Reverse Engineering Coding Problems: A Shortcut to Understanding Complex Challenges
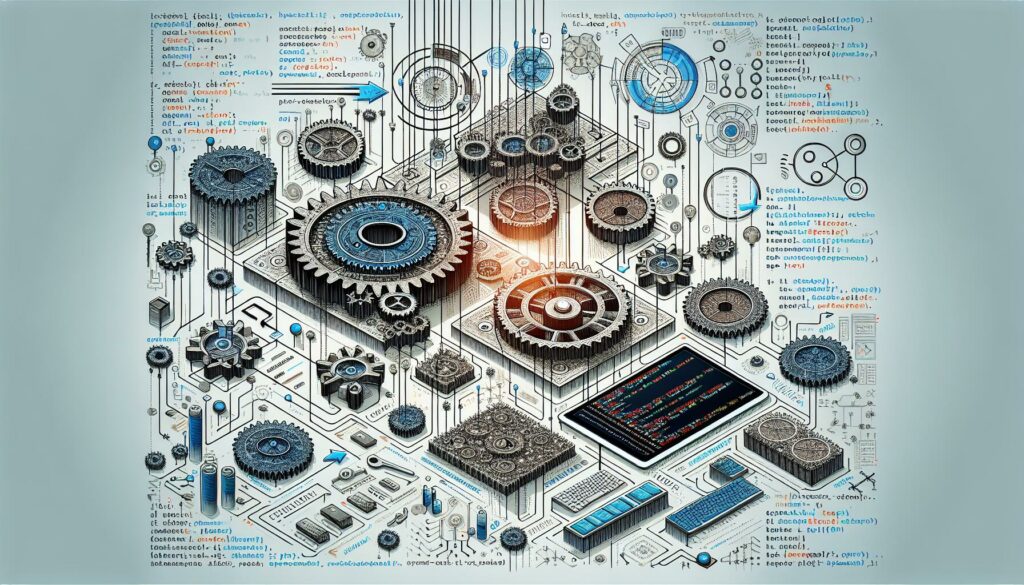
In the world of programming and software development, problem-solving is a crucial skill that separates great developers from the rest. While there are many approaches to tackling complex coding challenges, one technique that often gets overlooked is reverse engineering. This powerful method involves working backwards from the solution or known outputs to understand and solve tricky problems. In this article, we’ll explore the concept of reverse engineering coding problems, its benefits, and how you can apply this technique to enhance your problem-solving skills.
Understanding Reverse Engineering in Coding
Reverse engineering is a process of analyzing a system or product to determine how it works, often with the goal of creating a new system with similar functionality. In the context of coding problems, reverse engineering involves starting with the desired output or solution and working backwards to understand the steps needed to achieve that result.
This approach can be particularly useful when dealing with complex algorithms, data structures, or system designs. By breaking down the problem from the end result, you can gain insights into the underlying logic and patterns that might not be immediately apparent when approaching the problem from the beginning.
The Value of Reverse Engineering in Problem-Solving
Reverse engineering coding problems offers several benefits that can significantly improve your problem-solving skills:
- Clearer understanding of the problem: By starting with the solution, you can better grasp what the problem is actually asking for and what constraints you need to consider.
- Identification of patterns and relationships: Working backwards often reveals patterns and relationships between different elements of the problem that might not be obvious when starting from scratch.
- Improved algorithmic thinking: Reverse engineering forces you to think about the steps and logic required to reach a specific outcome, enhancing your ability to design efficient algorithms.
- Enhanced debugging skills: When you understand how a solution should work, it becomes easier to identify and fix issues in your own code.
- Faster problem-solving: With practice, reverse engineering can help you quickly recognize familiar patterns and solutions, speeding up your overall problem-solving process.
Step-by-Step Guide to Reverse Engineering Coding Problems
Let’s walk through a general approach to reverse engineering coding problems:
- Analyze the desired output: Start by thoroughly examining the expected result or solution. What does it look like? What are its characteristics?
- Identify key components: Break down the solution into its core components or steps. What are the essential elements that make up the final result?
- Work backwards: For each component, think about what needs to happen immediately before to produce that part of the solution.
- Look for patterns: As you work backwards, try to identify any recurring patterns or relationships between different steps.
- Consider edge cases: Think about how the solution handles different inputs or edge cases. This can provide insights into the underlying logic.
- Formulate a general approach: Based on your analysis, develop a high-level strategy for solving the problem.
- Implement and refine: Use your insights to implement a solution, then refine and optimize it based on your understanding of the problem.
Real-World Examples of Reverse Engineering Coding Problems
To better illustrate the power of reverse engineering, let’s look at some concrete examples of how this technique can be applied to solve complex coding problems.
Example 1: Reversing a Linked List
Problem: Given a singly linked list, reverse it in-place.
Let’s approach this problem using reverse engineering:
- Analyze the desired output: We want the last node to become the first, the second-to-last to become the second, and so on.
- Identify key components: We need to change the direction of each node’s pointer and update the head of the list.
- Work backwards: Starting from the last node, we need to make its next pointer point to the previous node.
- Look for patterns: This process of changing pointer direction needs to be repeated for each node.
- Consider edge cases: We need to handle empty lists and lists with only one node.
- Formulate a general approach: Iterate through the list, reversing pointers as we go, and keep track of the previous node.
Here’s a Python implementation based on this reverse-engineered approach:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverseLinkedList(head):
prev = None
current = head
while current is not None:
next_temp = current.next
current.next = prev
prev = current
current = next_temp
return prev # New head of the reversed list
Example 2: Implementing a Queue using Stacks
Problem: Implement a first-in-first-out (FIFO) queue using only two stacks.
Let’s reverse engineer this problem:
- Analyze the desired output: We need a queue that can enqueue and dequeue elements in FIFO order, but we can only use stack operations (push and pop).
- Identify key components: We need two stacks and methods for enqueue and dequeue operations.
- Work backwards: To dequeue (remove the first-in element), we need the bottom element of a stack. This suggests we might need to transfer elements between stacks.
- Look for patterns: The process of moving elements between stacks effectively reverses their order, which can be used to our advantage.
- Consider edge cases: We need to handle empty queues and efficient repeated operations.
- Formulate a general approach: Use one stack for enqueue operations and another for dequeue. Transfer elements between stacks only when necessary.
Here’s a Python implementation based on this reverse-engineered approach:
class Queue:
def __init__(self):
self.stack1 = [] # For enqueue
self.stack2 = [] # For dequeue
def enqueue(self, x):
self.stack1.append(x)
def dequeue(self):
if not self.stack2:
if not self.stack1:
return None # Queue is empty
while self.stack1:
self.stack2.append(self.stack1.pop())
return self.stack2.pop() if self.stack2 else None
def peek(self):
if not self.stack2:
if not self.stack1:
return None # Queue is empty
while self.stack1:
self.stack2.append(self.stack1.pop())
return self.stack2[-1] if self.stack2 else None
def empty(self):
return len(self.stack1) == 0 and len(self.stack2) == 0
Example 3: Implementing a Trie (Prefix Tree)
Problem: Implement a trie data structure for efficient string search and prefix matching.
Let’s apply reverse engineering to this problem:
- Analyze the desired output: We need a data structure that can efficiently store and search strings, particularly for prefix matching.
- Identify key components: We need nodes to represent characters, links between nodes, and a way to mark the end of words.
- Work backwards: To find a word or prefix quickly, we need to traverse the trie from the root, following links for each character.
- Look for patterns: Common prefixes are shared in the trie structure, which saves space and allows for efficient prefix matching.
- Consider edge cases: We need to handle empty strings, non-existent words, and case sensitivity.
- Formulate a general approach: Create a tree-like structure where each node represents a character and has links to child nodes for subsequent characters.
Here’s a Python implementation based on this reverse-engineered approach:
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def starts_with(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
Advanced Reverse Engineering Techniques
As you become more comfortable with basic reverse engineering, you can apply more advanced techniques to tackle even more complex problems:
1. Time Complexity Analysis
When reverse engineering a problem, pay close attention to the time complexity requirements. Often, the desired time complexity can give you clues about the optimal algorithm or data structure to use.
For example, if a problem requires O(log n) time complexity for search operations, it might suggest the use of a binary search or a balanced tree structure. Similarly, O(1) time complexity for insertions and deletions might indicate the need for a hash table.
2. Space-Time Tradeoffs
Sometimes, the solution to a problem involves trading off space for time or vice versa. When reverse engineering, consider how different space-time tradeoffs might lead to different solutions.
For instance, in dynamic programming problems, you might start by identifying the optimal substructure and then work backwards to determine whether a top-down (memoization) or bottom-up (tabulation) approach would be more suitable based on the problem’s constraints.
3. Pattern Recognition
As you gain experience with reverse engineering, you’ll start to recognize common patterns in problem solutions. This pattern recognition can help you quickly identify potential approaches to new problems.
Some common patterns to look out for include:
- Two-pointer techniques
- Sliding window approaches
- Divide and conquer strategies
- Graph traversal algorithms (DFS, BFS)
- Greedy algorithms
4. System Design Reverse Engineering
For larger-scale problems or system design questions, reverse engineering can be particularly powerful. Start by identifying the key components and interfaces of the desired system, then work backwards to determine how these components interact and what underlying technologies might be needed to support them.
For example, when designing a distributed cache system, you might start by defining the desired characteristics (fast read/write, scalability, consistency) and then work backwards to determine the architecture, data distribution strategy, and consistency protocols needed to achieve these goals.
Incorporating Reverse Engineering into Your Problem-Solving Toolkit
To make the most of reverse engineering in your coding practice, consider the following tips:
- Study existing solutions: When learning new algorithms or data structures, try to reverse engineer them to understand why they work the way they do.
- Practice with varied problems: Apply reverse engineering to a wide range of problem types to build your pattern recognition skills.
- Combine with other techniques: Use reverse engineering in conjunction with other problem-solving methods, such as breaking problems into smaller subproblems or looking for analogous problems you’ve solved before.
- Teach others: Explaining a reverse-engineered solution to someone else can deepen your understanding and help you articulate your thought process more clearly.
- Review and reflect: After solving a problem, take time to review your solution and consider how reverse engineering helped (or could have helped) in reaching the solution more efficiently.
Conclusion
Reverse engineering is a powerful technique that can significantly enhance your problem-solving skills in coding. By working backwards from the desired solution, you can gain valuable insights into complex problems, identify patterns more easily, and develop more efficient algorithms.
As you continue to develop your coding skills and prepare for technical interviews, remember that reverse engineering is just one tool in your problem-solving toolkit. Combine it with other techniques, practice regularly with diverse problems, and always strive to understand the underlying principles of the solutions you develop.
By mastering the art of reverse engineering coding problems, you’ll be better equipped to tackle complex challenges, optimize your solutions, and approach problem-solving with a more comprehensive and insightful perspective. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, incorporating reverse engineering into your problem-solving approach can give you a significant edge in understanding and solving complex coding challenges.