Regression vs Classification: Understanding the Key Differences in Machine Learning
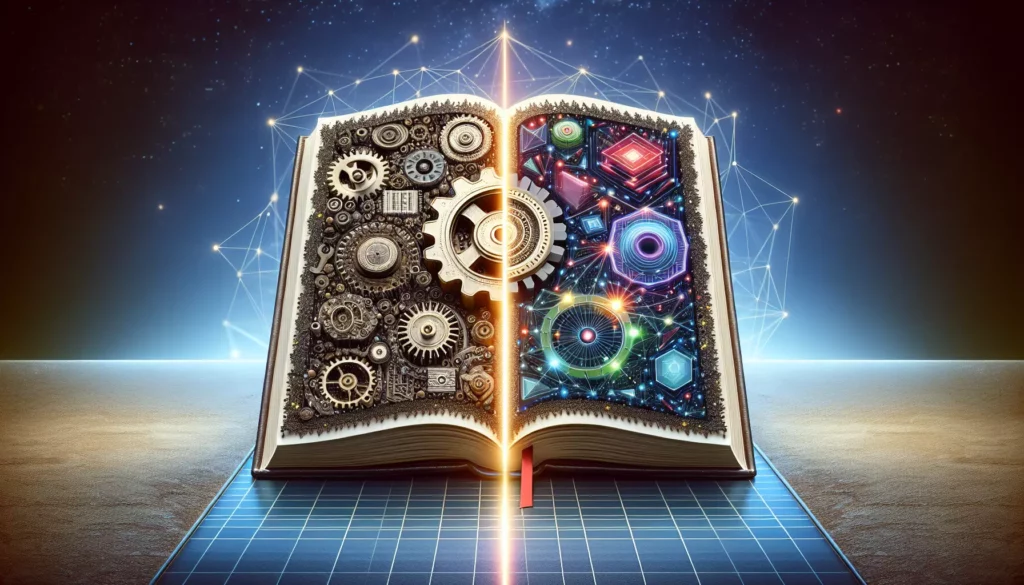
In the world of machine learning and data science, two fundamental types of predictive modeling stand out: regression and classification. These techniques form the backbone of many algorithms and applications in the field. Whether you’re a budding data scientist, a coding enthusiast, or someone preparing for technical interviews at major tech companies, understanding the differences between regression and classification is crucial.
In this comprehensive guide, we’ll dive deep into the concepts of regression and classification, explore their key differences, and provide practical examples to help solidify your understanding. By the end of this article, you’ll have a clear grasp of when to use each technique and how they fit into the broader landscape of machine learning.
Table of Contents
- What is Regression?
- What is Classification?
- Key Differences Between Regression and Classification
- When to Use Regression vs Classification
- Common Algorithms for Regression and Classification
- Practical Examples and Use Cases
- Evaluation Metrics for Regression and Classification
- Challenges and Considerations
- Future Trends and Advanced Techniques
- Conclusion
What is Regression?
Regression is a statistical method used in machine learning to predict a continuous output variable based on one or more input variables. In essence, regression models try to establish a relationship between independent variables (inputs) and a dependent variable (output) by fitting a line or curve to the data.
The primary goal of regression is to answer questions like “How much?” or “How many?” For example, predicting house prices, estimating sales figures, or forecasting temperature are all regression problems.
Types of Regression
- Linear Regression: The simplest form of regression, where the relationship between variables is modeled using a straight line.
- Polynomial Regression: Used when the relationship between variables is non-linear and can be modeled using a curved line.
- Multiple Regression: Involves multiple independent variables to predict the dependent variable.
- Logistic Regression: Despite its name, this is actually a classification algorithm used for binary outcomes.
Example of Linear Regression in Python
Here’s a simple example of how to implement linear regression using Python and the scikit-learn library:
import numpy as np
from sklearn.linear_model import LinearRegression
import matplotlib.pyplot as plt
# Generate sample data
X = np.array([1, 2, 3, 4, 5]).reshape(-1, 1)
y = np.array([2, 4, 5, 4, 5])
# Create and fit the model
model = LinearRegression()
model.fit(X, y)
# Make predictions
X_test = np.array([6, 7, 8]).reshape(-1, 1)
y_pred = model.predict(X_test)
# Plot the results
plt.scatter(X, y, color='blue')
plt.plot(X, model.predict(X), color='red')
plt.scatter(X_test, y_pred, color='green')
plt.show()
print(f"Predictions for X_test: {y_pred}")
This code snippet demonstrates how to create a simple linear regression model, fit it to some sample data, and make predictions on new data points.
What is Classification?
Classification is another fundamental task in machine learning where the goal is to predict a categorical output variable (class or label) based on input variables. Unlike regression, which predicts continuous values, classification models assign input data to predefined categories or classes.
Classification answers questions like “Which category?” or “Is it A or B?” For instance, determining whether an email is spam or not, identifying the species of a plant based on its features, or diagnosing a medical condition are all classification problems.
Types of Classification
- Binary Classification: The simplest form, where there are only two possible output classes (e.g., spam or not spam).
- Multi-class Classification: Involves three or more possible output classes (e.g., classifying animals into species).
- Multi-label Classification: Where each instance can belong to multiple classes simultaneously (e.g., tagging an image with multiple objects).
Example of Binary Classification in Python
Here’s a simple example of how to implement binary classification using the logistic regression algorithm in Python:
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
import numpy as np
# Generate sample data
X = np.random.randn(100, 2)
y = (X[:, 0] + X[:, 1] > 0).astype(int)
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions on the test set
y_pred = model.predict(X_test)
# Calculate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
This code demonstrates how to create a logistic regression model for binary classification, train it on a dataset, and evaluate its performance using accuracy as a metric.
Key Differences Between Regression and Classification
Now that we’ve introduced both regression and classification, let’s explore the key differences between these two fundamental machine learning techniques:
- Output Type:
- Regression: Predicts continuous, numerical values (e.g., price, temperature, height).
- Classification: Predicts discrete, categorical labels or classes (e.g., spam/not spam, dog/cat/bird).
- Goal:
- Regression: Aims to find the best-fitting line or curve that describes the relationship between variables.
- Classification: Aims to find the decision boundary that best separates different classes.
- Evaluation Metrics:
- Regression: Uses metrics like Mean Squared Error (MSE), Root Mean Squared Error (RMSE), and R-squared.
- Classification: Uses metrics like Accuracy, Precision, Recall, F1-score, and ROC AUC.
- Algorithms:
- Regression: Linear Regression, Polynomial Regression, Ridge Regression, Lasso Regression.
- Classification: Logistic Regression, Decision Trees, Random Forests, Support Vector Machines (SVM), Naive Bayes.
- Interpretation:
- Regression: Results are interpreted as the predicted value of the dependent variable.
- Classification: Results are interpreted as the probability of belonging to a certain class or the class label itself.
- Loss Functions:
- Regression: Typically uses Mean Squared Error (MSE) or Mean Absolute Error (MAE) as loss functions.
- Classification: Often uses Cross-Entropy Loss or Hinge Loss (for SVM) as loss functions.
When to Use Regression vs Classification
Choosing between regression and classification depends on the nature of your problem and the type of output you’re trying to predict. Here are some guidelines to help you decide:
Use Regression When:
- The output variable is continuous (e.g., price, temperature, age).
- You want to predict a specific numeric value.
- The relationship between variables can be described by a continuous function.
- Examples:
- Predicting house prices based on features like size, location, and number of rooms.
- Estimating a person’s income based on education level, experience, and other factors.
- Forecasting sales figures for the next quarter based on historical data and market trends.
Use Classification When:
- The output variable is categorical or discrete (e.g., yes/no, red/green/blue).
- You want to assign input data to predefined categories or classes.
- The goal is to make a decision or categorization based on input features.
- Examples:
- Determining whether an email is spam or not based on its content and metadata.
- Classifying images of animals into their respective species.
- Predicting whether a customer will churn or not based on their behavior and demographics.
Common Algorithms for Regression and Classification
Both regression and classification have a wide array of algorithms available. Here’s an overview of some common algorithms for each:
Regression Algorithms:
- Linear Regression: The simplest and most widely used regression algorithm, suitable for linear relationships between variables.
- Polynomial Regression: An extension of linear regression that can model non-linear relationships using polynomial functions.
- Ridge Regression: A regularized version of linear regression that helps prevent overfitting by adding a penalty term to the loss function.
- Lasso Regression: Similar to Ridge regression but uses a different penalty term that can lead to feature selection.
- Elastic Net: Combines the penalties of both Ridge and Lasso regression.
- Decision Tree Regression: Uses a tree-like model of decisions to make predictions.
- Random Forest Regression: An ensemble method that combines multiple decision trees to improve prediction accuracy and reduce overfitting.
Classification Algorithms:
- Logistic Regression: Despite its name, this is a classification algorithm used for binary and multi-class classification problems.
- K-Nearest Neighbors (KNN): A simple, instance-based learning algorithm that classifies new data points based on the majority class of their k nearest neighbors.
- Decision Trees: Similar to regression trees but used for classification tasks.
- Random Forests: An ensemble of decision trees used for classification.
- Support Vector Machines (SVM): A powerful algorithm that finds the optimal hyperplane to separate classes in high-dimensional space.
- Naive Bayes: A probabilistic classifier based on Bayes’ theorem, often used for text classification tasks.
- Gradient Boosting Classifiers: Ensemble methods like XGBoost, LightGBM, and CatBoost that build strong classifiers by combining weak learners.
Practical Examples and Use Cases
To further illustrate the differences between regression and classification, let’s explore some practical examples and use cases for each:
Regression Examples:
- House Price Prediction:
Predicting the price of a house based on features like square footage, number of bedrooms, location, and age of the property. This is a classic regression problem where the output (price) is a continuous value.
from sklearn.ensemble import RandomForestRegressor from sklearn.model_selection import train_test_split from sklearn.metrics import mean_squared_error import numpy as np # Assume X contains features and y contains house prices X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) model = RandomForestRegressor(n_estimators=100, random_state=42) model.fit(X_train, y_train) y_pred = model.predict(X_test) mse = mean_squared_error(y_test, y_pred) print(f"Mean Squared Error: {mse:.2f}")
- Stock Price Forecasting:
Predicting future stock prices based on historical data, market trends, and other relevant factors. This is a time series regression problem where the goal is to forecast continuous values over time.
from statsmodels.tsa.arima.model import ARIMA import pandas as pd # Assume df is a DataFrame with a 'Date' column and a 'Price' column df['Date'] = pd.to_datetime(df['Date']) df.set_index('Date', inplace=True) model = ARIMA(df['Price'], order=(1, 1, 1)) results = model.fit() # Forecast the next 30 days forecast = results.forecast(steps=30) print(forecast)
Classification Examples:
- Spam Email Detection:
Classifying emails as spam or not spam based on their content and metadata. This is a binary classification problem where the output is a categorical label (spam/not spam).
from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.naive_bayes import MultinomialNB from sklearn.pipeline import Pipeline from sklearn.metrics import accuracy_score, classification_report # Assume X contains email text and y contains labels (0 for not spam, 1 for spam) X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) pipeline = Pipeline([ ('tfidf', TfidfVectorizer()), ('clf', MultinomialNB()) ]) pipeline.fit(X_train, y_train) y_pred = pipeline.predict(X_test) print(f"Accuracy: {accuracy_score(y_test, y_pred):.2f}") print(classification_report(y_test, y_pred))
- Image Classification:
Classifying images into predefined categories (e.g., cat, dog, bird). This is a multi-class classification problem where the output is one of several possible class labels.
from tensorflow.keras.applications import MobileNetV2 from tensorflow.keras.preprocessing.image import ImageDataGenerator # Assume we have a directory structure with subdirectories for each class train_datagen = ImageDataGenerator(rescale=1./255, validation_split=0.2) train_generator = train_datagen.flow_from_directory( 'path/to/images', target_size=(224, 224), batch_size=32, class_mode='categorical', subset='training' ) validation_generator = train_datagen.flow_from_directory( 'path/to/images', target_size=(224, 224), batch_size=32, class_mode='categorical', subset='validation' ) base_model = MobileNetV2(weights='imagenet', include_top=False, input_shape=(224, 224, 3)) x = base_model.output x = GlobalAveragePooling2D()(x) x = Dense(1024, activation='relu')(x) output = Dense(num_classes, activation='softmax')(x) model = Model(inputs=base_model.input, outputs=output) model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy']) model.fit(train_generator, validation_data=validation_generator, epochs=10)
Evaluation Metrics for Regression and Classification
Choosing the right evaluation metrics is crucial for assessing the performance of your machine learning models. The metrics used for regression and classification differ due to the nature of their outputs.
Regression Metrics:
- Mean Squared Error (MSE): Measures the average squared difference between predicted and actual values. Lower values indicate better performance.
from sklearn.metrics import mean_squared_error mse = mean_squared_error(y_true, y_pred)
- Root Mean Squared Error (RMSE): The square root of MSE, which provides an error measure in the same unit as the target variable.
import numpy as np rmse = np.sqrt(mean_squared_error(y_true, y_pred))
- Mean Absolute Error (MAE): Measures the average absolute difference between predicted and actual values. Less sensitive to outliers than MSE.
from sklearn.metrics import mean_absolute_error mae = mean_absolute_error(y_true, y_pred)
- R-squared (R²): Represents the proportion of variance in the dependent variable that is predictable from the independent variable(s). Ranges from 0 to 1, with 1 indicating perfect prediction.
from sklearn.metrics import r2_score r2 = r2_score(y_true, y_pred)
Classification Metrics:
- Accuracy: The ratio of correct predictions to the total number of predictions. Simple but can be misleading for imbalanced datasets.
from sklearn.metrics import accuracy_score accuracy = accuracy_score(y_true, y_pred)
- Precision: The ratio of true positive predictions to the total number of positive predictions. Measures the model’s ability to avoid labeling negative instances as positive.
from sklearn.metrics import precision_score precision = precision_score(y_true, y_pred)
- Recall: The ratio of true positive predictions to the total number of actual positive instances. Measures the model’s ability to find all positive instances.
from sklearn.metrics import recall_score recall = recall_score(y_true, y_pred)
- F1-score: The harmonic mean of precision and recall, providing a single score that balances both metrics.
from sklearn.metrics import f1_score f1 = f1_score(y_true, y_pred)
- ROC AUC: Area Under the Receiver Operating Characteristic curve. Measures the model’s ability to distinguish between classes. Ranges from 0.5 (random guessing) to 1 (perfect classification).
from sklearn.metrics import roc_auc_score roc_auc = roc_auc_score(y_true, y_pred_proba)
Challenges and Considerations
When working with regression and classification models, there are several challenges and considerations to keep in mind:
Common Challenges:
- Overfitting: When a model learns the training data too well, including noise and outliers, leading to poor generalization on new data.
- Solution: Use regularization techniques, cross-validation, and ensemble methods.
- Underfitting: When a model is too simple to capture the underlying patterns in the data.
- Solution: Increase model complexity, add more relevant features, or use more sophisticated algorithms.
- Imbalanced Datasets: When one class in a classification problem has significantly more samples than others.
- Solution: Use techniques like oversampling, undersampling, or SMOTE (Synthetic Minority Over-sampling Technique).
- Feature Selection: Choosing the most relevant features to improve model performance and reduce complexity.
- Solution: Use techniques like Lasso regression, Random Forest feature importance, or recursive feature elimination.
- Handling Missing Data: Dealing with incomplete or missing values in the dataset.
- Solution: Use imputation techniques, or algorithms that can handle missing values (e.g., Random Forests).
Considerations:
- Model Interpretability: Some models (e.g., linear regression, decision trees) are more interpretable than others (e.g., neural networks, random forests).
- Consider using techniques like SHAP (SHapley Additive exPlanations) values for complex models.
- Computational Resources: More complex models may require significant computational power and time to train.
- Consider the trade-off between model complexity and available resources.
- Data Quality and Quantity: The performance of machine learning models heavily depends on the quality and quantity of available data.
- Invest time in data cleaning and collection to improve model performance.
- Ethical Considerations: Be aware of potential biases in your data and models, especially when dealing with sensitive information or making important decisions.
- Regularly audit your models for fairness and bias.
Future Trends and Advanced Techniques
As the field of machine learning continues to evolve, new trends and advanced techniques are emerging that blur the lines between regression and classification or offer novel approaches to these fundamental tasks:
- Transfer Learning: Using pre-trained models on large datasets and fine-tuning them for specific tasks, reducing the need for large amounts of labeled data.
from tensorflow.keras.applications import ResNet50 from tensorflow.keras.layers import Dense, GlobalAveragePooling2D from tensorflow.keras.models import Model base_model = ResNet50(weights='imagenet', include_top=False) x = base_model.output x = GlobalAveragePooling2D()(x) x = Dense(1024, activation='relu')(x) output = Dense(num_classes, activation='softmax')(x) model = Model(inputs=base_model.input, outputs=output) # Freeze base model layers for layer in base_model.layers: layer.trainable = False model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
- Automated Machine Learning (AutoML): Automating the process of model selection, hyperparameter tuning, and feature engineering.
from autosklearn.classification import AutoSklearnClassifier automl = AutoSklearnClassifier(time_left_for_this_task=3600, per_run_time_limit=300) automl.fit(X_train, y_train) y_pred = automl.predict(X_test)
- Multi-task Learning: Training models to perform multiple related tasks simultaneously, improving generalization and efficiency.
from tensorflow.keras.layers import Input, Dense, Flatten from tensorflow.keras.models import Model input_layer = Input(shape=(input_shape,)) shared_layer = Dense(64, activation='relu')(input_layer) shared_layer = Dense(32, activation='relu')(shared_layer) task1_output = Dense(1, activation='linear', name='regression_output')(shared_layer) task2_output = Dense(num_classes, activation='softmax', name='classification_output')(shared_layer) model = Model(inputs=input_layer, outputs=[task1_output, task2_output]) model.compile(optimizer='adam', loss={'regression_output': 'mse', 'classification_output': 'categorical_crossentropy'}, loss_weights={'regression_output': 1.0, 'classification_output': 1.0}, metrics={'regression_output': 'mae', 'classification_output': 'accuracy'})
- Explainable AI (XAI): Developing techniques to make complex models more interpretable and transparent.
import shap explainer = shap.TreeExplainer(model) shap_values = explainer.shap_values(X) shap.summary_plot(shap_values, X)
- Federated Learning: Training models on distributed datasets without centralizing the data, addressing privacy concerns.
import tensorflow_federated as tff # Define a simple model def create_keras_model(): return tf.keras.models.Sequential([ tf.keras.layers.Input(shape=(784,)), tf.keras.layers.Dense(10, activation='softmax') ]) # Wrap the model in TFF def model_fn(): keras_model = create_keras_model() return tff.learning.from_keras_model( keras_model, input_spec=preprocessed_example_dataset.element_spec, loss=tf.keras.losses.SparseCategoricalCrossentropy(), metrics=[tf.keras.metrics.SparseCategoricalAccuracy()] ) # Create a federated averaging process iterative_process = tff.learning.build_federated_averaging_process(model_fn)
Conclusion
Understanding the differences between regression and classification is crucial for any aspiring data scientist or machine learning practitioner. These two fundamental techniques form the backbone of many predictive modeling tasks and are essential knowledge for technical interviews at major tech companies.
To recap the key points:
- Regression predicts continuous numerical values, while classification predicts discrete categorical labels.
- The choice between regression and classification depends on the nature of your problem and the type of output you’re trying to predict.
- Both techniques have a wide array of algorithms available, each with its strengths and weaknesses.
- Proper evaluation metrics and careful consideration of challenges like overfitting and data quality are crucial for building effective models.
- Emerging trends like transfer learning, AutoML, and explainable AI are pushing the boundaries of what’s possible with regression and classification tasks.
As you continue your journey in machine learning and data science, remember that mastering these fundamental concepts will provide a solid foundation for tackling more complex problems and staying at the forefront of the field. Keep practicing, experimenting with different algorithms, and staying updated with the latest advancements to excel in your coding education and future technical interviews.