Software Localization: Adapting Your Code for a Global Audience
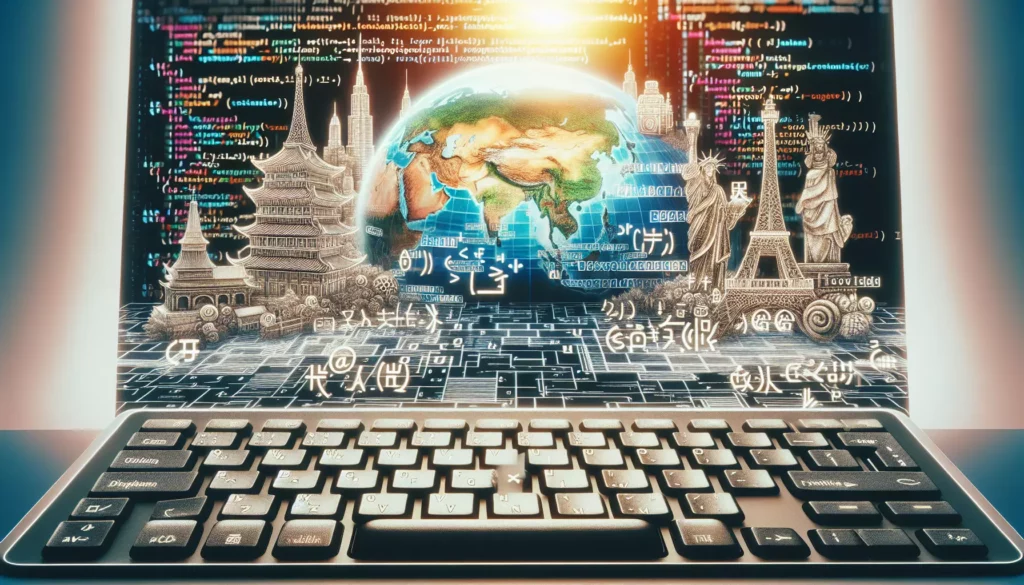
In today’s interconnected world, software development isn’t just about writing code that works; it’s about creating applications that can reach and resonate with users across different cultures and languages. This is where software localization comes into play. As developers, understanding and implementing localization techniques is crucial for expanding your software’s reach and ensuring its success in the global market. In this comprehensive guide, we’ll dive deep into the world of software localization, exploring its importance, best practices, and how to implement it effectively in your projects.
What is Software Localization?
Software localization is the process of adapting a software application to meet the linguistic, cultural, and technical requirements of a specific target market or locale. It goes beyond simple translation and involves tailoring various aspects of the software to suit the preferences and expectations of users in different regions.
Localization encompasses several key areas:
- Translation of user interface text
- Adaptation of graphics and icons
- Modification of date, time, and number formats
- Adjustment of character encoding and input methods
- Compliance with local regulations and legal requirements
- Cultural considerations in design and functionality
Why is Software Localization Important?
The importance of software localization cannot be overstated in our globalized economy. Here are some key reasons why localizing your software is crucial:
- Expanded Market Reach: Localization allows your software to be accessible and appealing to users in different countries, significantly expanding your potential user base.
- Improved User Experience: Users are more likely to engage with and appreciate software that speaks their language and respects their cultural norms.
- Increased User Adoption: Localized software tends to have higher adoption rates as it feels more familiar and user-friendly to the target audience.
- Competitive Advantage: In markets where localization is not common, offering a localized version of your software can give you a significant edge over competitors.
- Legal Compliance: Some countries have regulations requiring software to be available in local languages, making localization a legal necessity in certain markets.
Key Aspects of Software Localization
1. Language Translation
The most obvious aspect of localization is translating the user interface text, error messages, and documentation into the target language. However, this is not as straightforward as it might seem. Consider the following points:
- Context is Key: Translators need to understand the context in which strings are used to provide accurate translations.
- String Length Variations: Translated text may be longer or shorter than the original, which can affect UI layout.
- Idiomatic Expressions: Literal translations of idioms or colloquialisms may not make sense in other languages.
Here’s an example of how you might structure your code to support multiple languages:
// Localization.js
const translations = {
en: {
greeting: "Hello, world!",
button: "Click me"
},
es: {
greeting: "¡Hola, mundo!",
button: "Haz clic aquÃ"
},
// Add more languages as needed
};
function getLocalizedString(key, lang = 'en') {
return translations[lang][key] || translations['en'][key];
}
// Usage
console.log(getLocalizedString('greeting', 'es')); // Outputs: ¡Hola, mundo!
2. Date, Time, and Number Formats
Different regions have varying conventions for displaying dates, times, and numbers. Your software should adapt to these local formats to avoid confusion. For instance:
- Date format: MM/DD/YYYY (US) vs. DD/MM/YYYY (many European countries)
- Time format: 12-hour clock (with AM/PM) vs. 24-hour clock
- Number format: Use of comma or period as decimal separator
Here’s an example of how you might handle date formatting in JavaScript:
function formatDate(date, locale) {
return new Intl.DateTimeFormat(locale, {
year: 'numeric',
month: 'long',
day: 'numeric'
}).format(date);
}
const date = new Date('2023-05-15');
console.log(formatDate(date, 'en-US')); // May 15, 2023
console.log(formatDate(date, 'de-DE')); // 15. Mai 2023
3. Character Encoding and Input Methods
Ensure your software supports different character encodings to properly display text in various languages. Unicode, particularly UTF-8, is widely used for its comprehensive character support. Additionally, consider input methods for languages with large character sets or complex writing systems.
4. Graphics and Icons
Visual elements may need to be adapted for different cultures. Some considerations include:
- Colors: Different colors have varying connotations in different cultures.
- Symbols: Certain symbols may be inappropriate or misunderstood in some cultures.
- Images: Ensure images are culturally appropriate and relatable to the target audience.
5. Text Direction
Some languages, like Arabic and Hebrew, are written from right to left (RTL). Your software should support both left-to-right (LTR) and RTL text directions. This often requires adjustments to the UI layout and text alignment.
Here’s an example of how you might handle text direction in CSS:
.rtl-support {
direction: rtl;
text-align: right;
}
/* Usage in HTML */
<div class="rtl-support">
هذا نص باللغة العربية
</div>
Best Practices for Software Localization
1. Internationalization (i18n) First
Internationalization is the process of designing and developing software that can be easily localized. It’s crucial to implement i18n from the start of your project rather than as an afterthought. This involves:
- Separating user-facing strings from the code
- Using locale-independent methods for date, time, and number formatting
- Designing flexible layouts that can accommodate text expansion
2. Use Localization Frameworks and Libraries
Leverage existing localization frameworks and libraries to simplify the process. Some popular options include:
- React-i18next for React applications
- Angular’s built-in i18n support
- Flask-Babel for Python Flask applications
- Rails i18n for Ruby on Rails projects
Here’s an example using react-i18next:
import React from 'react';
import { useTranslation } from 'react-i18next';
function MyComponent() {
const { t } = useTranslation();
return (
<div>
<h1>{t('welcome.title')}</h1>
<p>{t('welcome.message')}</p>
</div>
);
}
3. Implement a Translation Management System (TMS)
A TMS can help streamline the translation process by:
- Centralizing string management
- Providing context for translators
- Tracking translation progress
- Facilitating collaboration between developers and translators
4. Context-Aware Translation
Provide translators with context for each string to ensure accurate translations. This can include:
- Screenshots of where the string appears in the UI
- Descriptions of the string’s purpose
- Variable placeholders and their meanings
5. Test Thoroughly
Localization testing is crucial to ensure a high-quality user experience. This includes:
- Linguistic testing: Checking for translation accuracy and cultural appropriateness
- Functional testing: Ensuring the software works correctly in all localized versions
- UI testing: Verifying that the layout adapts well to different languages
- Pseudo-localization: Testing with artificially lengthened strings to catch potential layout issues
Implementing Localization in Your Project
Now that we’ve covered the key aspects and best practices of software localization, let’s walk through a step-by-step process of implementing localization in a web application.
Step 1: Set Up the Project Structure
Organize your project to separate localizable content from the code. A common structure might look like this:
project/
├── src/
│ ├── components/
│ ├── locales/
│ │ ├── en/
│ │ │ └── translation.json
│ │ ├── es/
│ │ │ └── translation.json
│ │ └── ...
│ └── App.js
├── package.json
└── ...
Step 2: Install Localization Libraries
For a React application, you might use i18next and react-i18next. Install them using npm or yarn:
npm install i18next react-i18next
Step 3: Configure i18next
Create a configuration file for i18next, typically named i18n.js
:
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
import enTranslations from './locales/en/translation.json';
import esTranslations from './locales/es/translation.json';
i18n
.use(initReactI18next)
.init({
resources: {
en: { translation: enTranslations },
es: { translation: esTranslations },
},
lng: 'en', // default language
fallbackLng: 'en', // fallback language
interpolation: {
escapeValue: false, // react already safes from xss
},
});
export default i18n;
Step 4: Create Translation Files
Create JSON files for each language in the locales
directory. For example, en/translation.json
might look like this:
{
"greeting": "Hello, {{name}}!",
"buttons": {
"submit": "Submit",
"cancel": "Cancel"
},
"errors": {
"required": "This field is required"
}
}
Step 5: Use Translations in Components
Now you can use the translations in your React components:
import React from 'react';
import { useTranslation } from 'react-i18next';
function MyComponent() {
const { t } = useTranslation();
return (
<div>
<h1>{t('greeting', { name: 'John' })}</h1>
<button>{t('buttons.submit')}</button>
<button>{t('buttons.cancel')}</button>
</div>
);
}
Step 6: Implement Language Switching
Add a language switcher component to allow users to change the language:
import React from 'react';
import { useTranslation } from 'react-i18next';
function LanguageSwitcher() {
const { i18n } = useTranslation();
const changeLanguage = (lng) => {
i18n.changeLanguage(lng);
};
return (
<div>
<button onClick={() => changeLanguage('en')}>English</button>
<button onClick={() => changeLanguage('es')}>Español</button>
</div>
);
}
Step 7: Handle Pluralization and Complex Translations
i18next supports pluralization and other complex translation scenarios. Here’s an example of pluralization:
// In your translation file
{
"items": "{{count}} item",
"items_plural": "{{count}} items"
}
// In your React component
import React from 'react';
import { useTranslation } from 'react-i18next';
function ItemList({ count }) {
const { t } = useTranslation();
return (
<div>
{t('items', { count })}
</div>
);
}
Step 8: Implement RTL Support
For RTL language support, you can use CSS-in-JS solutions or CSS variables. Here’s an example using styled-components:
import styled from 'styled-components';
const Container = styled.div`
direction: ${props => props.isRTL ? 'rtl' : 'ltr'};
text-align: ${props => props.isRTL ? 'right' : 'left'};
`;
function App() {
const { i18n } = useTranslation();
const isRTL = i18n.dir() === 'rtl';
return (
<Container isRTL={isRTL}>
{/* Your app content */}
</Container>
);
}
Challenges in Software Localization
While localization offers numerous benefits, it also comes with its set of challenges:
1. Maintaining Consistency
Ensuring consistency across all localized versions of your software can be challenging, especially when dealing with multiple translators or teams.
2. Handling Context-Dependent Translations
Some words or phrases may have different meanings depending on the context, which can be difficult to convey to translators.
3. Managing Text Expansion
Translated text often takes up more space than the original, which can cause layout issues if not properly accounted for in the design.
4. Keeping Translations Updated
As your software evolves, keeping all language versions up-to-date can be time-consuming and resource-intensive.
5. Cultural Sensitivity
Ensuring that your software is culturally appropriate for all target markets requires in-depth knowledge of various cultures and customs.
Future Trends in Software Localization
As technology continues to evolve, so does the field of software localization. Here are some trends to watch:
1. AI-Powered Translation
Machine learning and AI are improving rapidly, potentially offering more accurate and context-aware automated translations in the future.
2. Continuous Localization
Integration of localization processes into continuous integration/continuous deployment (CI/CD) pipelines for more frequent and automated updates to localized versions.
3. Augmented Reality (AR) and Virtual Reality (VR) Localization
As AR and VR applications become more prevalent, new challenges and opportunities in localizing immersive experiences will emerge.
4. Voice User Interface (VUI) Localization
With the rise of voice-controlled devices and applications, localizing voice interfaces will become increasingly important.
Conclusion
Software localization is a crucial aspect of modern software development that can significantly impact your application’s global success. By understanding the key aspects of localization, following best practices, and implementing a robust localization strategy, you can create software that resonates with users across different cultures and languages.
Remember that localization is an ongoing process. As your software evolves and expands into new markets, continually refine your localization efforts to ensure the best possible user experience for all your users, regardless of their language or cultural background.
By mastering software localization, you’re not just translating your code; you’re opening doors to new markets, enhancing user experiences, and truly making your software speak the language of your global audience.