Senior Engineer Technical Interview Prep: A Comprehensive Guide
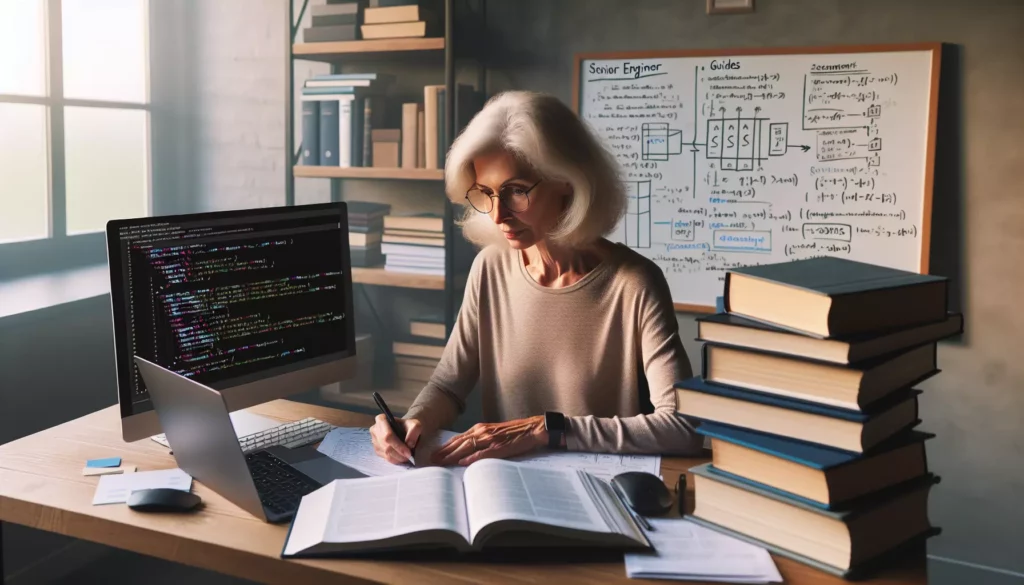
As you progress in your software engineering career, the technical interviews for senior engineer positions become increasingly challenging and complex. These interviews are designed to assess not only your coding skills but also your ability to design systems, solve complex problems, and lead technical initiatives. In this comprehensive guide, we’ll explore the key areas you need to focus on to ace your senior engineer technical interview and take your career to the next level.
Table of Contents
- Understanding the Expectations for Senior Engineers
- Core Competencies to Master
- Advanced Coding Skills and Problem-Solving
- System Design and Architecture
- Leadership and Communication Skills
- Behavioral Questions and Scenarios
- Navigating the Interview Process
- Resources for Preparation
- Conducting Mock Interviews
- Conclusion
1. Understanding the Expectations for Senior Engineers
Before diving into the specifics of interview preparation, it’s crucial to understand what companies are looking for in a senior engineer. Senior engineers are expected to:
- Demonstrate deep technical expertise and breadth of knowledge
- Lead complex projects and mentor junior developers
- Make high-level architectural decisions
- Contribute to the overall technical strategy of the organization
- Communicate effectively with both technical and non-technical stakeholders
- Solve complex problems efficiently and innovatively
With these expectations in mind, let’s explore the key areas you need to focus on for your interview preparation.
2. Core Competencies to Master
To succeed in a senior engineer technical interview, you should have a strong grasp of the following core competencies:
Data Structures and Algorithms
While you may have covered these topics earlier in your career, it’s essential to revisit and deepen your understanding of:
- Arrays and strings
- Linked lists
- Trees and graphs
- Stacks and queues
- Hash tables
- Heaps
- Dynamic programming
- Sorting and searching algorithms
Object-Oriented Programming (OOP)
Ensure you have a solid understanding of OOP concepts such as:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- Design patterns
Database Systems
Be prepared to discuss:
- Relational vs. NoSQL databases
- Query optimization
- Indexing strategies
- ACID properties
- Transactions and concurrency control
Distributed Systems
Familiarize yourself with concepts like:
- CAP theorem
- Consistency models
- Load balancing
- Sharding
- Replication
3. Advanced Coding Skills and Problem-Solving
As a senior engineer, you’re expected to write clean, efficient, and maintainable code. Here are some areas to focus on:
Code Optimization
Be prepared to optimize your code for:
- Time complexity
- Space complexity
- Readability
- Scalability
Design Patterns
Familiarize yourself with common design patterns and their applications, such as:
- Singleton
- Factory
- Observer
- Strategy
- Decorator
Problem-Solving Approach
Develop a systematic approach to problem-solving:
- Understand the problem thoroughly
- Identify constraints and edge cases
- Develop multiple solutions
- Analyze trade-offs between different approaches
- Implement the chosen solution
- Test and refine the solution
Code Example: Implementing a Thread-Safe Singleton
Here’s an example of implementing a thread-safe singleton pattern in Java:
public class ThreadSafeSingleton {
private static volatile ThreadSafeSingleton instance;
private static final Object lock = new Object();
private ThreadSafeSingleton() {
// Private constructor to prevent instantiation
}
public static ThreadSafeSingleton getInstance() {
if (instance == null) {
synchronized (lock) {
if (instance == null) {
instance = new ThreadSafeSingleton();
}
}
}
return instance;
}
// Other methods...
}
This implementation uses double-checked locking to ensure thread safety while maintaining performance.
4. System Design and Architecture
System design questions are a crucial part of senior engineer interviews. You should be able to design scalable, reliable, and efficient systems. Key areas to focus on include:
Scalability
- Horizontal vs. vertical scaling
- Caching strategies
- Database sharding
- Load balancing techniques
Reliability
- Fault tolerance
- Redundancy
- Disaster recovery
- Monitoring and alerting
Performance
- Latency optimization
- Throughput improvements
- Asynchronous processing
- Content delivery networks (CDNs)
Security
- Authentication and authorization
- Encryption
- Rate limiting
- OWASP top 10 vulnerabilities
System Design Example: URL Shortener
Let’s consider a high-level design for a URL shortener service:
- API Gateway: Handles incoming requests and performs rate limiting.
- Application Servers: Process requests and generate short URLs.
- Database: Stores mappings between short and long URLs.
- Cache: Improves read performance for frequently accessed URLs.
- Load Balancer: Distributes traffic across multiple application servers.
+-------------+
| API Gateway |
+-------------+
|
+-------------+
|Load Balancer|
+-------------+
/ \
+-----------+ +-----------+
| App Server| | App Server|
+-----------+ +-----------+
| |
+-----v-----+ +-----v-----+
| Cache | | Database |
+-----------+ +-----------+
This design addresses scalability through horizontal scaling of application servers, reliability through load balancing, and performance through caching.
5. Leadership and Communication Skills
As a senior engineer, you’ll be expected to lead projects and mentor junior developers. Prepare to discuss your experience in:
- Project management and planning
- Mentoring and coaching junior developers
- Collaborating with cross-functional teams
- Making technical decisions and justifying them
- Resolving conflicts within the team
Practice articulating complex technical concepts in simple terms, as you may need to explain your ideas to both technical and non-technical stakeholders.
6. Behavioral Questions and Scenarios
Expect behavioral questions that assess your problem-solving skills, leadership abilities, and cultural fit. Some common themes include:
- Handling disagreements with team members or managers
- Dealing with tight deadlines or changing requirements
- Making difficult technical decisions
- Overcoming challenges in previous projects
- Adapting to new technologies or methodologies
Use the STAR method (Situation, Task, Action, Result) to structure your responses effectively.
Example Behavioral Question and Response
Question: “Tell me about a time when you had to make a difficult technical decision that impacted the entire team.”
Response using STAR method:
- Situation: In my previous role, our team was developing a new e-commerce platform and needed to choose between two competing database technologies for our product catalog.
- Task: As the senior engineer, I was responsible for evaluating the options and making a recommendation that would best suit our long-term needs.
- Action: I conducted a thorough analysis of both technologies, considering factors such as scalability, query performance, and ease of maintenance. I also organized a series of meetings with team members to gather input and concerns. After compiling all the information, I presented my findings and recommendation to both the engineering team and management.
- Result: My recommendation was accepted, and we successfully implemented the chosen database technology. This decision led to a 30% improvement in query performance and significantly reduced maintenance overhead in the long run.
7. Navigating the Interview Process
The interview process for senior engineer positions typically involves multiple rounds:
- Initial Screening: Usually a phone or video call with a recruiter or hiring manager.
- Technical Phone Screen: A coding interview or technical discussion with an engineer.
- On-site Interviews: Multiple rounds of interviews, including:
- Coding interviews
- System design discussions
- Behavioral interviews
- Leadership and cultural fit assessments
- Final Interview: Often with a senior leader or executive.
Tips for success:
- Practice whiteboarding and explaining your thought process clearly
- Ask clarifying questions before diving into solutions
- Be prepared to discuss trade-offs in your design decisions
- Show enthusiasm for the company and role
- Have thoughtful questions prepared for your interviewers
8. Resources for Preparation
To help you prepare for your senior engineer technical interview, consider using the following resources:
Books
- “Designing Data-Intensive Applications” by Martin Kleppmann
- “System Design Interview” by Alex Xu
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Clean Code” by Robert C. Martin
Online Platforms
- LeetCode for coding practice
- HackerRank for algorithm challenges
- System Design Primer (GitHub repository)
- AlgoCademy for comprehensive coding education and interview prep
Courses
- MIT OpenCourseWare: Advanced Data Structures
- Coursera: Software Design and Architecture Specialization
- Udacity: Design of Computer Programs
9. Conducting Mock Interviews
One of the most effective ways to prepare for your senior engineer technical interview is to conduct mock interviews. Here are some strategies:
- Partner with a colleague or friend in the industry for mutual practice
- Use online platforms that offer mock interview services with experienced engineers
- Record yourself solving problems and explaining your thought process
- Join local tech meetups or online communities that organize mock interview sessions
During mock interviews, focus on:
- Clearly communicating your thought process
- Managing time effectively
- Handling pressure and unexpected questions
- Receiving and incorporating feedback
10. Conclusion
Preparing for a senior engineer technical interview requires a comprehensive approach that goes beyond just coding skills. By focusing on advanced technical concepts, system design, leadership abilities, and effective communication, you’ll be well-equipped to tackle even the most challenging interviews.
Remember that the interview process is not just about showcasing your skills, but also about demonstrating your potential as a senior leader in the organization. Show enthusiasm for solving complex problems, a willingness to learn and adapt, and a passion for mentoring others.
With thorough preparation and practice, you’ll be ready to confidently navigate your senior engineer technical interview and take the next step in your career. Good luck!