Python vs C++ for Coding Interviews: Which is Better?
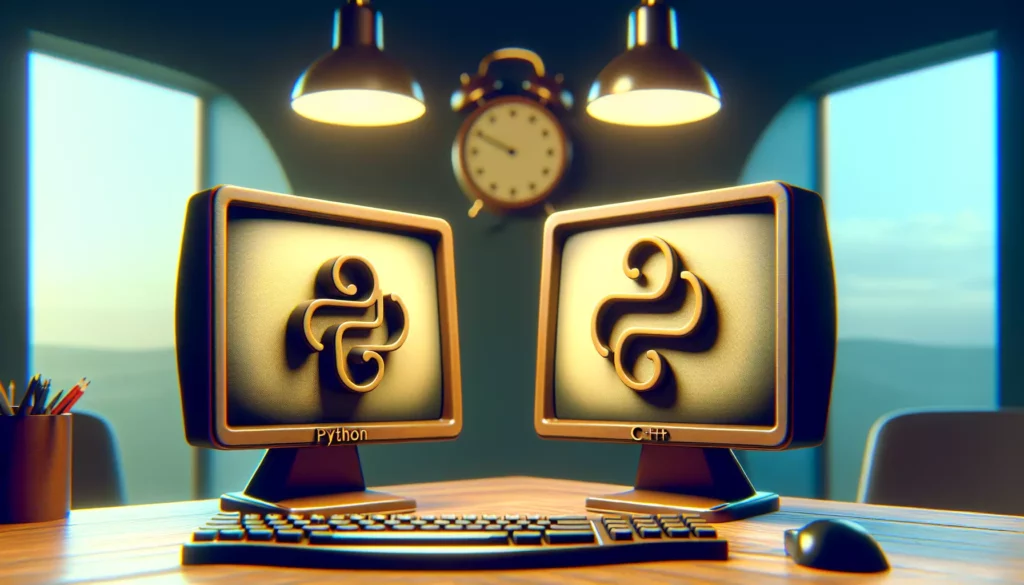
When preparing for coding interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), one of the most common dilemmas faced by candidates is choosing the right programming language. Two popular contenders in this arena are Python and C++. Both languages have their strengths and weaknesses, and the choice between them can significantly impact your interview performance and overall preparation strategy. In this comprehensive guide, we’ll dive deep into the comparison between Python and C++ for coding interviews, helping you make an informed decision that aligns with your skills and interview goals.
Understanding the Importance of Language Choice in Coding Interviews
Before we delve into the specifics of Python and C++, it’s crucial to understand why the choice of programming language matters in coding interviews. While it’s true that most companies allow candidates to use their preferred language, your choice can affect several aspects of your interview experience:
- Coding speed and efficiency
- Ability to implement complex algorithms
- Readability and clarity of your code
- Time management during the interview
- Confidence in solving problems
With these factors in mind, let’s explore how Python and C++ stack up against each other in the context of coding interviews.
Python: The Rising Star in Coding Interviews
Python has gained immense popularity in recent years, not just in the industry but also in coding interviews. Let’s examine the reasons behind its rise and the advantages it offers to interview candidates.
Advantages of Python in Coding Interviews
- Simplicity and Readability: Python’s clean and straightforward syntax makes it easier to write and read code quickly. This is particularly advantageous in high-pressure interview situations where clarity is crucial.
- Rapid Prototyping: Python allows for quick implementation of ideas, which is invaluable when you need to solve problems under time constraints.
- Rich Standard Library: Python’s extensive standard library provides built-in functions and data structures that can save time during interviews.
- Dynamic Typing: No need to declare variable types, which can speed up the coding process.
- Shorter Code: Python often requires fewer lines of code to implement solutions compared to C++, which can be beneficial in timed interviews.
Example: Implementing a Simple Algorithm in Python
Let’s look at how Python’s simplicity shines in implementing a basic algorithm. Here’s an example of a function to find the nth Fibonacci number:
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
# Example usage
print(fibonacci(10)) # Output: 55
As you can see, the implementation is concise and easy to understand, which can be a significant advantage during interviews.
Drawbacks of Python in Coding Interviews
While Python offers many benefits, it’s not without its drawbacks:
- Performance: Python is generally slower than C++, which might be a concern for very large inputs or time-critical problems.
- Lack of Static Typing: While dynamic typing speeds up coding, it can sometimes lead to runtime errors that might be caught earlier in statically-typed languages.
- Less Control Over Memory Management: Python’s automatic memory management, while convenient, offers less control compared to C++.
C++: The Time-Tested Language for Coding Interviews
C++ has long been a favorite among competitive programmers and remains a strong choice for coding interviews. Let’s explore why many candidates still prefer C++ and what advantages it offers.
Advantages of C++ in Coding Interviews
- Performance: C++ offers superior performance, which can be crucial for solving complex algorithmic problems efficiently.
- Low-Level Control: C++ provides more control over system resources and memory management, which can be beneficial for certain types of problems.
- STL (Standard Template Library): C++’s STL offers a rich set of data structures and algorithms that can be powerful tools in coding interviews.
- Static Typing: This can help catch errors early and potentially save time during the interview.
- Industry Relevance: Many companies, especially those working on system-level software or performance-critical applications, value C++ skills highly.
Example: Implementing the Same Algorithm in C++
Let’s implement the Fibonacci function in C++ for comparison:
#include <iostream>
int fibonacci(int n) {
if (n <= 1)
return n;
return fibonacci(n-1) + fibonacci(n-2);
}
int main() {
std::cout << fibonacci(10) << std::endl; // Output: 55
return 0;
}
While the C++ implementation is slightly more verbose, it offers the potential for better performance, especially for larger values of n.
Drawbacks of C++ in Coding Interviews
C++ also has its share of challenges in the interview context:
- Complexity: C++ has a steeper learning curve and more complex syntax, which can lead to more time spent on implementation details.
- Verbose Syntax: C++ often requires more lines of code to accomplish the same task compared to Python, which can be a disadvantage in time-constrained interviews.
- Manual Memory Management: While offering more control, manual memory management can also be a source of errors if not handled carefully.
- Longer Compilation Time: In interviews where you might need to run and test your code multiple times, C++’s compilation step can slow you down compared to Python’s interpreted nature.
Comparing Python and C++ in Different Aspects of Coding Interviews
To help you make an informed decision, let’s compare Python and C++ across various aspects relevant to coding interviews:
1. Syntax and Readability
Python: Known for its clean, readable syntax. Uses indentation for code blocks, making the structure visually clear.
C++: More verbose syntax with curly braces for code blocks. Can be less immediately readable, especially for complex algorithms.
Winner for Interviews: Python, due to its simplicity and readability, which can be crucial under interview pressure.
2. Speed of Coding
Python: Allows for rapid prototyping and quick implementation of ideas.
C++: Generally requires more setup and boilerplate code, which can slow down the initial coding process.
Winner for Interviews: Python, as it allows candidates to translate their thoughts into code more quickly.
3. Performance
Python: Generally slower execution, especially for computationally intensive tasks.
C++: Offers superior performance and is more efficient for complex algorithms and large datasets.
Winner for Interviews: C++, but this is often less critical in interview settings unless specifically dealing with performance-oriented questions.
4. Built-in Data Structures and Libraries
Python: Rich standard library with easy-to-use data structures like lists, dictionaries, and sets.
C++: Powerful STL offering efficient implementations of various data structures and algorithms.
Winner for Interviews: Tie. Both languages offer robust libraries, with Python’s being slightly easier to use on the fly.
5. Memory Management
Python: Automatic memory management with garbage collection.
C++: Manual memory management, offering more control but requiring more attention to detail.
Winner for Interviews: Python, as it reduces the cognitive load of managing memory during time-pressured interviews.
6. Debugging and Testing
Python: Easier to debug with clear error messages and straightforward testing.
C++: Debugging can be more complex, especially for memory-related issues.
Winner for Interviews: Python, due to its easier debugging process which can save crucial time during interviews.
Specific Interview Scenarios: Python vs C++
Let’s examine how Python and C++ compare in specific types of coding interview questions:
1. String Manipulation
Python Example:
def reverse_words(s):
return " ".join(s.split()[::-1])
print(reverse_words("Hello World")) # Output: "World Hello"
C++ Example:
#include <iostream>
#include <sstream>
#include <vector>
#include <algorithm>
std::string reverse_words(std::string s) {
std::vector<std::string> words;
std::istringstream iss(s);
std::string word;
while (iss >> word) {
words.push_back(word);
}
std::reverse(words.begin(), words.end());
std::string result;
for (const auto& w : words) {
if (!result.empty()) result += " ";
result += w;
}
return result;
}
int main() {
std::cout << reverse_words("Hello World") << std::endl; // Output: "World Hello"
return 0;
}
Analysis: Python’s simplicity shines in string manipulation tasks, often requiring fewer lines of code and leveraging powerful built-in functions.
2. Array/List Operations
Python Example:
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
print(two_sum([2, 7, 11, 15], 9)) # Output: [0, 1]
C++ Example:
#include <iostream>
#include <vector>
#include <unordered_map>
std::vector<int> two_sum(std::vector<int>& nums, int target) {
std::unordered_map<int, int> seen;
for (int i = 0; i < nums.size(); ++i) {
int complement = target - nums[i];
if (seen.find(complement) != seen.end()) {
return {seen[complement], i};
}
seen[nums[i]] = i;
}
return {};
}
int main() {
std::vector<int> nums = {2, 7, 11, 15};
auto result = two_sum(nums, 9);
std::cout << "[" << result[0] << ", " << result[1] << "]" << std::endl; // Output: [0, 1]
return 0;
}
Analysis: Both languages handle this efficiently, but Python’s syntax is more concise. C++ offers type safety and potentially better performance for large arrays.
3. Graph Algorithms
Python Example (BFS):
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
bfs(graph, 'A') # Output: A B C D E F
C++ Example (BFS):
#include <iostream>
#include <queue>
#include <unordered_map>
#include <unordered_set>
#include <vector>
void bfs(const std::unordered_map<char, std::vector<char>>& graph, char start) {
std::unordered_set<char> visited;
std::queue<char> queue;
queue.push(start);
visited.insert(start);
while (!queue.empty()) {
char vertex = queue.front();
queue.pop();
std::cout << vertex << " ";
for (char neighbor : graph.at(vertex)) {
if (visited.find(neighbor) == visited.end()) {
visited.insert(neighbor);
queue.push(neighbor);
}
}
}
}
int main() {
std::unordered_map<char, std::vector<char>> graph = {
{'A', {'B', 'C'}},
{'B', {'A', 'D', 'E'}},
{'C', {'A', 'F'}},
{'D', {'B'}},
{'E', {'B', 'F'}},
{'F', {'C', 'E'}}
};
bfs(graph, 'A'); // Output: A B C D E F
return 0;
}
Analysis: Both implementations are fairly similar, but Python’s version is slightly more concise. C++ might have a slight edge in performance for very large graphs.
Making the Choice: Factors to Consider
When deciding between Python and C++ for your coding interviews, consider the following factors:
- Your Proficiency: Choose the language you’re most comfortable with. Familiarity trumps theoretical advantages.
- Company Preference: Research if the company you’re interviewing with has a preference. Some companies might lean towards one language.
- Problem Type: For algorithmic problems, both languages work well. For system design or low-level programming questions, C++ might be more suitable.
- Time Constraints: If you’re worried about time management, Python’s conciseness can be advantageous.
- Interview Format: For whiteboard coding, Python’s simplicity can be beneficial. For take-home assignments, C++’s performance might be more relevant.
Strategies for Success Regardless of Language Choice
Regardless of whether you choose Python or C++, here are some strategies to excel in coding interviews:
- Focus on Problem-Solving: The core of coding interviews is your problem-solving ability, not language specifics.
- Practice Regularly: Use platforms like AlgoCademy to practice coding problems in your chosen language.
- Understand Data Structures and Algorithms: These fundamentals are crucial regardless of the language you use.
- Communicate Clearly: Explain your thought process as you code. This is often more important than the code itself.
- Time Management: Practice solving problems within time constraints to improve your speed and efficiency.
- Learn from Mistakes: Analyze your errors and learn from them. This iterative process is key to improvement.
Conclusion
In the Python vs C++ debate for coding interviews, there’s no one-size-fits-all answer. Python offers simplicity, readability, and rapid development, making it an excellent choice for many interview scenarios. C++, with its performance benefits and low-level control, remains a strong contender, especially for roles that require system-level programming or high-performance computing.
Ultimately, the best language is the one you’re most proficient in and comfortable using under pressure. Both Python and C++ are widely accepted in coding interviews, and your problem-solving skills, algorithmic knowledge, and ability to communicate your thoughts clearly are far more important than the specific language you choose.
Remember, the goal of coding interviews is not just to write code, but to demonstrate your thinking process, problem-solving approach, and ability to translate ideas into working solutions. Whether you choose Python, C++, or any other language, focus on honing these fundamental skills, and you’ll be well-prepared for success in your coding interviews.