Real-World Applications of Graph Algorithms: Powering Modern Technology
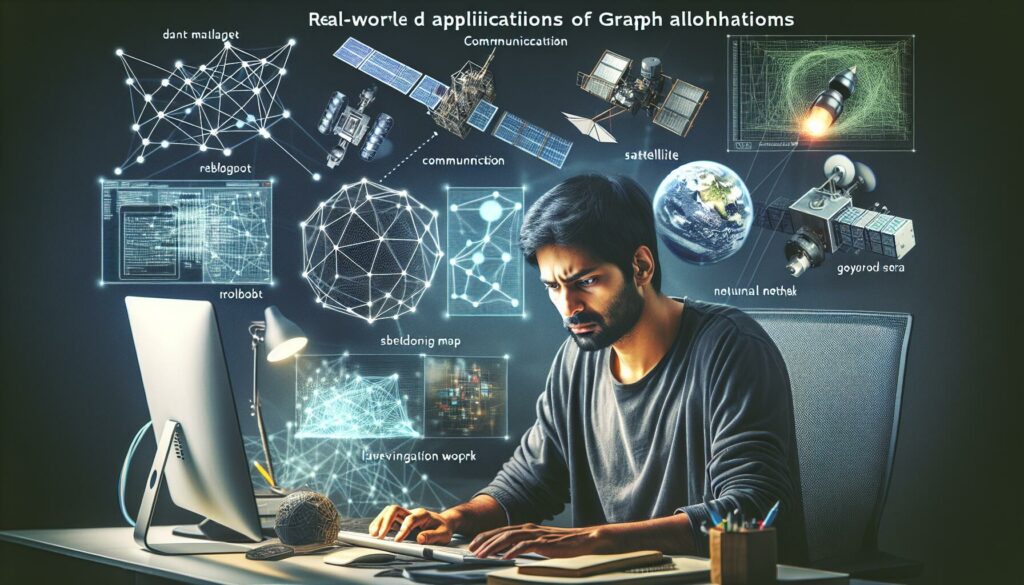
In the ever-evolving landscape of computer science and technology, graph algorithms stand out as powerful tools that solve complex problems across various domains. These algorithms, which operate on graph data structures, have found their way into numerous real-world applications, revolutionizing how we approach challenges in fields ranging from social networks to transportation systems. In this comprehensive guide, we’ll explore the fascinating world of graph algorithms and their practical implementations that shape our daily lives.
Understanding Graph Algorithms
Before diving into the applications, let’s briefly recap what graph algorithms are and why they’re so important in computer science.
What are Graph Algorithms?
Graph algorithms are a set of instructions designed to perform operations on graphs. A graph is a data structure consisting of nodes (or vertices) connected by edges. These algorithms help us analyze the relationships and connections within the graph, find optimal paths, detect patterns, and solve various computational problems efficiently.
Types of Graph Algorithms
There are several types of graph algorithms, each suited for different tasks:
- Traversal algorithms (e.g., Depth-First Search, Breadth-First Search)
- Shortest path algorithms (e.g., Dijkstra’s algorithm, Bellman-Ford algorithm)
- Minimum spanning tree algorithms (e.g., Kruskal’s algorithm, Prim’s algorithm)
- Flow algorithms (e.g., Ford-Fulkerson algorithm)
- Connectivity algorithms (e.g., Tarjan’s algorithm)
- Cycle detection algorithms
- Graph coloring algorithms
Real-World Applications of Graph Algorithms
Now, let’s explore how these algorithms are applied in various industries and technologies.
1. Social Network Analysis
Social media platforms like Facebook, LinkedIn, and Twitter heavily rely on graph algorithms to analyze user connections, recommend friends, and detect communities.
Friend Recommendations
Graph algorithms help identify mutual friends and suggest new connections based on the existing network structure. For example, Facebook might use a variation of the following algorithm to recommend friends:
function recommendFriends(user):
friends = getFriendList(user)
recommendations = new Set()
for friend in friends:
friendOfFriends = getFriendList(friend)
for potentialFriend in friendOfFriends:
if potentialFriend not in friends and potentialFriend != user:
recommendations.add(potentialFriend)
return sortByCommonConnections(recommendations)
Influence Measurement
Algorithms like PageRank (originally developed for web page ranking) are adapted to measure user influence in social networks. This helps identify key opinion leaders and influential users for marketing purposes.
2. Navigation and Mapping Services
Companies like Google Maps and Waze use graph algorithms extensively for route planning and traffic optimization.
Shortest Path Calculation
Dijkstra’s algorithm or its variants are commonly used to find the shortest or fastest route between two points. Here’s a simplified implementation of Dijkstra’s algorithm:
function dijkstra(graph, start, end):
distances = {node: float('inf') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
currentDist, currentNode = heapq.heappop(pq)
if currentNode == end:
return currentDist
if currentDist > distances[currentNode]:
continue
for neighbor, weight in graph[currentNode].items():
distance = currentDist + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return float('inf') # No path found
Traffic Optimization
Graph algorithms help in analyzing traffic patterns and suggesting alternative routes to avoid congestion. This involves real-time updates to the graph’s edge weights based on current traffic conditions.
3. Recommendation Systems
E-commerce platforms like Amazon and streaming services like Netflix use graph-based recommendation systems to suggest products or content to users.
Collaborative Filtering
Graph algorithms can be used to implement collaborative filtering, where recommendations are made based on the preferences of similar users. This can be represented as a bipartite graph between users and items.
Content-Based Recommendations
By modeling items and their attributes as a graph, content-based recommendation systems can suggest similar items based on shared characteristics.
4. Network Optimization
Telecommunication companies and internet service providers use graph algorithms to optimize network performance and reliability.
Network Flow Optimization
The Ford-Fulkerson algorithm or its variants are used to maximize the flow of data through a network. Here’s a basic implementation:
def ford_fulkerson(graph, source, sink):
def bfs(graph, source, sink, parent):
visited = [False] * len(graph)
queue = [source]
visited[source] = True
while queue:
u = queue.pop(0)
for ind, val in enumerate(graph[u]):
if visited[ind] == False and val > 0:
queue.append(ind)
visited[ind] = True
parent[ind] = u
if ind == sink:
return True
return False
parent = [-1] * len(graph)
max_flow = 0
while bfs(graph, source, sink, parent):
path_flow = float("Inf")
s = sink
while(s != source):
path_flow = min(path_flow, graph[parent[s]][s])
s = parent[s]
max_flow += path_flow
v = sink
while(v != source):
u = parent[v]
graph[u][v] -= path_flow
graph[v][u] += path_flow
v = parent[v]
return max_flow
Fault Tolerance
Graph algorithms help in identifying critical nodes and edges in a network, allowing for better planning of redundancy and fault tolerance measures.
5. Bioinformatics and Genomics
Graph algorithms play a crucial role in analyzing biological data, particularly in genomics and protein interaction networks.
Sequence Alignment
Graph-based algorithms are used for DNA and protein sequence alignment, which is crucial for understanding genetic similarities and differences between organisms.
Protein Interaction Networks
Researchers use graph algorithms to analyze protein-protein interaction networks, helping to understand cellular processes and identify potential drug targets.
6. Financial Systems
The financial sector leverages graph algorithms for various purposes, from fraud detection to risk assessment.
Fraud Detection
Graph algorithms can identify suspicious patterns in transaction networks, helping to detect potential fraud. For example, cycle detection algorithms can spot money laundering schemes.
Credit Scoring
By representing financial relationships as a graph, algorithms can assess creditworthiness by analyzing connections and transaction patterns.
7. Supply Chain Management
Graph algorithms optimize logistics and supply chain operations for companies dealing with complex distribution networks.
Inventory Routing
Minimum spanning tree algorithms like Kruskal’s or Prim’s can be used to optimize delivery routes and minimize transportation costs. Here’s a simple implementation of Kruskal’s algorithm:
class DisjointSet:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
def kruskal_mst(graph):
edges = [(w, u, v) for u in graph for v, w in graph[u].items()]
edges.sort()
vertices = list(graph.keys())
ds = DisjointSet(vertices)
mst = []
for w, u, v in edges:
if ds.find(u) != ds.find(v):
ds.union(u, v)
mst.append((u, v, w))
return mst
Supply Network Analysis
Graph algorithms help in identifying critical suppliers, bottlenecks, and vulnerabilities in the supply chain network.
8. Urban Planning and Smart Cities
Graph algorithms contribute to the development of smart cities and efficient urban planning.
Public Transportation Optimization
Algorithms like the Chinese Postman Problem (for route inspection) are used to design efficient bus routes and optimize public transportation networks.
Utility Network Management
Graph algorithms help in managing and optimizing water, electricity, and gas distribution networks in urban areas.
9. Computer Networking
The internet itself relies heavily on graph algorithms for efficient data routing and network management.
Routing Protocols
Algorithms like Dijkstra’s and Bellman-Ford are the foundation of routing protocols such as OSPF (Open Shortest Path First) and BGP (Border Gateway Protocol).
Network Topology Analysis
Graph algorithms help in analyzing and optimizing network topologies, ensuring efficient data transmission and network resilience.
10. Artificial Intelligence and Machine Learning
Graph algorithms are increasingly important in AI and ML, particularly in areas like knowledge representation and reasoning.
Knowledge Graphs
Companies like Google use knowledge graphs to enhance search results and provide more contextual information. Graph algorithms are crucial for querying and reasoning over these knowledge structures.
Graph Neural Networks
A growing field in machine learning, Graph Neural Networks (GNNs) use graph structures to process data, finding applications in areas like molecular property prediction and social influence prediction.
Implementing Graph Algorithms: Best Practices and Considerations
When implementing graph algorithms for real-world applications, several factors need to be considered:
Scalability
Real-world graphs can be enormous, containing millions or billions of nodes and edges. Implementations must be scalable, often requiring distributed computing techniques.
Performance Optimization
Efficient data structures (like adjacency lists or matrices) and algorithm optimizations are crucial for handling large-scale graphs.
Dynamic Graphs
Many real-world graphs change over time. Algorithms and data structures that can handle dynamic updates efficiently are often necessary.
Data Quality and Preprocessing
Real-world data is often noisy or incomplete. Proper data cleaning and preprocessing are essential for accurate results.
Algorithm Selection
Choosing the right algorithm for the specific problem and graph characteristics is crucial. Sometimes, approximation algorithms or heuristics may be preferred for large-scale problems.
Conclusion
Graph algorithms are the unsung heroes powering many of the technologies we rely on daily. From the social media platforms that connect us to the navigation systems that guide us, these algorithms work behind the scenes to solve complex problems efficiently. As we continue to generate and collect more interconnected data, the importance of graph algorithms in tackling real-world challenges will only grow.
For aspiring programmers and computer scientists, mastering graph algorithms is not just an academic exercise but a gateway to solving some of the most interesting and impactful problems in technology. Whether you’re interested in social network analysis, optimizing transportation systems, or pushing the boundaries of artificial intelligence, a solid understanding of graph algorithms will be an invaluable asset in your toolkit.
As we’ve seen through the various applications discussed, the versatility of graph algorithms makes them applicable across a wide range of industries and problem domains. By continuing to innovate and adapt these algorithms to new challenges, we can look forward to even more efficient, intelligent, and connected systems in the future.
Remember, the key to mastering graph algorithms lies not just in understanding their theoretical foundations, but in practicing their implementation and application to real-world problems. So, grab your favorite programming language, start coding, and explore the fascinating world of graphs and their algorithms. Who knows? Your next project might just be the next big application of graph algorithms that changes the world!