Robinhood Technical Interview Prep: A Comprehensive Guide
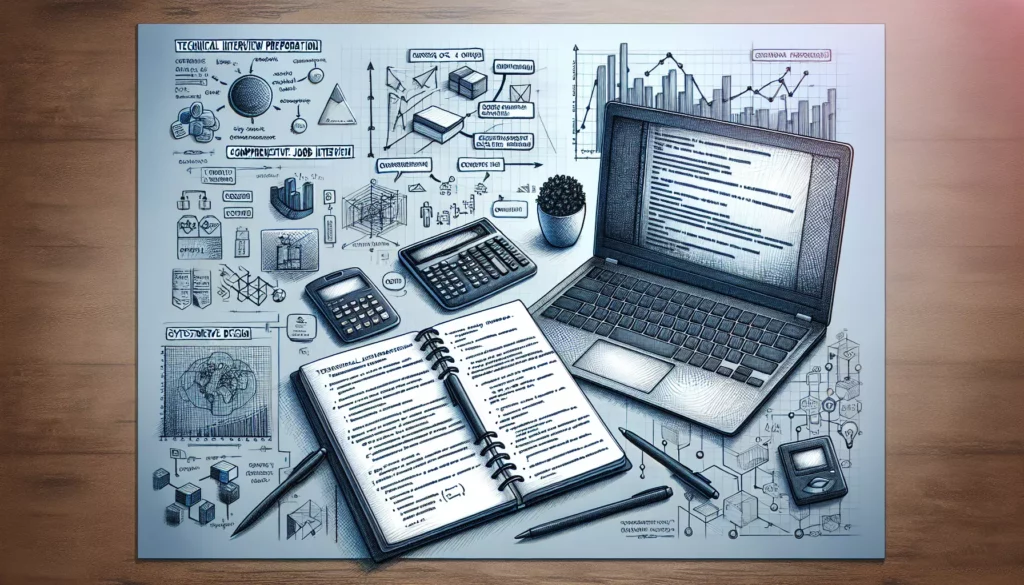
Are you gearing up for a technical interview at Robinhood? You’ve come to the right place! In this comprehensive guide, we’ll walk you through everything you need to know to ace your Robinhood technical interview. From understanding the interview process to mastering key coding concepts and tackling sample problems, we’ve got you covered.
Table of Contents
- Understanding Robinhood
- The Robinhood Interview Process
- Key Concepts to Master
- Coding Languages to Focus On
- Essential Data Structures
- Important Algorithms
- System Design Fundamentals
- Sample Problems and Solutions
- Tips and Tricks for Success
- Additional Resources
Understanding Robinhood
Before diving into the technical aspects of the interview, it’s crucial to understand Robinhood as a company. Robinhood is a financial services company that offers commission-free trading of stocks, exchange-traded funds (ETFs), options, and cryptocurrencies through a mobile app. The company’s mission is to democratize finance for all, making it accessible to everyone.
Key points about Robinhood:
- Founded in 2013 by Vladimir Tenev and Baiju Bhatt
- Pioneered commission-free trading in the US
- Focuses on user-friendly mobile and web applications
- Deals with high-frequency trading and real-time financial data
- Emphasizes security, scalability, and low-latency systems
Understanding these aspects of Robinhood will help you tailor your responses and demonstrate your alignment with the company’s goals during the interview process.
The Robinhood Interview Process
The technical interview process at Robinhood typically consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to discuss your background and interest in Robinhood.
- Technical Phone Interview: A 45-60 minute interview with an engineer, focusing on coding problems and algorithmic thinking.
- Take-home Assignment (optional): Some candidates may be asked to complete a coding project.
- On-site Interviews: A series of 4-5 interviews, including:
- Coding interviews
- System design interview
- Behavioral interview
Throughout this process, Robinhood assesses your technical skills, problem-solving abilities, communication, and cultural fit.
Key Concepts to Master
To excel in your Robinhood technical interview, you should be well-versed in the following key concepts:
- Data structures and algorithms
- Object-oriented programming
- Time and space complexity analysis
- Concurrency and multithreading
- Distributed systems
- Database design and SQL
- RESTful API design
- Version control (Git)
- Testing methodologies
- Basic understanding of financial markets and trading systems
Coding Languages to Focus On
While Robinhood doesn’t strictly require knowledge of specific programming languages, it’s beneficial to be proficient in one or more of the following:
- Python
- Java
- C++
- Go
- JavaScript (for frontend positions)
Python and Go are particularly popular at Robinhood, so having a strong grasp of these languages can be advantageous.
Essential Data Structures
Make sure you have a solid understanding of the following data structures:
- Arrays and dynamic arrays
- Linked lists (singly and doubly linked)
- Stacks and queues
- Hash tables
- Trees (binary trees, binary search trees, balanced trees)
- Heaps
- Graphs
- Tries
Be prepared to implement these data structures from scratch and explain their time and space complexities for various operations.
Important Algorithms
Familiarize yourself with the following algorithms and their applications:
- Sorting algorithms (QuickSort, MergeSort, HeapSort)
- Searching algorithms (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy algorithms
- Graph algorithms (Dijkstra’s, Bellman-Ford, Topological Sort)
- String manipulation algorithms
Practice implementing these algorithms and analyzing their time and space complexities.
System Design Fundamentals
For system design interviews, focus on the following areas:
- Scalability and load balancing
- Caching strategies
- Database sharding and replication
- Microservices architecture
- Message queues and event-driven systems
- Content delivery networks (CDNs)
- Consistency models in distributed systems
- Fault tolerance and disaster recovery
Be prepared to design systems that can handle high-frequency trading and real-time data processing, as these are crucial aspects of Robinhood’s infrastructure.
Sample Problems and Solutions
Here are a few sample problems that could come up in a Robinhood technical interview, along with their solutions:
1. Implement a Stock Price Tracker
Problem: Design a class StockTracker that keeps track of the current price of a stock and provides methods to update the price and calculate the maximum profit that could have been made by buying and selling the stock once.
class StockTracker:
def __init__(self):
self.prices = []
self.max_profit = 0
self.min_price = float('inf')
def update_price(self, price: float) -> None:
self.prices.append(price)
if price < self.min_price:
self.min_price = price
elif price - self.min_price > self.max_profit:
self.max_profit = price - self.min_price
def get_max_profit(self) -> float:
return self.max_profit
# Usage
tracker = StockTracker()
tracker.update_price(10)
tracker.update_price(7)
tracker.update_price(5)
tracker.update_price(8)
tracker.update_price(11)
tracker.update_price(9)
print(tracker.get_max_profit()) # Output: 6
This implementation uses O(1) time for each update_price operation and O(1) space overall.
2. Implement a Rate Limiter
Problem: Design a rate limiter class that allows a maximum of N requests per minute for each user.
from collections import deque
import time
class RateLimiter:
def __init__(self, limit: int, time_window: int):
self.limit = limit
self.time_window = time_window
self.requests = {}
def is_allowed(self, user_id: str) -> bool:
current_time = time.time()
if user_id not in self.requests:
self.requests[user_id] = deque()
user_requests = self.requests[user_id]
while user_requests and current_time - user_requests[0] >= self.time_window:
user_requests.popleft()
if len(user_requests) < self.limit:
user_requests.append(current_time)
return True
return False
# Usage
limiter = RateLimiter(5, 60) # 5 requests per minute
user = "user123"
for i in range(10):
if limiter.is_allowed(user):
print(f"Request {i+1} allowed")
else:
print(f"Request {i+1} denied")
time.sleep(10) # Sleep for 10 seconds between requests
This implementation uses a sliding window approach with O(1) time complexity for each is_allowed check and O(N) space complexity, where N is the number of users.
3. Design a Simple Order Book
Problem: Implement a basic order book that can add buy/sell orders and match them efficiently.
import heapq
class Order:
def __init__(self, price: float, quantity: int, is_buy: bool):
self.price = price
self.quantity = quantity
self.is_buy = is_buy
class OrderBook:
def __init__(self):
self.buy_orders = []
self.sell_orders = []
def add_order(self, order: Order):
if order.is_buy:
heapq.heappush(self.buy_orders, (-order.price, order))
else:
heapq.heappush(self.sell_orders, (order.price, order))
self.match_orders()
def match_orders(self):
while self.buy_orders and self.sell_orders:
best_buy = self.buy_orders[0][1]
best_sell = self.sell_orders[0][1]
if best_buy.price >= best_sell.price:
matched_quantity = min(best_buy.quantity, best_sell.quantity)
best_buy.quantity -= matched_quantity
best_sell.quantity -= matched_quantity
print(f"Matched: {matched_quantity} @ {best_sell.price}")
if best_buy.quantity == 0:
heapq.heappop(self.buy_orders)
if best_sell.quantity == 0:
heapq.heappop(self.sell_orders)
else:
break
# Usage
order_book = OrderBook()
order_book.add_order(Order(100, 10, True)) # Buy 10 @ $100
order_book.add_order(Order(99, 5, True)) # Buy 5 @ $99
order_book.add_order(Order(101, 7, False)) # Sell 7 @ $101
order_book.add_order(Order(98, 8, False)) # Sell 8 @ $98
This implementation uses heaps to efficiently maintain the best buy and sell orders, resulting in O(log N) time complexity for adding orders and O(M log N) for matching, where N is the number of orders and M is the number of matches.
Tips and Tricks for Success
- Practice, practice, practice: Solve coding problems regularly on platforms like LeetCode, HackerRank, or AlgoExpert.
- Mock interviews: Conduct mock interviews with friends or use services like Pramp to get comfortable with the interview format.
- Think out loud: Clearly communicate your thought process during the interview.
- Ask clarifying questions: Ensure you fully understand the problem before starting to code.
- Consider edge cases: Always think about and discuss potential edge cases in your solutions.
- Optimize your solutions: Start with a working solution, then look for ways to optimize it.
- Learn from your mistakes: If you make a mistake, acknowledge it and learn from it.
- Stay calm: Remember that interviewers are looking for problem-solving skills, not just perfect code.
- Research Robinhood: Familiarize yourself with Robinhood’s products, technologies, and recent news.
- Prepare questions: Have thoughtful questions ready to ask your interviewers about the company and the role.
Additional Resources
To further prepare for your Robinhood technical interview, consider the following resources:
- Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash
- “System Design Interview” by Alex Xu
- Online Courses:
- AlgoExpert’s SystemsExpert for system design preparation
- Coursera’s “Algorithms Specialization” by Stanford University
- MIT OpenCourseWare’s “Introduction to Algorithms”
- Coding Platforms:
- LeetCode
- HackerRank
- CodeSignal
- System Design Resources:
- Grokking the System Design Interview
- System Design Primer (GitHub repository)
- Robinhood Engineering Blog: Read about the technical challenges and solutions at Robinhood
Remember, preparation is key to success in technical interviews. By mastering the concepts and practicing regularly, you’ll be well-equipped to tackle the challenges of a Robinhood technical interview. Good luck!