Remove Invalid Parentheses: A Comprehensive Guide to Solving This Classic Algorithm Problem
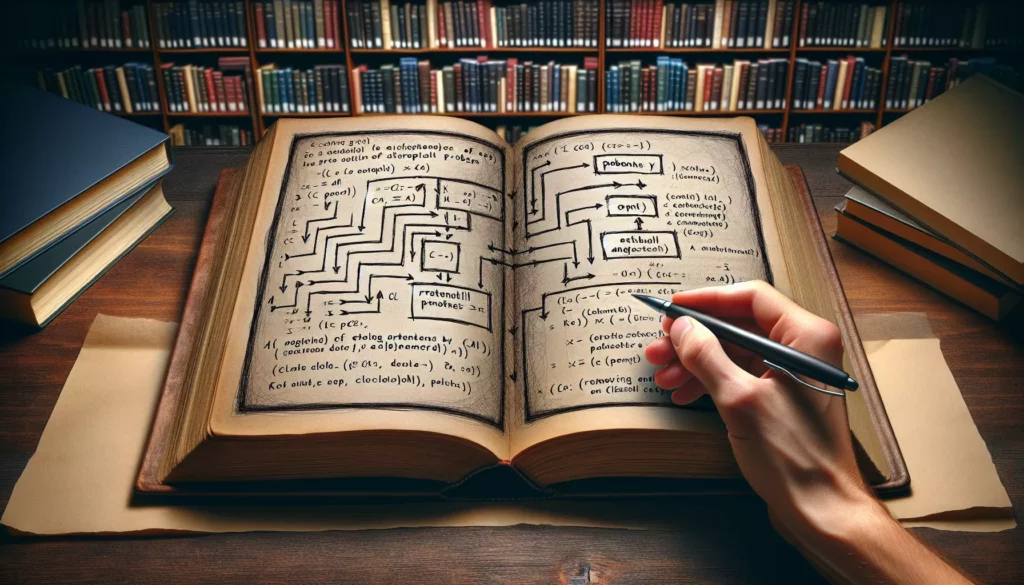
When it comes to coding interviews and algorithmic challenges, the “Remove Invalid Parentheses” problem is a classic that often appears in technical assessments for major tech companies. This problem not only tests your ability to work with strings and data structures but also challenges your problem-solving skills and understanding of recursion and backtracking. In this comprehensive guide, we’ll dive deep into this problem, explore multiple approaches to solve it, and provide you with the tools you need to tackle it confidently in your next coding interview.
Understanding the Problem
Before we jump into the solutions, let’s clearly define the problem:
Given a string s that contains parentheses and letters, remove the minimum number of invalid parentheses to make the input string valid. Return all possible results.
Note: The input string may contain letters other than the parentheses ( and ).
For example:
- Input: “()())()” Output: [“()()()”, “(())()”]
- Input: “(a)())()” Output: [“(a)()()”, “(a())()”]
- Input: “)(” Output: [“”]
The challenge here is not just to remove invalid parentheses, but to do so in a way that:
- Removes the minimum number of parentheses
- Finds all possible valid combinations
- Handles cases where there are characters other than parentheses
Approaching the Problem
There are several ways to approach this problem, each with its own trade-offs in terms of time complexity, space complexity, and implementation difficulty. We’ll explore three main approaches:
- Brute Force
- Backtracking
- BFS (Breadth-First Search)
1. Brute Force Approach
The brute force approach involves generating all possible substrings by removing parentheses and then checking each one for validity. While this method is straightforward, it’s not efficient for larger inputs.
Algorithm:
- Generate all possible substrings of the input string by removing parentheses.
- Check each substring for validity.
- Keep track of the maximum length of valid substrings.
- Return all valid substrings of the maximum length.
Implementation in Python:
from itertools import combinations
def removeInvalidParentheses(s):
def isValid(string):
count = 0
for char in string:
if char == '(':
count += 1
elif char == ')':
count -= 1
if count < 0:
return False
return count == 0
level = {s}
while True:
valid = list(filter(isValid, level))
if valid:
return valid
level = {s[:i] + s[i+1:] for s in level for i in range(len(s)) if s[i] in '()'}
This approach, while simple to understand, has a time complexity of O(2^n), where n is the length of the input string. This makes it impractical for longer inputs.
2. Backtracking Approach
The backtracking approach is more efficient than the brute force method. It involves systematically trying different combinations of parentheses removal while keeping track of the minimum number of removals needed.
Algorithm:
- Count the number of left and right parentheses that need to be removed.
- Use a recursive function to try removing left and right parentheses.
- Keep track of the current index, count of left and right parentheses, and the current string.
- If a valid string is found with the minimum number of removals, add it to the result set.
Implementation in Python:
class Solution:
def removeInvalidParentheses(self, s: str) -> List[str]:
left = 0
right = 0
# First pass: count the misplaced parentheses
for char in s:
if char == '(':
left += 1
elif char == ')':
if left == 0:
right += 1
else:
left -= 1
result = set()
self.backtrack(s, 0, left, right, 0, '', result)
return list(result)
def backtrack(self, s, index, left, right, open_count, current, result):
if index == len(s):
if left == 0 and right == 0 and open_count == 0:
result.add(current)
return
current_char = s[index]
if current_char != '(' and current_char != ')':
self.backtrack(s, index + 1, left, right, open_count, current + current_char, result)
else:
if current_char == '(':
if left > 0:
self.backtrack(s, index + 1, left - 1, right, open_count, current, result)
self.backtrack(s, index + 1, left, right, open_count + 1, current + current_char, result)
elif current_char == ')':
if right > 0:
self.backtrack(s, index + 1, left, right - 1, open_count, current, result)
if open_count > 0:
self.backtrack(s, index + 1, left, right, open_count - 1, current + current_char, result)
This backtracking approach is more efficient than the brute force method, with a time complexity that’s hard to express precisely but is generally much better than O(2^n) for most inputs.
3. BFS (Breadth-First Search) Approach
The BFS approach treats the problem as a graph traversal, where each node represents a string, and edges represent the removal of a single parenthesis.
Algorithm:
- Start with the original string as the root of the BFS.
- Generate all strings that can be formed by removing one parenthesis.
- Check if any of these strings are valid. If so, we’ve found the solution(s).
- If no valid strings are found, repeat the process with the strings from step 2.
Implementation in Python:
from collections import deque
class Solution:
def removeInvalidParentheses(self, s: str) -> List[str]:
def isValid(s):
count = 0
for char in s:
if char == '(':
count += 1
elif char == ')':
count -= 1
if count < 0:
return False
return count == 0
level = {s}
while True:
valid = list(filter(isValid, level))
if valid:
return valid
next_level = set()
for item in level:
for i in range(len(item)):
if item[i] in '()':
next_level.add(item[:i] + item[i+1:])
level = next_level
The BFS approach has a time complexity of O(2^n) in the worst case, but it’s generally faster in practice because it finds the solution at the earliest level possible.
Comparing the Approaches
Let’s compare these three approaches:
Approach | Time Complexity | Space Complexity | Pros | Cons |
---|---|---|---|---|
Brute Force | O(2^n) | O(n) | Simple to implement | Very slow for large inputs |
Backtracking | Difficult to express precisely, but generally better than O(2^n) | O(n) | More efficient than brute force | More complex implementation |
BFS | O(2^n) worst case, but often faster in practice | O(n) | Finds minimum removals quickly | Can be memory-intensive for large inputs |
Advanced Considerations
When tackling this problem in a real coding interview, there are several additional factors to consider:
1. Handling Edge Cases
Make sure your solution handles edge cases correctly:
- Empty string input
- String with no parentheses
- String with all invalid parentheses
- Very long strings
2. Optimizing for Large Inputs
If the interviewer asks about handling very large inputs, you might need to consider more optimized approaches:
- Using bit manipulation to represent substrings
- Implementing a more efficient string matching algorithm
- Considering parallel processing for extremely large inputs
3. Extending the Problem
Interviewers might ask you to extend the problem. Some possible extensions include:
- Handling multiple types of brackets (e.g., (), [], {})
- Finding the lexicographically smallest valid string
- Optimizing for space complexity in addition to time complexity
Practicing and Improving
To master this problem and similar algorithmic challenges, consider the following tips:
- Understand the fundamentals: Make sure you have a solid grasp of data structures (especially strings and stacks) and algorithms (recursion, backtracking, BFS).
- Practice regularly: Solve similar problems on platforms like LeetCode, HackerRank, or AlgoCademy.
- Analyze different approaches: For each problem, try to come up with multiple solutions and analyze their trade-offs.
- Mock interviews: Practice explaining your thought process out loud, as you would in a real interview.
- Time yourself: Try to solve the problem within a realistic time frame (e.g., 30-45 minutes).
- Review and reflect: After solving a problem, look at other people’s solutions to learn new techniques and optimizations.
Conclusion
The “Remove Invalid Parentheses” problem is a challenging but instructive algorithmic puzzle that tests various aspects of your coding skills. By understanding different approaches to solve this problem – from brute force to more optimized methods like backtracking and BFS – you’ll not only be better prepared for this specific challenge but also improve your overall problem-solving skills.
Remember, the key to success in coding interviews is not just knowing the solution to specific problems, but understanding the underlying principles and being able to apply them flexibly. Practice regularly, focus on understanding rather than memorizing, and always be ready to discuss your thought process.
As you continue your journey in algorithmic problem-solving, consider exploring related topics such as dynamic programming, graph algorithms, and advanced string manipulation techniques. These will further enhance your ability to tackle complex coding challenges and excel in technical interviews at top tech companies.
Happy coding, and best of luck in your future interviews!