Start Writing Bad Code: The Secret to Conquering Blank Page Syndrome
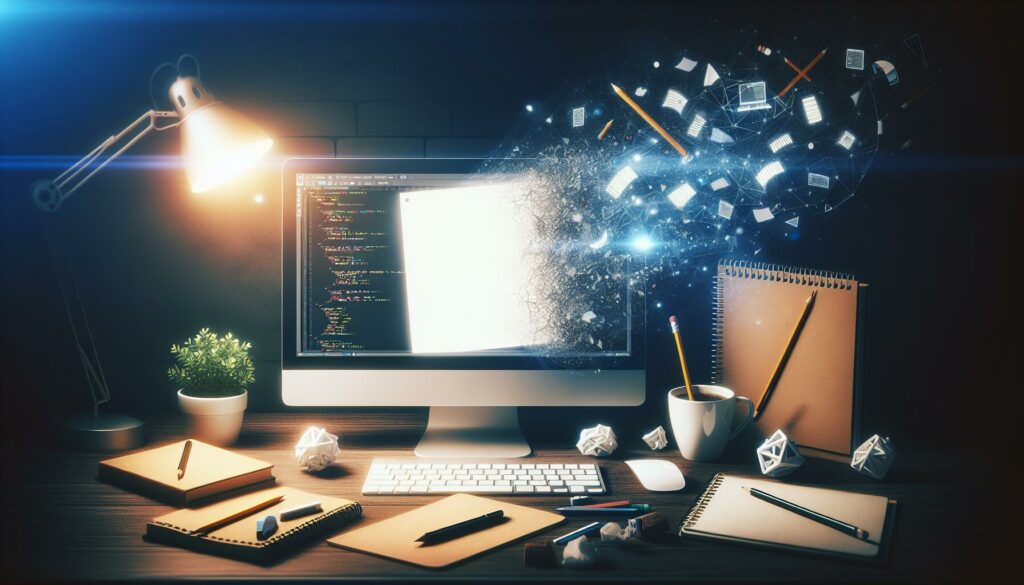
As programmers, we’ve all faced that dreaded moment: staring at a blank screen, cursor blinking mockingly, as we struggle to write that first line of code. This phenomenon, often referred to as “Blank Page Syndrome,” can be paralyzing, especially for beginners. But what if I told you that the secret to overcoming this hurdle is to start by writing bad code? Yes, you read that right. In this article, we’ll explore why embracing imperfection can be the key to unlocking your coding potential and how this approach aligns with the principles of continuous learning and improvement that platforms like AlgoCademy champion.
The Paralysis of Perfection
Before we dive into the benefits of writing bad code, let’s understand why Blank Page Syndrome occurs in the first place. Often, it’s rooted in our desire for perfection. We want our code to be elegant, efficient, and bug-free from the get-go. This perfectionism, while admirable, can be incredibly counterproductive, especially when you’re just starting out or tackling a new problem.
The fear of writing imperfect code can lead to:
- Procrastination
- Overthinking simple problems
- Decreased confidence in your coding abilities
- Slower progress in learning and project completion
Recognizing these pitfalls is the first step towards overcoming them. And that’s where the “write bad code” approach comes in.
The Power of Bad Code
Writing bad code might seem counterintuitive, especially when platforms like AlgoCademy emphasize the importance of algorithmic thinking and efficient problem-solving. However, this approach is not about deliberately writing poor-quality code that you’ll use in production. Instead, it’s about giving yourself permission to write imperfect, messy code as a starting point.
Here’s why this approach can be so powerful:
1. It Gets You Started
The hardest part of coding is often just getting started. By allowing yourself to write bad code, you remove the pressure of perfection and simply begin. Once you have something on the screen, no matter how crude, you’ve overcome the initial hurdle.
2. It Builds Momentum
As you start writing, even if it’s not great code, you’ll find that ideas begin to flow more freely. This momentum can carry you through the initial stages of problem-solving and help you maintain focus.
3. It Provides a Foundation for Improvement
Bad code gives you something concrete to improve upon. It’s much easier to refactor and optimize existing code than to create perfect code from scratch.
4. It Encourages Experimentation
When you’re not worried about writing perfect code, you’re more likely to try new approaches and think outside the box. This experimentation is crucial for learning and innovation.
Implementing the “Write Bad Code” Approach
Now that we understand the benefits, let’s look at how to implement this approach effectively:
1. Set a Time Limit
Give yourself a specific amount of time (e.g., 15-30 minutes) to write as much code as possible without worrying about quality. This time pressure can help override your perfectionist tendencies.
2. Focus on Functionality First
Aim to get the basic functionality working before you worry about optimization. If you’re solving a problem on AlgoCademy, for instance, focus on getting a solution that passes the test cases, even if it’s not the most efficient.
3. Use Pseudocode
If you’re really struggling to get started, begin by writing pseudocode. This can help you outline your logic without getting bogged down in syntax.
4. Embrace Comments
Use comments liberally to explain your thought process, even (or especially) when your code is messy. This will help you when you come back to refactor.
5. Refactor in Stages
Once you have a working solution, refactor your code in stages. Start with the most glaring issues and work your way towards optimization.
A Practical Example
Let’s look at a practical example of how this approach might work. Suppose you’re tackling a classic problem: reversing a string. Here’s how you might approach it using the “write bad code” method:
Step 1: Write Bad Code (Time Limit: 5 minutes)
// Function to reverse a string
function reverseString(str) {
// Convert string to array
let arr = str.split("");
// Create a new array to store reversed characters
let reversedArr = [];
// Loop through the original array backwards
for (let i = arr.length - 1; i >= 0; i--) {
// Add each character to the new array
reversedArr.push(arr[i]);
}
// Join the reversed array back into a string
let reversedStr = reversedArr.join("");
// Return the reversed string
return reversedStr;
}
// Test the function
console.log(reverseString("hello")); // should output "olleh"
This code works, but it’s not very efficient. It creates unnecessary arrays and uses a loop when there are simpler methods available. However, the important thing is that we have a working solution to build upon.
Step 2: Refactor (Time Limit: 10 minutes)
function reverseString(str) {
return str.split("").reverse().join("");
}
console.log(reverseString("hello")); // outputs "olleh"
This refactored version is much more concise and efficient. We’ve eliminated the unnecessary loop and extra array, using built-in JavaScript methods instead.
Step 3: Optimize (If Needed)
For this simple example, our refactored version is probably sufficient. However, if we were dealing with very long strings or needed to optimize for performance, we might consider a more efficient approach:
function reverseString(str) {
let reversed = "";
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
console.log(reverseString("hello")); // outputs "olleh"
This version avoids the overhead of creating arrays and uses a single loop to build the reversed string.
Applying This Approach to AlgoCademy Challenges
The “write bad code” approach can be particularly effective when tackling challenges on platforms like AlgoCademy. Here’s how you can apply it:
1. Read and Understand the Problem
Take the time to thoroughly read and understand the problem statement. Make sure you grasp what the expected input and output should be.
2. Write a Quick and Dirty Solution
Don’t worry about efficiency or elegance at first. Focus on writing a solution that correctly solves the problem, even if it’s not optimal.
3. Test Your Solution
Use AlgoCademy’s testing features to check if your solution passes the basic test cases. If it doesn’t, debug and adjust your code until it does.
4. Analyze and Refactor
Once you have a working solution, take a step back and analyze your code. Look for areas where you can improve efficiency, readability, or both.
5. Learn from Model Solutions
After you’ve submitted your solution, take the time to study the model solutions provided by AlgoCademy. Compare these to your own code and try to understand the different approaches and optimizations used.
Overcoming Common Obstacles
Even with the “write bad code” approach, you might still encounter some obstacles. Here’s how to overcome them:
1. “I don’t know where to start”
Solution: Break the problem down into smaller steps. Write pseudocode for each step, then tackle them one at a time.
2. “My code is too messy”
Solution: Remember, the goal is to get something working first. You can always clean it up later. Use comments to explain your logic if the code itself is unclear.
3. “I’m stuck on a particular part”
Solution: Skip it for now and move on to other parts of the problem. You can come back to it later with fresh eyes.
4. “I’m worried my approach is completely wrong”
Solution: It’s okay if your approach isn’t perfect. Implement it anyway. You’ll learn more from seeing why it doesn’t work than from not trying at all.
The Psychology of Embracing Imperfection
Embracing the “write bad code” approach requires a shift in mindset. It’s about valuing progress over perfection and recognizing that every line of code you write, good or bad, is an opportunity to learn.
This mindset aligns well with the growth mindset that’s crucial for success in programming. It encourages:
- Resilience in the face of challenges
- A willingness to make and learn from mistakes
- Continuous improvement rather than expecting instant mastery
- Reduced anxiety and increased enjoyment of the coding process
By allowing yourself to write bad code, you’re not lowering your standards. Instead, you’re creating a safe space for experimentation and learning, which ultimately leads to better code in the long run.
Balancing Bad Code with Best Practices
While the “write bad code” approach is a powerful tool for overcoming Blank Page Syndrome and making progress, it’s important to balance this with an understanding of best practices and coding standards. Here’s how to strike that balance:
1. Use Bad Code as a Starting Point, Not an End Goal
Remember that the purpose of writing bad code is to get started and generate ideas. Always plan to refactor and improve your code once you have a working solution.
2. Learn from Your Mistakes
As you refactor your “bad” code, pay attention to the improvements you’re making. Understanding why certain approaches are better will help you write better code in the future.
3. Study Good Code
Take time to study well-written, efficient code. Platforms like AlgoCademy often provide model solutions to problems. Analyze these to understand what makes them effective.
4. Practice Deliberate Improvement
After writing your initial “bad” code, challenge yourself to improve specific aspects in each iteration. For example, focus on improving time complexity in one round, then space complexity in another.
5. Seek Feedback
Share your code with peers or mentors. Getting constructive feedback on both your initial attempts and your refactored versions can provide valuable insights.
Leveraging AlgoCademy’s Features for Improvement
AlgoCademy and similar platforms offer various features that can support your journey from writing bad code to mastering efficient, elegant solutions:
1. Step-by-Step Guidance
Use the platform’s guided approach to break down complex problems into manageable steps. This can help you structure your “bad” code in a way that’s easier to refactor later.
2. AI-Powered Assistance
Take advantage of AI-powered hints and suggestions. These can help you identify areas for improvement in your code and suggest more efficient approaches.
3. Performance Metrics
Pay attention to the performance metrics provided for your solutions. Use these as benchmarks to gauge the effectiveness of your refactoring efforts.
4. Community Forums
Engage with the community forums to discuss different approaches to problems. Seeing how others tackle the same challenges can broaden your perspective and improve your problem-solving skills.
5. Skill Tracking
Use the platform’s skill tracking features to identify areas where you consistently struggle. This can help you focus your learning efforts and track your improvement over time.
Conclusion: Embracing the Journey from Bad to Better
The “Start Writing Bad Code” approach is more than just a trick to overcome Blank Page Syndrome. It’s a philosophy that embraces the iterative nature of coding and aligns perfectly with the learning journey that platforms like AlgoCademy support.
By giving yourself permission to write bad code, you’re:
- Overcoming the paralysis of perfectionism
- Building momentum in your coding practice
- Creating opportunities for learning and improvement
- Developing resilience and a growth mindset
Remember, every expert programmer started by writing bad code. The key is to keep writing, keep learning, and keep improving. Embrace the process, use the resources available to you, and don’t be afraid to make mistakes. With each line of code you write, whether it’s “bad” or “good,” you’re taking another step on your journey to becoming a skilled programmer.
So the next time you’re faced with a blank screen and a coding challenge, take a deep breath and start writing. Your first attempt might not be perfect, but it will be the beginning of something great. Happy coding!