Software Engineering Interview Prep: Your Path to Success
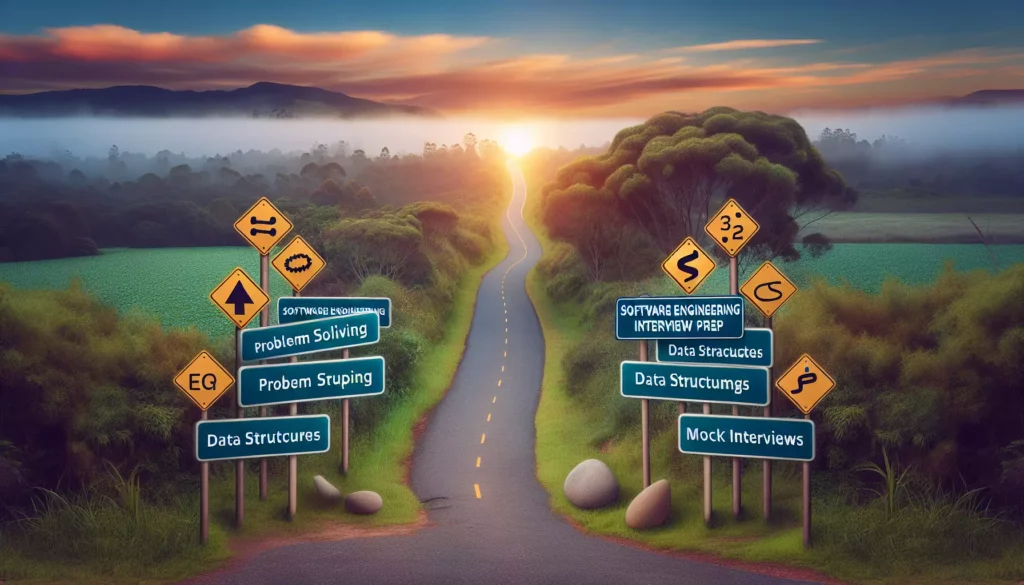
Are you aiming to land your dream job at a top tech company? The road to success in software engineering interviews can be challenging, but with the right preparation and resources, you can confidently tackle even the toughest technical interviews. In this comprehensive guide, we’ll explore the key components of a complete software engineering interview prep course and how it can propel your career to new heights.
Why Proper Interview Preparation Matters
The tech industry is highly competitive, with companies like Google, Amazon, and Facebook receiving thousands of applications for coveted software engineering positions. To stand out from the crowd, you need more than just a solid resume – you need to excel in the interview process.
A well-structured interview prep course can:
- Boost your confidence
- Sharpen your problem-solving skills
- Familiarize you with common interview questions and patterns
- Help you understand the expectations of top tech companies
- Improve your coding efficiency and algorithmic thinking
Key Components of a Complete Software Engineering Interview Prep Course
1. Data Structures and Algorithms
A solid understanding of data structures and algorithms is crucial for acing technical interviews. A comprehensive prep course should cover:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching Algorithms
- Dynamic Programming
- Greedy Algorithms
For example, let’s look at a simple implementation of a binary search algorithm in Python:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Element found at index: {result}")
Understanding and implementing such algorithms efficiently is key to succeeding in technical interviews.
2. System Design
For more senior positions, system design questions are increasingly common. A good prep course should cover:
- Scalability principles
- Load balancing
- Caching strategies
- Database design and optimization
- Microservices architecture
- API design
Here’s a basic example of how you might approach a system design question for a URL shortener:
class URLShortener:
def __init__(self):
self.url_map = {}
self.counter = 0
def shorten(self, long_url):
self.counter += 1
short_code = self._encode(self.counter)
self.url_map[short_code] = long_url
return f"https://short.url/{short_code}"
def redirect(self, short_code):
return self.url_map.get(short_code, "URL not found")
def _encode(self, number):
chars = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
base = len(chars)
result = []
while number > 0:
number, remainder = divmod(number, base)
result.append(chars[remainder])
return "".join(reversed(result))
# Example usage
shortener = URLShortener()
short_url = shortener.shorten("https://example.com/very/long/url")
print(f"Shortened URL: {short_url}")
original_url = shortener.redirect(short_url.split('/')[-1])
print(f"Original URL: {original_url}")
This example demonstrates a basic implementation of a URL shortener, which is a common system design question. A complete prep course would dive deeper into scaling this system to handle millions of requests.
3. Coding Challenges and Mock Interviews
Practice makes perfect. A comprehensive interview prep course should provide:
- A wide range of coding challenges, from easy to hard
- Timed coding exercises to simulate interview conditions
- Mock interviews with feedback
- Peer programming sessions
These practical exercises help you apply your knowledge and get comfortable with the pressure of real interviews.
4. Behavioral Interview Preparation
Technical skills are crucial, but so are soft skills. Your prep course should cover:
- Common behavioral questions
- The STAR method for answering questions
- How to showcase your achievements
- Techniques for handling difficult questions
For example, you might practice answering a question like, “Tell me about a time when you faced a significant technical challenge and how you overcame it.”
5. Language-Specific Preparation
While algorithmic thinking is language-agnostic, being proficient in specific programming languages is important. A good prep course should offer:
- Language-specific tips and tricks
- Best practices and idiomatic code examples
- Common pitfalls to avoid
For instance, if you’re preparing for a Python-focused role, you might learn about list comprehensions:
# Traditional approach
squares = []
for i in range(10):
squares.append(i**2)
# List comprehension
squares = [i**2 for i in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Understanding such language-specific features can make your code more elegant and efficient during interviews.
The AlgoCademy Advantage
While there are many resources available for interview preparation, AlgoCademy stands out by offering a comprehensive, interactive, and personalized learning experience. Here’s why AlgoCademy is the ideal choice for your software engineering interview prep:
1. AI-Powered Learning
AlgoCademy leverages cutting-edge AI technology to provide:
- Personalized learning paths based on your current skill level and goals
- Intelligent problem recommendations that adapt to your progress
- Real-time feedback on your code, helping you improve instantly
2. Comprehensive Curriculum
The AlgoCademy curriculum covers all aspects of interview preparation, including:
- In-depth lessons on data structures and algorithms
- System design workshops
- Behavioral interview training
- Language-specific modules for popular programming languages
3. Interactive Coding Environment
Practice makes perfect, and AlgoCademy provides the perfect environment for honing your skills:
- Browser-based IDE for instant coding practice
- Vast library of coding challenges, from easy to FAANG-level difficulty
- Timed coding exercises to simulate real interview conditions
4. Mock Interviews and Peer Programming
Gain confidence and experience with:
- AI-powered mock interviews that provide instant feedback
- Peer programming sessions to improve collaboration skills
- Community forums to discuss problems and solutions with fellow learners
5. Track Your Progress
Stay motivated and focused with:
- Detailed progress tracking and performance analytics
- Skill gap analysis to identify areas for improvement
- Achievement badges and certifications to showcase your skills
Success Stories
Don’t just take our word for it. Here are some success stories from AlgoCademy alumni:
“Thanks to AlgoCademy, I went from struggling with basic algorithms to confidently solving complex problems. I recently landed a job at Google, and I couldn’t have done it without the structured learning path and constant practice AlgoCademy provided.” – Sarah T., Software Engineer at Google
“The system design workshops on AlgoCademy were a game-changer for me. They helped me understand large-scale systems and ace my interviews at Amazon. The interactive nature of the platform made learning enjoyable and effective.” – Michael L., Senior Software Developer at Amazon
Preparing for Your Future
Investing in a complete software engineering interview prep course is not just about landing your next job – it’s about building a strong foundation for your entire career. The skills you develop through rigorous preparation will serve you well beyond the interview room, helping you become a more efficient, thoughtful, and valuable software engineer.
With AlgoCademy, you’re not just memorizing solutions or following a rigid curriculum. You’re developing a problem-solving mindset, learning to approach complex challenges systematically, and building the confidence to tackle any technical interview or real-world programming task.
Start Your Journey Today
Ready to take your software engineering career to the next level? AlgoCademy offers a free trial, allowing you to experience the power of AI-driven learning and comprehensive interview preparation. Don’t leave your dream job to chance – start your journey with AlgoCademy today and unlock your full potential as a software engineer.
Remember, the road to success in software engineering interviews may be challenging, but with the right tools and dedication, you can achieve your goals. AlgoCademy is here to guide you every step of the way, from your first coding challenge to your dream job offer.
Sign up now and join thousands of successful software engineers who have transformed their careers with AlgoCademy. Your future in tech starts here!
Conclusion
A complete software engineering interview prep course is an invaluable asset in today’s competitive tech landscape. By mastering data structures and algorithms, honing your system design skills, practicing coding challenges, and preparing for behavioral interviews, you’ll be well-equipped to ace even the most demanding technical interviews.
AlgoCademy offers all of this and more, with its AI-powered learning platform, comprehensive curriculum, and interactive coding environment. Whether you’re a fresh graduate looking to break into the industry or an experienced developer aiming for a position at a top tech company, AlgoCademy has the tools and resources you need to succeed.
Don’t leave your career to chance. Invest in yourself, start your preparation with AlgoCademy, and take the first step towards your dream job in software engineering. The tech world is waiting for you – are you ready to rise to the challenge?