Securing Your Code: Basics of Cybersecurity
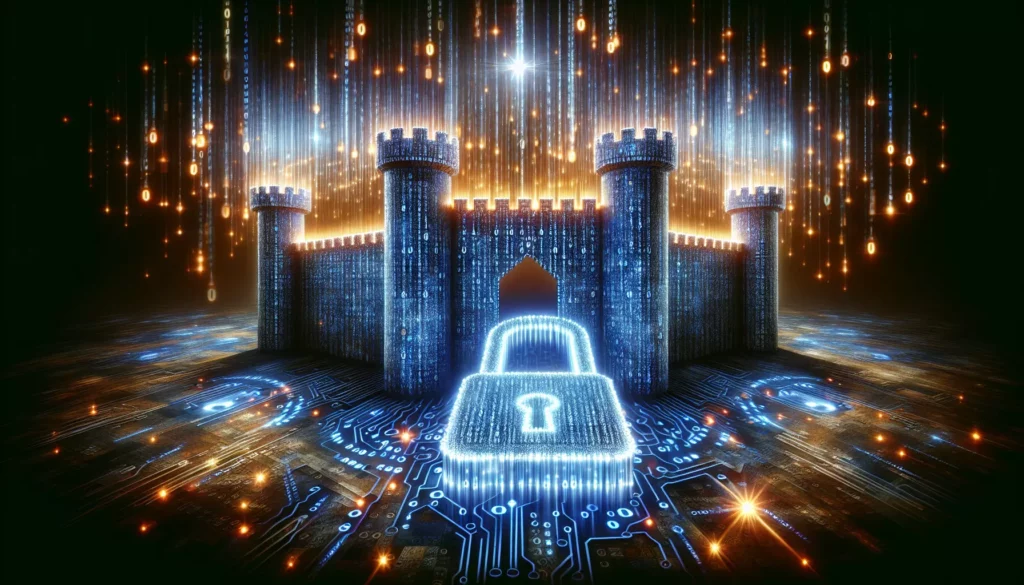
In today’s digital landscape, where data breaches and cyber attacks are becoming increasingly common, understanding the basics of cybersecurity is crucial for every programmer. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, incorporating security practices into your code is essential. This article will explore the fundamentals of cybersecurity and provide practical tips to help you write more secure code.
Why Cybersecurity Matters in Coding
Before diving into the specifics, it’s important to understand why cybersecurity is so critical in the world of programming. Here are a few key reasons:
- Protecting sensitive data: Your code may handle user information, financial data, or other confidential details that need to be safeguarded.
- Maintaining system integrity: Secure code helps prevent unauthorized access and modifications to your software.
- Building trust: Users and clients are more likely to trust and use applications that prioritize security.
- Compliance: Many industries have strict regulations regarding data protection and security practices.
- Career advancement: Knowledge of cybersecurity principles can make you a more valuable asset to potential employers, especially when applying to major tech companies.
Common Security Vulnerabilities
To write secure code, you need to be aware of common vulnerabilities that attackers might exploit. Here are some of the most prevalent security issues:
1. Injection Attacks
Injection attacks occur when an attacker inserts malicious code into a program, typically through user input. SQL injection is a common example, where an attacker manipulates SQL queries to gain unauthorized access to a database.
Example of vulnerable code:
username = input("Enter username: ")
query = "SELECT * FROM users WHERE username = '" + username + "'"
# Execute the query (UNSAFE!)
To prevent injection attacks, always use parameterized queries or prepared statements:
username = input("Enter username: ")
query = "SELECT * FROM users WHERE username = ?"
# Execute the query with username as a parameter (SAFE)
2. Cross-Site Scripting (XSS)
XSS attacks involve injecting malicious scripts into web pages viewed by other users. This can lead to stolen cookies, session hijacking, or other malicious activities.
Example of vulnerable code:
<!-- HTML -->
<input type="text" id="userInput">
<button onclick="displayInput()">Submit</button>
<div id="output"></div>
// JavaScript
function displayInput() {
var input = document.getElementById("userInput").value;
document.getElementById("output").innerHTML = input; // UNSAFE!
}
To prevent XSS attacks, always sanitize and encode user input before displaying it:
function displayInput() {
var input = document.getElementById("userInput").value;
var sanitizedInput = DOMPurify.sanitize(input); // Use a library like DOMPurify
document.getElementById("output").textContent = sanitizedInput; // SAFE
}
3. Broken Authentication
Broken authentication vulnerabilities allow attackers to bypass or compromise authentication mechanisms. This can lead to unauthorized access to user accounts and sensitive information.
Example of vulnerable code:
def login(username, password):
user = db.query(f"SELECT * FROM users WHERE username='{username}' AND password='{password}'")
if user:
return True
return False
To improve authentication security:
- Use strong, salted password hashing algorithms (e.g., bcrypt, Argon2)
- Implement multi-factor authentication
- Use secure session management
- Enforce strong password policies
import bcrypt
def login(username, password):
user = db.query("SELECT * FROM users WHERE username = ?", (username,))
if user and bcrypt.checkpw(password.encode('utf-8'), user.password_hash):
return True
return False
4. Insecure Direct Object References (IDOR)
IDOR vulnerabilities occur when an application exposes a reference to an internal implementation object, such as a file, directory, or database key, without proper access control checks.
Example of vulnerable code:
@app.route('/user/<int:user_id>/profile')
def user_profile(user_id):
user = User.query.get(user_id)
return render_template('profile.html', user=user) # UNSAFE!
To prevent IDOR vulnerabilities, implement proper access controls:
@app.route('/user/<int:user_id>/profile')
@login_required
def user_profile(user_id):
if current_user.id != user_id and not current_user.is_admin:
abort(403) # Forbidden
user = User.query.get(user_id)
return render_template('profile.html', user=user) # SAFE
5. Security Misconfiguration
Security misconfiguration vulnerabilities arise from insecure default configurations, incomplete or ad hoc configurations, open cloud storage, misconfigured HTTP headers, and verbose error messages containing sensitive information.
To prevent security misconfigurations:
- Use secure default configurations
- Remove unnecessary features, components, and documentation
- Review and update configurations regularly
- Implement security headers (e.g., Content Security Policy, X-Frame-Options)
- Use proper error handling to avoid leaking sensitive information
Best Practices for Secure Coding
Now that we’ve covered some common vulnerabilities, let’s explore best practices for writing secure code:
1. Input Validation and Sanitization
Always validate and sanitize user input to ensure it meets expected formats and doesn’t contain malicious content. Use whitelisting approaches when possible, and implement strict input validation on both client and server sides.
def validate_username(username):
# Use a regular expression to check for valid characters and length
return re.match(r'^[a-zA-Z0-9_]{3,20}$', username) is not None
username = input("Enter username: ")
if validate_username(username):
# Process the username
else:
print("Invalid username format")
2. Principle of Least Privilege
Apply the principle of least privilege by granting only the minimum necessary permissions to users, processes, and applications. This limits the potential damage if a compromise occurs.
// Instead of granting full admin access
grant_admin_access(user)
// Grant specific permissions as needed
grant_permission(user, "read_reports")
grant_permission(user, "edit_profile")
3. Use Secure Communication Protocols
Always use secure communication protocols (e.g., HTTPS, SSH) when transmitting sensitive data. Avoid using deprecated or insecure protocols.
import requests
# Use HTTPS for secure communication
response = requests.get("https://api.example.com/data")
# Avoid using unencrypted HTTP
# response = requests.get("http://api.example.com/data") # UNSAFE!
4. Implement Proper Authentication and Session Management
Use strong authentication mechanisms, secure session management, and implement features like password complexity requirements, account lockouts, and multi-factor authentication.
from flask import Flask, session
from flask_bcrypt import Bcrypt
import os
app = Flask(__name__)
app.secret_key = os.urandom(24)
bcrypt = Bcrypt(app)
@app.route('/login', methods=['POST'])
def login():
username = request.form['username']
password = request.form['password']
user = User.query.filter_by(username=username).first()
if user and bcrypt.check_password_hash(user.password, password):
session['user_id'] = user.id
session.permanent = True # Use permanent session
app.permanent_session_lifetime = timedelta(minutes=30) # Set session timeout
return redirect(url_for('dashboard'))
else:
return "Invalid credentials", 401
5. Keep Dependencies Updated
Regularly update your dependencies and libraries to ensure you have the latest security patches. Use tools like npm audit (for Node.js) or safety (for Python) to check for known vulnerabilities in your dependencies.
# For Python projects, use pip to update dependencies
pip install --upgrade <package_name>
# For Node.js projects, use npm to update dependencies
npm update
# Run security audits
npm audit # For Node.js
safety check # For Python
6. Implement Proper Error Handling
Implement proper error handling to prevent sensitive information from being exposed in error messages. Use generic error messages for users and log detailed error information securely for debugging purposes.
try:
# Perform some operation
result = perform_operation()
except Exception as e:
# Log the detailed error for debugging
logging.error(f"An error occurred: {str(e)}")
# Return a generic error message to the user
return "An error occurred. Please try again later.", 500
7. Use Secure Coding Practices for Specific Languages
Each programming language has its own set of security best practices. Familiarize yourself with language-specific security guidelines and follow them consistently.
Python-specific security practices:
- Use `secrets` module for cryptographically strong random numbers instead of `random` module
- Avoid using `eval()` or `exec()` with untrusted input
- Use `subprocess.run()` with `shell=False` when executing system commands
JavaScript-specific security practices:
- Use `const` and `let` instead of `var` to prevent variable hoisting
- Avoid using `eval()` or `new Function()` with untrusted input
- Use `Object.freeze()` to create immutable objects
8. Implement Security Headers
Implement security headers in your web applications to provide an additional layer of protection against various attacks.
from flask import Flask, Response
app = Flask(__name__)
@app.after_request
def add_security_headers(response):
response.headers['X-Frame-Options'] = 'SAMEORIGIN'
response.headers['X-XSS-Protection'] = '1; mode=block'
response.headers['X-Content-Type-Options'] = 'nosniff'
response.headers['Strict-Transport-Security'] = 'max-age=31536000; includeSubDomains'
response.headers['Content-Security-Policy'] = "default-src 'self'"
return response
9. Use Encryption for Sensitive Data
Always encrypt sensitive data, both in transit and at rest. Use strong, industry-standard encryption algorithms and key management practices.
from cryptography.fernet import Fernet
def encrypt_data(data):
key = Fernet.generate_key()
f = Fernet(key)
encrypted_data = f.encrypt(data.encode())
return key, encrypted_data
def decrypt_data(key, encrypted_data):
f = Fernet(key)
decrypted_data = f.decrypt(encrypted_data).decode()
return decrypted_data
# Usage
sensitive_data = "This is sensitive information"
key, encrypted = encrypt_data(sensitive_data)
decrypted = decrypt_data(key, encrypted)
print(f"Decrypted data: {decrypted}")
10. Implement Logging and Monitoring
Implement comprehensive logging and monitoring to detect and respond to security incidents quickly. Log security-relevant events and regularly review logs for suspicious activities.
import logging
from flask import Flask, request
app = Flask(__name__)
logging.basicConfig(filename='app.log', level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s')
@app.route('/login', methods=['POST'])
def login():
username = request.form['username']
# Perform login logic here
logging.info(f"Login attempt for user: {username}")
# Rest of the login code
Tools for Improving Code Security
There are numerous tools available to help you improve the security of your code. Here are some popular options:
1. Static Application Security Testing (SAST) Tools
- Bandit: A tool designed to find common security issues in Python code.
- ESLint: A pluggable linting utility for JavaScript that can include security-focused rules.
- SonarQube: An open-source platform for continuous inspection of code quality and security.
2. Dynamic Application Security Testing (DAST) Tools
- OWASP ZAP (Zed Attack Proxy): An open-source web application security scanner.
- Burp Suite: A platform for performing security testing of web applications.
3. Dependency Scanning Tools
- npm audit: A built-in security feature for Node.js projects.
- Safety: A command-line tool that checks Python dependencies for known security vulnerabilities.
- Snyk: A developer-first solution that automates finding and fixing vulnerabilities in your dependencies.
4. Code Review Tools
- GitHub Security: Provides security alerts, automated security updates, and code scanning capabilities.
- GitLab Security Dashboard: Offers a centralized view of security vulnerabilities across projects.
Conclusion
Securing your code is an essential skill for any programmer, especially those aiming to work at major tech companies. By understanding common vulnerabilities, implementing best practices, and utilizing security tools, you can significantly improve the security of your applications.
Remember that security is an ongoing process, not a one-time task. Stay informed about the latest security threats and best practices, and continuously update your skills and knowledge in this area. As you prepare for technical interviews and advance in your coding journey, demonstrating a strong understanding of cybersecurity principles will set you apart and make you a valuable asset to any development team.
Practice implementing these security measures in your coding projects, and consider incorporating security-focused challenges into your algorithmic problem-solving exercises. By doing so, you’ll not only improve the security of your code but also enhance your overall programming skills and readiness for technical interviews at top tech companies.