Snap Inc. Technical Interview Prep: A Comprehensive Guide
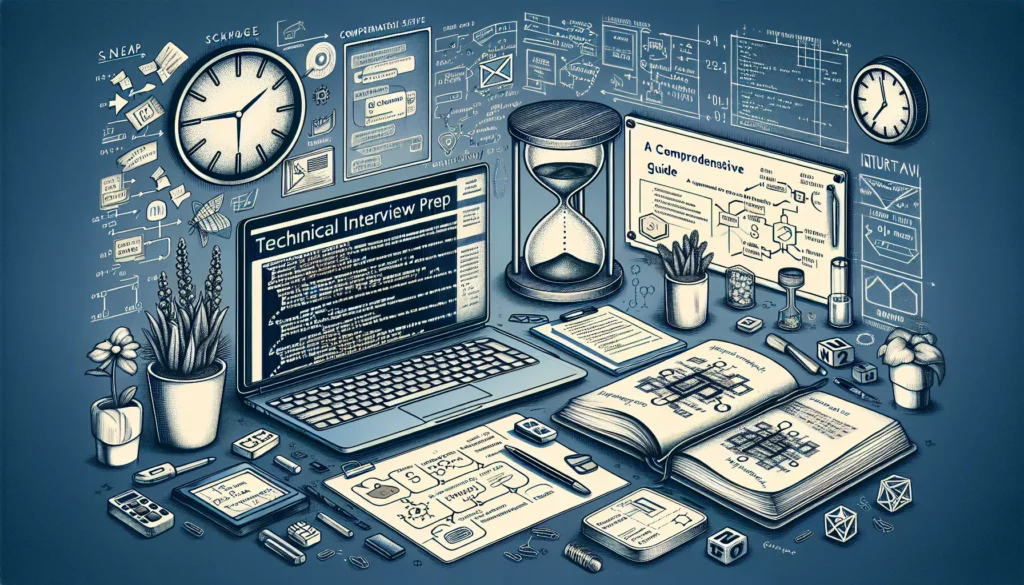
Preparing for a technical interview at Snap Inc. can be both exciting and challenging. As one of the leading social media and technology companies, Snap Inc. is known for its innovative products like Snapchat and Spectacles. To join their talented team of engineers, you’ll need to showcase your coding skills, problem-solving abilities, and technical knowledge. In this comprehensive guide, we’ll walk you through everything you need to know to ace your Snap Inc. technical interview.
Table of Contents
- Understanding Snap Inc. and Its Technology Stack
- The Snap Inc. Interview Process
- Core Concepts to Master
- Common Coding Questions and Challenges
- System Design and Architecture
- Behavioral Questions and Company Culture
- Preparation Tips and Resources
- What to Expect on Interview Day
- Post-Interview Follow-up
- Conclusion
1. Understanding Snap Inc. and Its Technology Stack
Before diving into interview preparation, it’s crucial to understand Snap Inc.’s technology stack and the kind of work you might be doing if you join the company. Snap Inc. uses a variety of technologies to power its products, including:
- Programming Languages: Python, Java, C++, Objective-C, Swift
- Mobile Development: Android SDK, iOS SDK
- Web Technologies: JavaScript, React, Node.js
- Databases: MySQL, Redis, Cassandra
- Cloud Services: Google Cloud Platform
- Machine Learning and Computer Vision
- Augmented Reality (AR) technologies
Familiarizing yourself with these technologies will give you a better understanding of the company’s technical needs and help you tailor your preparation accordingly.
2. The Snap Inc. Interview Process
The interview process at Snap Inc. typically consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to discuss your background and the role.
- Technical Phone Interview: A coding interview conducted over the phone or video call, usually lasting 45-60 minutes.
- On-site Interviews: If you pass the phone interviews, you’ll be invited for on-site interviews, which typically include:
- 2-3 coding interviews
- 1 system design interview
- 1-2 behavioral interviews
- Team Matching: If you perform well in the on-site interviews, you may have additional conversations to find the right team fit.
Understanding this process will help you prepare effectively for each stage of the interview.
3. Core Concepts to Master
To excel in your Snap Inc. technical interview, you should have a strong grasp of the following core computer science concepts:
Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
Algorithms
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Recursion
- Greedy Algorithms
- Graph Algorithms (Shortest Path, Minimum Spanning Tree)
Time and Space Complexity Analysis
Understanding Big O notation and being able to analyze the efficiency of your solutions is crucial.
Object-Oriented Programming (OOP) Principles
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
Operating Systems and Concurrency
- Processes and Threads
- Synchronization and Deadlocks
- Memory Management
Networking
- TCP/IP Protocol
- HTTP and RESTful APIs
- WebSockets
4. Common Coding Questions and Challenges
While the specific questions you’ll encounter may vary, here are some common types of coding challenges you should be prepared for:
String Manipulation
Example: Implement a function to reverse a string in-place.
def reverse_string(s):
chars = list(s)
left, right = 0, len(chars) - 1
while left < right:
chars[left], chars[right] = chars[right], chars[left]
left += 1
right -= 1
return ''.join(chars)
# Test the function
print(reverse_string("hello")) # Output: olleh
Array Problems
Example: Find the maximum subarray sum in an array of integers.
def max_subarray_sum(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Test the function
print(max_subarray_sum([-2, 1, -3, 4, -1, 2, 1, -5, 4])) # Output: 6
Tree and Graph Traversals
Example: Implement a depth-first search (DFS) for a binary tree.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def dfs(root):
if not root:
return
print(root.val) # Process the current node
dfs(root.left) # Recursively traverse left subtree
dfs(root.right) # Recursively traverse right subtree
# Create a sample binary tree
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
# Perform DFS
dfs(root) # Output: 1 2 4 5 3
Dynamic Programming
Example: Solve the classic “Climbing Stairs” problem.
def climb_stairs(n):
if n <= 2:
return n
dp = [0] * (n + 1)
dp[1] = 1
dp[2] = 2
for i in range(3, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Test the function
print(climb_stairs(5)) # Output: 8
Bit Manipulation
Example: Count the number of set bits in an integer.
def count_set_bits(n):
count = 0
while n:
count += n & 1
n >>= 1
return count
# Test the function
print(count_set_bits(7)) # Output: 3 (binary representation of 7 is 111)
Remember, it’s not just about solving the problem but also about explaining your thought process, discussing trade-offs, and optimizing your solution.
5. System Design and Architecture
For more senior positions, you’ll likely face system design questions. These assess your ability to design large-scale distributed systems. Some topics to focus on include:
- Scalability and Load Balancing
- Database Sharding and Replication
- Caching Strategies
- Microservices Architecture
- API Design
- Real-time Systems (relevant for Snapchat’s messaging features)
- Content Delivery Networks (CDNs)
- Data Storage Solutions (SQL vs. NoSQL)
A sample system design question might be: “Design a system like Snapchat’s Stories feature.” Here’s a high-level approach:
- Clarify Requirements: Understand the scale (number of users, story views), features (video/image upload, viewing, expiration), and performance expectations.
- High-Level Design: Sketch out the main components (user service, content storage, CDN, etc.).
- Data Model: Design the database schema for users, stories, and views.
- Detailed Component Design: Dive into each component, discussing technologies and trade-offs.
- Scalability: Discuss how the system can handle millions of users and story uploads/views.
- Performance Optimization: Consider caching strategies, CDN usage for content delivery, etc.
- Security and Privacy: Discuss measures to ensure user data protection and content privacy.
Remember to think out loud and explain your reasoning throughout the design process.
6. Behavioral Questions and Company Culture
Snap Inc. values creativity, innovation, and a user-focused mindset. Prepare for behavioral questions that assess these qualities:
- “Tell me about a time when you had to solve a complex technical problem.”
- “How do you stay updated with the latest technology trends?”
- “Describe a situation where you had to work with a difficult team member.”
- “How do you approach learning new technologies or programming languages?”
- “Can you share an example of how you’ve contributed to improving user experience in a previous project?”
Use the STAR method (Situation, Task, Action, Result) to structure your responses. Also, familiarize yourself with Snap Inc.’s values and try to align your answers with their culture.
7. Preparation Tips and Resources
To maximize your chances of success, consider the following preparation strategies:
- Practice Coding: Use platforms like LeetCode, HackerRank, or AlgoCademy to practice coding problems regularly.
- Mock Interviews: Conduct mock interviews with friends or use services like Pramp to simulate real interview conditions.
- Review Computer Science Fundamentals: Brush up on core CS concepts using resources like “Cracking the Coding Interview” by Gayle Laakmann McDowell.
- System Design Preparation: Study system design principles using resources like “Designing Data-Intensive Applications” by Martin Kleppmann or “System Design Interview” by Alex Xu.
- Stay Updated: Follow Snap Inc.’s engineering blog and tech news to stay informed about their latest technologies and challenges.
- Language Proficiency: Ensure you’re comfortable coding in at least one programming language (preferably one used by Snap Inc.).
- Time Management: Practice solving problems within time constraints to improve your speed and efficiency.
8. What to Expect on Interview Day
On the day of your on-site interview at Snap Inc., be prepared for:
- A full day of interviews (usually 4-6 hours)
- Coding on a whiteboard or computer (ask your recruiter about the format)
- Lunch with a team member (this is often part of the interview process)
- Questions about your background and experience
- A tour of the office (if interviews are conducted on-site)
Tips for interview day:
- Arrive early and well-rested
- Bring a copy of your resume and a notebook
- Dress comfortably but professionally (Snap Inc. typically has a casual dress code)
- Stay hydrated and take breaks when offered
- Ask questions about the team, projects, and company culture
9. Post-Interview Follow-up
After your interview:
- Send a thank-you email to your interviewers or recruiter within 24 hours.
- Reflect on the interview experience and note any areas for improvement.
- Be patient as the hiring team makes their decision.
- If you don’t hear back within the timeframe provided, politely follow up with your recruiter.
10. Conclusion
Preparing for a technical interview at Snap Inc. requires dedication, practice, and a well-rounded understanding of computer science concepts and current technologies. By focusing on core algorithms and data structures, honing your problem-solving skills, and understanding Snap Inc.’s unique challenges and culture, you’ll be well-equipped to showcase your abilities and potentially land a role at this innovative company.
Remember, the interview process is not just about proving your technical skills but also demonstrating your passion for technology, your ability to learn and adapt, and your potential to contribute to Snap Inc.’s mission of empowering people to express themselves, live in the moment, and learn about the world.
Good luck with your Snap Inc. interview preparation! With the right approach and mindset, you’ll be well on your way to joining the team behind some of the most exciting innovations in social media and technology.