Software Localization and Globalization: Adapting Your Code for a Global Audience
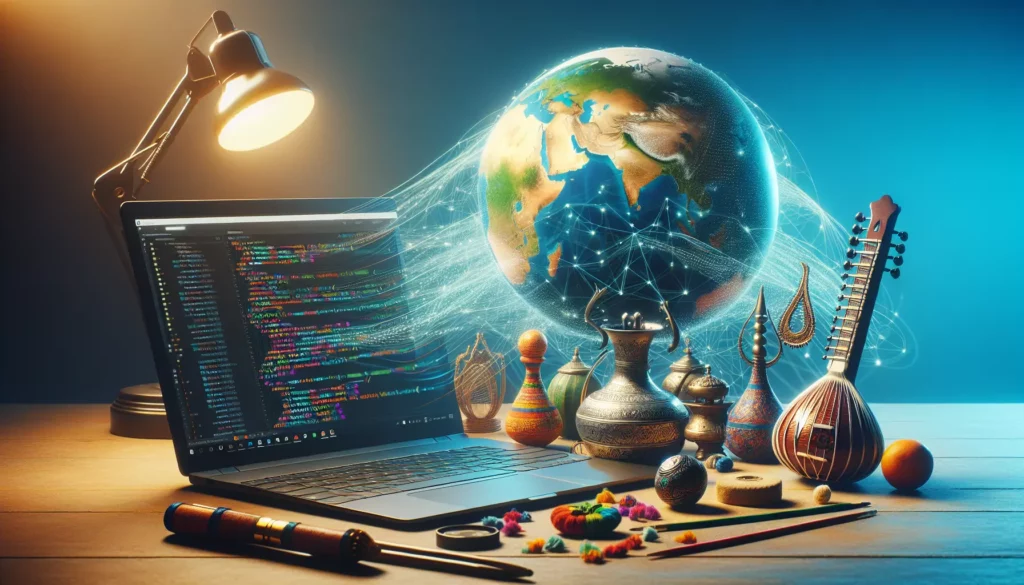
In today’s interconnected world, software applications are no longer confined to a single region or language. As developers, we must consider the global reach of our products and ensure they can be easily adapted to different cultures and regions. This process, known as software localization and globalization, is crucial for creating inclusive and user-friendly applications that can succeed in the international market. In this comprehensive guide, we’ll explore the concepts of software localization and globalization, their importance, and how to implement them effectively in your coding projects.
Understanding Software Localization and Globalization
Before we dive into the specifics, let’s clarify the difference between localization and globalization:
Software Localization
Software localization is the process of adapting a software application to meet the linguistic, cultural, and technical requirements of a specific target market or locale. This involves translating the user interface, adjusting date and time formats, currency symbols, and other locale-specific elements.
Software Globalization
Software globalization, on the other hand, is the practice of designing and developing software that can be easily adapted to various languages and regions without requiring significant changes to the source code. It involves creating a flexible architecture that supports multiple languages, character sets, and cultural conventions from the ground up.
While localization focuses on adapting an existing product for a specific market, globalization is about creating a product that can be easily localized for multiple markets.
The Importance of Software Localization and Globalization
Implementing localization and globalization strategies in your software development process offers several benefits:
- Expanded market reach: By making your software accessible to users in different countries and languages, you can significantly increase your potential user base and market share.
- Improved user experience: Users are more likely to engage with and enjoy using software that speaks their language and respects their cultural norms.
- Competitive advantage: In a global marketplace, offering localized versions of your software can give you an edge over competitors who haven’t invested in localization.
- Legal compliance: Some countries have regulations requiring software to be available in local languages or to adhere to specific cultural standards.
- Increased revenue: By catering to a wider audience, you can potentially increase your software’s revenue and return on investment.
Key Aspects of Software Localization
When localizing software, there are several key aspects to consider:
1. Language Translation
The most obvious aspect of localization is translating the user interface text, error messages, and documentation into the target language. This process goes beyond simple word-for-word translation and requires consideration of context, idiomatic expressions, and cultural nuances.
2. Date and Time Formats
Different regions use various date and time formats. For example:
- USA: MM/DD/YYYY (e.g., 04/15/2023)
- Europe: DD/MM/YYYY (e.g., 15/04/2023)
- Japan: YYYY年MM月DD日 (e.g., 2023年04月15日)
Your software should be able to display dates and times in the format appropriate for the user’s locale.
3. Number and Currency Formats
Number formatting, including decimal and thousands separators, varies between regions. For example:
- USA: 1,234.56
- Germany: 1.234,56
- India: 1,23,456.78
Currency symbols and their placement also differ (e.g., $100 vs 100€).
4. Text Direction
While many languages are written left-to-right (LTR), some languages like Arabic and Hebrew are written right-to-left (RTL). Your user interface should be able to accommodate both text directions.
5. Cultural Considerations
Be aware of cultural sensitivities when designing icons, choosing colors, or using imagery. What’s acceptable in one culture may be offensive in another.
6. Legal Requirements
Different countries may have specific legal requirements for software, such as data protection laws or accessibility standards. Ensure your localized versions comply with local regulations.
Implementing Globalization in Your Code
To create globally-ready software, consider the following practices:
1. Separate UI Text from Code
Store all user-facing text in separate resource files instead of hardcoding them in your source code. This makes it easier to translate and manage different language versions.
For example, in a Java application, you might use properties files:
// messages_en.properties
greeting=Hello, world!
// messages_fr.properties
greeting=Bonjour, le monde!
Then, in your code, you can load the appropriate resource bundle based on the user’s locale:
import java.util.ResourceBundle;
import java.util.Locale;
public class LocalizedApp {
public static void main(String[] args) {
Locale locale = new Locale("fr", "FR");
ResourceBundle messages = ResourceBundle.getBundle("messages", locale);
System.out.println(messages.getString("greeting"));
}
}
2. Use Unicode for Character Encoding
Unicode supports characters from virtually all of the world’s writing systems. Using Unicode (e.g., UTF-8) as your character encoding ensures that your software can handle text in any language.
3. Implement Flexible UI Layouts
Design your user interface to accommodate text expansion and contraction. Translated text can be significantly longer or shorter than the original, so your UI should be able to adjust dynamically.
In web development, you can use CSS flexbox or grid layouts to create flexible designs:
<!-- HTML -->
<div class="container">
<div class="item">Label:</div>
<div class="item">Value</div>
</div>
/* CSS */
.container {
display: flex;
flex-wrap: wrap;
}
.item {
flex: 1 1 auto;
min-width: 100px;
}
4. Use Locale-Aware Functions for Formatting
Many programming languages and frameworks provide locale-aware functions for formatting dates, numbers, and currencies. Use these instead of implementing your own formatting logic.
For example, in JavaScript:
const number = 1234567.89;
const date = new Date("2023-04-15");
// US English formatting
console.log(new Intl.NumberFormat("en-US").format(number)); // 1,234,567.89
console.log(new Intl.DateTimeFormat("en-US").format(date)); // 4/15/2023
// German formatting
console.log(new Intl.NumberFormat("de-DE").format(number)); // 1.234.567,89
console.log(new Intl.DateTimeFormat("de-DE").format(date)); // 15.4.2023
5. Support Right-to-Left (RTL) Languages
For web applications, you can use the dir
attribute to specify text direction:
<html lang="ar" dir="rtl">
<!-- Arabic content here -->
</html>
In CSS, you can use logical properties to create layouts that work for both LTR and RTL languages:
.container {
margin-inline-start: 10px; /* Instead of margin-left */
padding-inline-end: 20px; /* Instead of padding-right */
}
6. Use Pluralization Rules
Different languages have different rules for pluralization. Instead of using simple singular/plural forms, implement a system that can handle complex pluralization rules.
For example, using the ICU message format:
const messages = {
en: {
items: "{count, plural, =0{No items} one{# item} other{# items}}"
},
ru: {
items: "{count, plural, =0{Ðет Ñлементов} one{# Ñлемент} few{# Ñлемента} many{# Ñлементов} other{# Ñлементов}}"
}
};
function formatMessage(locale, count) {
const formatter = new Intl.MessageFormat(messages[locale].items, locale);
return formatter.format({ count });
}
console.log(formatMessage("en", 0)); // No items
console.log(formatMessage("en", 1)); // 1 item
console.log(formatMessage("en", 5)); // 5 items
console.log(formatMessage("ru", 0)); // Ðет Ñлементов
console.log(formatMessage("ru", 1)); // 1 Ñлемент
console.log(formatMessage("ru", 3)); // 3 Ñлемента
console.log(formatMessage("ru", 5)); // 5 Ñлементов
Best Practices for Software Localization and Globalization
To ensure successful localization and globalization of your software, consider the following best practices:
1. Plan for Localization from the Start
Incorporate localization considerations into your software design and development process from the beginning. It’s much easier and cost-effective to create a globally-ready application from the start than to retrofit an existing application for localization.
2. Use Localization Tools and Frameworks
Take advantage of localization tools and frameworks that can streamline the process. These tools can help manage translations, automate resource file generation, and provide testing capabilities for different locales.
Some popular localization tools and frameworks include:
- GNU gettext
- React-Intl
- Angular’s i18n
- Django’s internationalization framework
- Rails I18n
3. Implement a Robust Testing Strategy
Thoroughly test your application in different locales to ensure that all localized elements work correctly. This includes:
- Testing with various language packs
- Verifying date, time, and number formats
- Checking text rendering and layout in different languages
- Testing RTL layouts
- Verifying that all functionality works correctly in each locale
4. Use Professional Translation Services
While machine translation has improved significantly, it’s still not perfect for software localization. Invest in professional human translators who understand both the source and target languages, as well as the context of your software.
5. Provide Context for Translators
Give translators as much context as possible about where and how each string is used in your application. This can help avoid misunderstandings and ensure more accurate translations.
6. Use String Concatenation Sparingly
Avoid constructing sentences by concatenating translated fragments. Word order can vary significantly between languages, making such constructions difficult to translate correctly.
Instead of:
const message = t("Welcome") + " " + userName + "! " + t("You have") + " " + unreadCount + " " + t("unread messages");
Use a single, parameterized message:
const message = t("Welcome {userName}! You have {unreadCount} unread messages", { userName, unreadCount });
7. Keep Resource Files Updated
As your software evolves, make sure to update all language resource files consistently. Implement a process to flag new or modified strings that need translation.
8. Consider Cultural Differences in UX Design
Remember that user expectations and preferences can vary significantly between cultures. Consider conducting user research in your target markets to inform your UX design decisions.
Challenges in Software Localization and Globalization
While implementing localization and globalization strategies can greatly benefit your software, it’s important to be aware of potential challenges:
1. Increased Development Time and Cost
Creating globally-ready software requires additional planning, development, and testing time. It also involves ongoing costs for translation and maintenance of multiple language versions.
2. Complexity in Code Management
Managing multiple language resources and ensuring consistency across all localized versions can increase the complexity of your codebase and development process.
3. Cultural Sensitivities
Navigating cultural differences and avoiding unintentional offense can be challenging, especially when dealing with many different target markets.
4. Technical Limitations
Some programming languages or frameworks may have limited support for certain localization features, requiring custom solutions or workarounds.
5. Maintenance and Updates
Keeping all localized versions up-to-date and in sync with the main version of your software can be a significant ongoing effort.
Conclusion
Software localization and globalization are essential considerations in today’s global software market. By implementing these practices, you can create more inclusive, user-friendly applications that can succeed in diverse markets around the world.
Remember that localization and globalization are ongoing processes, not one-time tasks. As your software evolves, continue to refine and improve your localization strategies to ensure the best possible experience for users across all supported locales.
By mastering these skills, you’ll be better equipped to create software that truly speaks the language of your users, wherever they may be. As you continue your journey in coding education and skill development, keep these principles in mind to create more versatile and globally-ready applications.