Spotify Technical Interview Prep: A Comprehensive Guide
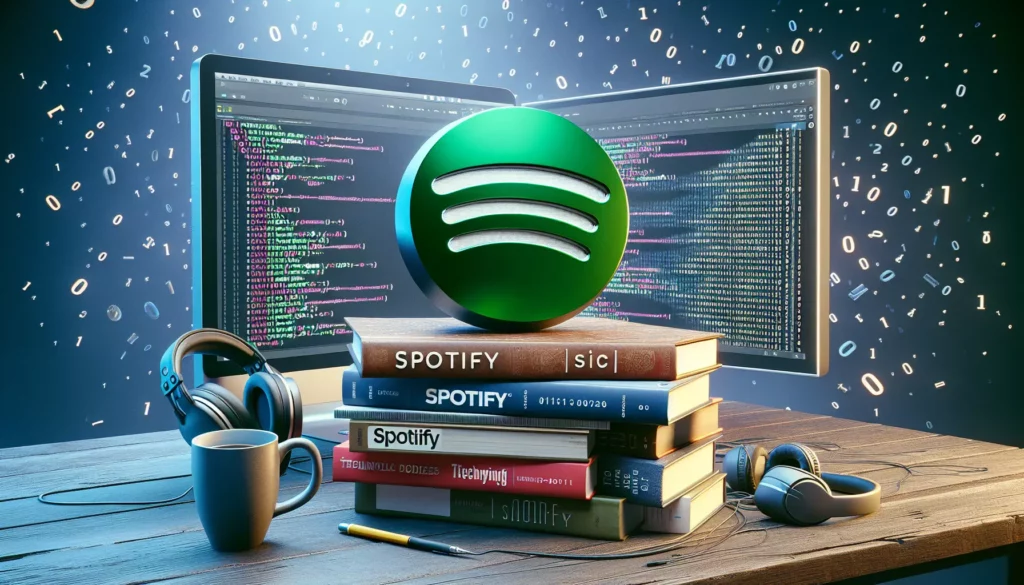
Are you gearing up for a technical interview at Spotify? You’ve come to the right place! In this comprehensive guide, we’ll walk you through everything you need to know to ace your Spotify technical interview. From understanding the interview process to mastering key coding concepts and practicing with sample questions, we’ve got you covered. Let’s dive in and prepare you for success in your Spotify technical interview!
Table of Contents
- Understanding the Spotify Interview Process
- Key Skills and Knowledge Areas
- Coding Languages to Focus On
- Essential Data Structures
- Must-Know Algorithms
- System Design Concepts
- Sample Interview Questions and Solutions
- Tips and Tricks for Interview Success
- Additional Resources for Preparation
- Conclusion
1. Understanding the Spotify Interview Process
Before diving into the technical aspects, it’s crucial to understand what to expect during the Spotify interview process. Typically, the process consists of several stages:
- Initial Screening: This usually involves a phone call or online assessment to gauge your basic qualifications and interest in the role.
- Technical Phone Interview: You’ll likely have one or two technical phone interviews where you’ll be asked to solve coding problems in real-time, often using a shared coding environment.
- On-site Interviews: If you pass the initial stages, you’ll be invited for on-site interviews. These typically include multiple rounds of technical interviews, a system design interview, and behavioral interviews.
- Final Decision: After the on-site interviews, the hiring team will make a decision based on your performance across all stages.
Throughout this process, Spotify evaluates not only your technical skills but also your problem-solving approach, communication abilities, and cultural fit within the company.
2. Key Skills and Knowledge Areas
To excel in your Spotify technical interview, you should be well-versed in the following areas:
- Strong proficiency in at least one programming language (preferably Java, Python, or JavaScript)
- Solid understanding of data structures and algorithms
- Knowledge of system design principles
- Familiarity with distributed systems and microservices architecture
- Understanding of database concepts (both SQL and NoSQL)
- Experience with cloud platforms (e.g., AWS, Google Cloud, or Azure)
- Knowledge of version control systems (e.g., Git)
- Familiarity with agile development methodologies
- Understanding of testing and debugging practices
- Knowledge of API design and RESTful services
3. Coding Languages to Focus On
While Spotify uses various programming languages, it’s essential to be proficient in at least one of the following:
Java
Java is widely used at Spotify, especially for backend services. Make sure you’re comfortable with:
- Object-oriented programming concepts
- Java Collections Framework
- Concurrency and multithreading
- Java 8+ features (lambdas, streams, optional)
Python
Python is popular for data analysis and machine learning tasks at Spotify. Focus on:
- Python data structures and their time complexities
- List comprehensions and generator expressions
- Python’s standard libraries (e.g., collections, itertools)
- Basic understanding of libraries like NumPy and Pandas
JavaScript
For frontend roles, JavaScript proficiency is crucial. Key areas include:
- ES6+ features
- Asynchronous programming (Promises, async/await)
- Closures and scope
- Familiarity with popular frameworks (e.g., React, Angular, or Vue.js)
4. Essential Data Structures
A solid understanding of data structures is crucial for acing your Spotify technical interview. Be prepared to implement and discuss the following data structures:
Arrays and Strings
Arrays and strings are fundamental data structures used in many algorithms. Make sure you’re comfortable with:
- Array manipulation techniques
- String parsing and manipulation
- Time and space complexities of common operations
Linked Lists
Understand the implementation and operations of both singly and doubly linked lists. Key points include:
- Insertion and deletion operations
- Reversing a linked list
- Detecting cycles in a linked list
Stacks and Queues
These data structures are often used in various algorithms and system designs. Focus on:
- Implementation using arrays and linked lists
- Applications of stacks (e.g., balancing parentheses, implementing undo functionality)
- Applications of queues (e.g., breadth-first search, task scheduling)
Trees and Graphs
Trees and graphs are essential for many complex problems. Be familiar with:
- Binary trees and binary search trees
- Tree traversal algorithms (in-order, pre-order, post-order)
- Graph representations (adjacency list, adjacency matrix)
- Basic graph algorithms (BFS, DFS)
Hash Tables
Hash tables are crucial for efficient lookups and are often used in system design. Understand:
- Hash function design
- Collision resolution techniques
- Time and space complexities of hash table operations
Heaps
Heaps are useful for priority queue implementations and certain sorting algorithms. Know:
- Min-heap and max-heap properties
- Heap operations (insertion, deletion, heapify)
- Applications of heaps (e.g., finding the k-th largest element)
5. Must-Know Algorithms
Spotify’s technical interviews often include algorithm-based questions. Make sure you’re well-versed in the following algorithms and techniques:
Sorting Algorithms
Understand the implementation, time complexity, and use cases of various sorting algorithms:
- Quick Sort
- Merge Sort
- Heap Sort
- Counting Sort
- Radix Sort
Searching Algorithms
Be familiar with different searching techniques and their applications:
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
Dynamic Programming
Dynamic programming is a powerful technique for solving optimization problems. Focus on:
- Identifying DP problems
- Memoization and tabulation approaches
- Common DP problems (e.g., Fibonacci sequence, longest common subsequence)
Greedy Algorithms
Understand when and how to apply greedy algorithms to solve problems efficiently:
- Activity selection problem
- Huffman coding
- Dijkstra’s algorithm for shortest paths
Graph Algorithms
Given Spotify’s focus on recommendation systems and network analysis, graph algorithms are crucial:
- Shortest path algorithms (Dijkstra’s, Bellman-Ford)
- Minimum spanning tree algorithms (Kruskal’s, Prim’s)
- Topological sorting
- Strongly connected components
String Manipulation Algorithms
String processing is often relevant in music-related applications:
- String matching algorithms (e.g., KMP, Rabin-Karp)
- Regular expression matching
- Edit distance and string similarity measures
6. System Design Concepts
System design is a crucial aspect of Spotify’s technical interviews, especially for more senior positions. Be prepared to discuss and design large-scale systems. Key areas to focus on include:
Scalability
Understand how to design systems that can handle millions of users:
- Horizontal vs. vertical scaling
- Load balancing techniques
- Caching strategies (e.g., CDN, Redis)
- Database sharding and partitioning
Microservices Architecture
Spotify heavily relies on microservices. Be familiar with:
- Advantages and challenges of microservices
- Service discovery and registration
- Inter-service communication (e.g., REST, gRPC)
- API gateway pattern
Distributed Systems
Understanding distributed systems is crucial for designing robust, scalable applications:
- CAP theorem
- Consistency models
- Distributed caching
- Message queues and event-driven architectures
Database Design
Know when to use different types of databases and how to design them efficiently:
- Relational vs. NoSQL databases
- Database normalization and denormalization
- Indexing strategies
- ACID properties
Real-time Processing
Given Spotify’s focus on real-time recommendations and analytics:
- Stream processing concepts
- Real-time analytics architectures
- Familiarity with technologies like Apache Kafka or Apache Flink
Security and Authentication
Understand basic security concepts and authentication mechanisms:
- OAuth 2.0 and OpenID Connect
- SSL/TLS
- API security best practices
7. Sample Interview Questions and Solutions
To help you prepare, here are some sample questions you might encounter in a Spotify technical interview, along with brief solution outlines:
Question 1: Implement a playlist shuffle algorithm
Design an algorithm to shuffle a playlist of songs, ensuring that each song has an equal probability of being played and no two consecutive plays of the playlist will have the same song order.
Solution outline:
- Use the Fisher-Yates shuffle algorithm to randomly permute the playlist.
- Keep track of the previous shuffle.
- If the new shuffle results in any song being in the same position as the previous shuffle, re-shuffle until this condition is met.
def shuffle_playlist(playlist):
n = len(playlist)
for i in range(n - 1, 0, -1):
j = random.randint(0, i)
playlist[i], playlist[j] = playlist[j], playlist[i]
return playlist
def unique_shuffle(playlist, previous_shuffle):
while True:
new_shuffle = shuffle_playlist(playlist.copy())
if all(new_shuffle[i] != previous_shuffle[i] for i in range(len(playlist))):
return new_shuffle
Question 2: Design a system for Spotify’s “Discover Weekly” feature
Outline the high-level architecture for Spotify’s “Discover Weekly” feature, which generates a personalized playlist for users based on their listening history and preferences.
Solution outline:
- Data Collection: Track user listening history, liked songs, and playlists.
- Feature Extraction: Extract relevant features from songs (genre, tempo, mood, etc.).
- User Profiling: Create user profiles based on listening habits and preferences.
- Recommendation Engine: Use collaborative filtering and content-based filtering to generate recommendations.
- Playlist Generation: Create a diverse playlist from the recommendations, ensuring a good mix of familiar and new content.
- Delivery System: Schedule and deliver the playlist to users on a weekly basis.
Question 3: Implement a function to find the top K most frequent words in a large text file
Design and implement an efficient algorithm to find the K most frequently occurring words in a large text file, where K is a parameter passed to the function.
Solution outline:
- Use a hash map to count the frequency of each word.
- Use a min-heap of size K to keep track of the top K frequent words.
- Iterate through the hash map, updating the min-heap as necessary.
import heapq
from collections import Counter
def top_k_frequent_words(file_path, k):
word_counts = Counter()
with open(file_path, 'r') as file:
for line in file:
words = line.strip().lower().split()
word_counts.update(words)
return heapq.nlargest(k, word_counts.keys(), key=word_counts.get)
8. Tips and Tricks for Interview Success
To increase your chances of success in your Spotify technical interview, keep these tips in mind:
- Practice, practice, practice: Regularly solve coding problems on platforms like LeetCode, HackerRank, or AlgoCademy.
- Think out loud: Communicate your thought process clearly as you work through problems.
- Ask clarifying questions: Ensure you fully understand the problem before diving into a solution.
- Consider edge cases: Always think about and address potential edge cases in your solutions.
- Optimize your solutions: After solving a problem, consider ways to improve its time and space complexity.
- Be familiar with Spotify’s products: Understanding Spotify’s features and challenges can help you provide more relevant answers.
- Review your past projects: Be prepared to discuss your previous work in detail, including challenges faced and solutions implemented.
- Stay calm under pressure: Remember that interviewers are interested in your problem-solving approach, not just the final answer.
- Practice mock interviews: Conduct mock interviews with friends or use online platforms to simulate the interview experience.
- Be honest about what you don’t know: If you’re unsure about something, it’s better to admit it and discuss how you’d go about finding the answer.
9. Additional Resources for Preparation
To further enhance your preparation for the Spotify technical interview, consider exploring these resources:
- Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- “Designing Data-Intensive Applications” by Martin Kleppmann
- Online Courses:
- AlgoCademy’s comprehensive coding interview preparation courses
- Coursera’s “Algorithms Specialization” by Stanford University
- Udacity’s “Data Structures and Algorithms Nanodegree”
- Coding Platforms:
- LeetCode
- HackerRank
- CodeSignal
- System Design Resources:
- Grokking the System Design Interview
- System Design Primer (GitHub repository)
- Spotify Engineering Blog: Read about Spotify’s technical challenges and solutions directly from their engineering team.
- GitHub: Explore open-source projects related to music streaming and recommendation systems.
10. Conclusion
Preparing for a Spotify technical interview requires dedication, practice, and a broad understanding of computer science fundamentals, algorithms, and system design. By focusing on the key areas outlined in this guide and consistently practicing your problem-solving skills, you’ll be well-equipped to tackle the challenges presented in your interview.
Remember that the interview process is not just about finding the correct answer, but also about demonstrating your approach to problem-solving, your ability to communicate technical concepts clearly, and your passion for technology and music.
As you prepare, leverage the resources provided, including AlgoCademy’s comprehensive coding interview preparation courses, to gain hands-on experience and confidence. With thorough preparation and the right mindset, you’ll be well on your way to impressing your interviewers and landing that dream job at Spotify.
Good luck with your preparation, and may your Spotify technical interview be as smooth and harmonious as your favorite playlist!