Software Testing Methodologies: A Comprehensive Guide for Modern Developers
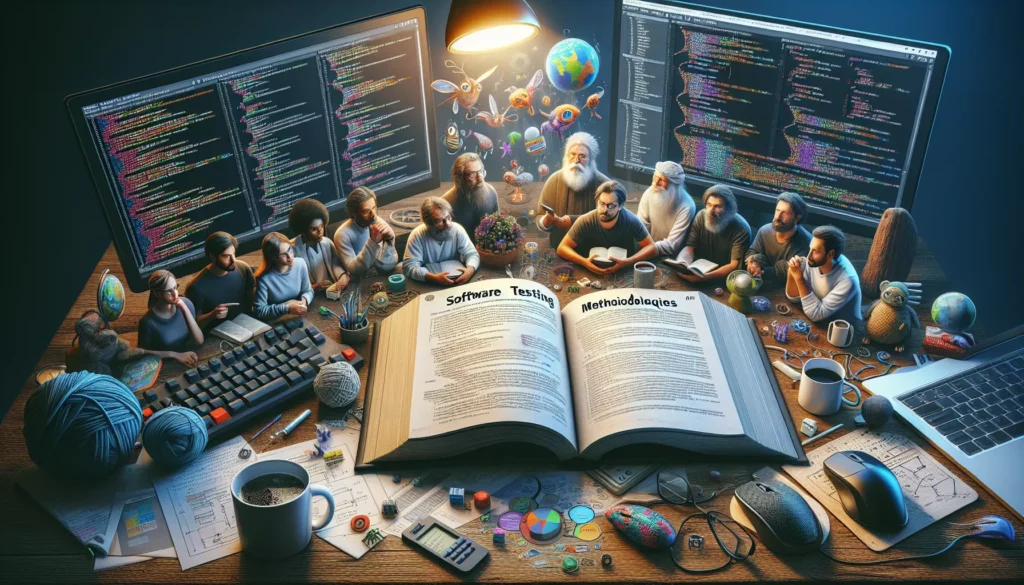
In the ever-evolving landscape of software development, ensuring the quality and reliability of applications is paramount. As coding education platforms like AlgoCademy continue to prepare aspiring developers for careers in tech giants like FAANG companies, understanding various software testing methodologies becomes increasingly crucial. This comprehensive guide will delve into the world of software testing, exploring different methodologies, their applications, and how they contribute to creating robust, error-free software.
Table of Contents
- Introduction to Software Testing
- The Importance of Software Testing
- Types of Software Testing
- Software Testing Methodologies
- Agile Testing Methodologies
- Test Automation
- Best Practices in Software Testing
- Popular Testing Tools and Frameworks
- The Future of Software Testing
- Conclusion
1. Introduction to Software Testing
Software testing is a critical process in the software development lifecycle that involves evaluating and verifying that a software application or program works as expected. It’s an integral part of quality assurance (QA) and aims to identify bugs, errors, and defects in the software before it reaches the end-user.
As platforms like AlgoCademy focus on developing strong coding skills and algorithmic thinking, it’s equally important for aspiring developers to understand how to validate their code through effective testing methodologies. This knowledge not only improves the quality of software but also prepares developers for the rigorous standards expected in top tech companies.
2. The Importance of Software Testing
The significance of software testing cannot be overstated in today’s digital landscape. Here are some key reasons why testing is crucial:
- Quality Assurance: Testing ensures that the software meets the required quality standards and functions as intended.
- Cost-Effectiveness: Identifying and fixing bugs early in the development process is far less expensive than addressing issues post-release.
- Security: Testing helps identify vulnerabilities and security loopholes, crucial for protecting user data and maintaining trust.
- User Satisfaction: Well-tested software provides a better user experience, leading to higher user satisfaction and retention.
- Compliance: Many industries have regulatory requirements that mandate thorough testing of software systems.
3. Types of Software Testing
Before diving into specific methodologies, it’s important to understand the various types of software testing. These can be broadly categorized into functional and non-functional testing:
Functional Testing
- Unit Testing: Testing individual components or functions of the software.
- Integration Testing: Verifying that different modules or services work well together.
- System Testing: Testing the system as a whole to ensure it meets specified requirements.
- Acceptance Testing: Determining if the system satisfies the acceptance criteria and is acceptable for delivery.
Non-Functional Testing
- Performance Testing: Evaluating how the system performs under various conditions.
- Security Testing: Identifying vulnerabilities and security risks in the system.
- Usability Testing: Assessing how user-friendly and intuitive the software is.
- Compatibility Testing: Ensuring the software works across different environments, devices, and configurations.
4. Software Testing Methodologies
Software testing methodologies are structured approaches to testing that help ensure comprehensive coverage and efficiency. Here are some of the most widely used methodologies:
Waterfall Model
In the Waterfall model, testing is performed sequentially after the development phase is complete. While this model is less flexible, it can be effective for projects with well-defined requirements and minimal expected changes.
V-Model
The V-Model, or Verification and Validation model, aligns each development stage with a corresponding testing phase. This model emphasizes early test planning and provides a structured approach to testing.
Incremental Testing
This methodology involves testing each increment or build of the software as it’s developed. It allows for earlier detection of issues and more frequent feedback.
Spiral Model
The Spiral model combines elements of both design and prototyping. Testing is performed iteratively, with each iteration including risk analysis and evaluation.
5. Agile Testing Methodologies
Agile methodologies have revolutionized software development, and testing practices have evolved to align with these approaches. Agile testing emphasizes continuous testing throughout the development process.
Test-Driven Development (TDD)
TDD involves writing tests before writing the actual code. This approach ensures that code is testable from the start and helps developers think through requirements and design before implementation.
Here’s a simple example of TDD in Python:
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
def test_add_mixed_numbers(self):
self.assertEqual(add(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
Behavior-Driven Development (BDD)
BDD extends TDD by focusing on the behavior of the system from the user’s perspective. It often uses a natural language construct to describe tests, making it easier for non-technical stakeholders to understand and contribute to the testing process.
Acceptance Test-Driven Development (ATDD)
ATDD involves creating acceptance tests based on the user’s requirements before development begins. This ensures that the development team has a clear understanding of the desired outcome from the user’s perspective.
6. Test Automation
Test automation is a crucial aspect of modern software testing methodologies. It involves using specialized tools to control the execution of tests and compare actual outcomes with predicted outcomes.
Benefits of Test Automation
- Faster execution of repetitive tests
- Improved test coverage
- Earlier detection of defects
- Reduced human error in testing
- Ability to run tests that are difficult to perform manually
Automation Testing Process
- Test Tool Selection
- Define Scope of Automation
- Planning, Design, and Development
- Test Execution
- Maintenance
Here’s a simple example of an automated test using Python’s unittest framework:
import unittest
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
class PythonOrgSearch(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Firefox()
def test_search_in_python_org(self):
driver = self.driver
driver.get("http://www.python.org")
self.assertIn("Python", driver.title)
elem = driver.find_element_by_name("q")
elem.send_keys("pycon")
elem.send_keys(Keys.RETURN)
assert "No results found." not in driver.page_source
def tearDown(self):
self.driver.close()
if __name__ == "__main__":
unittest.main()
7. Best Practices in Software Testing
To ensure effective and efficient testing, consider the following best practices:
- Start Testing Early: Begin testing as soon as possible in the development lifecycle.
- Test Planning: Develop a comprehensive test plan that outlines the testing strategy, objectives, and schedule.
- Prioritize Tests: Focus on critical functionality and high-risk areas first.
- Maintain Test Independence: Ensure that tests are independent of each other to avoid cascading failures.
- Use Realistic Data: Test with data that closely resembles real-world scenarios.
- Continuous Integration and Continuous Testing: Implement CI/CD pipelines that include automated testing.
- Code Reviews: Conduct peer reviews of test code to ensure quality and maintainability.
- Regular Reporting: Provide clear, concise reports on test results and progress.
8. Popular Testing Tools and Frameworks
There are numerous tools and frameworks available for software testing. Here are some popular ones:
Unit Testing Frameworks
- JUnit (Java)
- NUnit (.NET)
- PyTest (Python)
- Mocha (JavaScript)
Automation Testing Tools
- Selenium (Web Applications)
- Appium (Mobile Applications)
- JMeter (Performance Testing)
- Postman (API Testing)
Continuous Integration Tools
- Jenkins
- Travis CI
- CircleCI
Here’s an example of a simple unit test using PyTest:
import pytest
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
def test_factorial():
assert factorial(0) == 1
assert factorial(1) == 1
assert factorial(5) == 120
with pytest.raises(RecursionError):
factorial(1000) # This should raise a RecursionError
9. The Future of Software Testing
As technology continues to evolve, so do software testing methodologies. Some emerging trends in software testing include:
- AI and Machine Learning in Testing: Using AI to generate test cases, predict defects, and optimize test execution.
- IoT Testing: As the Internet of Things grows, testing for IoT devices and ecosystems becomes more critical.
- Big Data Testing: Ensuring the accuracy and performance of systems handling large volumes of data.
- DevOps and Continuous Testing: Further integration of testing into the development process, with an emphasis on continuous feedback and improvement.
- Shift-Left Testing: Moving testing earlier in the development cycle to catch issues sooner and reduce costs.
10. Conclusion
Software testing is an indispensable part of the software development process. As we’ve explored in this comprehensive guide, there are various methodologies and approaches to ensure the quality and reliability of software applications. From traditional methods like the Waterfall model to modern Agile practices like TDD and BDD, each approach has its strengths and applications.
For aspiring developers, particularly those using platforms like AlgoCademy to prepare for roles in top tech companies, understanding these testing methodologies is crucial. It not only helps in creating more robust and reliable code but also aligns with the industry’s best practices and expectations.
As the field of software development continues to evolve, staying updated with the latest testing trends and tools will be key to success. By embracing comprehensive testing practices, developers can ensure they’re creating high-quality software that meets user needs and stands up to the rigorous standards of the tech industry.
Remember, effective testing is not just about finding bugs; it’s about improving the overall quality of the software and providing confidence in its functionality. As you continue your journey in software development, make testing an integral part of your coding practice, and you’ll be well-prepared for the challenges and opportunities that lie ahead in your career.