Solving Linear Equations with Algorithms: A Comprehensive Guide
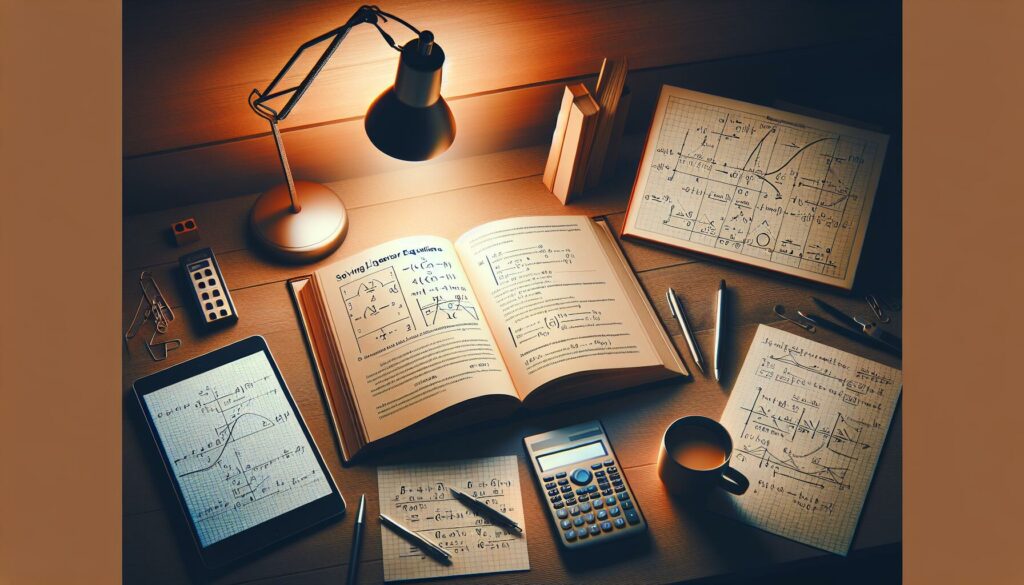
Linear equations are fundamental to many areas of mathematics and computer science. They form the backbone of numerous real-world applications, from physics and engineering to economics and data science. In this comprehensive guide, we’ll explore various algorithms for solving linear equations, their implementations, and their applications in the world of programming and beyond.
Table of Contents
- Introduction to Linear Equations
- Gaussian Elimination
- LU Decomposition
- Jacobi Method
- Gauss-Seidel Method
- Conjugate Gradient Method
- Applications of Linear Equation Solvers
- Implementing Linear Equation Solvers in Code
- Optimization Techniques for Linear Equation Solvers
- Conclusion
1. Introduction to Linear Equations
Linear equations are algebraic equations in which each term is either a constant or the product of a constant and a single variable. They can be represented in the form:
aâ‚xâ‚ + aâ‚‚xâ‚‚ + ... + aâ‚™xâ‚™ = b
Where aâ‚, aâ‚‚, …, aâ‚™ are constants (coefficients), xâ‚, xâ‚‚, …, xâ‚™ are variables, and b is the constant term.
A system of linear equations consists of multiple linear equations that need to be solved simultaneously. In matrix form, this can be represented as:
Ax = b
Where A is the coefficient matrix, x is the vector of variables, and b is the vector of constant terms.
Solving linear equations is crucial in various fields, including:
- Computer Graphics: Transformations and projections
- Machine Learning: Linear regression and optimization
- Physics: Modeling physical systems
- Economics: Supply and demand analysis
- Engineering: Structural analysis and circuit design
Now, let’s dive into the algorithms used to solve these equations efficiently.
2. Gaussian Elimination
Gaussian elimination is one of the most fundamental methods for solving systems of linear equations. It works by transforming the augmented matrix of the system into row echelon form through a series of elementary row operations.
Steps of Gaussian Elimination:
- Create the augmented matrix [A|b]
- Convert the matrix to row echelon form
- Back-substitute to find the solution
Here’s a simple implementation of Gaussian elimination in Python:
import numpy as np
def gaussian_elimination(A, b):
n = len(b)
# Create augmented matrix
Ab = np.column_stack((A, b))
for i in range(n):
# Find pivot
max_element = abs(Ab[i][i])
max_row = i
for k in range(i + 1, n):
if abs(Ab[k][i]) > max_element:
max_element = abs(Ab[k][i])
max_row = k
# Swap maximum row with current row
Ab[i], Ab[max_row] = Ab[max_row], Ab[i].copy()
# Make all rows below this one 0 in current column
for k in range(i + 1, n):
c = -Ab[k][i] / Ab[i][i]
for j in range(i, n + 1):
if i == j:
Ab[k][j] = 0
else:
Ab[k][j] += c * Ab[i][j]
# Solve equation Ax=b using back substitution
x = np.zeros(n)
for i in range(n - 1, -1, -1):
x[i] = Ab[i][n] / Ab[i][i]
for k in range(i - 1, -1, -1):
Ab[k][n] -= Ab[k][i] * x[i]
return x
# Example usage
A = np.array([[2, 1, -1],
[-3, -1, 2],
[-2, 1, 2]])
b = np.array([8, -11, -3])
solution = gaussian_elimination(A, b)
print("Solution:", solution)
Gaussian elimination has a time complexity of O(n³) for an n × n system, making it efficient for small to medium-sized systems but less practical for very large systems.
3. LU Decomposition
LU decomposition is another method for solving systems of linear equations. It decomposes the coefficient matrix A into the product of a lower triangular matrix L and an upper triangular matrix U.
The main advantage of LU decomposition is that once the decomposition is done, it can be used to solve multiple systems with the same coefficient matrix but different right-hand sides efficiently.
Steps of LU Decomposition:
- Decompose A into L and U matrices
- Solve Ly = b for y using forward substitution
- Solve Ux = y for x using back substitution
Here’s a Python implementation of LU decomposition:
import numpy as np
def lu_decomposition(A):
n = len(A)
L = np.zeros((n, n))
U = np.zeros((n, n))
for i in range(n):
L[i][i] = 1
for j in range(i, n):
sum1 = sum(L[i][k] * U[k][j] for k in range(i))
U[i][j] = A[i][j] - sum1
for j in range(i + 1, n):
sum2 = sum(L[j][k] * U[k][i] for k in range(i))
L[j][i] = (A[j][i] - sum2) / U[i][i]
return L, U
def forward_substitution(L, b):
n = len(b)
y = np.zeros(n)
for i in range(n):
y[i] = b[i] - sum(L[i][j] * y[j] for j in range(i))
return y
def back_substitution(U, y):
n = len(y)
x = np.zeros(n)
for i in range(n - 1, -1, -1):
x[i] = (y[i] - sum(U[i][j] * x[j] for j in range(i + 1, n))) / U[i][i]
return x
def solve_lu(A, b):
L, U = lu_decomposition(A)
y = forward_substitution(L, b)
x = back_substitution(U, y)
return x
# Example usage
A = np.array([[2, 1, -1],
[-3, -1, 2],
[-2, 1, 2]])
b = np.array([8, -11, -3])
solution = solve_lu(A, b)
print("Solution:", solution)
LU decomposition also has a time complexity of O(n³) for the decomposition, but subsequent solves with different right-hand sides can be done in O(n²) time.
4. Jacobi Method
The Jacobi method is an iterative algorithm for solving systems of linear equations. It’s particularly useful for large, sparse systems where direct methods like Gaussian elimination might be impractical.
Steps of the Jacobi Method:
- Start with an initial guess for the solution
- For each equation, solve for its variable in terms of the others
- Use these equations to compute a new approximation
- Repeat steps 2-3 until convergence or a maximum number of iterations is reached
Here’s a Python implementation of the Jacobi method:
import numpy as np
def jacobi_method(A, b, max_iterations=1000, tolerance=1e-10):
n = len(b)
x = np.zeros(n)
for iteration in range(max_iterations):
x_new = np.zeros(n)
for i in range(n):
s1 = sum(A[i][j] * x[j] for j in range(n) if j != i)
x_new[i] = (b[i] - s1) / A[i][i]
if np.allclose(x, x_new, rtol=tolerance):
return x_new
x = x_new
return x
# Example usage
A = np.array([[10, -1, 2],
[-1, 11, -1],
[2, -1, 10]])
b = np.array([6, 25, -11])
solution = jacobi_method(A, b)
print("Solution:", solution)
The Jacobi method has a time complexity of O(kn²) where k is the number of iterations. It converges for diagonally dominant matrices or symmetric positive-definite matrices.
5. Gauss-Seidel Method
The Gauss-Seidel method is another iterative algorithm for solving systems of linear equations. It’s similar to the Jacobi method but often converges faster because it uses updated values as soon as they are available.
Steps of the Gauss-Seidel Method:
- Start with an initial guess for the solution
- For each equation, solve for its variable using the most recent values of the other variables
- Repeat step 2 until convergence or a maximum number of iterations is reached
Here’s a Python implementation of the Gauss-Seidel method:
import numpy as np
def gauss_seidel(A, b, max_iterations=1000, tolerance=1e-10):
n = len(b)
x = np.zeros(n)
for iteration in range(max_iterations):
x_new = np.copy(x)
for i in range(n):
s1 = sum(A[i][j] * x_new[j] for j in range(i))
s2 = sum(A[i][j] * x[j] for j in range(i + 1, n))
x_new[i] = (b[i] - s1 - s2) / A[i][i]
if np.allclose(x, x_new, rtol=tolerance):
return x_new
x = x_new
return x
# Example usage
A = np.array([[10, -1, 2],
[-1, 11, -1],
[2, -1, 10]])
b = np.array([6, 25, -11])
solution = gauss_seidel(A, b)
print("Solution:", solution)
Like the Jacobi method, the Gauss-Seidel method has a time complexity of O(kn²) where k is the number of iterations. It generally converges faster than the Jacobi method for the same types of matrices.
6. Conjugate Gradient Method
The Conjugate Gradient method is an iterative algorithm for solving systems of linear equations where the matrix is symmetric and positive-definite. It’s particularly effective for large, sparse systems.
Key Features of the Conjugate Gradient Method:
- Converges in at most n steps for an n × n matrix (in exact arithmetic)
- Each iteration involves a matrix-vector product and a few vector operations
- Can be very efficient for sparse matrices
Here’s a Python implementation of the Conjugate Gradient method:
import numpy as np
def conjugate_gradient(A, b, max_iterations=1000, tolerance=1e-10):
x = np.zeros_like(b)
r = b - np.dot(A, x)
p = r.copy()
for iteration in range(max_iterations):
Ap = np.dot(A, p)
alpha = np.dot(r, r) / np.dot(p, Ap)
x += alpha * p
r_new = r - alpha * Ap
if np.linalg.norm(r_new) < tolerance:
return x
beta = np.dot(r_new, r_new) / np.dot(r, r)
p = r_new + beta * p
r = r_new
return x
# Example usage
A = np.array([[4, 1],
[1, 3]])
b = np.array([1, 2])
solution = conjugate_gradient(A, b)
print("Solution:", solution)
The Conjugate Gradient method has a time complexity of O(kn²) where k is the number of iterations, but for sparse matrices, this can be much lower, potentially O(kn).
7. Applications of Linear Equation Solvers
Linear equation solvers have a wide range of applications across various fields:
1. Machine Learning and Data Science
- Linear Regression: Solving normal equations
- Principal Component Analysis (PCA): Eigenvalue problems
- Support Vector Machines: Quadratic programming problems
2. Computer Graphics
- 3D Transformations: Matrix operations for rotations, translations, and scaling
- Ray Tracing: Solving ray-object intersection equations
- Physics Simulations: Solving equations of motion
3. Engineering
- Structural Analysis: Solving for forces and displacements in structures
- Circuit Analysis: Solving for currents and voltages in electrical networks
- Control Systems: Solving state-space equations
4. Economics and Finance
- Portfolio Optimization: Solving for optimal asset allocations
- Input-Output Analysis: Modeling economic interdependencies
- Price Equilibrium: Solving supply and demand equations
5. Scientific Computing
- Finite Element Analysis: Solving partial differential equations
- Computational Fluid Dynamics: Solving Navier-Stokes equations
- Quantum Mechanics: Solving Schrödinger equations
8. Implementing Linear Equation Solvers in Code
When implementing linear equation solvers in code, there are several considerations to keep in mind:
1. Choice of Programming Language
Different programming languages have different strengths when it comes to numerical computing:
- Python: Excellent for prototyping and has powerful libraries like NumPy and SciPy
- MATLAB: Designed specifically for matrix operations and numerical computing
- C/C++: Offers high performance but requires more low-level implementation
- Julia: Combines ease of use with high performance for numerical computing
2. Use of Numerical Libraries
Many languages have libraries that provide optimized implementations of linear algebra operations:
- NumPy and SciPy for Python
- Eigen for C++
- LAPACK for Fortran and C
- Intel MKL for various languages
3. Handling Special Cases
Robust implementations need to handle various special cases:
- Singular matrices
- Nearly singular matrices (ill-conditioned systems)
- Systems with no solution or infinite solutions
4. Precision Considerations
The choice between single precision (float) and double precision (double) can affect both accuracy and performance:
- Double precision offers more accuracy but can be slower
- Single precision can be faster but may lead to more numerical errors
5. Parallelization
For large systems, parallelization can significantly improve performance:
- Multi-threading for shared-memory systems
- Distributed computing for very large problems
- GPU acceleration for certain types of problems
Example: Implementing Gaussian Elimination with Partial Pivoting
Here’s an implementation of Gaussian elimination with partial pivoting in C++, demonstrating some of these considerations:
#include <iostream>
#include <vector>
#include <cmath>
#include <stdexcept>
std::vector<double> gaussian_elimination(std::vector<std::vector<double>> A, std::vector<double> b) {
int n = b.size();
// Augment the matrix A with vector b
for (int i = 0; i < n; i++) {
A[i].push_back(b[i]);
}
for (int i = 0; i < n; i++) {
// Partial Pivoting
int max_row = i;
for (int k = i + 1; k < n; k++) {
if (std::abs(A[k][i]) > std::abs(A[max_row][i])) {
max_row = k;
}
}
std::swap(A[i], A[max_row]);
// Check for singular matrix
if (std::abs(A[i][i]) < 1e-10) {
throw std::runtime_error("Matrix is singular or nearly singular");
}
// Elimination
for (int k = i + 1; k < n; k++) {
double factor = A[k][i] / A[i][i];
for (int j = i; j <= n; j++) {
A[k][j] -= factor * A[i][j];
}
}
}
// Back substitution
std::vector<double> x(n);
for (int i = n - 1; i >= 0; i--) {
x[i] = A[i][n];
for (int j = i + 1; j < n; j++) {
x[i] -= A[i][j] * x[j];
}
x[i] /= A[i][i];
}
return x;
}
int main() {
std::vector<std::vector<double>> A = {{2, 1, -1},
{-3, -1, 2},
{-2, 1, 2}};
std::vector<double> b = {8, -11, -3};
try {
std::vector<double> solution = gaussian_elimination(A, b);
std::cout << "Solution: ";
for (double x : solution) {
std::cout << x << " ";
}
std::cout << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
This implementation includes error handling for singular matrices and uses partial pivoting for improved numerical stability.
9. Optimization Techniques for Linear Equation Solvers
Optimizing linear equation solvers is crucial for handling large-scale problems efficiently. Here are some techniques to improve performance:
1. Sparse Matrix Representation
For systems with many zero elements, using sparse matrix formats can significantly reduce memory usage and computation time:
- Compressed Sparse Row (CSR) format
- Compressed Sparse Column (CSC) format
- Coordinate (COO) format
2. Preconditioning
For iterative methods, preconditioning can improve convergence rates:
- Jacobi preconditioning
- Incomplete LU factorization
- Symmetric Successive Over-Relaxation (SSOR)
3. Block Algorithms
Block algorithms can improve cache utilization and enable better use of vector instructions:
- Block LU decomposition
- Block Jacobi method
- Block Conjugate Gradient method
4. Mixed Precision Algorithms
Using a combination of single and double precision can balance speed and accuracy:
- Perform main computations in single precision
- Use double precision for critical steps or refinement
5. Parallel and Distributed Algorithms
Leveraging parallel computing can dramatically speed up computations:
- Multi-threaded implementations using OpenMP or Intel Threading Building Blocks
- Distributed memory parallelism using MPI
- GPU acceleration using CUDA or OpenCL
6. Algorithm Selection and Hybrid Methods
Choosing the right algorithm or combining methods can lead to significant improvements:
- Use direct methods for small, dense systems
- Use iterative methods for large, sparse systems
- Combine direct and iterative methods for certain problem structures
Example: Sparse Matrix-Vector Multiplication
Here’s an example of how to implement sparse matrix-vector multiplication using the CSR format in C++:
#include <vector>
#include <iostream>
struct CSRMatrix {
std::vector<double> values;
std::vector<int> column_indices;
std::vector<int> row_pointers;
};
std::vector<double> csr_matrix_vector_multiply(const CSRMatrix& A, const std::vector<double>& x) {
std::vector<double> y(A.row_pointers.size() - 1, 0.0);
#pragma omp parallel for
for (int i = 0; i < y.size(); i++) {
for (int j = A.row_pointers[i]; j < A.row_pointers[i+1]; j++) {
y[i] += A.values[j] * x[A.column_indices[j]];
}
}
return y;
}
int main() {
CSRMatrix A;
A.values = {1, 2, 3, 4, 5, 6};
A.column_indices = {0, 2, 1, 0, 1, 2};
A.row_pointers = {0, 2, 4, 6};
std::vector<double> x = {1, 2, 3};
std::vector<double> y = csr_matrix_vector_multiply(A, x);
std::cout << "Result: ";
for (double val : y) {
std::cout << val << " ";
}
std::cout << std::endl;
return 0;
}
This implementation uses OpenMP for parallelization and the CSR format for efficient storage and computation with sparse matrices.
10. Conclusion
Solving linear equations is a fundamental task in many areas of science, engineering, and computer science. The choice of algorithm depends on the specific characteristics of the problem, such as the size and structure of the system, the required accuracy, and the available computational resources.
Direct methods like Gaussian elimination and LU decomposition are excellent for small to medium-sized dense systems, offering exact solutions (barring numerical errors). Iterative methods like Jacobi, Gauss-Seidel, and Conjugate Gradient are more suitable for large, sparse systems, where they can converge to an approximate solution much faster than direct methods.
As we’ve seen, implementing these algorithms efficiently requires careful consideration of various factors, including:
- The choice of programming language and numerical libraries
- Proper handling of special cases and numerical stability issues
- Optimization techniques such as sparse matrix representations and parallelization
- The trade-offs between accuracy and performance
As problems in science and engineering continue to grow in scale and complexity, the development of more efficient and robust linear equation solvers remains an active area of research. Advances in hardware, such as increasingly powerful GPUs and specialized AI accelerators, are also opening up new possibilities for tackling even larger systems of equations.
By mastering these algorithms and techniques, developers and data scientists can tackle a wide range of real-world problems, from optimizing large-scale machine learning models to simulating complex physical systems. The ability to efficiently solve linear equations is truly a fundamental skill in the toolkit of any computational scientist or engineer.