Step-by-Step Guide to Writing Perfect Code in Your Coding Interview
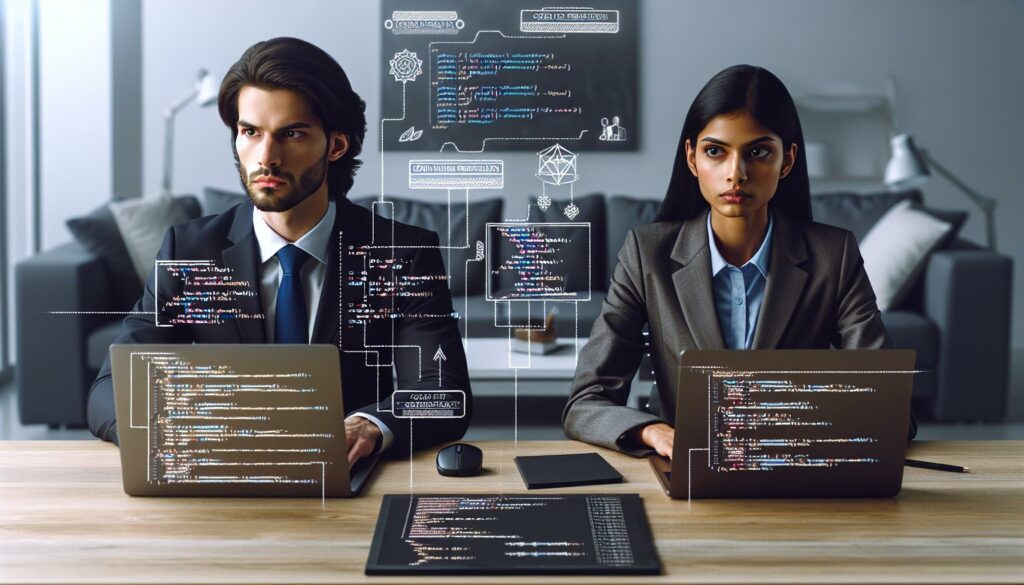
Coding interviews can be nerve-wracking experiences, especially when you’re trying to showcase your skills under pressure. Whether you’re interviewing for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech firm, the ability to write clean, efficient, and bug-free code is crucial. In this comprehensive guide, we’ll walk you through a step-by-step process to help you excel in your coding interview and produce high-quality code that will impress your interviewers.
1. Understand the Problem
Before you start writing any code, it’s essential to fully grasp the problem at hand. This step is crucial and often overlooked by nervous candidates eager to start coding immediately.
Steps to understand the problem:
- Listen carefully to the interviewer’s description of the problem.
- Ask clarifying questions to ensure you understand all requirements and constraints.
- Repeat the problem back to the interviewer in your own words to confirm your understanding.
- Identify the input and expected output of the problem.
- Discuss any edge cases or potential assumptions with the interviewer.
For example, if you’re asked to implement a function that finds the maximum subarray sum, you might ask:
- Can the array contain negative numbers?
- What should be returned if the array is empty?
- Is there a constraint on the array size or the range of numbers?
2. Plan Your Approach
Once you have a clear understanding of the problem, take a moment to plan your approach. This step involves thinking through the problem logically and considering different algorithms or data structures that might be useful.
Steps to plan your approach:
- Break down the problem into smaller, manageable steps.
- Consider multiple approaches and their trade-offs.
- Think about time and space complexity.
- Sketch out a high-level algorithm or flowchart.
- Discuss your thought process with the interviewer.
For our maximum subarray sum example, you might consider:
- A brute force approach (checking all subarrays)
- Kadane’s algorithm (dynamic programming approach)
- Divide and conquer approach
Discussing these options with your interviewer shows your problem-solving skills and ability to consider multiple solutions.
3. Write Pseudocode
Before diving into actual code, it’s often helpful to write pseudocode. This step allows you to organize your thoughts and create a blueprint for your solution without getting bogged down in syntax details.
Benefits of writing pseudocode:
- Helps clarify your thinking and approach
- Allows you to focus on the logic without syntax distractions
- Provides a roadmap for your actual code implementation
- Makes it easier to explain your approach to the interviewer
Here’s an example of pseudocode for Kadane’s algorithm to solve the maximum subarray sum problem:
function maxSubarraySum(arr):
max_sum = current_sum = arr[0]
for each element in arr (starting from the second element):
current_sum = max(element, current_sum + element)
max_sum = max(max_sum, current_sum)
return max_sum
4. Implement the Solution
Now that you have a clear plan and pseudocode, it’s time to implement your solution in actual code. During this step, focus on writing clean, readable, and efficient code.
Tips for implementing your solution:
- Choose meaningful variable and function names
- Use consistent indentation and formatting
- Break down complex logic into smaller, reusable functions
- Comment your code where necessary, especially for complex parts
- Consider edge cases as you code
Here’s an example implementation of the maximum subarray sum problem in Python:
def max_subarray_sum(arr):
if not arr:
return 0 # Handle empty array case
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
result = max_subarray_sum(arr)
print(f"Maximum subarray sum: {result}") # Output: Maximum subarray sum: 6
5. Test Your Code
After implementing your solution, it’s crucial to test your code thoroughly. This step helps catch bugs and demonstrates your attention to detail and commitment to quality.
Steps for testing your code:
- Start with simple test cases to verify basic functionality
- Test edge cases (e.g., empty input, single element, all negative numbers)
- Use larger or more complex inputs to check for efficiency
- Consider corner cases that might break your code
- Walk through your code step-by-step with a sample input
For our maximum subarray sum example, you might test the following cases:
def test_max_subarray_sum():
assert max_subarray_sum([]) == 0
assert max_subarray_sum([-1]) == -1
assert max_subarray_sum([1, 2, 3]) == 6
assert max_subarray_sum([-2, 1, -3, 4, -1, 2, 1, -5, 4]) == 6
assert max_subarray_sum([-1, -2, -3]) == -1
print("All test cases passed!")
test_max_subarray_sum()
6. Optimize Your Solution
Once you have a working solution, consider if there are any optimizations you can make. This step shows your ability to think critically about efficiency and demonstrates your knowledge of advanced programming concepts.
Ways to optimize your solution:
- Analyze the time and space complexity of your current solution
- Consider using more efficient data structures or algorithms
- Look for redundant calculations or unnecessary operations
- Discuss potential trade-offs between time and space complexity
- Consider preprocessing or caching techniques if applicable
In our maximum subarray sum example, the Kadane’s algorithm solution is already optimal with O(n) time complexity and O(1) space complexity. However, you could discuss potential optimizations for other problems or variations of this problem.
7. Explain Your Approach and Code
Throughout the interview process, it’s important to communicate your thoughts clearly. Explaining your approach and walking through your code helps the interviewer understand your problem-solving process and coding style.
Tips for explaining your code:
- Provide a high-level overview of your approach
- Explain the key steps in your algorithm
- Discuss the time and space complexity of your solution
- Highlight any important design decisions or trade-offs
- Be prepared to explain any specific lines of code if asked
For example, you might explain the maximum subarray sum solution like this:
“I’ve implemented Kadane’s algorithm to solve this problem. The key idea is to maintain two variables: `max_sum` for the overall maximum sum, and `current_sum` for the maximum sum ending at the current position. We iterate through the array once, updating these variables at each step. The time complexity is O(n) since we make a single pass through the array, and the space complexity is O(1) as we only use a constant amount of extra space.”
8. Handle Follow-up Questions
After presenting your solution, be prepared for follow-up questions. Interviewers often ask these to assess your depth of understanding and ability to think on your feet.
Common types of follow-up questions:
- How would you modify your solution to handle a different constraint?
- Can you think of any alternative approaches to solve this problem?
- How would your solution scale with larger inputs?
- What if the input was coming from a stream or was too large to fit in memory?
- How would you parallelize your algorithm if possible?
For the maximum subarray sum problem, a follow-up question might be:
“How would you modify your solution to return the start and end indices of the maximum subarray in addition to the sum?”
To answer this, you could modify your code as follows:
def max_subarray_sum_with_indices(arr):
if not arr:
return 0, -1, -1 # Sum, start index, end index for empty array
max_sum = current_sum = arr[0]
max_start = max_end = current_start = 0
for i, num in enumerate(arr[1:], 1):
if num > current_sum + num:
current_sum = num
current_start = i
else:
current_sum += num
if current_sum > max_sum:
max_sum = current_sum
max_start = current_start
max_end = i
return max_sum, max_start, max_end
# Example usage
arr = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
result, start, end = max_subarray_sum_with_indices(arr)
print(f"Maximum subarray sum: {result}, from index {start} to {end}")
# Output: Maximum subarray sum: 6, from index 3 to 6
9. Reflect on Your Performance
After the interview, take some time to reflect on your performance. This step is crucial for continuous improvement and preparing for future interviews.
Questions to ask yourself:
- What parts of the interview did I handle well?
- Were there any areas where I struggled or could improve?
- Did I communicate my thoughts clearly throughout the process?
- Were there any concepts or algorithms I need to review?
- How can I better prepare for similar questions in the future?
Consider keeping a journal of your interview experiences, noting down the problems you encountered, your approaches, and areas for improvement. This can be an invaluable resource for future preparation.
10. Practice, Practice, Practice
The key to excelling in coding interviews is consistent practice. The more you practice, the more comfortable and confident you’ll become in tackling a wide range of problems under pressure.
Ways to practice coding interview skills:
- Solve problems on platforms like LeetCode, HackerRank, or CodeSignal
- Participate in coding competitions or hackathons
- Contribute to open-source projects
- Conduct mock interviews with friends or use services like Pramp
- Review and implement common data structures and algorithms
Remember to time yourself when practicing to simulate interview conditions and work on explaining your thought process out loud as you code.
Conclusion
Writing perfect code in a coding interview is a skill that can be developed with practice and the right approach. By following this step-by-step guide, you’ll be well-equipped to tackle coding challenges with confidence and precision. Remember to understand the problem thoroughly, plan your approach, write clean and efficient code, test rigorously, and communicate clearly throughout the process.
Keep in mind that interviewers are not just looking for the correct solution, but also assessing your problem-solving skills, coding style, and ability to think critically under pressure. By demonstrating these qualities along with your technical skills, you’ll greatly increase your chances of success in coding interviews.
As you continue to practice and refine your skills, you’ll find that the process becomes more natural and less stressful. Embrace each interview as an opportunity to learn and grow, and don’t be discouraged by setbacks. With persistence and dedication, you’ll be well on your way to mastering the art of coding interviews and landing your dream job in the tech industry.