Square (Block) Technical Interview Prep: A Comprehensive Guide
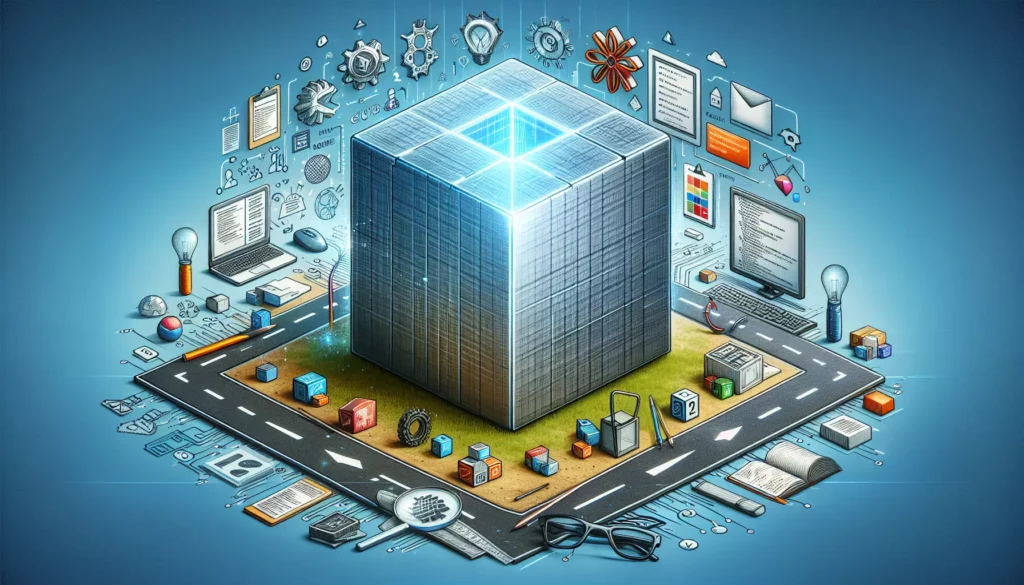
Are you gearing up for a technical interview at Square (now known as Block)? You’re in the right place! This comprehensive guide will walk you through everything you need to know to ace your interview and land that dream job at one of the most innovative fintech companies in the world.
Table of Contents
- Understanding Square (Block)
- The Interview Process at Square
- Technical Skills to Master
- Common Coding Challenges
- System Design Questions
- Behavioral Questions
- Tips and Tricks for Success
- Additional Resources
- Conclusion
1. Understanding Square (Block)
Before diving into the technical aspects of your interview prep, it’s crucial to understand the company you’re applying to. Square, now operating under the name Block, is a financial services and digital payments company founded by Jack Dorsey and Jim McKelvey in 2009.
Key points to know about Square (Block):
- Primary focus on financial technology and digital payments
- Offers a range of products including point-of-sale systems, payment hardware, and the Cash App
- Strong emphasis on blockchain technology and cryptocurrencies
- Culture of innovation, inclusivity, and economic empowerment
Understanding the company’s mission, values, and products will not only help you during the interview process but also allow you to gauge if the company aligns with your career goals and personal values.
2. The Interview Process at Square
The interview process at Square typically consists of several stages:
- Initial Phone Screen: A brief call with a recruiter to discuss your background and the role.
- Technical Phone Interview: A 45-60 minute interview focusing on coding and problem-solving skills.
- On-site Interviews: A series of interviews (usually 4-5) that cover various aspects:
- Coding interviews
- System design discussion
- Behavioral questions
- Team fit assessment
- Final Decision: The hiring team meets to discuss your performance and make a decision.
Each stage is designed to assess different aspects of your skills, knowledge, and cultural fit within the company.
3. Technical Skills to Master
To excel in your Square technical interview, you should be proficient in the following areas:
Programming Languages
While Square uses various languages, having a strong command of at least one of these is crucial:
- Java
- Ruby
- Python
- JavaScript
- Go
Data Structures
Ensure you have a solid understanding of fundamental data structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
Algorithms
Be prepared to implement and discuss various algorithms:
- Sorting (Quick Sort, Merge Sort, etc.)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Graph Algorithms (Dijkstra’s, Bellman-Ford, etc.)
Web Technologies
Given Square’s focus on fintech and digital payments, knowledge of web technologies is valuable:
- RESTful APIs
- HTTP/HTTPS protocols
- Web security concepts (CSRF, XSS, etc.)
- Frontend frameworks (React, Angular, Vue.js)
Database Systems
Understanding both relational and non-relational databases is important:
- SQL (MySQL, PostgreSQL)
- NoSQL (MongoDB, Cassandra)
- Database design and normalization
4. Common Coding Challenges
While the specific questions may vary, here are some types of coding challenges you might encounter during your Square interview:
String Manipulation
Example: Implement a function to reverse words in a string while preserving whitespace and initial word order.
def reverse_words(s):
words = s.split()
reversed_words = [word[::-1] for word in words]
return ' '.join(reversed_words)
# Test the function
input_string = "Hello World from Square"
print(reverse_words(input_string)) # Output: "olleH dlroW morf erauqS"
Array Problems
Example: Find the maximum subarray sum in a given array of integers.
def max_subarray_sum(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Test the function
array = [-2, 1, -3, 4, -1, 2, 1, -5, 4]
print(max_subarray_sum(array)) # Output: 6
Tree Traversal
Example: Implement an in-order traversal of a binary tree without using recursion.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
result.append(current.val)
current = current.right
return result
# Test the function
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
print(inorder_traversal(root)) # Output: [4, 2, 5, 1, 3]
Graph Algorithms
Example: Implement a function to detect if a cycle exists in a directed graph.
from collections import defaultdict
class Graph:
def __init__(self, vertices):
self.graph = defaultdict(list)
self.V = vertices
def add_edge(self, u, v):
self.graph[u].append(v)
def is_cyclic_util(self, v, visited, rec_stack):
visited[v] = True
rec_stack[v] = True
for neighbor in self.graph[v]:
if visited[neighbor] == False:
if self.is_cyclic_util(neighbor, visited, rec_stack) == True:
return True
elif rec_stack[neighbor] == True:
return True
rec_stack[v] = False
return False
def is_cyclic(self):
visited = [False] * self.V
rec_stack = [False] * self.V
for node in range(self.V):
if visited[node] == False:
if self.is_cyclic_util(node, visited, rec_stack) == True:
return True
return False
# Test the function
g = Graph(4)
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
print("Graph contains cycle: " + str(g.is_cyclic())) # Output: Graph contains cycle: True
5. System Design Questions
System design questions are a crucial part of the Square interview process, especially for more senior positions. These questions assess your ability to design scalable, reliable, and efficient systems. Here are some topics you should be prepared to discuss:
Designing a Payment Processing System
Given Square’s core business, you might be asked to design a simplified version of a payment processing system. Key considerations include:
- Transaction flow (from point of sale to settlement)
- Data storage and retrieval
- Security measures (encryption, fraud detection)
- Scalability and performance optimization
- Handling failures and ensuring data consistency
Designing a Distributed Cache
Understanding caching mechanisms is crucial for high-performance systems. Be prepared to discuss:
- Cache eviction policies (LRU, LFU, etc.)
- Consistency models (eventual consistency vs. strong consistency)
- Partitioning and replication strategies
- Handling cache misses and updates
Designing a Real-time Analytics Dashboard
Square provides various analytics tools to its merchants. You might be asked to design a system that can:
- Ingest and process large volumes of data in real-time
- Aggregate data for different time windows (hourly, daily, monthly)
- Provide low-latency queries for dashboard visualizations
- Scale to handle increasing data volumes and user load
Key System Design Principles
Regardless of the specific question, keep these principles in mind:
- Scalability: How does your system handle increasing load?
- Reliability: How do you ensure the system is fault-tolerant?
- Availability: How do you minimize downtime?
- Performance: How do you optimize for speed and efficiency?
- Security: How do you protect sensitive data and prevent unauthorized access?
6. Behavioral Questions
While technical skills are crucial, Square also values cultural fit and soft skills. Be prepared to answer behavioral questions that assess your problem-solving abilities, teamwork, and alignment with Square’s values. Here are some examples:
- Describe a time when you had to work on a challenging project. How did you approach it?
- Tell me about a situation where you had a conflict with a team member. How did you resolve it?
- How do you stay updated with the latest technologies and industry trends?
- Describe a time when you had to make a difficult decision. What was your thought process?
- How do you handle feedback, both positive and constructive?
- Tell me about a time when you failed. What did you learn from the experience?
When answering these questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
7. Tips and Tricks for Success
To maximize your chances of success in your Square technical interview, consider the following tips:
Before the Interview
- Review Square’s products and recent news to show your interest in the company.
- Practice coding problems on platforms like LeetCode, HackerRank, or AlgoCademy.
- Brush up on system design concepts and practice explaining complex systems.
- Prepare questions to ask your interviewers about the role and the company.
During the Interview
- Communicate clearly and think out loud while solving problems.
- Ask clarifying questions before jumping into solutions.
- Consider edge cases and discuss potential optimizations.
- If you’re stuck, don’t be afraid to ask for hints.
- Show enthusiasm for the role and the company.
After the Interview
- Send a thank-you email to your interviewers.
- Reflect on the experience and note areas for improvement.
- Be patient while waiting for feedback, but don’t hesitate to follow up if you haven’t heard back after a week.
8. Additional Resources
To further prepare for your Square technical interview, consider exploring these resources:
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- “Clean Code” by Robert C. Martin
Online Platforms
- LeetCode: For practicing coding problems
- HackerRank: For coding challenges and practice interviews
- AlgoCademy: For interactive coding tutorials and interview prep
- Pramp: For mock technical interviews with peers
Blogs and Websites
- Square Engineering Blog: To understand the company’s technical challenges and solutions
- High Scalability: For system design case studies
- GeeksforGeeks: For algorithm explanations and coding problems
9. Conclusion
Preparing for a technical interview at Square (Block) requires a combination of strong coding skills, system design knowledge, and cultural fit. By focusing on these areas and following the tips provided in this guide, you’ll be well-equipped to showcase your abilities and land that dream job at Square.
Remember, the key to success is consistent practice and a positive attitude. Even if you don’t get every question right, demonstrating your problem-solving approach, communication skills, and enthusiasm for the role can make a lasting impression on your interviewers.
Good luck with your Square technical interview! With thorough preparation and the right mindset, you’re already on your way to success. Keep coding, keep learning, and don’t forget to enjoy the process of growth and development that comes with interview preparation.