Recursion Demystified: A Comprehensive Guide to Understanding and Mastering Recursive Programming
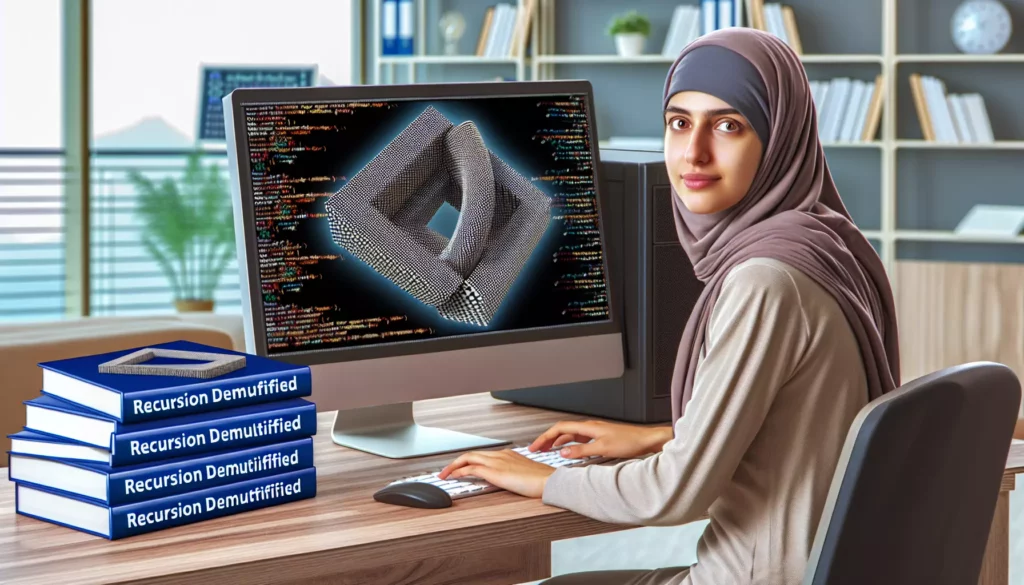
Recursion is a fundamental concept in computer science and programming that often challenges beginners and experienced developers alike. In this comprehensive guide, we’ll dive deep into the world of recursion, exploring its definition, applications, and practical implementations. By the end of this article, you’ll have a solid understanding of recursion and be equipped to tackle recursive problems with confidence.
Table of Contents
- What is Recursion?
- How Recursion Works
- Key Components of Recursion
- Advantages and Disadvantages of Recursion
- Common Recursive Problems
- Recursion vs. Iteration
- Debugging Recursive Functions
- Optimization Techniques for Recursive Algorithms
- Real-World Applications of Recursion
- Tips for Mastering Recursion
- Conclusion
1. What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem by breaking it down into smaller, more manageable subproblems. In essence, a recursive function solves a problem by solving smaller instances of the same problem.
To understand recursion, it’s often helpful to think of it as a process of “divide and conquer.” The function divides the problem into smaller subproblems, solves them, and then combines the results to solve the original problem.
2. How Recursion Works
To illustrate how recursion works, let’s consider a simple example: calculating the factorial of a number. The factorial of a non-negative integer n, denoted as n!, is the product of all positive integers from 1 to n.
Here’s a recursive implementation of the factorial function in Python:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
Let’s break down how this recursive function works:
- The function first checks if n is 0 or 1. If so, it returns 1 (base case).
- If n is greater than 1, the function returns n multiplied by the factorial of (n – 1).
- This process continues, with each recursive call reducing n by 1, until it reaches the base case.
- Once the base case is reached, the function starts returning values and combining them to compute the final result.
For example, if we call factorial(5), the function will make the following recursive calls:
factorial(5) = 5 * factorial(4)
factorial(4) = 4 * factorial(3)
factorial(3) = 3 * factorial(2)
factorial(2) = 2 * factorial(1)
factorial(1) = 1 (base case)
Then, the function will combine these results:
factorial(1) = 1
factorial(2) = 2 * 1 = 2
factorial(3) = 3 * 2 = 6
factorial(4) = 4 * 6 = 24
factorial(5) = 5 * 24 = 120
Thus, the final result of factorial(5) is 120.
3. Key Components of Recursion
To implement a recursive function successfully, you need to understand and include the following key components:
Base Case
The base case is the condition that stops the recursion. It’s a simple case where the function can return a result without making any further recursive calls. In our factorial example, the base case is when n is 0 or 1.
Recursive Case
The recursive case is where the function calls itself with a modified input. This step breaks down the problem into a smaller subproblem. In the factorial example, the recursive case is n * factorial(n – 1).
Progress Towards the Base Case
Each recursive call should move the problem closer to the base case. This ensures that the recursion will eventually terminate. In our example, we’re reducing n by 1 in each recursive call, moving towards the base case of n = 0 or n = 1.
4. Advantages and Disadvantages of Recursion
Advantages
- Elegant and concise code for problems with recursive structure
- Can simplify complex problems by breaking them down into smaller, more manageable subproblems
- Often leads to more readable and maintainable code for certain types of problems
- Naturally suited for problems involving tree-like or hierarchical data structures
Disadvantages
- Can be less efficient than iterative solutions due to function call overhead
- Risk of stack overflow errors for deep recursion
- May be harder to understand and debug for beginners
- Can lead to excessive memory usage if not optimized
5. Common Recursive Problems
Many classic programming problems lend themselves well to recursive solutions. Here are some common examples:
Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. Here’s a recursive implementation:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n - 1) + fibonacci(n - 2)
Binary Search
Binary search is an efficient algorithm for searching a sorted array. Here’s a recursive implementation:
def binary_search(arr, target, low, high):
if low > high:
return -1
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] > target:
return binary_search(arr, target, low, mid - 1)
else:
return binary_search(arr, target, mid + 1, high)
Tree Traversal
Recursion is particularly useful for traversing tree-like data structures. Here’s an example of in-order traversal of a binary tree:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.val)
inorder_traversal(root.right)
6. Recursion vs. Iteration
While recursion and iteration can often solve the same problems, they have different characteristics and trade-offs:
Recursion
- Often leads to more elegant and concise code for problems with recursive structure
- Can be easier to understand for problems that are naturally recursive
- May have higher memory usage due to the call stack
- Can lead to stack overflow errors for deep recursion
Iteration
- Generally more efficient in terms of memory usage and execution time
- Avoids the risk of stack overflow errors
- Can be more straightforward for simple problems
- May lead to more complex code for problems with recursive structure
Here’s an example of the factorial function implemented both recursively and iteratively:
# Recursive implementation
def factorial_recursive(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial_recursive(n - 1)
# Iterative implementation
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
Both implementations produce the same result, but the iterative version may be more efficient for large inputs.
7. Debugging Recursive Functions
Debugging recursive functions can be challenging due to their self-referential nature. Here are some tips to help you debug recursive functions effectively:
- Use print statements: Add print statements at key points in your function to track the flow of execution and the values of important variables.
- Visualize the call stack: Use tools like Python Tutor (pythontutor.com) to visualize the call stack and see how the recursive calls unfold.
- Start with small inputs: Begin by testing your function with small, simple inputs to ensure it works correctly before moving on to more complex cases.
- Check base cases: Ensure that your base cases are correct and that the function returns the expected values for these simple cases.
- Trace the recursion: Manually trace through the recursive calls for small inputs to understand how the function behaves.
- Use a debugger: Utilize your IDE’s debugger to step through the function execution and examine variable values at each step.
8. Optimization Techniques for Recursive Algorithms
While recursive solutions can be elegant, they may sometimes suffer from performance issues. Here are some techniques to optimize recursive algorithms:
Tail Recursion
Tail recursion is a form of recursion where the recursive call is the last operation in the function. Some programming languages and compilers can optimize tail-recursive functions to use constant stack space. Here’s an example of tail-recursive factorial function:
def factorial_tail(n, accumulator=1):
if n == 0 or n == 1:
return accumulator
else:
return factorial_tail(n - 1, n * accumulator)
Memoization
Memoization is a technique where you cache the results of expensive function calls and return the cached result when the same inputs occur again. This can significantly improve the performance of recursive functions that compute the same subproblems multiple times. Here’s an example of memoized Fibonacci function:
def fibonacci_memo(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_memo(n - 1, memo) + fibonacci_memo(n - 2, memo)
return memo[n]
Dynamic Programming
Dynamic programming is an optimization technique that solves complex problems by breaking them down into simpler subproblems. It’s often used to optimize recursive algorithms by storing the results of subproblems and reusing them. Here’s an example of computing Fibonacci numbers using dynamic programming:
def fibonacci_dp(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i - 1] + dp[i - 2]
return dp[n]
9. Real-World Applications of Recursion
Recursion is not just a theoretical concept; it has many practical applications in real-world software development. Here are some areas where recursion is commonly used:
File System Operations
Recursion is often used to traverse directory structures, search for files, or perform operations on nested folders. Here’s an example of a recursive function that lists all files in a directory and its subdirectories:
import os
def list_files(directory):
for root, dirs, files in os.walk(directory):
for file in files:
print(os.path.join(root, file))
for dir in dirs:
list_files(os.path.join(directory, dir))
Parsing and Processing Data Structures
Recursion is useful for parsing and processing hierarchical data structures like XML, JSON, or abstract syntax trees. Here’s a simple example of parsing a nested dictionary:
def parse_nested_dict(data, prefix=''):
for key, value in data.items():
if isinstance(value, dict):
parse_nested_dict(value, prefix + key + '.')
else:
print(f"{prefix}{key}: {value}")
Graph Algorithms
Many graph algorithms, such as depth-first search (DFS) and flood fill, are naturally recursive. Here’s an example of a recursive DFS implementation:
def dfs(graph, node, visited=None):
if visited is None:
visited = set()
visited.add(node)
print(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
Divide and Conquer Algorithms
Recursion is at the heart of many divide and conquer algorithms, such as quicksort and merge sort. Here’s a recursive implementation of quicksort:
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
10. Tips for Mastering Recursion
Mastering recursion takes practice and patience. Here are some tips to help you become proficient in recursive programming:
- Start with simple problems: Begin with basic recursive problems like factorial or Fibonacci and gradually move to more complex ones.
- Identify the base case: Always start by identifying and implementing the base case(s) that will terminate the recursion.
- Trust the recursion: When writing recursive functions, assume that the recursive calls will work correctly and focus on solving the current subproblem.
- Visualize the call stack: Draw or visualize the call stack to understand how the recursive calls unfold and how the results are combined.
- Practice, practice, practice: Solve a variety of recursive problems to build your intuition and problem-solving skills.
- Analyze time and space complexity: Understand the time and space complexity of your recursive solutions and learn to optimize them when necessary.
- Compare with iterative solutions: Implement both recursive and iterative solutions for the same problem to understand the trade-offs between the two approaches.
- Learn common recursive patterns: Familiarize yourself with common recursive patterns like divide and conquer, backtracking, and dynamic programming.
- Use recursion thoughtfully: While recursion can lead to elegant solutions, it’s not always the best choice. Learn when to use recursion and when to opt for iterative approaches.
- Explore tail recursion: Understand tail recursion and how it can be optimized in certain programming languages.
11. Conclusion
Recursion is a powerful and elegant programming technique that allows you to solve complex problems by breaking them down into simpler subproblems. While it may seem challenging at first, with practice and understanding, you can master recursive thinking and apply it effectively in your programming projects.
Remember that recursion is not just a theoretical concept but a practical tool used in many real-world applications, from file system operations to advanced algorithms. By understanding the core principles of recursion, its advantages and disadvantages, and common optimization techniques, you’ll be well-equipped to tackle a wide range of programming challenges.
As you continue your journey in programming and computer science, don’t shy away from recursive problems. Embrace them as opportunities to strengthen your problem-solving skills and deepen your understanding of algorithmic thinking. With time and practice, you’ll find that recursion becomes a natural and powerful tool in your programming toolkit.
Keep exploring, keep practicing, and most importantly, enjoy the process of unraveling the elegant simplicity of recursive solutions!