Software Internationalization (i18n) and Localization (l10n): A Comprehensive Guide for Developers
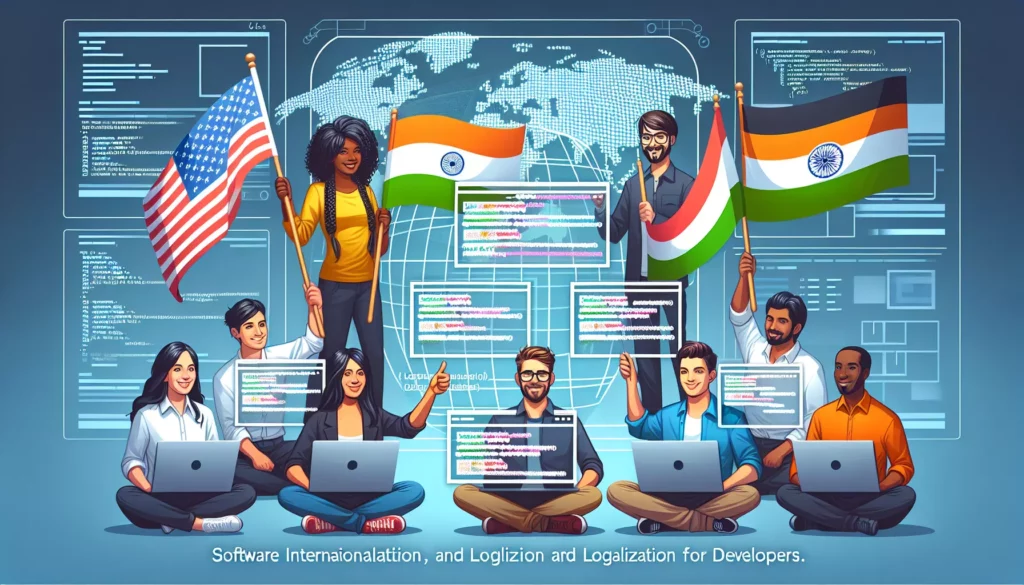
In today’s globalized world, creating software that can reach a diverse, international audience is more important than ever. This is where software internationalization (i18n) and localization (l10n) come into play. These processes are crucial for adapting software to different languages, cultures, and regions, ensuring that your application can be used and understood by people around the world. In this comprehensive guide, we’ll dive deep into the world of i18n and l10n, exploring their importance, key concepts, best practices, and how to implement them effectively in your software development projects.
Table of Contents
- Understanding Internationalization (i18n) and Localization (l10n)
- The Importance of i18n and l10n
- Key Concepts in i18n and l10n
- Best Practices for i18n and l10n
- Implementing i18n and l10n in Your Software
- Testing Internationalized and Localized Software
- Tools and Frameworks for i18n and l10n
- Common Challenges and How to Overcome Them
- Case Studies: Successful i18n and l10n Implementations
- Future Trends in i18n and l10n
- Conclusion
1. Understanding Internationalization (i18n) and Localization (l10n)
Before we dive into the details, let’s clarify what internationalization and localization mean and how they differ:
Internationalization (i18n)
Internationalization is the process of designing and developing software in a way that makes it capable of adapting to various languages and regions without requiring significant engineering changes. The term “i18n” comes from the first and last letters of “internationalization” with 18 letters in between.
Localization (l10n)
Localization is the process of adapting internationalized software for a specific region or language. This includes translating text, adjusting images and colors, using proper local formats for dates, currencies, and numbers, and addressing cultural considerations. The term “l10n” follows the same pattern as “i18n,” with 10 letters between “l” and “n” in “localization.”
In essence, internationalization lays the groundwork for localization. A well-internationalized application can be more easily localized for different markets.
2. The Importance of i18n and l10n
Implementing i18n and l10n in your software development process offers numerous benefits:
- Expanded Market Reach: By making your software accessible to users in different countries and languages, you can significantly increase your potential user base and market share.
- Improved User Experience: Users feel more comfortable and engaged when using software in their native language and with familiar cultural references.
- Legal Compliance: Some regions have laws requiring software to be available in local languages, especially for government or public service applications.
- Competitive Advantage: Offering localized versions of your software can give you an edge over competitors who haven’t invested in i18n and l10n.
- Easier Maintenance: A well-internationalized codebase is often easier to maintain and update across multiple languages and regions.
3. Key Concepts in i18n and l10n
To effectively implement i18n and l10n, it’s crucial to understand these key concepts:
Locale
A locale is a combination of language and region that defines a user’s language, country, and any special variant preferences. For example, “en-US” represents English as used in the United States, while “en-GB” represents English as used in Great Britain.
Character Encoding
Character encoding is the method used to represent characters in digital form. Unicode, particularly UTF-8, is widely used for its ability to represent characters from virtually all writing systems.
Resource Bundles
Resource bundles are files containing locale-specific objects, such as translated strings, images, and other resources. They allow you to separate localizable elements from your code.
Message Formatting
Message formatting involves creating templates for strings that can include variables, pluralization rules, and other dynamic elements. This allows for more flexible and context-aware translations.
Bidirectional Text Support
Some languages, like Arabic and Hebrew, are written from right to left. Supporting bidirectional text is crucial for proper display and interaction in these languages.
4. Best Practices for i18n and l10n
Follow these best practices to ensure successful implementation of i18n and l10n in your software:
1. Plan for i18n from the Start
Incorporate internationalization considerations into your software design from the beginning. It’s much easier and cost-effective to build with i18n in mind than to retrofit an existing application.
2. Separate Content from Code
Keep translatable content separate from your source code. Use resource files or databases to store strings and other locale-specific content.
3. Use Unicode
Adopt Unicode (preferably UTF-8) for character encoding to ensure support for all languages and scripts.
4. Avoid Hardcoding
Don’t hardcode any text, date formats, currency symbols, or other locale-specific elements in your code. Instead, use variables and load these from resource bundles.
5. Consider Text Expansion
Be aware that translated text may be longer or shorter than the original. Design your UI to accommodate text of varying lengths.
6. Use Proper Date, Time, and Number Formatting
Implement locale-aware formatting for dates, times, numbers, and currencies. Don’t assume everyone uses the same formats as your home country.
7. Support Right-to-Left Languages
Ensure your UI can adapt to right-to-left languages, including proper text alignment and mirroring of UI elements when necessary.
8. Implement Pluralization
Different languages have different rules for plurals. Use a pluralization system that can handle various language rules.
9. Provide Context for Translators
Give translators context about where and how strings are used in your application. This helps them provide more accurate and appropriate translations.
10. Test Thoroughly
Conduct thorough testing of your internationalized and localized software, including testing with native speakers of target languages.
5. Implementing i18n and l10n in Your Software
Now that we’ve covered the key concepts and best practices, let’s look at how to implement i18n and l10n in your software:
Step 1: Analyze Your Application
Begin by analyzing your application to identify all elements that need to be internationalized, such as:
- User interface text
- Images with text or cultural symbols
- Date and time displays
- Number and currency formats
- Sort orders
- Cultural considerations (colors, icons, etc.)
Step 2: Set Up Your Development Environment
Choose and set up appropriate i18n libraries or frameworks for your programming language and development environment. Some popular options include:
- Java: ResourceBundle and MessageFormat classes
- JavaScript: i18next, React-Intl, or Angular’s built-in i18n support
- Python: gettext
- Ruby: I18n gem
Step 3: Externalize Strings
Move all user-facing strings from your code into separate resource files. Here’s an example using a JSON format:
{
"en": {
"greeting": "Hello, {name}!",
"farewell": "Goodbye!"
},
"es": {
"greeting": "¡Hola, {name}!",
"farewell": "¡Adiós!"
}
}
Step 4: Implement Locale Detection and Switching
Develop a mechanism to detect the user’s preferred locale and allow them to switch between available languages. This often involves reading browser settings or providing a language selection menu.
Step 5: Use Locale-Aware Formatting
Implement locale-aware formatting for dates, numbers, and currencies. Many programming languages and frameworks provide built-in support for this. For example, in JavaScript:
const number = 1234567.89;
const date = new Date();
console.log(new Intl.NumberFormat('de-DE').format(number));
// Output: 1.234.567,89
console.log(new Intl.DateTimeFormat('de-DE').format(date));
// Output: 17.4.2023
Step 6: Handle Pluralization
Implement a system to handle pluralization rules for different languages. Many i18n libraries provide this functionality. Here’s an example using the i18next library in JavaScript:
i18next.init({
lng: 'en',
resources: {
en: {
translation: {
key_one: '{{count}} item',
key_other: '{{count}} items'
}
}
}
});
i18next.t('key', { count: 1 }); // output: "1 item"
i18next.t('key', { count: 2 }); // output: "2 items"
Step 7: Support Bidirectional Text
Ensure your application supports bidirectional text for languages like Arabic and Hebrew. This may involve using CSS properties like direction
and text-align
, and potentially mirroring your UI layout.
Step 8: Localize Other Resources
Don’t forget to localize non-text resources such as images, icons, and audio files if they contain language-specific or culturally-specific content.
6. Testing Internationalized and Localized Software
Thorough testing is crucial to ensure your internationalized and localized software works correctly across all supported locales. Here are some key areas to focus on:
Functional Testing
- Verify that all translated strings are displayed correctly
- Check that date, time, number, and currency formats are correct for each locale
- Ensure that sorting and searching work properly with locale-specific characters
- Test locale switching functionality
UI Testing
- Check for text overflow or truncation, especially with longer translations
- Verify that the UI layout adapts correctly for right-to-left languages
- Ensure that images and icons are appropriate for each locale
Linguistic Testing
- Have native speakers review the translations in context
- Check for grammatical errors, mistranslations, or culturally inappropriate content
Performance Testing
- Verify that switching between locales doesn’t cause significant performance issues
- Check that loading times are acceptable for all localized resources
7. Tools and Frameworks for i18n and l10n
There are numerous tools and frameworks available to assist with internationalization and localization. Here are some popular options:
Development Frameworks and Libraries
- React-Intl: Internationalization for React applications
- Angular i18n: Built-in internationalization support for Angular
- i18next: A powerful internationalization framework for JavaScript
- Ruby on Rails I18n: Internationalization support for Ruby on Rails
- Django Internationalization: i18n support for Python Django applications
Translation Management Systems
- Lokalise: Cloud-based localization and translation management platform
- Crowdin: Localization management platform for agile teams
- Transifex: Localization platform for digital content
Testing Tools
- Pseudolocalization: Technique to test internationalization readiness
- Localization Testing Tools: Various tools for automated localization testing
8. Common Challenges and How to Overcome Them
Implementing i18n and l10n can present several challenges. Here are some common issues and strategies to address them:
Challenge: Managing Large Numbers of Translations
Solution: Use a Translation Management System (TMS) to organize and manage your translations efficiently. These systems can help streamline the translation process and maintain consistency across your project.
Challenge: Ensuring Translation Quality
Solution: Provide context for translators, use style guides, and implement a review process involving native speakers. Consider using professional translation services for critical content.
Challenge: Handling Dynamic Content
Solution: Use parameterized messages and implement a robust message formatting system that can handle variables, pluralization, and gender-specific translations.
Challenge: Maintaining Performance with Multiple Locales
Solution: Implement lazy loading for locale data, only loading the resources needed for the current locale. Use efficient data structures for storing and retrieving localized content.
Challenge: Adapting UI for Different Languages
Solution: Design your UI with flexibility in mind. Use relative units (like em or %) instead of fixed sizes, and implement responsive design principles to accommodate varying text lengths.
9. Case Studies: Successful i18n and l10n Implementations
Let’s look at a few examples of companies that have successfully implemented internationalization and localization:
Case Study 1: Airbnb
Airbnb supports over 62 languages and numerous locales. They use a custom-built translation management system called Polyglot, which allows for continuous localization. Airbnb’s approach includes:
- Automating the translation workflow
- Using machine learning to improve translation quality
- Implementing a robust testing process for localized versions
Case Study 2: Netflix
Netflix has successfully expanded globally by investing heavily in localization. Their strategy includes:
- Creating localized content for specific markets
- Offering subtitles and dubbing in multiple languages
- Adapting their UI and recommendation algorithms for different cultures
Case Study 3: Duolingo
As a language learning platform, Duolingo places a high priority on i18n and l10n. Their approach includes:
- Using a crowdsourced translation model to rapidly expand to new languages
- Implementing a robust pluralization system to handle various language rules
- Adapting their content and exercises for cultural relevance in different regions
10. Future Trends in i18n and l10n
As technology evolves, so do the practices of internationalization and localization. Here are some trends to watch:
AI-Powered Translation
Machine learning and AI are improving rapidly, leading to more accurate and context-aware automated translations. While human oversight is still crucial, AI can significantly speed up the localization process.
Continuous Localization
With the rise of agile development practices, there’s a growing trend towards continuous localization, where translation happens in parallel with development rather than as a separate phase.
Voice User Interfaces
As voice-controlled devices become more prevalent, there’s an increasing need for localization of voice interfaces, including considerations for different accents and dialects.
Augmented and Virtual Reality
As AR and VR applications grow, they bring new challenges for i18n and l10n, such as localizing 3D environments and handling spatial text.
11. Conclusion
Internationalization and localization are crucial processes for creating software that can reach a global audience. By implementing i18n and l10n effectively, you can expand your market reach, improve user experience, and gain a competitive advantage in the global marketplace.
Remember that i18n and l10n are ongoing processes, not one-time tasks. As your software evolves, so should your internationalization and localization efforts. Stay updated with the latest tools and best practices, and always keep your users’ diverse needs in mind.
By following the principles, best practices, and implementation strategies outlined in this guide, you’ll be well-equipped to create software that truly speaks to users around the world, regardless of their language or cultural background.