Reservoir Sampling: A Powerful Technique for Handling Large Data Streams
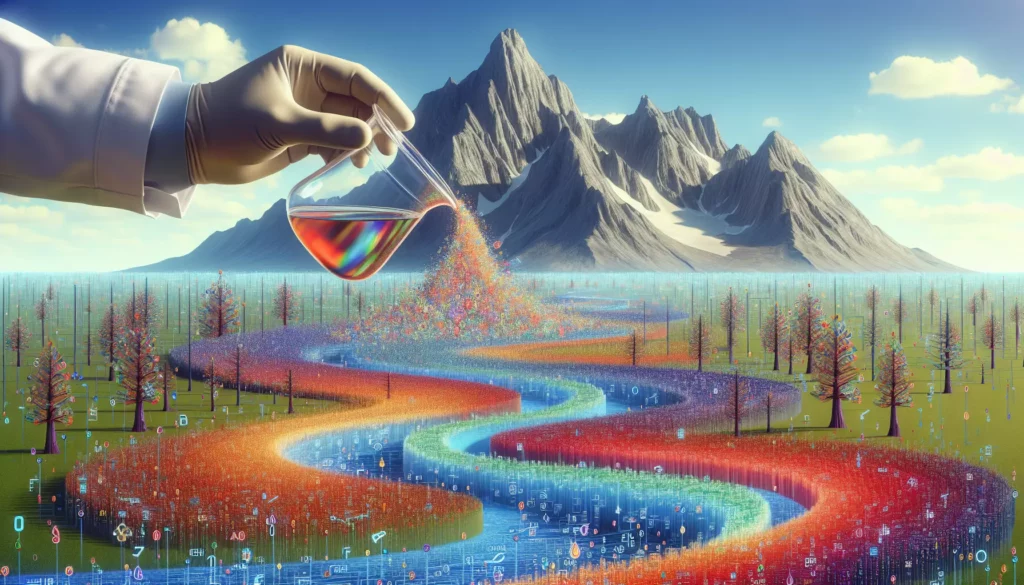
In the world of big data and algorithms, efficiently processing and sampling from large data streams is a crucial skill. One powerful technique that addresses this challenge is Reservoir Sampling. This algorithm allows us to select a random sample of k items from a stream of data of unknown or infinite length. In this comprehensive guide, we’ll explore the concept of Reservoir Sampling, its implementation, applications, and variations.
What is Reservoir Sampling?
Reservoir Sampling is a family of randomized algorithms designed to sample k items from a population of unknown size n, where n may be very large or even infinite. The key feature of Reservoir Sampling is that it can process a stream of data in a single pass, using only O(k) memory, regardless of the size of the input stream.
The basic idea behind Reservoir Sampling is to maintain a “reservoir” of k items as you process the stream. Initially, the reservoir is filled with the first k items. Then, for each subsequent item, you decide whether to include it in the reservoir or not based on a carefully chosen probability.
The Algorithm (Algorithm R)
The most common version of Reservoir Sampling is known as Algorithm R, introduced by Jeffrey Vitter in 1985. Here’s how it works:
- Create an array (the reservoir) of size k and fill it with the first k items from the stream.
- For each subsequent item i (where i > k):
- Generate a random number j between 1 and i (inclusive).
- If j ≤ k, replace the j-th item in the reservoir with the i-th item from the stream.
This algorithm ensures that at any point during the stream processing, each item seen so far has an equal probability of being in the reservoir.
Implementation in Python
Let’s implement the basic Reservoir Sampling algorithm in Python:
import random
def reservoir_sampling(stream, k):
reservoir = []
for i, item in enumerate(stream):
if i < k:
reservoir.append(item)
else:
j = random.randint(0, i)
if j < k:
reservoir[j] = item
return reservoir
# Example usage
stream = range(1000000) # Simulating a large stream of data
sample = reservoir_sampling(stream, 10)
print(sample)
This implementation works for any iterable stream of data and returns a list of k randomly sampled items.
How Does It Work?
The key to understanding Reservoir Sampling is to consider the probability of each item ending up in the final sample. Let’s break it down:
- For the first k items, the probability is 1 (100%) as they are automatically included in the reservoir.
- For the k+1 item, the probability of being selected is k/(k+1), and the probability of replacing any specific item in the reservoir is 1/k * k/(k+1) = 1/(k+1).
- For the k+2 item, the probability of being selected is k/(k+2), and again, the probability of replacing any specific item is 1/(k+2).
- This pattern continues for all subsequent items.
By the end of the stream, each item (including those in the initial reservoir) has an equal probability of k/n of being in the final sample, where n is the total number of items in the stream.
Time and Space Complexity
One of the main advantages of Reservoir Sampling is its efficiency:
- Time Complexity: O(n), where n is the number of items in the stream. We process each item once.
- Space Complexity: O(k), where k is the size of the reservoir. We only need to store k items in memory at any given time.
This makes Reservoir Sampling particularly useful for processing very large datasets or streams of unknown size, as it doesn’t require storing the entire dataset in memory.
Applications of Reservoir Sampling
Reservoir Sampling has numerous practical applications in various fields:
- Big Data Analysis: When dealing with datasets too large to fit in memory, Reservoir Sampling allows for efficient random sampling.
- Stream Processing: In scenarios where data is continuously flowing and the total size is unknown or infinite, Reservoir Sampling provides a way to maintain a representative sample.
- Online Advertising: For selecting a diverse set of ads to display from a large pool of candidates.
- Social Media: Sampling user interactions or content for analysis or display.
- Machine Learning: Creating balanced datasets for training models, especially when dealing with imbalanced classes.
- Database Systems: For query optimization and approximate query processing.
Variations and Extensions
While Algorithm R is the most well-known version of Reservoir Sampling, several variations and extensions have been developed to address specific needs or improve efficiency:
1. Weighted Reservoir Sampling
In some cases, you might want to sample items with different probabilities based on certain weights. Weighted Reservoir Sampling allows for this by adjusting the selection probability for each item based on its weight.
Here’s a simple implementation of weighted Reservoir Sampling:
import random
def weighted_reservoir_sampling(stream, k):
reservoir = []
weights_sum = 0
for i, (item, weight) in enumerate(stream):
weights_sum += weight
if i < k:
reservoir.append(item)
else:
j = random.uniform(0, weights_sum)
if j < weight:
reservoir[random.randint(0, k-1)] = item
return reservoir
# Example usage
stream = [(x, x**2) for x in range(1000)] # Items with weights
sample = weighted_reservoir_sampling(stream, 10)
print(sample)
2. Algorithm L (for Large k)
When k is large relative to n, Algorithm R can be inefficient as it generates many random numbers that don’t result in replacements. Algorithm L, also introduced by Vitter, addresses this by using skipping to reduce the number of random number generations.
3. Distributed Reservoir Sampling
For distributed systems processing large streams of data across multiple nodes, variations of Reservoir Sampling have been developed to maintain a consistent sample across the distributed environment.
Challenges and Considerations
While Reservoir Sampling is a powerful technique, it’s important to be aware of some challenges and considerations:
- Randomness Quality: The quality of the random number generator can affect the uniformity of the sampling. In critical applications, it’s important to use a high-quality random number generator.
- Sampling Without Replacement: The basic algorithm samples with replacement. If you need sampling without replacement, you’ll need to modify the algorithm or use additional data structures.
- Adapting to Changing k: If the desired sample size k needs to change dynamically, additional complexity is introduced.
- Parallel Processing: While distributed versions exist, parallelizing Reservoir Sampling can be challenging while maintaining the correct probabilities.
Implementing Reservoir Sampling in Different Programming Languages
To give you a broader perspective, let’s implement Reservoir Sampling in a few different programming languages:
Java Implementation
import java.util.Random;
public class ReservoirSampling {
public static int[] reservoirSample(int[] stream, int k) {
int[] reservoir = new int[k];
Random random = new Random();
// Fill the reservoir array
for (int i = 0; i < k; i++) {
reservoir[i] = stream[i];
}
// Process remaining elements
for (int i = k; i < stream.length; i++) {
int j = random.nextInt(i + 1);
if (j < k) {
reservoir[j] = stream[i];
}
}
return reservoir;
}
public static void main(String[] args) {
int[] stream = new int[1000000];
for (int i = 0; i < stream.length; i++) {
stream[i] = i;
}
int[] sample = reservoirSample(stream, 10);
for (int item : sample) {
System.out.print(item + " ");
}
}
}
C++ Implementation
#include <iostream>
#include <vector>
#include <random>
#include <ctime>
std::vector<int> reservoirSample(const std::vector<int>& stream, int k) {
std::vector<int> reservoir(k);
std::mt19937 gen(time(0));
// Fill the reservoir array
for (int i = 0; i < k; i++) {
reservoir[i] = stream[i];
}
// Process remaining elements
for (int i = k; i < stream.size(); i++) {
std::uniform_int_distribution<> dis(0, i);
int j = dis(gen);
if (j < k) {
reservoir[j] = stream[i];
}
}
return reservoir;
}
int main() {
std::vector<int> stream(1000000);
for (int i = 0; i < stream.size(); i++) {
stream[i] = i;
}
std::vector<int> sample = reservoirSample(stream, 10);
for (int item : sample) {
std::cout << item << " ";
}
std::cout << std::endl;
return 0;
}
JavaScript Implementation
function reservoirSample(stream, k) {
const reservoir = [];
for (let i = 0; i < stream.length; i++) {
if (i < k) {
reservoir.push(stream[i]);
} else {
const j = Math.floor(Math.random() * (i + 1));
if (j < k) {
reservoir[j] = stream[i];
}
}
}
return reservoir;
}
// Example usage
const stream = Array.from({length: 1000000}, (_, i) => i);
const sample = reservoirSample(stream, 10);
console.log(sample);
Advanced Topics in Reservoir Sampling
1. Adaptive Sampling
In some scenarios, you might want to adjust the sampling rate dynamically based on certain conditions. Adaptive Sampling techniques allow you to modify the reservoir size or sampling probabilities on-the-fly, which can be useful in scenarios like network traffic monitoring or adaptive machine learning algorithms.
2. Time-Decayed Reservoir Sampling
When dealing with evolving data streams, recent items might be more relevant than older ones. Time-Decayed Reservoir Sampling introduces a decay factor to give higher importance to more recent items while still maintaining a representative sample of the entire stream.
3. Distinct Reservoir Sampling
In some applications, you might want to sample distinct elements from a stream that contains duplicates. Distinct Reservoir Sampling algorithms have been developed to address this specific need, ensuring that the reservoir contains only unique items while maintaining randomness.
4. Sliding Window Reservoir Sampling
For applications that need to maintain a sample over a sliding window of the most recent N items in a stream, variations of Reservoir Sampling have been developed to efficiently update the sample as the window slides.
Real-World Case Studies
To better understand the practical applications of Reservoir Sampling, let’s look at a few real-world case studies:
1. Twitter’s Tweet Sampling
Twitter uses a version of Reservoir Sampling to provide its streaming API. This allows developers to access a random sample of all tweets in real-time, which is crucial for various analytics and research purposes.
2. Google’s BigQuery
Google’s BigQuery, a fully managed, serverless data warehouse, uses Reservoir Sampling as part of its approximate aggregate functions. This allows for quick estimation of results on large datasets without the need to process all the data.
3. Blockchain Analysis
In blockchain analysis, Reservoir Sampling can be used to maintain a representative sample of transactions or addresses for various analytical purposes, helping to manage the ever-growing size of blockchain data.
Best Practices and Tips
When implementing and using Reservoir Sampling in your projects, keep these best practices in mind:
- Use a good random number generator: The quality of your sampling depends heavily on the randomness of your number generator. In production systems, consider using cryptographically secure random number generators.
- Consider the size of k: Choose k based on your specific needs and the statistical properties you want to maintain. A larger k will give you a more representative sample but will require more memory.
- Test with different stream sizes: Ensure your implementation works correctly for both small and large streams, including edge cases.
- Be aware of biases: While Reservoir Sampling provides an unbiased sample, be cautious of introducing biases in how you use or interpret the results.
- Optimize for your use case: If you have specific requirements (e.g., weighted sampling, distinct elements), use or develop a variant of the algorithm that addresses those needs.
Conclusion
Reservoir Sampling is a powerful and elegant algorithm that solves the problem of sampling from data streams of unknown or infinite size. Its simplicity, efficiency, and versatility make it a valuable tool in the toolkit of any data scientist or software engineer dealing with large-scale data processing.
As we’ve explored in this comprehensive guide, Reservoir Sampling has numerous applications across various domains, from big data analysis to online advertising and machine learning. Its ability to provide a representative sample with minimal memory usage makes it particularly well-suited for modern data processing challenges.
Moreover, the various extensions and adaptations of the basic algorithm demonstrate its flexibility in addressing specific needs, such as weighted sampling, time-decay, or distributed processing.
As data continues to grow in volume and velocity, techniques like Reservoir Sampling will only become more important. Whether you’re building a real-time analytics system, designing a distributed database, or developing machine learning models on streaming data, understanding and applying Reservoir Sampling can significantly enhance your ability to work with large-scale data efficiently.
By mastering Reservoir Sampling and its variants, you’ll be well-equipped to tackle a wide range of sampling and data processing challenges in your future projects and career. Keep experimenting, adapting the algorithm to your specific needs, and always be on the lookout for new developments in this fascinating area of computer science and data analysis.