SAML and OpenID: Implementing Secure Authentication in Modern Applications
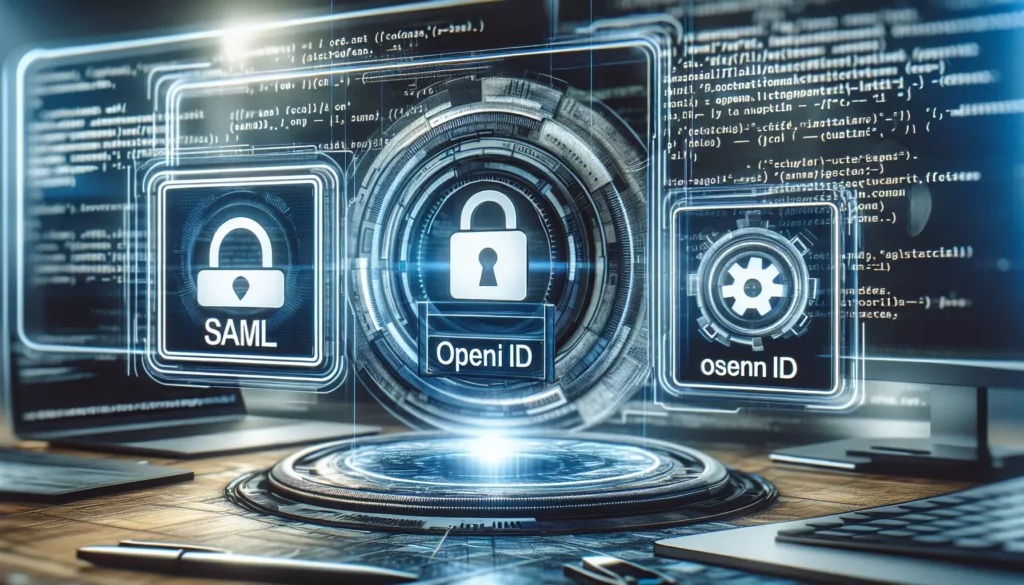
In today’s digital landscape, secure authentication is a critical component of any application. As developers, it’s essential to understand and implement robust authentication mechanisms to protect user data and ensure the integrity of our systems. Two popular protocols that have gained significant traction in recent years are SAML (Security Assertion Markup Language) and OpenID. In this comprehensive guide, we’ll dive deep into these authentication protocols, explore their differences, and learn how to implement them in your applications.
Table of Contents
- What is SAML?
- What is OpenID?
- SAML vs. OpenID: Key Differences
- Implementing SAML Authentication
- Implementing OpenID Authentication
- Best Practices for Secure Authentication
- The Future of Authentication
- Conclusion
What is SAML?
SAML, or Security Assertion Markup Language, is an XML-based open standard for exchanging authentication and authorization data between parties. It’s primarily used for Single Sign-On (SSO) solutions, allowing users to access multiple applications with a single set of credentials.
Key Components of SAML
- Identity Provider (IdP): The system that performs authentication and provides identity information to the service provider.
- Service Provider (SP): The application or service that the user wants to access.
- Assertions: XML documents containing authentication statements, attribute statements, and/or authorization decision statements about the user.
How SAML Works
- The user attempts to access a resource on the Service Provider.
- The SP generates a SAML request and redirects the user to the IdP.
- The IdP authenticates the user and generates a SAML response.
- The user is redirected back to the SP with the SAML response.
- The SP validates the response and grants access to the resource.
What is OpenID?
OpenID is an open standard and decentralized authentication protocol that allows users to be authenticated by cooperating sites (known as Relying Parties) using a third-party identity provider service. It eliminates the need for webmasters to provide their own ad hoc login systems and allows users to consolidate their digital identities.
Key Components of OpenID
- End User: The person who wants to access a service or application.
- Relying Party (RP): The application or service that wants to verify the identity of the End User.
- OpenID Provider (OP): The service that authenticates the End User and provides identity information to the Relying Party.
How OpenID Works
- The user attempts to access a resource on the Relying Party.
- The RP discovers the user’s OpenID Provider.
- The user is redirected to the OP for authentication.
- The OP authenticates the user and generates an authentication response.
- The user is redirected back to the RP with the authentication response.
- The RP validates the response and grants access to the resource.
SAML vs. OpenID: Key Differences
While both SAML and OpenID are authentication protocols, they have some key differences:
Feature | SAML | OpenID |
---|---|---|
Primary Use Case | Enterprise SSO | Web-based SSO for consumer applications |
Protocol | XML-based | HTTP-based |
Complexity | More complex to implement | Simpler to implement |
Flexibility | Highly flexible and customizable | Less flexible but easier to use |
Security | Generally considered more secure | Secure, but with fewer built-in security features |
Implementing SAML Authentication
Implementing SAML authentication can be complex, but it provides a high level of security and flexibility. Here’s a step-by-step guide to implementing SAML in your application:
1. Choose a SAML Library
Select a SAML library that suits your programming language and framework. Some popular options include:
- Python: python3-saml
- Java: OpenSAML
- Node.js: node-saml
- .NET: ITfoxtec.Identity.Saml2
2. Configure Your Service Provider
Set up your application as a Service Provider by configuring the following:
- Entity ID: A unique identifier for your SP
- Assertion Consumer Service (ACS) URL: The endpoint where SAML responses will be received
- SP Certificate: Used for signing SAML requests and decrypting responses
3. Set Up the Identity Provider
Configure your chosen IdP (e.g., Okta, Auth0, or Azure AD) with the following information:
- SP Entity ID
- ACS URL
- SP Certificate
4. Implement SAML Request Generation
When a user attempts to access a protected resource, generate a SAML authentication request:
<?xml version="1.0" encoding="UTF-8"?>
<samlp:AuthnRequest xmlns:samlp="urn:oasis:names:tc:SAML:2.0:protocol"
xmlns:saml="urn:oasis:names:tc:SAML:2.0:assertion"
ID="_RANDOM_ID"
Version="2.0"
IssueInstant="2023-05-01T12:00:00Z"
Destination="https://idp.example.com/SAML2/SSO/POST"
AssertionConsumerServiceURL="https://sp.example.com/acs"
ProtocolBinding="urn:oasis:names:tc:SAML:2.0:bindings:HTTP-POST">
<saml:Issuer>https://sp.example.com/metadata</saml:Issuer>
<samlp:NameIDPolicy Format="urn:oasis:names:tc:SAML:1.1:nameid-format:emailAddress"
AllowCreate="true"/>
</samlp:AuthnRequest>
5. Process SAML Response
After the user authenticates with the IdP, you’ll receive a SAML response. Process this response by:
- Verifying the signature
- Validating the assertion
- Extracting user information
Here’s an example of processing a SAML response using Python and the python3-saml library:
from onelogin.saml2.auth import OneLogin_Saml2_Auth
from onelogin.saml2.utils import OneLogin_Saml2_Utils
def process_saml_response(request):
req = prepare_flask_request(request)
auth = OneLogin_Saml2_Auth(req, custom_base_path=settings.SAML_FOLDER)
auth.process_response()
errors = auth.get_errors()
if not errors:
if auth.is_authenticated():
attributes = auth.get_attributes()
username = auth.get_nameid()
# Process user information and log them in
else:
# Authentication failed
else:
# Handle errors
Implementing OpenID Authentication
Implementing OpenID authentication is generally simpler than SAML. Here’s a step-by-step guide to implementing OpenID Connect (OIDC), the latest version of the OpenID protocol:
1. Choose an OpenID Library
Select an OpenID library that supports your programming language and framework. Some popular options include:
- Python: oic
- Java: Nimbus OAuth 2.0 SDK with OpenID Connect extensions
- Node.js: openid-client
- .NET: IdentityModel
2. Register Your Application
Register your application with an OpenID Provider (e.g., Google, Auth0, or Okta) to obtain the following:
- Client ID
- Client Secret
- Redirect URI
3. Configure Your Application
Set up your application with the OpenID Provider’s configuration, including:
- Authorization Endpoint
- Token Endpoint
- UserInfo Endpoint
- JSON Web Key Set (JWKS) URI
4. Implement Authentication Flow
The OpenID Connect authentication flow typically involves the following steps:
- Redirect the user to the Authorization Endpoint
- Receive the authorization code
- Exchange the code for tokens
- Validate the ID Token
- Retrieve user information from the UserInfo Endpoint
Here’s an example of implementing the OpenID Connect flow using Python and the oic library:
from oic.oic import Client
from oic.utils.authn.client import CLIENT_AUTHN_METHOD
client = Client(client_authn_method=CLIENT_AUTHN_METHOD)
client.provider_config(issuer="https://accounts.google.com")
client.client_id = "YOUR_CLIENT_ID"
client.client_secret = "YOUR_CLIENT_SECRET"
# Generate authorization URL
args = {
"client_id": client.client_id,
"response_type": "code",
"scope": ["openid", "profile", "email"],
"redirect_uri": "https://your-app.com/callback",
}
auth_url = client.construct_AuthorizationRequest(request_args=args).to_url()
# Redirect user to auth_url
# In the callback route
def callback():
code = request.args.get("code")
# Exchange code for tokens
token_response = client.do_access_token_request(
state=session["state"],
request_args={"code": code},
authn_method="client_secret_basic"
)
# Validate ID Token
id_token = token_response["id_token"]
claims = client.verify_id_token(id_token, ["nonce"])
# Retrieve user information
userinfo = client.do_user_info_request(token=token_response["access_token"])
# Process user information and log them in
Best Practices for Secure Authentication
Regardless of whether you choose SAML or OpenID, follow these best practices to ensure secure authentication:
- Use HTTPS: Always use HTTPS to encrypt communication between the client, service provider, and identity provider.
- Implement proper session management: Use secure session cookies and implement proper logout procedures.
- Validate all inputs: Sanitize and validate all user inputs to prevent injection attacks.
- Keep libraries up to date: Regularly update your authentication libraries to patch security vulnerabilities.
- Implement multi-factor authentication (MFA): Add an extra layer of security by requiring additional authentication factors.
- Use strong encryption: Employ strong encryption algorithms for signing and encrypting tokens and assertions.
- Implement proper error handling: Avoid leaking sensitive information through error messages.
- Conduct regular security audits: Perform regular security assessments to identify and address potential vulnerabilities.
The Future of Authentication
As technology evolves, so do authentication methods. Here are some trends and technologies that are shaping the future of authentication:
1. Passwordless Authentication
Passwordless authentication methods, such as biometrics, hardware tokens, and magic links, are gaining popularity due to their improved security and user experience.
2. Decentralized Identity
Blockchain-based decentralized identity solutions aim to give users more control over their digital identities and reduce reliance on centralized identity providers.
3. Continuous Authentication
Continuous authentication systems monitor user behavior throughout a session, adjusting access levels based on risk assessments.
4. Artificial Intelligence and Machine Learning
AI and ML technologies are being used to detect anomalies and potential security threats in real-time, enhancing authentication systems.
5. Zero Trust Architecture
The Zero Trust security model, which assumes no trust and verifies every access request, is becoming increasingly important in modern authentication systems.
Conclusion
Implementing secure authentication is crucial for protecting user data and maintaining the integrity of your applications. Both SAML and OpenID offer robust solutions for authentication, each with its own strengths and use cases. By understanding these protocols and following best practices, you can create secure, user-friendly authentication systems that meet the needs of your applications and users.
As you continue to develop your coding skills and prepare for technical interviews, remember that authentication and security are critical aspects of modern software development. Familiarizing yourself with these concepts will not only make you a better developer but also increase your value to potential employers, especially when targeting positions at major tech companies.
Keep exploring, practicing, and staying up-to-date with the latest developments in authentication and security. With dedication and continuous learning, you’ll be well-equipped to tackle authentication challenges in your future projects and excel in your coding career.