Recursive vs. Iterative Algorithms: Pros and Cons
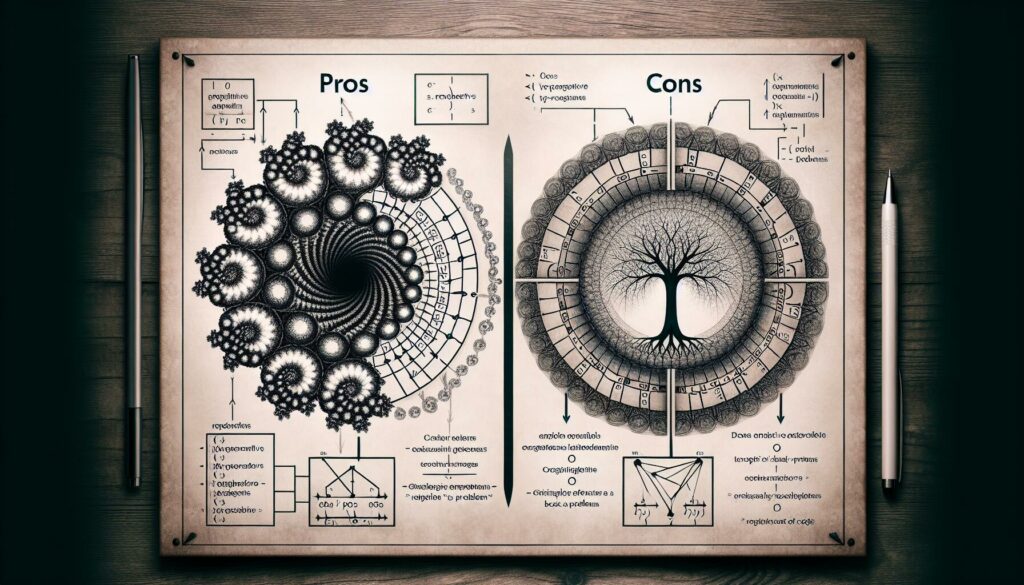
In the world of programming and algorithm design, two fundamental approaches stand out: recursive and iterative algorithms. Both methods aim to solve problems and implement solutions, but they do so in distinctly different ways. Understanding the pros and cons of each approach is crucial for developers, especially those preparing for technical interviews at major tech companies. In this comprehensive guide, we’ll dive deep into recursive and iterative algorithms, exploring their strengths, weaknesses, and best use cases.
Understanding Recursive Algorithms
Recursive algorithms are based on the concept of recursion, where a function calls itself to solve a problem by breaking it down into smaller, more manageable subproblems. This approach follows the divide-and-conquer paradigm and often results in elegant and concise code.
How Recursive Algorithms Work
A recursive algorithm typically consists of two main components:
- Base case: This is the simplest form of the problem that can be solved directly without further recursion.
- Recursive case: This is where the function calls itself with a simpler or smaller input, gradually working towards the base case.
Let’s look at a classic example of a recursive algorithm: calculating the factorial of a number.
def factorial(n):
if n == 0 or n == 1: # Base case
return 1
else: # Recursive case
return n * factorial(n - 1)
In this example, the base case is when n is 0 or 1, and the recursive case multiplies n by the factorial of (n-1).
Pros of Recursive Algorithms
- Simplicity and Elegance: Recursive solutions often mirror the problem’s natural structure, making them easier to understand and implement for certain types of problems.
- Code Conciseness: Recursive algorithms can often be expressed in fewer lines of code compared to their iterative counterparts.
- Solving Complex Problems: Some problems, particularly those involving tree-like or hierarchical structures, are more intuitively solved using recursion.
- Divide and Conquer: Recursion naturally implements the divide-and-conquer strategy, which is efficient for many algorithmic problems.
Cons of Recursive Algorithms
- Stack Overflow Risk: Deep recursion can lead to stack overflow errors, especially for large inputs.
- Memory Overhead: Each recursive call adds a new layer to the call stack, potentially consuming more memory than an iterative solution.
- Performance Overhead: The overhead of function calls and stack management can make recursive solutions slower for some problems.
- Difficulty in Debugging: Tracing through recursive calls can be more challenging when debugging complex problems.
Understanding Iterative Algorithms
Iterative algorithms solve problems through repetition of a set of instructions. They typically use loops (such as for or while loops) to achieve the desired outcome without calling themselves.
How Iterative Algorithms Work
An iterative algorithm follows these general steps:
- Initialize variables
- Set up a loop with a condition
- Update variables in each iteration
- Check the condition and repeat or exit
Let’s rewrite the factorial example using an iterative approach:
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
This iterative version uses a loop to multiply numbers from 1 to n, accumulating the result.
Pros of Iterative Algorithms
- Memory Efficiency: Iterative algorithms generally use less memory as they don’t rely on the call stack for each step.
- Better Performance: For many problems, iterative solutions can be faster due to less function call overhead.
- Easier Debugging: Iterative code is often easier to step through and debug.
- No Stack Overflow Risk: Iterative algorithms are not susceptible to stack overflow errors for large inputs.
Cons of Iterative Algorithms
- Code Complexity: For some problems, especially those with tree-like structures, iterative solutions can be more complex and harder to understand.
- Less Intuitive for Certain Problems: Some naturally recursive problems may be less intuitive when expressed iteratively.
- Potential for Off-by-One Errors: Iterative solutions, especially those involving loops, can be prone to off-by-one errors if not carefully implemented.
- State Management: In complex scenarios, managing state across iterations can become challenging and error-prone.
Comparing Recursive and Iterative Approaches
To better understand the differences between recursive and iterative approaches, let’s compare them across several key factors:
1. Problem-Solving Approach
Recursive: Breaks down the problem into smaller subproblems of the same type.
Iterative: Solves the problem through repeated execution of a set of instructions.
2. Code Readability
Recursive: Often more concise and can be more intuitive for problems that have a natural recursive structure.
Iterative: Generally more straightforward for simple problems but can become complex for problems with nested structures.
3. Performance
Recursive: Can be slower due to function call overhead and potential for redundant calculations (if not optimized).
Iterative: Often faster, especially for problems where the recursive solution would involve many repeated calculations.
4. Memory Usage
Recursive: Uses more memory due to the call stack, which can be a limitation for deep recursions.
Iterative: Generally more memory-efficient as it doesn’t rely on the call stack for each step.
5. Debugging
Recursive: Can be more challenging to debug due to the nature of nested function calls.
Iterative: Often easier to debug as the state changes are more visible and sequential.
When to Use Recursive vs. Iterative Algorithms
Choosing between recursive and iterative approaches depends on various factors. Here are some guidelines to help you decide:
Use Recursive Algorithms When:
- The problem has a natural recursive structure: For example, tree traversals, graph traversals, or divide-and-conquer algorithms like QuickSort or MergeSort.
- The solution in recursive form is simpler or more intuitive: Sometimes, a recursive solution can be much clearer and easier to understand.
- The depth of recursion is known and manageable: If you can guarantee that the recursion won’t go too deep, avoiding stack overflow issues.
- Memory usage is not a primary concern: In scenarios where the additional memory usage of the call stack is acceptable.
Use Iterative Algorithms When:
- Optimizing for performance: When the recursive solution would involve many redundant calculations or function call overheads.
- Dealing with large inputs: To avoid potential stack overflow errors that might occur with deep recursion.
- Memory efficiency is crucial: In memory-constrained environments or when dealing with large datasets.
- The problem is naturally iterative: For simple loops or when the solution doesn’t benefit from a recursive structure.
Optimization Techniques
Both recursive and iterative algorithms can be optimized to improve their performance and efficiency. Here are some techniques:
Optimizing Recursive Algorithms
- Tail Recursion: Restructuring the recursive call to be the last operation in the function, allowing some compilers to optimize it into an iterative form.
- Memoization: Storing the results of expensive function calls and returning the cached result when the same inputs occur again.
- Recursion with Accumulator: Passing an accumulator parameter to avoid redundant calculations in each recursive call.
Example of tail recursion for factorial calculation:
def factorial_tail(n, accumulator=1):
if n == 0 or n == 1:
return accumulator
else:
return factorial_tail(n - 1, n * accumulator)
Optimizing Iterative Algorithms
- Loop Unrolling: Reducing the number of iterations by performing multiple operations in a single iteration.
- Efficient Data Structures: Using appropriate data structures to minimize the time complexity of operations within the loop.
- Early Termination: Adding conditions to exit the loop early when the desired result is achieved.
Example of loop unrolling for summing an array:
def sum_array_unrolled(arr):
sum = 0
n = len(arr)
for i in range(0, n - 1, 2):
sum += arr[i] + arr[i + 1]
if n % 2 != 0:
sum += arr[-1]
return sum
Case Studies: Recursive vs. Iterative Implementations
Let’s examine a few classic problems and compare their recursive and iterative implementations:
1. Fibonacci Sequence
Recursive Implementation:
def fibonacci_recursive(n):
if n
Iterative Implementation:
def fibonacci_iterative(n):
if n
In this case, the iterative version is significantly more efficient, especially for larger values of n, as it avoids the exponential number of redundant calculations in the naive recursive approach.
2. Binary Search
Recursive Implementation:
def binary_search_recursive(arr, target, low, high):
if low > high:
return -1
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] > target:
return binary_search_recursive(arr, target, low, mid - 1)
else:
return binary_search_recursive(arr, target, mid + 1, high)
Iterative Implementation:
def binary_search_iterative(arr, target):
low, high = 0, len(arr) - 1
while low target:
high = mid - 1
else:
low = mid + 1
return -1
Both implementations have similar time complexity (O(log n)), but the iterative version may be slightly more efficient due to less function call overhead.
3. Tree Traversal (Inorder)
Recursive Implementation:
def inorder_recursive(root):
if root:
inorder_recursive(root.left)
print(root.val)
inorder_recursive(root.right)
Iterative Implementation:
def inorder_iterative(root):
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
print(current.val)
current = current.right
The recursive version is more intuitive and easier to understand, while the iterative version requires explicit stack management but may be more efficient for very deep trees.
Impact on Technical Interviews
Understanding both recursive and iterative approaches is crucial for technical interviews, especially for positions at major tech companies. Here’s why:
- Problem-Solving Flexibility: Interviewers often look for candidates who can approach problems from multiple angles. Being comfortable with both recursive and iterative solutions demonstrates versatility.
- Optimization Skills: You may be asked to optimize a solution, which could involve converting a recursive solution to an iterative one (or vice versa) for better performance or memory usage.
- Understanding Trade-offs: Being able to discuss the pros and cons of each approach shows depth of knowledge and critical thinking skills.
- Code Quality: Writing clean, efficient code in both styles can impress interviewers and showcase your coding abilities.
- Handling Edge Cases: Both approaches have different considerations for edge cases (e.g., stack overflow in recursion), and understanding these can be important.
Conclusion
Both recursive and iterative algorithms have their place in a programmer’s toolkit. Recursive algorithms often provide elegant solutions to problems with natural recursive structures, while iterative algorithms can offer better performance and memory efficiency for many scenarios.
As you prepare for technical interviews or work on real-world projects, practice implementing solutions using both approaches. This will not only improve your problem-solving skills but also help you make informed decisions about which method to use based on the specific requirements of each problem.
Remember, the choice between recursive and iterative approaches isn’t always clear-cut. It depends on factors like the nature of the problem, performance requirements, memory constraints, and even personal or team coding preferences. By mastering both techniques, you’ll be well-equipped to tackle a wide range of algorithmic challenges and excel in your programming career.