteboard
- Discuss system design with peers or in online forums
3. Analyze Existing Code and Systems
Regularly review and analyze code and systems:
- Contribute to open-source projects
- Participate in code reviews
- Analyze the architecture of applications you use daily
4. Broaden Your Knowledge
Expand your understanding beyond just programming:
- Study computer science fundamentals (data structures, algorithms, operating systems)
- Learn about software development methodologies
- Stay updated with industry trends and new technologies
5. Work on Projects
Apply your skills to real-world projects:
- Develop personal projects from scratch
- Contribute to open-source projects
- Participate in hackathons or coding competitions
The Role of Platforms Like AlgoCademy
Platforms like AlgoCademy play a crucial role in developing engineering thinking:
- Structured Learning: They provide a structured approach to learning algorithms and data structures, essential for developing algorithmic thinking.
- Problem Variety: A wide range of problems helps in understanding different problem-solving paradigms.
- Interactive Tutorials: Step-by-step guidance helps in understanding the thought process behind solutions.
- AI-Powered Assistance: Personalized hints and feedback can help learners develop problem-solving skills at their own pace.
- Interview Preparation: Focused resources for technical interview preparation help in applying engineering thinking to interview scenarios.
Conclusion
While coding skills are undoubtedly important, thinking like an engineer is what truly sets apart exceptional software professionals. By shifting focus from merely writing code to understanding problems, designing efficient solutions, and considering broader system implications, developers can significantly enhance their problem-solving abilities and career prospects.
For those aiming to excel in technical interviews and build successful careers in software engineering, particularly at top tech companies, developing this engineering mindset is crucial. It’s not just about knowing how to code; it’s about understanding why we code and how to approach complex problems systematically.
Platforms like AlgoCademy provide valuable resources and structured approaches to help develop these skills. However, the journey to thinking like an engineer is ongoing and requires continuous learning, practice, and application of knowledge to real-world problems.
Remember, the goal is not just to write code, but to solve problems effectively and create robust, scalable solutions. By adopting an engineering mindset, you’ll not only improve your coding skills but also elevate your ability to tackle complex challenges in the ever-evolving field of software development.