SQL Indexes: Boosting Database Performance for Efficient Queries
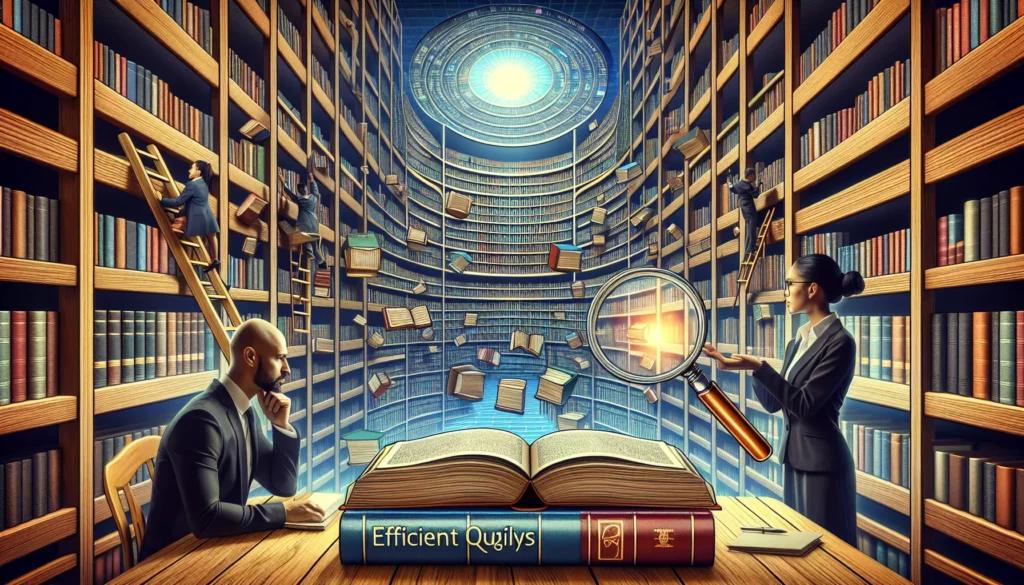
In the world of database management and optimization, SQL indexes play a crucial role in enhancing query performance and overall system efficiency. As data volumes continue to grow exponentially, understanding and effectively implementing indexes becomes increasingly important for developers and database administrators alike. This comprehensive guide will delve into the intricacies of SQL indexes, exploring their types, benefits, and best practices for implementation.
What are SQL Indexes?
SQL indexes are database objects that provide a quick lookup mechanism for locating data in tables. They function similarly to the index of a book, allowing the database engine to find specific rows quickly without scanning the entire table. By creating an index on one or more columns, you can significantly improve the speed of data retrieval operations, especially for large tables.
How SQL Indexes Work
When an index is created, the database engine generates a separate data structure that stores a sorted version of the indexed columns along with pointers to the corresponding table rows. This structure enables the database to perform binary searches, which are much faster than sequential scans of the entire table.
For example, consider a table with millions of records. Without an index, finding a specific record would require scanning each row sequentially until a match is found. With an index, the database can quickly narrow down the search to a small subset of rows, dramatically reducing the time required to locate the desired data.
Types of SQL Indexes
There are several types of indexes available in SQL databases, each suited for different scenarios and data types. Let’s explore the most common types:
1. Clustered Indexes
A clustered index determines the physical order of data in a table. Each table can have only one clustered index because the data can be sorted in only one order. In most database management systems, the primary key of a table automatically becomes the clustered index.
Key characteristics of clustered indexes:
- They store the actual data rows at the leaf level of the index.
- They provide faster data access for queries that return a range of values.
- They are particularly useful for columns frequently used in sorting operations.
2. Non-Clustered Indexes
Non-clustered indexes are separate from the actual data rows. They contain a copy of the indexed columns and a pointer to the corresponding data row. Unlike clustered indexes, a table can have multiple non-clustered indexes.
Key characteristics of non-clustered indexes:
- They are useful for columns frequently used in search conditions (WHERE clauses).
- They can improve the performance of queries that return a small subset of rows.
- They require additional storage space and maintenance overhead.
3. Unique Indexes
Unique indexes ensure that no duplicate values are entered in specific columns. They can be applied to both clustered and non-clustered indexes and are commonly used to enforce data integrity constraints.
4. Composite Indexes
Composite indexes are created on multiple columns of a table. They are useful when queries frequently filter or sort on a combination of columns.
5. Covering Indexes
A covering index includes all the columns required to execute a query. This type of index can significantly improve query performance by eliminating the need to access the actual table data.
Benefits of SQL Indexes
Implementing SQL indexes offers several advantages:
- Improved Query Performance: Indexes can dramatically reduce the time required to retrieve data, especially for large tables.
- Efficient Sorting and Grouping: Indexes can speed up ORDER BY and GROUP BY operations.
- Unique Constraints: Unique indexes help maintain data integrity by preventing duplicate entries.
- Foreign Key Optimization: Indexes on foreign key columns can improve the performance of join operations.
- Full-Text Search: Specialized full-text indexes enable efficient searching of text data.
When to Use SQL Indexes
While indexes can significantly improve query performance, they are not always necessary or beneficial. Consider creating indexes in the following scenarios:
- Columns frequently used in WHERE clauses
- Columns used in JOIN conditions
- Columns used in ORDER BY or GROUP BY clauses
- Tables with a large number of rows
- Columns with high cardinality (many unique values)
When to Avoid SQL Indexes
In some cases, creating indexes may not be beneficial or could even negatively impact performance:
- Small tables with few rows
- Columns with low cardinality (few unique values)
- Tables that undergo frequent large-scale INSERT, UPDATE, or DELETE operations
- Columns rarely used in queries
Best Practices for SQL Indexing
To maximize the benefits of SQL indexes while minimizing potential drawbacks, consider the following best practices:
1. Analyze Query Patterns
Before creating indexes, analyze the most common and resource-intensive queries in your application. This will help you identify which columns are most frequently used in WHERE clauses, JOIN conditions, and sorting operations.
2. Use the Appropriate Index Type
Choose the right type of index based on your specific requirements. For example, use clustered indexes for columns frequently used in range queries, and non-clustered indexes for columns often used in equality comparisons.
3. Consider Composite Indexes
If your queries frequently filter on multiple columns, consider creating a composite index. The order of columns in a composite index is crucial for optimal performance.
4. Monitor and Maintain Indexes
Regularly monitor index usage and performance. Remove or modify indexes that are rarely used or no longer beneficial. Rebuild or reorganize indexes periodically to maintain their efficiency.
5. Be Mindful of Index Overhead
Remember that indexes consume additional storage space and require maintenance during data modifications. Balance the performance gains against the increased storage and maintenance costs.
6. Use Covering Indexes for Frequently Executed Queries
For queries that are executed frequently and return a small subset of columns, consider creating covering indexes to improve performance.
7. Avoid Over-Indexing
Creating too many indexes can lead to decreased performance during data modifications and increased storage requirements. Strive for a balance between query performance and maintenance overhead.
Implementing SQL Indexes
The syntax for creating indexes may vary slightly depending on the specific database management system you’re using. Here are some general examples of how to create indexes in SQL:
Creating a Simple Index
CREATE INDEX idx_lastname
ON employees (last_name);
This creates a non-clustered index on the last_name column of the employees table.
Creating a Unique Index
CREATE UNIQUE INDEX idx_email
ON users (email);
This creates a unique index on the email column of the users table, ensuring that no duplicate email addresses are allowed.
Creating a Composite Index
CREATE INDEX idx_name_email
ON customers (last_name, first_name, email);
This creates a composite index on the last_name, first_name, and email columns of the customers table.
Creating a Clustered Index
CREATE CLUSTERED INDEX idx_order_date
ON orders (order_date);
This creates a clustered index on the order_date column of the orders table. Remember that a table can have only one clustered index.
Monitoring and Optimizing SQL Indexes
To ensure that your indexes continue to provide optimal performance, it’s essential to monitor their usage and effectiveness regularly. Most database management systems provide tools and techniques for index analysis and optimization:
1. Execution Plan Analysis
Examine the execution plans of your most critical queries to see how indexes are being used. Look for table scans or index scans that could be optimized with better indexing strategies.
2. Index Usage Statistics
Many database systems provide built-in views or functions to show index usage statistics. For example, in SQL Server, you can use the sys.dm_db_index_usage_stats dynamic management view to see how often each index is used and whether it’s used for seeks, scans, or lookups.
3. Index Fragmentation
Over time, indexes can become fragmented, which can negatively impact their performance. Regularly check for index fragmentation and rebuild or reorganize indexes as needed.
4. Missing Index Recommendations
Some database systems can suggest missing indexes based on query patterns. While these recommendations can be helpful, always evaluate them in the context of your specific application needs and overall indexing strategy.
5. Query Store
In SQL Server, the Query Store feature can help you identify queries that have degraded in performance over time, potentially due to changes in data distribution or missing indexes.
Advanced Indexing Techniques
As you become more proficient with SQL indexing, consider exploring these advanced techniques to further optimize your database performance:
1. Filtered Indexes
Filtered indexes are partial indexes that include only a subset of rows in a table. They can be useful for improving query performance on specific subsets of data while reducing the overall index size and maintenance overhead.
CREATE INDEX idx_active_users
ON users (username)
WHERE is_active = 1;
2. Columnstore Indexes
Columnstore indexes are designed for analytical queries and data warehousing scenarios. They store and process data in a column-oriented format, which can significantly improve the performance of queries that aggregate large amounts of data.
3. Spatial Indexes
For databases that work with geographic or geometric data, spatial indexes can dramatically improve the performance of queries involving spatial operations.
4. Full-Text Indexes
Full-text indexes enable efficient searching and ranking of text data, making them ideal for applications that require complex text-based queries.
Common Pitfalls in SQL Indexing
While implementing SQL indexes, be aware of these common pitfalls:
1. Over-Indexing
Creating too many indexes can lead to increased storage requirements and slower data modification operations. Strive for a balance between query performance and maintenance overhead.
2. Ignoring Data Distribution
The effectiveness of an index can vary depending on the distribution of data in the indexed columns. Regularly analyze data distribution and adjust your indexing strategy accordingly.
3. Neglecting Index Maintenance
Failing to maintain indexes can lead to fragmentation and decreased performance over time. Implement a regular maintenance schedule to keep your indexes optimized.
4. Incorrect Index Column Order
In composite indexes, the order of columns can significantly impact performance. Place the most selective columns first and align the index column order with your query patterns.
5. Indexing Computed Columns Incorrectly
When indexing computed columns, ensure that the expressions are deterministic and precise to avoid unexpected behavior.
Conclusion
SQL indexes are powerful tools for optimizing database performance and enhancing query efficiency. By understanding the various types of indexes, their benefits, and best practices for implementation, you can significantly improve the performance of your database-driven applications.
Remember that effective indexing is an ongoing process that requires regular monitoring, analysis, and refinement. As your data and query patterns evolve, so should your indexing strategy. By staying informed about advanced indexing techniques and being mindful of common pitfalls, you can ensure that your databases continue to perform optimally, even as they grow and change over time.
Mastering SQL indexes is a valuable skill for any developer or database administrator. It not only enhances the performance of your current projects but also provides a solid foundation for tackling more complex database optimization challenges in the future. As you continue to work with databases, keep experimenting with different indexing strategies and stay updated on the latest features and best practices in your chosen database management system.