Rolling Hash: A Powerful Technique for String Manipulation in Programming
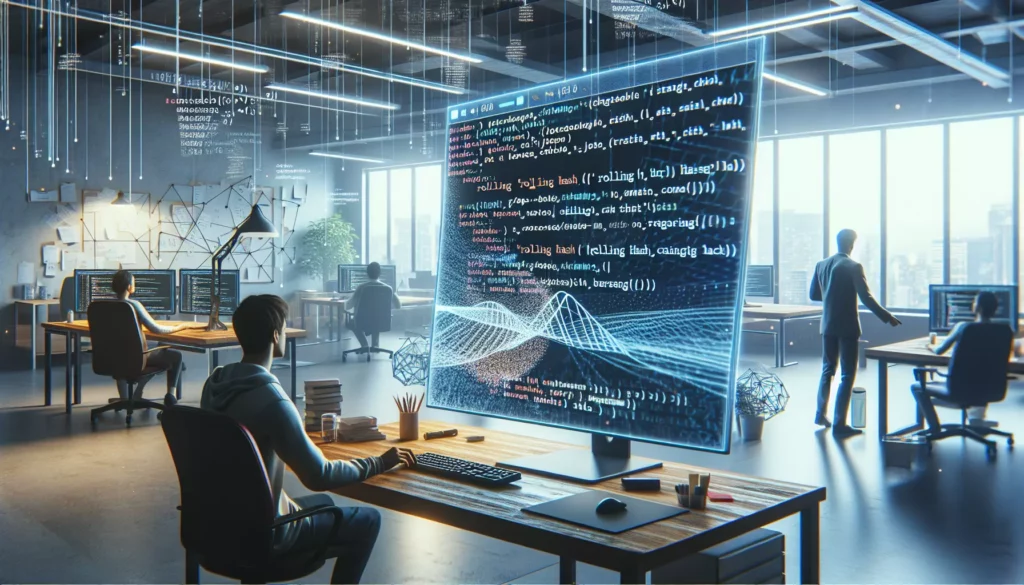
In the world of algorithmic programming and string manipulation, efficiency is key. As developers, we often encounter problems that require us to search for patterns within large texts, compare substrings, or perform various operations on strings. One powerful technique that can significantly optimize these operations is the Rolling Hash algorithm. In this comprehensive guide, we’ll dive deep into the concept of Rolling Hash, its implementation, and its applications in solving complex programming problems.
What is Rolling Hash?
Rolling Hash, also known as rolling checksum or sliding hash, is a technique used to compute a hash value over a fixed-size window of data that “rolls” through a larger dataset. It’s particularly useful when dealing with strings or sequences of data where we need to calculate hash values for multiple overlapping substrings efficiently.
The key advantage of Rolling Hash is its ability to compute the hash value of the next window in constant time, using the hash value of the previous window. This property makes it an excellent choice for various string algorithms, especially those involving pattern matching or substring comparisons.
How Does Rolling Hash Work?
The basic idea behind Rolling Hash is to use a hash function that allows for easy updates as the window slides through the data. Here’s a step-by-step breakdown of how it works:
- Choose a suitable hash function that can be easily updated.
- Calculate the initial hash value for the first window of data.
- As the window slides, remove the contribution of the outgoing element from the hash value.
- Add the contribution of the incoming element to the hash value.
- Repeat steps 3 and 4 for each slide of the window.
The magic of Rolling Hash lies in its ability to perform steps 3 and 4 in constant time, regardless of the window size.
Implementing Rolling Hash
Let’s implement a basic Rolling Hash function in Python to understand its mechanics better:
def rolling_hash(s, window_size):
if len(s) < window_size:
return None
base = 256 # Using ASCII values
mod = 1000000007 # A large prime number
# Calculate the initial hash value
hash_value = 0
for i in range(window_size):
hash_value = (hash_value * base + ord(s[i])) % mod
yield hash_value
# Calculate hash values for subsequent windows
for i in range(window_size, len(s)):
# Remove the contribution of the outgoing character
hash_value = (hash_value - ord(s[i - window_size]) * pow(base, window_size - 1, mod)) % mod
# Add the contribution of the incoming character
hash_value = (hash_value * base + ord(s[i])) % mod
yield hash_value
# Example usage
s = "abcdefghijklmnop"
window_size = 3
for hash_val in rolling_hash(s, window_size):
print(hash_val)
In this implementation, we use a simple polynomial hash function. The base (256) is chosen to represent ASCII characters, and we use modular arithmetic with a large prime number to prevent integer overflow and reduce collisions.
Applications of Rolling Hash
Rolling Hash finds applications in various algorithms and problem-solving scenarios. Let’s explore some of the most common use cases:
1. String Matching Algorithms
One of the most popular applications of Rolling Hash is in string matching algorithms, particularly in the Rabin-Karp algorithm. This algorithm uses Rolling Hash to efficiently search for occurrences of a pattern within a larger text.
Here’s a basic implementation of the Rabin-Karp algorithm using our Rolling Hash function:
def rabin_karp(text, pattern):
n, m = len(text), len(pattern)
if m > n:
return []
pattern_hash = sum(ord(pattern[i]) * 256**(m-1-i) for i in range(m)) % 1000000007
matches = []
for i, window_hash in enumerate(rolling_hash(text, m)):
if window_hash == pattern_hash and text[i:i+m] == pattern:
matches.append(i)
return matches
# Example usage
text = "ABABDABACDABABCABAB"
pattern = "ABABCABAB"
print(rabin_karp(text, pattern)) # Output: [10]
This algorithm has an average-case time complexity of O(n+m), where n is the length of the text and m is the length of the pattern, making it more efficient than naive string matching approaches in many scenarios.
2. Detecting Duplicate Substrings
Rolling Hash can be used to efficiently detect duplicate substrings within a larger string. This is particularly useful in data compression algorithms or when looking for repeated patterns in DNA sequences.
Here’s an example of how to use Rolling Hash to find all duplicate substrings of a given length:
from collections import defaultdict
def find_duplicates(s, length):
hash_to_index = defaultdict(list)
duplicates = []
for i, hash_val in enumerate(rolling_hash(s, length)):
hash_to_index[hash_val].append(i)
for hash_val, indices in hash_to_index.items():
if len(indices) > 1:
# Verify to avoid hash collisions
substring = s[indices[0]:indices[0]+length]
if all(s[i:i+length] == substring for i in indices):
duplicates.append((substring, indices))
return duplicates
# Example usage
s = "ABAACABAACABAAC"
length = 5
print(find_duplicates(s, length))
# Output: [('ABAAC', [0, 5, 10])]
This algorithm can detect duplicates in O(n) time complexity, where n is the length of the string.
3. Polynomial Hash for Strings
Rolling Hash can be used to create a unique hash value for strings, which is useful in various scenarios such as comparing substrings or creating hash tables for strings.
Here’s an implementation of a polynomial hash function using Rolling Hash:
def polynomial_hash(s):
base = 256
mod = 1000000007
hash_value = 0
for char in s:
hash_value = (hash_value * base + ord(char)) % mod
return hash_value
# Example usage
s1 = "hello"
s2 = "world"
print(polynomial_hash(s1))
print(polynomial_hash(s2))
This hash function can be used to quickly compare strings or substrings, which is particularly useful in algorithms that require frequent string comparisons.
Optimizing Rolling Hash
While the basic implementation of Rolling Hash is already efficient, there are several techniques we can use to further optimize its performance:
1. Precomputing Powers
In our Rolling Hash implementation, we frequently calculate powers of the base. We can precompute these values to save time:
def precompute_powers(base, max_power, mod):
powers = [1] * (max_power + 1)
for i in range(1, max_power + 1):
powers[i] = (powers[i-1] * base) % mod
return powers
# Usage in rolling_hash function
powers = precompute_powers(256, window_size, mod)
hash_value = (hash_value - ord(s[i - window_size]) * powers[window_size - 1]) % mod
2. Using Bit Operations
If our base is a power of 2 (e.g., 256), we can use bit operations for faster computation:
base = 256 # 2^8
mod = (1 << 61) - 1 # Mersenne prime
# In rolling_hash function
hash_value = ((hash_value << 8) | ord(s[i])) & mod
3. Double Hashing
To reduce the probability of hash collisions, we can use two different hash functions and combine their results:
def double_hash(s, window_size):
base1, mod1 = 256, 1000000007
base2, mod2 = 257, 1000000009
hash1 = hash2 = 0
for i in range(window_size):
hash1 = (hash1 * base1 + ord(s[i])) % mod1
hash2 = (hash2 * base2 + ord(s[i])) % mod2
yield (hash1, hash2)
for i in range(window_size, len(s)):
hash1 = (hash1 * base1 - ord(s[i - window_size]) * pow(base1, window_size, mod1) + ord(s[i])) % mod1
hash2 = (hash2 * base2 - ord(s[i - window_size]) * pow(base2, window_size, mod2) + ord(s[i])) % mod2
yield (hash1, hash2)
Common Pitfalls and How to Avoid Them
While Rolling Hash is a powerful technique, there are some common pitfalls to be aware of:
1. Hash Collisions
Hash collisions occur when two different inputs produce the same hash value. This can lead to false positives in string matching algorithms. To mitigate this:
- Use a large prime number as the modulus.
- Implement double hashing or use multiple hash functions.
- Always verify matches by comparing the actual substrings.
2. Integer Overflow
When working with large strings or windows, integer overflow can occur. To prevent this:
- Use modular arithmetic consistently throughout your calculations.
- Choose appropriate data types (e.g., long long in C++) to handle large numbers.
- Consider using a language or library that supports arbitrary-precision arithmetic.
3. Choosing Appropriate Base and Modulus
The choice of base and modulus can significantly impact the performance and collision rate of your Rolling Hash function. Consider the following when choosing these values:
- The base should be larger than the size of your alphabet (e.g., 256 for ASCII).
- The modulus should be a large prime number to reduce collisions.
- For bit manipulation optimizations, choose a base that is a power of 2 and a modulus of the form 2^k – 1.
Advanced Applications of Rolling Hash
Beyond the basic applications we’ve discussed, Rolling Hash has some fascinating advanced uses in computer science and software engineering:
1. Longest Common Substring
Rolling Hash can be used to efficiently solve the Longest Common Substring problem. By hashing all substrings of two strings and comparing the hashes, we can find the longest common substring in O(n*m) time, where n and m are the lengths of the strings.
2. Palindrome Detection
Rolling Hash can be used to detect palindromes in a string. By computing forward and backward hashes simultaneously, we can quickly identify palindromic substrings.
3. Data Deduplication
In data storage systems, Rolling Hash is used for data deduplication. By computing hashes of data chunks, duplicate data can be identified and eliminated, saving storage space.
4. File Synchronization
Rolling Hash is used in file synchronization algorithms like rsync. It allows for efficient identification of changed portions of files, minimizing the amount of data that needs to be transferred during synchronization.
Rolling Hash in Competitive Programming
For those interested in competitive programming or preparing for technical interviews, Rolling Hash is a valuable technique to master. It often appears in problems related to string manipulation, pattern matching, and substring operations. Here are some tips for using Rolling Hash effectively in competitive programming:
- Implement a reusable Rolling Hash class or function that you can quickly adapt to different problems.
- Practice problems that involve string matching, substring comparisons, and pattern finding.
- Be prepared to combine Rolling Hash with other algorithms and data structures for more complex problems.
- Understand the time and space complexity trade-offs when using Rolling Hash compared to other string algorithms.
Conclusion
Rolling Hash is a powerful and versatile technique that can significantly optimize string manipulation algorithms. Its ability to compute hash values for sliding windows in constant time makes it an invaluable tool for solving a wide range of problems efficiently.
As we’ve explored in this article, Rolling Hash finds applications in string matching, duplicate detection, and various other areas of computer science and software engineering. By understanding the principles behind Rolling Hash and practicing its implementation, you’ll be well-equipped to tackle complex string-related problems in your programming journey.
Remember that while Rolling Hash is a powerful technique, it’s not a silver bullet. Always consider the specific requirements of your problem and the potential trade-offs when deciding whether to use Rolling Hash or alternative approaches. With practice and experience, you’ll develop an intuition for when and how to best apply this fascinating algorithm.
As you continue to explore the world of algorithms and data structures, keep Rolling Hash in your toolkit. It’s a technique that demonstrates the elegance and efficiency that can be achieved in computer science when clever mathematical principles are applied to solve practical problems.