Remove None from List in Python: A Comprehensive Guide
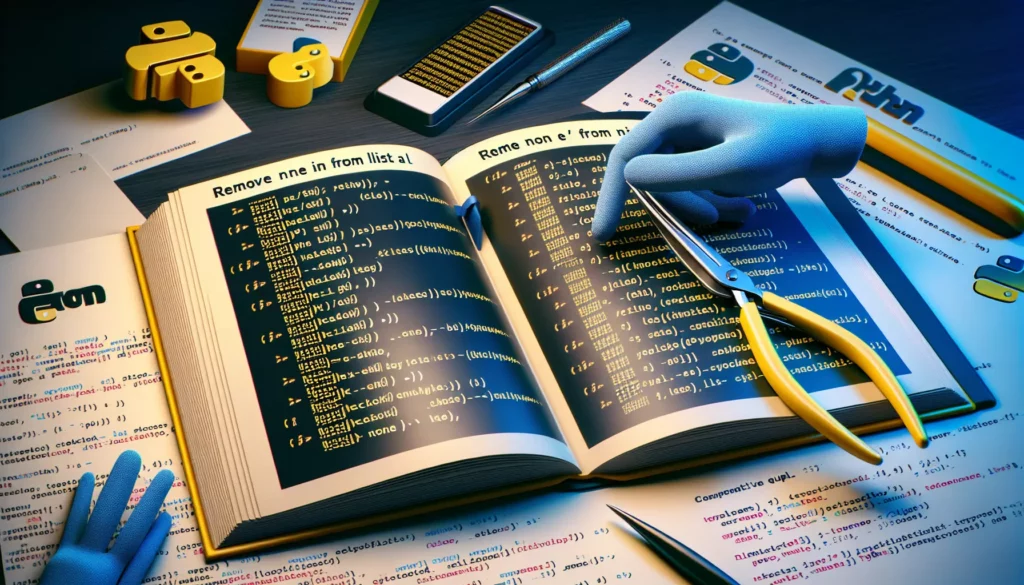
When working with lists in Python, you may encounter situations where you need to remove None
values from your data. This process, known as filtering out None
values, is a common task in data cleaning and preprocessing. In this comprehensive guide, we’ll explore various methods to remove None
from a list in Python, discussing their pros and cons, and providing practical examples for each approach.
Table of Contents
- Understanding None in Python
- Method 1: Using a List Comprehension
- Method 2: Using the filter() Function
- Method 3: Using a for Loop
- Method 4: Using the remove() Method
- Method 5: Using the list() Constructor with filter()
- Performance Comparison
- Handling None in Nested Lists
- Best Practices and Tips
- Common Pitfalls and How to Avoid Them
- Conclusion
1. Understanding None in Python
Before we dive into the methods of removing None
from a list, it’s essential to understand what None
represents in Python. None
is a special constant in Python that represents the absence of a value or a null value. It’s often used to signify that a variable has no value assigned to it.
Here are some key points about None
:
None
is a singleton object of theNoneType
class.- It’s used to represent the absence of a value, similar to null in other programming languages.
None
is falsy in boolean contexts.- It’s commonly used as a default return value for functions that don’t explicitly return anything.
Now that we have a basic understanding of None
, let’s explore different methods to remove it from a list.
2. Method 1: Using a List Comprehension
List comprehension is a concise and readable way to create new lists based on existing lists. It’s an elegant solution for filtering out None
values from a list.
Syntax:
new_list = [item for item in original_list if item is not None]
Example:
original_list = [1, None, 2, None, 3, 4, None, 5]
filtered_list = [item for item in original_list if item is not None]
print(filtered_list)
# Output: [1, 2, 3, 4, 5]
Pros:
- Concise and readable
- Creates a new list without modifying the original
- Efficient for small to medium-sized lists
Cons:
- May not be as efficient for very large lists due to memory usage
- Less intuitive for beginners
3. Method 2: Using the filter() Function
The filter()
function is a built-in Python function that creates an iterator of elements for which a function returns true. We can use it with a lambda function to remove None
values from a list.
Syntax:
new_list = list(filter(lambda x: x is not None, original_list))
Example:
original_list = [1, None, 2, None, 3, 4, None, 5]
filtered_list = list(filter(lambda x: x is not None, original_list))
print(filtered_list)
# Output: [1, 2, 3, 4, 5]
Pros:
- Functional programming approach
- Creates a new list without modifying the original
- Can be more memory-efficient than list comprehension for large lists
Cons:
- Slightly less readable than list comprehension
- May be slower for small lists due to function call overhead
4. Method 3: Using a for Loop
For those who prefer a more traditional approach, using a for loop to iterate through the list and append non-None values to a new list is a straightforward method.
Example:
original_list = [1, None, 2, None, 3, 4, None, 5]
filtered_list = []
for item in original_list:
if item is not None:
filtered_list.append(item)
print(filtered_list)
# Output: [1, 2, 3, 4, 5]
Pros:
- Easy to understand, especially for beginners
- Flexible, allowing for more complex filtering logic if needed
- Creates a new list without modifying the original
Cons:
- More verbose than other methods
- May be less efficient for large lists compared to built-in functions or list comprehensions
5. Method 4: Using the remove() Method
If you need to modify the original list in-place, you can use the remove()
method in combination with a while loop to remove all None
values.
Example:
original_list = [1, None, 2, None, 3, 4, None, 5]
while None in original_list:
original_list.remove(None)
print(original_list)
# Output: [1, 2, 3, 4, 5]
Pros:
- Modifies the original list in-place, saving memory
- Simple to implement
Cons:
- Can be inefficient for large lists with many
None
values - Modifies the original list, which may not always be desirable
6. Method 5: Using the list() Constructor with filter()
This method combines the filter()
function with the list()
constructor and the None
keyword to create a new list without None
values.
Syntax:
new_list = list(filter(None, original_list))
Example:
original_list = [1, None, 2, None, 3, 4, None, 5]
filtered_list = list(filter(None, original_list))
print(filtered_list)
# Output: [1, 2, 3, 4, 5]
Pros:
- Very concise
- Efficient for large lists
- Creates a new list without modifying the original
Cons:
- May be less intuitive for beginners
- Will also remove other falsy values (e.g., 0, empty strings) if present in the list
7. Performance Comparison
When choosing a method to remove None
from a list, it’s important to consider the performance implications, especially for large datasets. Let’s compare the performance of the methods we’ve discussed using the timeit
module.
import timeit
def test_list_comprehension(lst):
return [item for item in lst if item is not None]
def test_filter_lambda(lst):
return list(filter(lambda x: x is not None, lst))
def test_for_loop(lst):
result = []
for item in lst:
if item is not None:
result.append(item)
return result
def test_remove_method(lst):
lst_copy = lst.copy()
while None in lst_copy:
lst_copy.remove(None)
return lst_copy
def test_filter_none(lst):
return list(filter(None, lst))
# Create a large list with None values
large_list = [None if i % 10 == 0 else i for i in range(100000)]
# Test each method
print("List Comprehension:", timeit.timeit(lambda: test_list_comprehension(large_list), number=100))
print("Filter with Lambda:", timeit.timeit(lambda: test_filter_lambda(large_list), number=100))
print("For Loop:", timeit.timeit(lambda: test_for_loop(large_list), number=100))
print("Remove Method:", timeit.timeit(lambda: test_remove_method(large_list), number=100))
print("Filter None:", timeit.timeit(lambda: test_filter_none(large_list), number=100))
The results may vary depending on your system, but generally, you’ll find that:
- List comprehension and
filter(None, ...)
are usually the fastest methods. - The for loop approach is slightly slower but still efficient.
- The
remove()
method is typically the slowest, especially for large lists with manyNone
values.
8. Handling None in Nested Lists
Sometimes, you may need to remove None
values from nested lists. Here’s an example of how to do this using a recursive function:
def remove_none_recursive(item):
if isinstance(item, list):
return [remove_none_recursive(x) for x in item if x is not None]
return item
nested_list = [1, [2, None, 3], [4, [5, None]], None, 6]
result = remove_none_recursive(nested_list)
print(result)
# Output: [1, [2, 3], [4, [5]], 6]
This function recursively processes each element of the list, removing None
values at all levels of nesting.
9. Best Practices and Tips
When working with None
values in lists, keep these best practices in mind:
- Use
is not None
instead of!= None
for comparison. It’s more explicit and slightly faster. - Consider the size of your list when choosing a method. For small lists, readability might be more important than performance.
- If you’re working with large datasets, consider using NumPy arrays or Pandas DataFrames, which have optimized methods for handling missing values.
- Be cautious when using methods that modify the original list if you need to preserve the original data.
- When using
filter(None, ...)
, be aware that it will also remove other falsy values (0, ”, [], {}, etc.).
10. Common Pitfalls and How to Avoid Them
Pitfall 1: Modifying a List While Iterating
Avoid modifying a list while iterating over it, as this can lead to unexpected results:
# Incorrect
original_list = [1, None, 2, None, 3]
for item in original_list:
if item is None:
original_list.remove(item) # This can cause issues
# Correct
original_list = [1, None, 2, None, 3]
original_list = [item for item in original_list if item is not None]
Pitfall 2: Confusing None with Other Falsy Values
Be careful not to confuse None
with other falsy values:
# This will remove None, but also 0 and empty strings
filtered_list = list(filter(bool, [0, None, '', 1, 2]))
print(filtered_list) # Output: [1, 2]
# To remove only None
filtered_list = list(filter(lambda x: x is not None, [0, None, '', 1, 2]))
print(filtered_list) # Output: [0, '', 1, 2]
Pitfall 3: Inefficient Removal in Large Lists
For very large lists, avoid using methods that repeatedly search through the list:
# Inefficient for large lists
while None in large_list:
large_list.remove(None)
# More efficient
large_list = [item for item in large_list if item is not None]
11. Conclusion
Removing None
values from a list in Python is a common task that can be accomplished through various methods. Each approach has its strengths and weaknesses, and the best choice depends on your specific use case, the size of your data, and your performance requirements.
Here’s a quick summary of when to use each method:
- List Comprehension: Use for small to medium-sized lists when you want a readable and concise solution.
- filter() Function: Ideal for larger lists and when you prefer a functional programming approach.
- For Loop: Good for beginners or when you need more complex filtering logic.
- remove() Method: Use when you need to modify the original list in-place and don’t mind the performance hit for larger lists.
- list(filter(None, …)): Best for very large lists when you want to remove all falsy values, not just None.
Remember to consider the context of your project, the size of your data, and the potential for future scaling when choosing a method. By understanding these different approaches and their implications, you’ll be well-equipped to handle None
values in your Python lists efficiently and effectively.