Sorting Algorithms: The Foundation of Efficient Programming
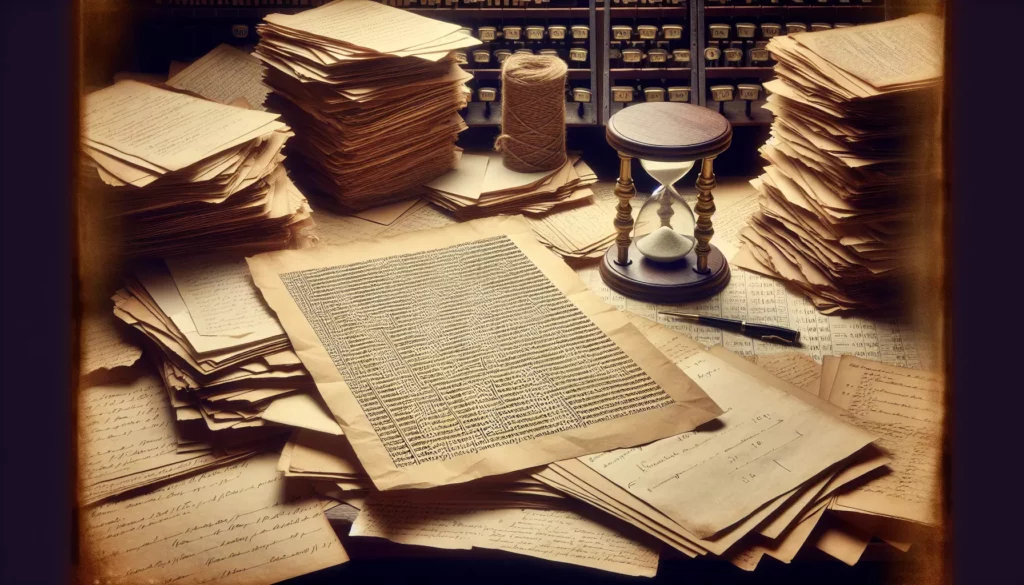
In the world of computer science and programming, sorting algorithms play a crucial role in organizing and managing data efficiently. These algorithms are fundamental building blocks that every programmer should understand and master. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, a solid grasp of sorting algorithms is essential.
In this comprehensive guide, we’ll explore various sorting algorithms, their implementations, time and space complexities, and real-world applications. We’ll also discuss how understanding these algorithms can help you become a more efficient programmer and excel in technical interviews.
1. Introduction to Sorting Algorithms
Sorting algorithms are methods used to arrange elements in a specific order, typically ascending or descending. These algorithms are fundamental to computer science and are used in countless applications, from organizing data in databases to optimizing search operations.
The importance of sorting algorithms extends beyond just arranging data. They serve as excellent examples for understanding algorithm design, time complexity analysis, and space efficiency. Many sorting algorithms also introduce key programming concepts such as recursion, divide-and-conquer strategies, and in-place operations.
2. Common Sorting Algorithms
Let’s dive into some of the most common sorting algorithms you’re likely to encounter in your programming journey and during technical interviews.
2.1 Bubble Sort
Bubble Sort is one of the simplest sorting algorithms. It repeatedly steps through the list, compares adjacent elements, and swaps them if they’re in the wrong order. While it’s not efficient for large datasets, it’s easy to understand and implement.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
arr = [64, 34, 25, 12, 22, 11, 90]
sorted_arr = bubble_sort(arr)
print(sorted_arr) # Output: [11, 12, 22, 25, 34, 64, 90]
Time Complexity: O(n^2) in the worst and average cases, where n is the number of elements.
Space Complexity: O(1) as it sorts in-place.
2.2 Selection Sort
Selection Sort divides the input list into two parts: a sorted portion at the left end and an unsorted portion at the right end. It repeatedly selects the smallest (or largest) element from the unsorted portion and moves it to the sorted portion.
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_idx = i
for j in range(i + 1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
# Example usage
arr = [64, 25, 12, 22, 11]
sorted_arr = selection_sort(arr)
print(sorted_arr) # Output: [11, 12, 22, 25, 64]
Time Complexity: O(n^2) in all cases.
Space Complexity: O(1) as it sorts in-place.
2.3 Insertion Sort
Insertion Sort builds the final sorted array one item at a time. It’s much less efficient on large lists than more advanced algorithms such as QuickSort, HeapSort, or MergeSort. However, it performs well for small datasets and is often used as part of more sophisticated algorithms.
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
# Example usage
arr = [12, 11, 13, 5, 6]
sorted_arr = insertion_sort(arr)
print(sorted_arr) # Output: [5, 6, 11, 12, 13]
Time Complexity: O(n^2) in the worst and average cases, but O(n) in the best case when the array is already sorted.
Space Complexity: O(1) as it sorts in-place.
2.4 Merge Sort
Merge Sort is an efficient, stable, divide-and-conquer algorithm. It divides the unsorted list into n sublists, each containing one element, then repeatedly merges sublists to produce new sorted sublists until there is only one sublist remaining.
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
L = arr[:mid]
R = arr[mid:]
merge_sort(L)
merge_sort(R)
i = j = k = 0
while i < len(L) and j < len(R):
if L[i] < R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
return arr
# Example usage
arr = [38, 27, 43, 3, 9, 82, 10]
sorted_arr = merge_sort(arr)
print(sorted_arr) # Output: [3, 9, 10, 27, 38, 43, 82]
Time Complexity: O(n log n) in all cases.
Space Complexity: O(n) due to the temporary arrays created during the merge process.
2.5 QuickSort
QuickSort is a highly efficient sorting algorithm and is based on partitioning of array of data into smaller arrays. It picks an element as pivot and partitions the given array around the picked pivot.
def quicksort(arr):
if len(arr) <= 1:
return arr
else:
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
# Example usage
arr = [10, 7, 8, 9, 1, 5]
sorted_arr = quicksort(arr)
print(sorted_arr) # Output: [1, 5, 7, 8, 9, 10]
Time Complexity: O(n log n) on average, O(n^2) in the worst case.
Space Complexity: O(log n) due to the recursive calls.
3. Advanced Sorting Algorithms
While the algorithms mentioned above are fundamental and commonly used, there are several advanced sorting algorithms that offer improved performance in specific scenarios.
3.1 HeapSort
HeapSort is a comparison-based sorting algorithm that uses a binary heap data structure. It’s similar to Selection Sort where we first find the minimum element and place it at the beginning. We repeat this process for the remaining elements.
def heapify(arr, n, i):
largest = i
l = 2 * i + 1
r = 2 * i + 2
if l < n and arr[i] < arr[l]:
largest = l
if r < n and arr[largest] < arr[r]:
largest = r
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
heapify(arr, n, largest)
def heap_sort(arr):
n = len(arr)
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
for i in range(n - 1, 0, -1):
arr[i], arr[0] = arr[0], arr[i]
heapify(arr, i, 0)
return arr
# Example usage
arr = [12, 11, 13, 5, 6, 7]
sorted_arr = heap_sort(arr)
print(sorted_arr) # Output: [5, 6, 7, 11, 12, 13]
Time Complexity: O(n log n) in all cases.
Space Complexity: O(1) as it sorts in-place.
3.2 Counting Sort
Counting Sort is an integer sorting algorithm that operates by counting the number of objects that possess distinct key values, and applying prefix sum on those counts to determine the positions of each key value in the output sequence.
def counting_sort(arr):
max_val = max(arr)
m = max_val + 1
count = [0] * m
for a in arr:
count[a] += 1
i = 0
for a in range(m):
for c in range(count[a]):
arr[i] = a
i += 1
return arr
# Example usage
arr = [4, 2, 2, 8, 3, 3, 1]
sorted_arr = counting_sort(arr)
print(sorted_arr) # Output: [1, 2, 2, 3, 3, 4, 8]
Time Complexity: O(n + k) where n is the number of elements and k is the range of input.
Space Complexity: O(k) for the counting array.
3.3 Radix Sort
Radix Sort is a non-comparative integer sorting algorithm that sorts data with integer keys by grouping keys by the individual digits which share the same significant position and value.
def counting_sort_for_radix(arr, exp):
n = len(arr)
output = [0] * n
count = [0] * 10
for i in range(n):
index = arr[i] // exp
count[index % 10] += 1
for i in range(1, 10):
count[i] += count[i - 1]
i = n - 1
while i >= 0:
index = arr[i] // exp
output[count[index % 10] - 1] = arr[i]
count[index % 10] -= 1
i -= 1
for i in range(n):
arr[i] = output[i]
def radix_sort(arr):
max_val = max(arr)
exp = 1
while max_val // exp > 0:
counting_sort_for_radix(arr, exp)
exp *= 10
return arr
# Example usage
arr = [170, 45, 75, 90, 802, 24, 2, 66]
sorted_arr = radix_sort(arr)
print(sorted_arr) # Output: [2, 24, 45, 66, 75, 90, 170, 802]
Time Complexity: O(d * (n + k)) where d is the number of digits in the largest number, n is the number of elements, and k is the range of input (usually 10 for decimal numbers).
Space Complexity: O(n + k)
4. Choosing the Right Sorting Algorithm
Selecting the appropriate sorting algorithm depends on various factors:
- Size of the dataset: For small datasets, simple algorithms like Insertion Sort might be faster due to less overhead. For larger datasets, more efficient algorithms like QuickSort or MergeSort are preferred.
- Nature of the data: If the data is already partially sorted, algorithms like Insertion Sort can be very efficient. For random data, QuickSort often performs well.
- Memory constraints: If memory is a concern, in-place sorting algorithms like HeapSort might be preferable.
- Stability requirement: If maintaining the relative order of equal elements is important, stable sorts like MergeSort should be used.
- Time complexity: For large datasets where performance is critical, algorithms with O(n log n) average-case time complexity like QuickSort, MergeSort, or HeapSort are often the best choice.
5. Sorting Algorithms in Real-World Applications
Understanding sorting algorithms is not just an academic exercise; these algorithms have numerous real-world applications:
- Database Systems: Sorting is crucial in database operations, especially for optimizing queries and indexing.
- File Systems: Operating systems use sorting algorithms to organize files and directories.
- Search Engines: Sorting is used to rank search results based on relevance.
- Data Analysis: Many data analysis tasks require sorted data for efficient processing and visualization.
- Compression Algorithms: Some compression techniques use sorting as a preprocessing step.
- Computer Graphics: Sorting is used in rendering pipelines to determine the order of drawing objects.
6. Optimizing Sorting Algorithms
While the basic implementations of sorting algorithms are important to understand, in practice, many optimizations can be applied:
6.1 Hybrid Algorithms
Many modern sorting implementations use hybrid approaches. For example, the standard library sort functions in languages like C++ and Java use a combination of QuickSort, HeapSort, and Insertion Sort. This approach takes advantage of the strengths of different algorithms based on the size and nature of the data.
6.2 Parallel Sorting
With the rise of multi-core processors, parallel sorting algorithms have become increasingly important. Algorithms like Parallel Merge Sort can significantly speed up sorting on large datasets by utilizing multiple CPU cores.
6.3 External Sorting
When dealing with datasets too large to fit in memory, external sorting algorithms are used. These algorithms efficiently sort data that resides in slower external memory like hard drives.
7. Sorting in Technical Interviews
Sorting algorithms are a favorite topic in technical interviews, especially at major tech companies. Here are some tips for tackling sorting-related interview questions:
- Understand the basics: Be able to implement and explain the common sorting algorithms like QuickSort, MergeSort, and HeapSort.
- Know the trade-offs: Understand the time and space complexity of different algorithms and when to use each.
- Practice implementation: Be comfortable coding sorting algorithms from scratch.
- Analyze edge cases: Consider how different algorithms perform with already sorted data, reverse sorted data, or data with many duplicates.
- Optimize for the problem: If given a specific problem, think about how you can tailor or optimize a sorting algorithm for that particular scenario.
8. Advanced Topics in Sorting
As you delve deeper into the world of sorting algorithms, you’ll encounter more advanced topics:
8.1 Sorting in Linear Time
While comparison-based sorting algorithms have a lower bound of O(n log n), non-comparison based sorts like Counting Sort, Radix Sort, and Bucket Sort can achieve O(n) time complexity under certain conditions.
8.2 Sorting Networks
Sorting networks are algorithms for sorting that are specially designed for efficient implementation in hardware. They consist of a fixed sequence of compare-and-swap operations that are performed regardless of the input data.
8.3 Quantum Sorting
With the advent of quantum computing, new sorting algorithms that leverage quantum mechanics are being developed. These algorithms promise to solve certain sorting problems faster than classical algorithms.
9. Conclusion
Sorting algorithms are a fundamental part of computer science and play a crucial role in efficient programming. From simple algorithms like Bubble Sort to more complex ones like QuickSort and advanced topics like parallel sorting, understanding these algorithms is essential for any programmer.
As you progress in your coding journey, whether you’re preparing for technical interviews or working on large-scale software projects, the knowledge of sorting algorithms will prove invaluable. They not only help in solving specific sorting problems but also introduce important programming concepts and algorithm design techniques.
Remember, the key to mastering sorting algorithms is practice. Implement these algorithms, analyze their performance with different datasets, and try to optimize them. As you gain more experience, you’ll develop an intuition for choosing the right algorithm for each situation, making you a more effective and efficient programmer.
Keep exploring, keep coding, and remember that every great programmer started with understanding these fundamental algorithms. Happy sorting!