Should Junior Devs Stop Focusing on Frameworks?
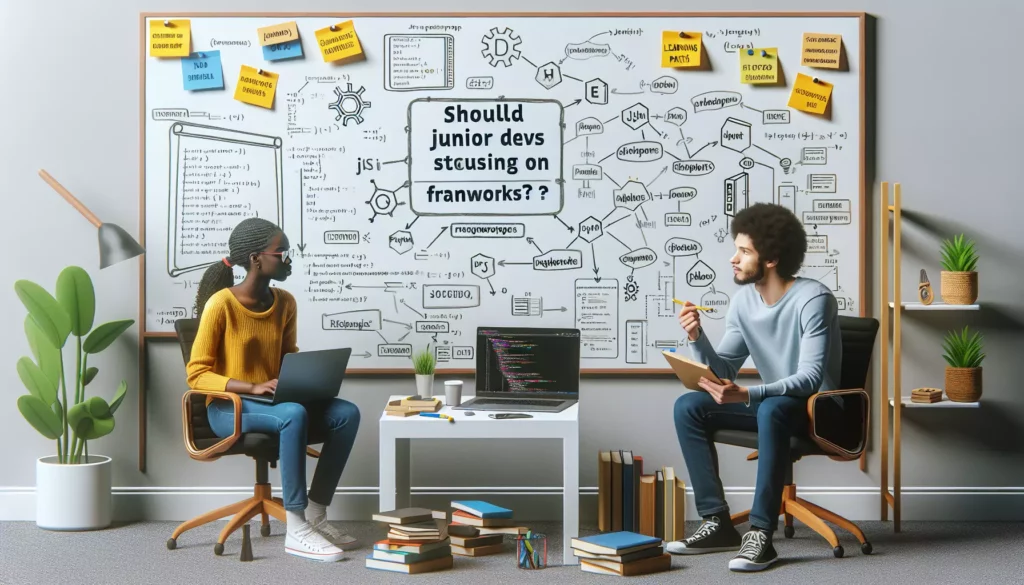
In the ever-evolving world of software development, junior developers often find themselves at a crossroads. On one side, there’s the allure of trendy frameworks promising rapid development and impressive results. On the other, there’s the foundational knowledge of core programming concepts and languages. The question arises: should junior devs stop focusing on frameworks and instead concentrate on building a solid foundation in programming fundamentals? Let’s dive deep into this topic and explore the pros and cons of each approach.
The Framework Frenzy
Frameworks have become increasingly popular in recent years, and for good reason. They offer a structured approach to building applications, often with pre-built components and tools that can significantly speed up development time. Popular frameworks like React, Angular, and Vue.js for frontend development, or Django and Ruby on Rails for backend work, have large communities and extensive documentation, making them attractive options for beginners.
Advantages of Learning Frameworks
- Rapid Development: Frameworks provide a set of tools and conventions that allow developers to build applications quickly.
- Industry Demand: Many job listings specifically request experience with certain frameworks.
- Community Support: Popular frameworks have large communities, making it easier to find help and resources.
- Best Practices: Frameworks often incorporate industry best practices, exposing developers to good coding habits.
Disadvantages of Focusing on Frameworks
- Dependency: Over-reliance on frameworks can lead to difficulty in understanding core programming concepts.
- Limited Flexibility: Frameworks can be constraining when trying to implement custom solutions.
- Rapid Changes: The framework landscape changes quickly, potentially making specific framework knowledge obsolete.
- Shallow Understanding: Focusing solely on frameworks may result in a surface-level understanding of programming principles.
The Case for Fundamentals
While frameworks offer immediate gratification and can help junior developers build impressive projects quickly, there’s a strong argument for focusing on programming fundamentals first. This approach involves mastering core concepts, data structures, algorithms, and design patterns that form the backbone of computer science and software development.
Advantages of Focusing on Fundamentals
- Deep Understanding: Learning fundamentals provides a comprehensive understanding of how programming languages and systems work.
- Adaptability: A strong foundation makes it easier to learn new languages, frameworks, and technologies.
- Problem-Solving Skills: Fundamentals enhance analytical thinking and problem-solving abilities.
- Long-term Career Growth: Solid foundational knowledge is valuable throughout a developer’s career, regardless of technological changes.
Disadvantages of Focusing Solely on Fundamentals
- Slower Initial Progress: It may take longer to build complex applications without the help of frameworks.
- Less Immediate Job Readiness: Some entry-level positions may require specific framework knowledge.
- Potential Demotivation: The abstract nature of some fundamental concepts can be discouraging for beginners seeking tangible results.
Finding the Right Balance
The reality is that both approaches have their merits, and the ideal path for junior developers likely lies somewhere in between. Here’s a suggested approach that combines the best of both worlds:
- Start with Core Languages: Begin by mastering a core language like JavaScript, Python, or Java. Understand the syntax, data types, control structures, and object-oriented programming concepts.
- Build Simple Projects: Create small projects using vanilla (framework-free) code to reinforce your understanding of the language and basic web technologies (HTML, CSS, etc.).
- Study Data Structures and Algorithms: Dedicate time to learning fundamental data structures (arrays, linked lists, trees, graphs) and algorithms (sorting, searching, dynamic programming). Platforms like AlgoCademy can be invaluable for this step.
- Explore Design Patterns: Familiarize yourself with common design patterns and architectural concepts. This knowledge will serve you well regardless of the framework you use.
- Introduce Frameworks: Once you have a solid foundation, start exploring frameworks. You’ll find that your fundamental knowledge makes learning frameworks much easier and more meaningful.
- Build More Complex Projects: Use frameworks to build larger, more complex projects. This will help you understand the benefits and limitations of frameworks in real-world scenarios.
- Continuous Learning: Keep up with both fundamental computer science concepts and new framework developments. The tech world is always evolving, and a balanced approach to learning will serve you well.
The Role of Problem-Solving and Algorithmic Thinking
Regardless of whether you’re using a framework or writing vanilla code, problem-solving skills and algorithmic thinking are crucial for any developer. These skills form the core of what it means to be a programmer and are often what separate great developers from good ones.
Platforms like AlgoCademy focus on developing these critical skills through interactive coding challenges and tutorials. By working through algorithmic problems, you’ll enhance your ability to break down complex issues, devise efficient solutions, and write clean, optimized code. These skills are transferable across all programming paradigms and are highly valued by employers.
Example: Solving a Common Coding Challenge
Let’s look at a simple example of how focusing on fundamentals can improve your problem-solving skills. Consider the classic “FizzBuzz” problem:
Write a program that prints the numbers from 1 to 100. But for multiples of three print “Fizz” instead of the number and for the multiples of five print “Buzz”. For numbers which are multiples of both three and five print “FizzBuzz”.
A junior developer focused solely on frameworks might struggle with this problem or immediately reach for a framework-specific solution. However, a developer with a strong grasp of fundamentals might approach it like this:
function fizzBuzz() {
for (let i = 1; i <= 100; i++) {
let output = '';
if (i % 3 === 0) output += 'Fizz';
if (i % 5 === 0) output += 'Buzz';
console.log(output || i);
}
}
fizzBuzz();
This solution demonstrates an understanding of loops, conditionals, and modular arithmetic – all fundamental programming concepts. The ability to solve such problems efficiently is valuable regardless of the framework or technology stack you’re working with.
The Importance of Understanding What’s “Under the Hood”
One of the main arguments for focusing on fundamentals before diving into frameworks is the importance of understanding what’s happening “under the hood”. Frameworks often abstract away many complexities, which can be both a blessing and a curse.
When you understand the underlying principles, you’re better equipped to:
- Debug issues more effectively
- Optimize performance
- Make informed decisions about when and how to use framework features
- Contribute to open-source projects or create your own libraries
For example, consider the concept of state management in frontend frameworks. React’s useState hook or Vue’s reactive data seem almost magical at first. But when you understand the principles of reactivity and how JavaScript’s event loop works, these features become much clearer. This deeper understanding allows you to use these tools more effectively and troubleshoot issues when they arise.
Example: Understanding Asynchronous Programming
Asynchronous programming is a fundamental concept in JavaScript that’s crucial for web development. Many frameworks provide abstractions for handling asynchronous operations, but understanding the basics is invaluable. Here’s a simple example using vanilla JavaScript:
console.log('Start');
setTimeout(() => {
console.log('Timeout callback');
}, 0);
Promise.resolve().then(() => {
console.log('Promise resolved');
});
console.log('End');
The output of this code might surprise developers who aren’t familiar with JavaScript’s event loop and the difference between the task queue and the microtask queue:
Start
End
Promise resolved
Timeout callback
Understanding why this happens requires knowledge of how JavaScript handles asynchronous operations, which is a fundamental concept not tied to any specific framework.
The Long-Term Career Perspective
When considering whether to focus on frameworks or fundamentals, it’s crucial to think about long-term career prospects. The tech industry moves quickly, and what’s popular today may not be in demand five years from now.
Frameworks come and go, but fundamental programming principles remain relatively constant. A developer with a strong foundation in computer science concepts, data structures, algorithms, and software design patterns will be well-equipped to adapt to new technologies as they emerge.
Moreover, as you progress in your career, you may find that higher-level positions require a deeper understanding of computer science principles. Senior developers, software architects, and technical leads often need to make decisions that go beyond the scope of any single framework.
Career Progression and Learning Path
Here’s a potential learning path that balances fundamentals and practical skills:
- Master a Core Language: Choose a language like JavaScript, Python, or Java and become proficient in it.
- Learn Data Structures and Algorithms: Use resources like AlgoCademy to study and practice implementing common data structures and algorithms.
- Understand Web Fundamentals: Learn how the web works, including HTTP, APIs, and basic frontend technologies (HTML, CSS).
- Explore Software Design Patterns: Study common design patterns and architectural principles.
- Pick a Framework: Choose a popular framework in your preferred area (frontend, backend, or full-stack) and build projects with it.
- Continuous Learning: Keep up with new developments, but always relate them back to fundamental principles.
By following this path, you’ll develop a well-rounded skill set that makes you valuable in the short term and sets you up for long-term career success.
The Role of Coding Challenges and Technical Interviews
Another important consideration in the frameworks vs. fundamentals debate is the role of coding challenges and technical interviews, particularly for positions at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google).
These companies, and many others following their lead, often focus their interview processes on fundamental computer science concepts, algorithmic problem-solving, and system design. They rarely ask framework-specific questions, understanding that a developer with strong foundational skills can quickly learn any required framework.
Platforms like AlgoCademy are designed to help developers prepare for these types of interviews. They focus on:
- Algorithmic thinking
- Efficient problem-solving
- Optimization techniques
- Time and space complexity analysis
By honing these skills, you’re not only preparing for technical interviews but also building a strong foundation that will serve you throughout your career, regardless of the specific technologies you work with.
Example: A Typical Coding Interview Question
Let’s look at a common type of question you might encounter in a coding interview:
Given an array of integers, find two numbers such that they add up to a specific target number.
A solution to this problem requires understanding of data structures (arrays, hash maps) and algorithmic thinking. Here’s an efficient solution in JavaScript:
function twoSum(nums, target) {
const map = new Map();
for (let i = 0; i < nums.length; i++) {
const complement = target - nums[i];
if (map.has(complement)) {
return [map.get(complement), i];
}
map.set(nums[i], i);
}
return [];
}
console.log(twoSum([2, 7, 11, 15], 9)); // Output: [0, 1]
This solution demonstrates knowledge of hash maps for efficient lookups, a fundamental concept that’s valuable across all areas of programming.
Conclusion: A Balanced Approach is Key
So, should junior devs stop focusing on frameworks? The answer isn’t a simple yes or no. While frameworks are valuable tools that can boost productivity and are often in demand in the job market, they shouldn’t be the sole focus of a junior developer’s learning journey.
A balanced approach that prioritizes fundamental programming concepts, problem-solving skills, and algorithmic thinking, while also including practical experience with frameworks, is likely the best path forward. This approach will create well-rounded developers who are not only job-ready in the short term but also set up for long-term career success.
Remember:
- Strong fundamentals make learning new frameworks and technologies easier.
- Problem-solving skills and algorithmic thinking are valuable across all areas of software development.
- Understanding what’s “under the hood” leads to more efficient and effective use of frameworks.
- A focus on fundamentals prepares you for technical interviews and career advancement.
Platforms like AlgoCademy can be invaluable in this journey, providing structured learning paths that focus on core computer science concepts while offering practical, hands-on coding experience. By combining this type of foundational learning with practical project work, possibly involving frameworks, junior developers can build a robust skill set that will serve them well throughout their careers.
In the end, the goal should be to become a versatile, adaptable developer who can tackle complex problems regardless of the specific tools or frameworks at hand. By balancing fundamentals with practical skills, junior developers can set themselves up for success in the dynamic and ever-changing world of software development.