Stack vs Heap: Understanding Memory Allocation in Programming
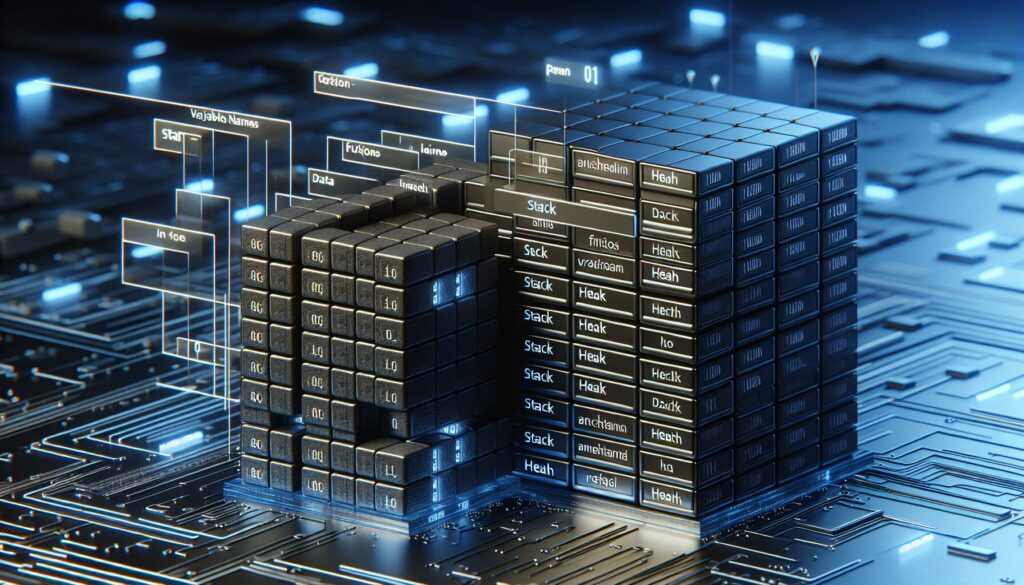
When it comes to programming, understanding how memory is allocated and managed is crucial for writing efficient and bug-free code. Two fundamental concepts in memory management are the stack and the heap. In this comprehensive guide, we’ll dive deep into the differences between stack and heap memory, their characteristics, and when to use each. By the end of this article, you’ll have a solid grasp of these concepts, which will prove invaluable as you progress in your coding journey and prepare for technical interviews at top tech companies.
Table of Contents
- What is Memory in Programming?
- Stack Memory: The Fast and Furious
- Heap Memory: The Flexible Powerhouse
- Stack vs Heap: Key Differences
- When to Use Stack vs Heap
- Memory Management in Different Programming Languages
- Common Issues and Best Practices
- Stack and Heap in Technical Interviews
- Conclusion
1. What is Memory in Programming?
Before we dive into the specifics of stack and heap, let’s briefly discuss what memory means in the context of programming. In computer science, memory refers to the physical devices used to store programs and data on a temporary or permanent basis. When we talk about memory in relation to program execution, we’re typically referring to Random Access Memory (RAM).
RAM is divided into different sections, each serving a specific purpose. Two of the most important sections for programmers to understand are the stack and the heap. These areas of memory are used to store data during program execution, but they operate in fundamentally different ways.
2. Stack Memory: The Fast and Furious
The stack is a region of memory that follows a Last-In-First-Out (LIFO) structure. Think of it like a stack of plates: the last plate you put on top is the first one you’ll take off. In programming, the stack is used to store local variables, function parameters, and return addresses.
Key Characteristics of Stack Memory:
- Fast Access: Stack memory allocation and deallocation are very fast operations.
- Limited Size: The stack has a fixed size, which is typically determined at the start of the program.
- Automatic Memory Management: Variables are automatically allocated and deallocated as functions are called and return.
- Scope-Based: Variables on the stack have a limited scope, typically within the function they’re declared in.
- Value Types: Primitive data types and value types are usually stored on the stack.
Example of Stack Memory Usage:
Let’s look at a simple C++ function to illustrate stack memory usage:
int calculateSum(int a, int b) {
int result = a + b;
return result;
}
int main() {
int x = 5;
int y = 10;
int sum = calculateSum(x, y);
return 0;
}
In this example, the variables x
, y
, and sum
in the main
function, as well as a
, b
, and result
in the calculateSum
function, are all stored on the stack. When calculateSum
is called, a new stack frame is created for its local variables, and when it returns, that frame is automatically removed.
3. Heap Memory: The Flexible Powerhouse
The heap is a region of memory used for dynamic memory allocation. Unlike the stack, which has a rigid structure, the heap is more flexible and can be accessed in any order. The heap is used for storing objects and data structures whose size can change during runtime.
Key Characteristics of Heap Memory:
- Dynamic Allocation: Memory can be allocated and deallocated at any time during program execution.
- Larger Size: The heap can typically hold much more data than the stack.
- Slower Access: Allocating and freeing memory on the heap is slower compared to stack operations.
- Manual Memory Management: In some languages, the programmer is responsible for freeing heap memory to prevent memory leaks.
- Global Access: Objects on the heap can be accessed from any part of the program.
- Reference Types: Objects and reference types are usually stored on the heap.
Example of Heap Memory Usage:
Here’s a C++ example demonstrating heap memory allocation:
class Person {
public:
Person(std::string name, int age) : name(name), age(age) {}
std::string name;
int age;
};
int main() {
Person* john = new Person("John Doe", 30);
// Use john...
delete john; // Remember to free the memory!
return 0;
}
In this example, the Person
object is created on the heap using the new
keyword. It’s important to note that in C++, we need to manually free this memory using delete
to avoid memory leaks.
4. Stack vs Heap: Key Differences
Now that we’ve explored both stack and heap memory, let’s summarize the key differences between them:
Aspect | Stack | Heap |
---|---|---|
Allocation/Deallocation | Automatic | Manual (in some languages) |
Speed | Fast | Slower |
Size | Limited | Larger |
Fragmentation | No fragmentation | Can become fragmented |
Access | LIFO order | Any order |
Typical Usage | Local variables, function calls | Dynamic data structures, objects |
5. When to Use Stack vs Heap
Choosing between stack and heap memory depends on various factors. Here are some guidelines to help you decide:
Use Stack When:
- You have small, fixed-size data.
- You need fast allocation and deallocation.
- You’re working with local variables that don’t need to persist beyond their scope.
- You’re dealing with value types or primitives.
- You want to avoid the overhead of memory management.
Use Heap When:
- You need to allocate a large block of memory.
- You’re working with data whose size can change.
- You need the data to persist beyond the scope of the current function.
- You’re dealing with complex objects or data structures.
- You need to share data between different parts of your program.
6. Memory Management in Different Programming Languages
Different programming languages handle memory management in various ways, particularly when it comes to heap memory. Let’s look at how some popular languages approach this:
C and C++
These languages give programmers direct control over memory allocation and deallocation. You use malloc()
or new
to allocate memory on the heap and free()
or delete
to deallocate it. This level of control can lead to efficient programs but also introduces the risk of memory leaks and dangling pointers if not managed carefully.
Java
Java uses automatic memory management through garbage collection. Objects are allocated on the heap, and the garbage collector automatically frees memory that’s no longer being used. While this reduces the risk of memory leaks, it can introduce performance overhead.
Python
Python also uses garbage collection for memory management. It handles memory allocation and deallocation behind the scenes, allowing developers to focus on writing code without worrying about explicit memory management.
Rust
Rust takes a unique approach with its ownership system. It ensures memory safety without a garbage collector by enforcing strict rules about how memory is allocated and used. This approach provides the efficiency of manual memory management with the safety of automatic management.
7. Common Issues and Best Practices
Understanding stack and heap memory is crucial for avoiding common programming pitfalls and optimizing your code. Here are some issues to be aware of and best practices to follow:
Stack Overflow
This occurs when the call stack exceeds its limit, often due to excessive recursion or allocating too many large objects on the stack. To avoid this:
- Be cautious with recursive functions and ensure they have proper base cases.
- Consider using heap allocation for large objects or data structures.
- Be aware of your program’s stack size limits, which can vary by platform and compiler settings.
Memory Leaks
Memory leaks happen when allocated heap memory is not properly freed, leading to gradual memory consumption. To prevent this:
- Always free allocated memory when it’s no longer needed (in languages that require manual management).
- Use smart pointers in C++ to automate memory management.
- Be cautious with circular references in garbage-collected languages, as they can prevent objects from being collected.
Fragmentation
Heap fragmentation occurs when free memory is split into many small chunks, making it difficult to allocate large contiguous blocks. To mitigate this:
- Try to allocate memory in consistent sizes when possible.
- Consider using memory pools for frequently allocated objects of the same size.
- In some cases, periodically “defragmenting” your heap by reallocating and compacting objects can help.
Best Practices
- Prefer stack allocation for small, short-lived objects.
- Use heap allocation judiciously, only when necessary.
- In languages with manual memory management, follow the RAII (Resource Acquisition Is Initialization) principle.
- Use appropriate data structures to minimize unnecessary memory usage.
- Profile your application to identify memory bottlenecks and optimize accordingly.
8. Stack and Heap in Technical Interviews
Understanding stack and heap memory is crucial for technical interviews, especially when applying to top tech companies. Here are some common interview questions and scenarios related to stack and heap:
Sample Interview Questions:
- What’s the difference between stack and heap memory?
- When would you choose to allocate memory on the heap instead of the stack?
- How does a stack overflow occur, and how can it be prevented?
- Explain how memory leaks can happen and strategies to avoid them.
- How does garbage collection work, and what are its pros and cons?
- In C++, what’s the difference between
new
andmalloc()
? - How does Rust’s ownership system differ from traditional memory management approaches?
Coding Challenges:
Interviewers might also present coding challenges that test your understanding of memory management. For example:
- Implementing a memory-efficient data structure
- Optimizing an algorithm to reduce memory usage
- Debugging code with memory leaks or stack overflow issues
- Writing a custom memory allocator
To prepare for these types of questions and challenges:
- Practice implementing data structures from scratch, paying attention to memory usage.
- Study common memory-related issues and how to diagnose them.
- Familiarize yourself with memory profiling tools.
- Review language-specific memory management features and best practices.
9. Conclusion
Understanding the differences between stack and heap memory is fundamental to becoming a proficient programmer. The stack offers fast, automatic memory management for small, short-lived data, while the heap provides flexibility for larger, dynamic allocations. By choosing the appropriate memory allocation strategy, you can write more efficient, robust code and avoid common pitfalls like stack overflows and memory leaks.
As you continue your coding journey and prepare for technical interviews, keep these concepts in mind. Practice working with both stack and heap memory in your projects, and don’t shy away from diving into the specifics of memory management in your chosen programming languages. This knowledge will not only make you a better programmer but also give you a significant advantage in technical interviews at top tech companies.
Remember, mastering memory management is an ongoing process. As you encounter new programming challenges and languages, you’ll continue to refine your understanding and skills. Keep coding, keep learning, and don’t hesitate to dive deep into the inner workings of the programs you write. Happy coding!