Russian Doll Envelopes: A Deep Dive into Dynamic Programming
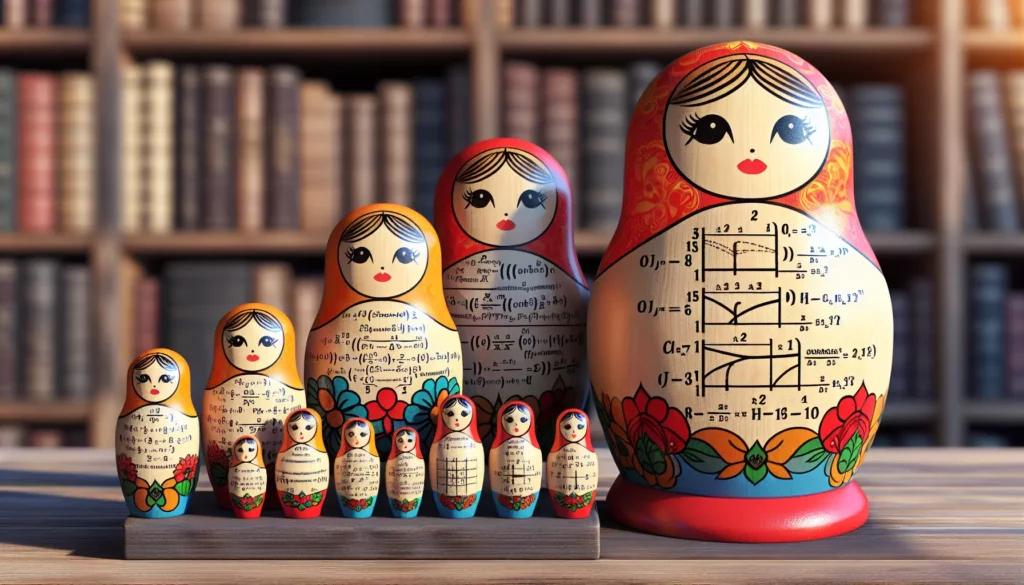
Welcome to another exciting exploration of algorithmic problem-solving! Today, we’re going to unpack a fascinating challenge known as the “Russian Doll Envelopes” problem. This problem is not only an excellent exercise in dynamic programming but also a great way to sharpen your skills for technical interviews at top tech companies. So, let’s dive in and see how we can solve this intriguing puzzle!
Understanding the Problem
Before we jump into the solution, let’s clearly define the problem we’re trying to solve:
You are given a 2D array
envelopes
whereenvelopes[i] = [wi, hi]
represents the width and height of an envelope. One envelope can fit into another if and only if both the width and height of one envelope are greater than the other envelope’s width and height. What is the maximum number of envelopes you can Russian doll (i.e., put one inside the other)?
This problem is a variation of the classic Longest Increasing Subsequence (LIS) problem, but with a twist – we’re dealing with two dimensions instead of one.
Approaching the Solution
To solve this problem, we’ll break it down into several steps:
- Sort the envelopes
- Apply the Longest Increasing Subsequence algorithm
- Optimize our solution
Let’s go through each of these steps in detail.
Step 1: Sorting the Envelopes
The first step in our solution is to sort the envelopes. But how should we sort them? We want to sort them in a way that allows us to reduce this 2D problem to a 1D problem. Here’s how we’ll do it:
- Sort the envelopes by width in ascending order
- If two envelopes have the same width, sort them by height in descending order
Why do we sort this way? By sorting the widths in ascending order, we ensure that we’re always considering envelopes that can potentially fit inside each other. The trick with sorting heights in descending order for envelopes of the same width is to avoid counting multiple envelopes of the same width.
Here’s how we can implement this sorting in Python:
def maxEnvelopes(envelopes):
# Sort by width (ascending) and then by height (descending)
envelopes.sort(key=lambda x: (x[0], -x[1]))
# Rest of the code...
Step 2: Applying the Longest Increasing Subsequence Algorithm
Now that we’ve sorted our envelopes, we can focus on finding the longest increasing subsequence based on the heights of the envelopes. This is because, after sorting, we know that the widths are already in increasing order.
The Longest Increasing Subsequence problem can be solved using dynamic programming. Here’s a basic implementation:
def maxEnvelopes(envelopes):
envelopes.sort(key=lambda x: (x[0], -x[1]))
n = len(envelopes)
dp = [1] * n
for i in range(1, n):
for j in range(i):
if envelopes[i][1] > envelopes[j][1]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp) if dp else 0
In this implementation:
- We initialize a
dp
array wheredp[i]
represents the length of the longest increasing subsequence ending at indexi
. - We iterate through the envelopes, comparing each envelope with all previous envelopes.
- If the current envelope’s height is greater than a previous envelope’s height, we update
dp[i]
to be the maximum of its current value anddp[j] + 1
. - Finally, we return the maximum value in the
dp
array, which represents the length of the longest increasing subsequence.
Step 3: Optimizing Our Solution
While the above solution works, it has a time complexity of O(n^2), which might not be efficient enough for large inputs. We can optimize this further using a binary search approach, reducing the time complexity to O(n log n).
Here’s the optimized solution:
from bisect import bisect_left
def maxEnvelopes(envelopes):
envelopes.sort(key=lambda x: (x[0], -x[1]))
dp = []
for _, h in envelopes:
i = bisect_left(dp, h)
if i == len(dp):
dp.append(h)
else:
dp[i] = h
return len(dp)
In this optimized version:
- We still sort the envelopes as before.
- We maintain a
dp
array wheredp[i]
is the smallest height of an envelope that ends an increasing subsequence of lengthi+1
. - For each envelope, we use binary search (
bisect_left
) to find the correct position to insert its height in thedp
array. - If the height is greater than all heights in
dp
, we append it, effectively increasing the length of the longest subsequence. - Otherwise, we update the value at the found position, potentially allowing for a longer subsequence in the future.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our optimized solution:
Time Complexity
- Sorting the envelopes takes O(n log n) time.
- The main loop runs n times, and each iteration performs a binary search, which takes O(log n) time.
- Therefore, the overall time complexity is O(n log n).
Space Complexity
- We use an additional array
dp
to store the heights. - In the worst case,
dp
could store all n heights. - Thus, the space complexity is O(n).
Common Pitfalls and Edge Cases
When solving the Russian Doll Envelopes problem, there are several pitfalls and edge cases to be aware of:
- Empty Input: Always check if the input array is empty and return 0 if it is.
- Single Envelope: If there’s only one envelope, the answer is always 1.
- Equal Dimensions: Remember that envelopes with equal dimensions cannot be nested inside each other.
- Sorting Order: The key to this problem is the sorting step. Make sure you sort by width ascending and height descending for equal widths.
- Overflow: For very large inputs, be cautious of integer overflow in languages with fixed-size integers.
Related Problems and Variations
The Russian Doll Envelopes problem is part of a family of algorithmic challenges. Here are some related problems that you might find interesting:
- Longest Increasing Subsequence (LIS): This is the 1D version of our problem and forms the basis of the solution.
- Box Stacking Problem: Similar to Russian Doll Envelopes, but with 3D boxes instead of 2D envelopes.
- Building Bridges: A problem where you need to connect points on two parallel lines without intersections, maximizing the number of connections.
- Maximum Height by Stacking Cuboids: A 3D version of the envelope problem where you can rotate the cuboids.
Real-world Applications
While nesting envelopes might seem like a purely academic exercise, the concepts behind this problem have several real-world applications:
- Package Optimization: In logistics and shipping, optimizing how items are packed can save space and reduce costs.
- Circuit Board Design: When designing circuit boards, components often need to be arranged in a way that larger components don’t overlap smaller ones.
- Document Layout: In desktop publishing or web design, arranging text boxes or images of different sizes can be modeled as a variation of this problem.
- Resource Allocation: In computer science, allocating resources (like memory or processing power) to tasks with varying requirements can be optimized using similar algorithms.
Interview Tips
If you encounter this problem or a similar one in a technical interview, here are some tips to help you succeed:
- Clarify the Problem: Make sure you understand all aspects of the problem. Ask questions about the input format, constraints, and expected output.
- Think Aloud: Share your thought process with the interviewer. They’re interested in how you approach problems, not just the final solution.
- Start Simple: Begin with a brute-force solution if you can’t immediately see the optimal approach. You can optimize it later.
- Optimize Step-by-Step: If you start with a less efficient solution, explain how you would optimize it. This shows your ability to iterate and improve your code.
- Test Your Solution: Before declaring you’re done, walk through your solution with a small example to catch any logical errors.
- Analyze Complexity: Be prepared to discuss the time and space complexity of your solution.
- Consider Edge Cases: Mention and handle edge cases in your solution, such as empty input or single-element input.
Conclusion
The Russian Doll Envelopes problem is a fantastic example of how seemingly complex problems can be solved elegantly using fundamental algorithmic concepts. By breaking down the problem, applying sorting techniques, and leveraging the Longest Increasing Subsequence algorithm, we’ve created an efficient solution to a challenging problem.
This problem teaches us valuable lessons about problem-solving in computer science:
- The importance of problem transformation (2D to 1D in this case)
- The power of sorting as a preprocessing step
- The application of dynamic programming and its optimization using binary search
- The significance of time and space complexity analysis in developing efficient algorithms
As you continue your journey in algorithmic problem-solving, remember that many complex problems can be approached by breaking them down into simpler, known problems. The skills you’ve developed solving this problem will serve you well in tackling a wide range of algorithmic challenges, both in interviews and in real-world software development.
Keep practicing, stay curious, and happy coding!