Software Maintenance and Legacy Systems: Navigating the Challenges of Aging Code
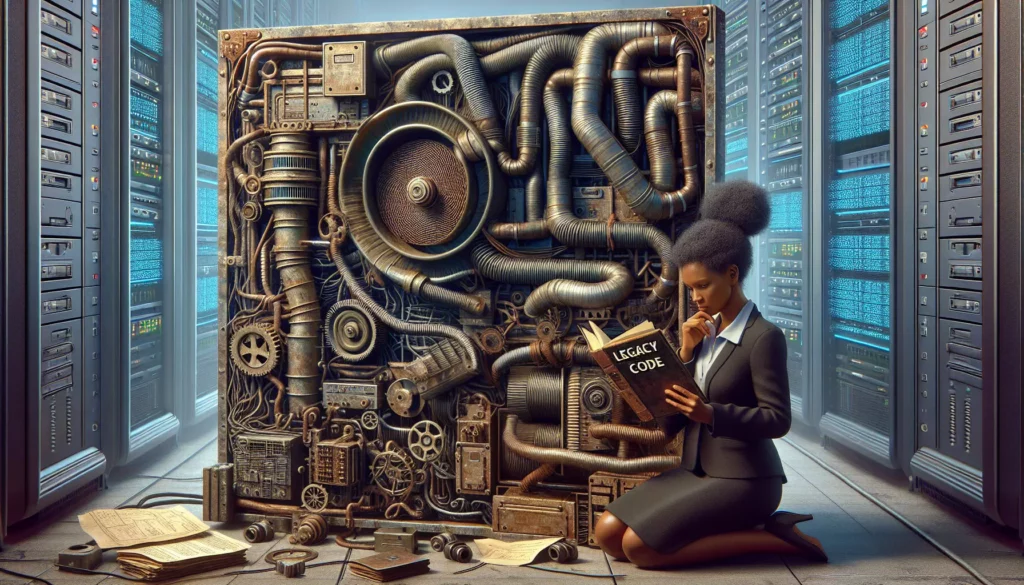
In the fast-paced world of technology, where new programming languages and frameworks seem to emerge almost daily, it’s easy to get caught up in the excitement of cutting-edge development. However, a significant portion of the software industry revolves around maintaining and updating existing systems, many of which could be classified as “legacy” software. In this comprehensive guide, we’ll dive deep into the world of software maintenance and legacy systems, exploring the challenges, strategies, and best practices that developers need to know to effectively manage older software.
Understanding Software Maintenance
Software maintenance is the process of modifying and updating existing software systems after they have been deployed. This can include fixing bugs, improving performance, adding new features, or adapting the software to work with new hardware or operating systems. Maintenance is a critical aspect of the software development lifecycle, often consuming more time and resources than the initial development phase.
Types of Software Maintenance
There are four main types of software maintenance:
- Corrective Maintenance: Fixing bugs and errors in the software.
- Adaptive Maintenance: Modifying the software to work with changes in the environment, such as new hardware or operating systems.
- Perfective Maintenance: Improving the software’s performance or adding new features.
- Preventive Maintenance: Updating the software to prevent future problems.
What Are Legacy Systems?
Legacy systems are older software applications or platforms that continue to be used by an organization despite being outdated or obsolete. These systems often play critical roles in business operations but may be difficult to maintain or update due to their age, complexity, or lack of documentation.
Characteristics of Legacy Systems
- Outdated technology or programming languages
- Lack of vendor support
- Limited documentation
- Difficulty integrating with modern systems
- High maintenance costs
- Critical to business operations
Challenges in Maintaining Legacy Systems
Maintaining legacy systems presents several unique challenges for developers and organizations:
1. Lack of Documentation
Many legacy systems suffer from poor or outdated documentation. This can make it difficult for new developers to understand the system’s architecture, code structure, and business logic.
2. Outdated Technologies
Legacy systems often use obsolete programming languages, frameworks, or libraries. This can make it challenging to find developers with the necessary skills to maintain the system.
3. Integration Issues
Integrating legacy systems with modern technologies and platforms can be complex and time-consuming.
4. Security Vulnerabilities
Older systems may not have been designed with modern security practices in mind, making them vulnerable to cyber attacks.
5. Performance Issues
Legacy systems may struggle to handle increased workloads or modern performance expectations.
6. Scalability Limitations
Many legacy systems were not designed with scalability in mind, making it difficult to adapt to growing business needs.
Strategies for Maintaining Legacy Systems
Despite these challenges, there are several strategies that organizations can employ to effectively maintain and update legacy systems:
1. Code Refactoring
Refactoring involves restructuring existing code without changing its external behavior. This can improve readability, reduce complexity, and make the system easier to maintain.
Example of refactoring a complex function:
// Before refactoring
function calculateTotal(items) {
let total = 0;
for (let i = 0; i < items.length; i++) {
if (items[i].type === 'A') {
total += items[i].price * 0.9; // 10% discount
} else if (items[i].type === 'B') {
total += items[i].price * 0.95; // 5% discount
} else {
total += items[i].price;
}
}
return total;
}
// After refactoring
function calculateTotal(items) {
return items.reduce((total, item) => total + applyDiscount(item), 0);
}
function applyDiscount(item) {
const discounts = {
'A': 0.9,
'B': 0.95,
'default': 1
};
return item.price * (discounts[item.type] || discounts['default']);
}
2. Incremental Modernization
Instead of completely rewriting a legacy system, consider modernizing it incrementally. This approach involves gradually replacing or updating components of the system over time.
3. Automated Testing
Implementing automated tests can help ensure that changes to the legacy system don’t introduce new bugs or break existing functionality.
import unittest
class TestLegacySystem(unittest.TestCase):
def test_calculate_total(self):
items = [
{'type': 'A', 'price': 100},
{'type': 'B', 'price': 200},
{'type': 'C', 'price': 300}
]
expected_total = 90 + 190 + 300 # 555
self.assertEqual(calculateTotal(items), expected_total)
if __name__ == '__main__':
unittest.main()
4. Documentation Improvement
Invest time in improving and updating documentation for the legacy system. This can include code comments, architecture diagrams, and user manuals.
5. Containerization
Using containerization technologies like Docker can help isolate legacy applications and make them easier to deploy and manage in modern environments.
# Dockerfile for a legacy PHP application
FROM php:7.2-apache
COPY . /var/www/html/
RUN docker-php-ext-install mysqli
EXPOSE 80
6. API Wrappers
Create modern API wrappers around legacy systems to improve integration with newer applications and services.
from flask import Flask, jsonify
import legacy_system
app = Flask(__name__)
@app.route('/api/v1/calculate_total', methods=['POST'])
def api_calculate_total():
items = request.json['items']
total = legacy_system.calculateTotal(items)
return jsonify({'total': total})
if __name__ == '__main__':
app.run(debug=True)
Best Practices for Software Maintenance
To effectively maintain and update legacy systems, consider the following best practices:
1. Understand the System
Before making any changes, take the time to thoroughly understand the legacy system’s architecture, code structure, and business logic.
2. Version Control
Use a version control system like Git to track changes and manage different versions of the codebase.
git init
git add .
git commit -m "Initial commit of legacy system"
git branch feature/modernization
git checkout feature/modernization
3. Continuous Integration and Deployment
Implement CI/CD pipelines to automate testing and deployment processes, ensuring that changes are thoroughly tested before being applied to the production system.
4. Code Review
Establish a code review process to ensure that changes to the legacy system are properly vetted and adhere to best practices.
5. Performance Monitoring
Use monitoring tools to track the performance of the legacy system and identify areas for improvement.
6. Regular Updates
Keep the system’s dependencies, libraries, and frameworks as up-to-date as possible to minimize security vulnerabilities and improve compatibility with modern systems.
7. Knowledge Transfer
Ensure that knowledge about the legacy system is shared among team members to prevent a single point of failure.
When to Consider Replacing a Legacy System
While maintenance can extend the life of a legacy system, there may come a time when replacement is the best option. Consider replacing a legacy system when:
- The cost of maintenance exceeds the cost of replacement
- The system can no longer meet business requirements
- Security vulnerabilities cannot be adequately addressed
- The system is hindering business growth or innovation
- Support for critical components is no longer available
Preparing for Legacy System Replacement
If you decide to replace a legacy system, follow these steps to ensure a smooth transition:
- Thoroughly document the current system’s functionality and business processes
- Identify and prioritize requirements for the new system
- Plan for data migration from the old system to the new one
- Develop a phased approach to replace the system incrementally if possible
- Provide training and support for users transitioning to the new system
- Run the old and new systems in parallel during the transition period
- Establish clear success criteria for the replacement project
The Role of DevOps in Legacy System Maintenance
DevOps practices can significantly improve the maintenance and updating of legacy systems. By adopting DevOps principles, organizations can:
- Automate testing and deployment processes
- Improve collaboration between development and operations teams
- Implement continuous monitoring and feedback loops
- Reduce the time and risk associated with updates and changes
- Enhance system reliability and stability
Conclusion
Software maintenance and managing legacy systems are critical skills for any developer or organization working with established software applications. While these tasks can be challenging, they are essential for ensuring the continued functionality, security, and relevance of older systems that often play crucial roles in business operations.
By understanding the challenges associated with legacy systems and employing effective maintenance strategies, developers can extend the life of these systems, improve their performance, and gradually modernize them to meet current business needs. Whether you’re refactoring code, implementing automated testing, or planning for a system replacement, the key is to approach the task with a clear strategy and a commitment to best practices.
As you continue your journey in software development, remember that working with legacy systems can be an invaluable learning experience. It provides insights into the evolution of technology, teaches you to navigate complex codebases, and hones your problem-solving skills. Embrace the challenges of software maintenance, and you’ll become a more well-rounded and versatile developer in the process.
Keep learning, stay curious, and always look for opportunities to improve and modernize the systems you work with. The skills you develop in maintaining and updating legacy systems will serve you well throughout your career in software development.