Python Scripting: A Comprehensive Guide for Beginners and Beyond
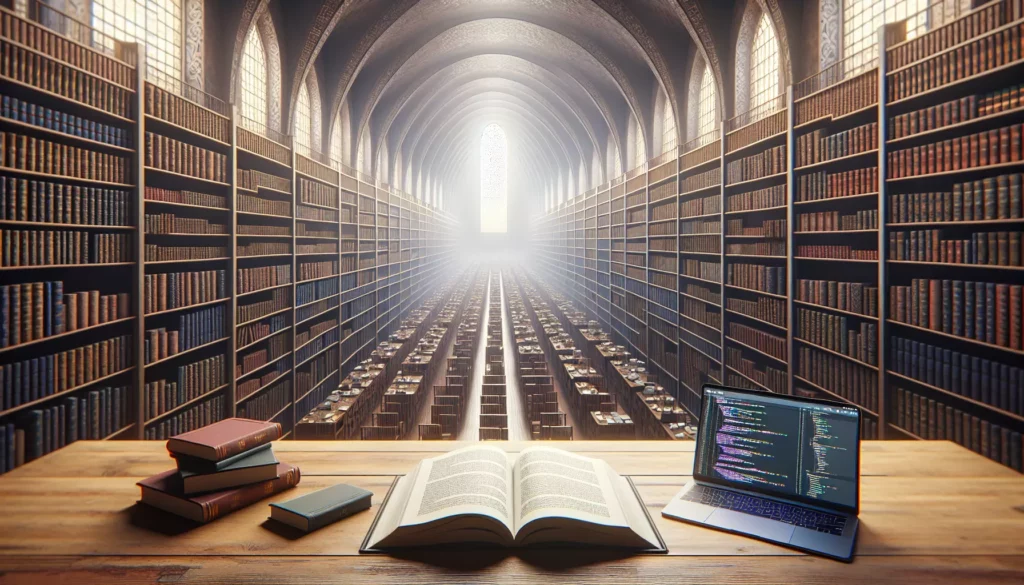
Introduction to Python Scripting
Python scripting is an essential skill for anyone looking to dive into the world of programming, automation, or data analysis. As a versatile and beginner-friendly language, Python has become increasingly popular for scripting tasks across various domains. In this comprehensive guide, we’ll explore the fundamentals of Python scripting, its applications, and how it can enhance your coding skills, particularly in preparation for technical interviews at major tech companies.
What is Python Scripting?
Python scripting refers to the process of writing and executing Python code to automate tasks, process data, or perform specific operations. Unlike larger software applications, scripts are typically shorter programs designed to accomplish specific tasks quickly and efficiently. Python’s simplicity and extensive library support make it an ideal language for scripting purposes.
Key Features of Python for Scripting:
- Readability: Python’s clean and straightforward syntax makes it easy to write and understand scripts.
- Versatility: Python can be used for a wide range of tasks, from simple file operations to complex data analysis.
- Large Standard Library: Python comes with a comprehensive standard library, reducing the need for external dependencies.
- Cross-platform Compatibility: Python scripts can run on various operating systems with minimal modifications.
- Active Community: A vast community of developers contributes to Python’s ecosystem, providing resources and support.
Getting Started with Python Scripting
To begin your journey in Python scripting, you’ll need to set up your development environment. Here’s a step-by-step guide to get you started:
1. Install Python
Visit the official Python website (python.org) and download the latest version of Python for your operating system. Follow the installation instructions provided.
2. Choose an Integrated Development Environment (IDE)
While you can write Python scripts in any text editor, using an IDE can significantly enhance your productivity. Some popular options include:
- PyCharm: A full-featured IDE with excellent debugging capabilities.
- Visual Studio Code: A lightweight, customizable editor with Python support.
- IDLE: Python’s built-in IDE, suitable for beginners.
3. Write Your First Python Script
Let’s create a simple “Hello, World!” script to get started:
print("Hello, World!")
Save this code in a file with a .py extension (e.g., hello_world.py) and run it using your Python interpreter or IDE.
Basic Python Scripting Concepts
To become proficient in Python scripting, you’ll need to understand several fundamental concepts:
1. Variables and Data Types
Python uses variables to store data. The language supports various data types, including:
- Integers: Whole numbers (e.g., 42)
- Floats: Decimal numbers (e.g., 3.14)
- Strings: Text enclosed in quotes (e.g., “Hello”)
- Booleans: True or False values
- Lists: Ordered collections of items
- Dictionaries: Key-value pairs
Example:
age = 25
name = "Alice"
is_student = True
grades = [85, 90, 78, 92]
person = {"name": "Bob", "age": 30}
2. Control Structures
Control structures allow you to manage the flow of your script. Key concepts include:
Conditional Statements:
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
Loops:
for grade in grades:
print(grade)
while age < 30:
print(f"Age: {age}")
age += 1
3. Functions
Functions are reusable blocks of code that perform specific tasks. They help organize your code and promote modularity:
def greet(name):
return f"Hello, {name}!"
message = greet("Alice")
print(message)
4. File Operations
Python makes it easy to work with files, which is crucial for many scripting tasks:
with open("example.txt", "w") as file:
file.write("This is a sample text.")
with open("example.txt", "r") as file:
content = file.read()
print(content)
Advanced Python Scripting Techniques
As you progress in your Python scripting journey, you’ll encounter more advanced concepts that can significantly enhance your scripts’ functionality and efficiency:
1. Error Handling
Proper error handling is crucial for creating robust scripts. Python’s try-except blocks allow you to catch and handle exceptions gracefully:
try:
result = 10 / 0
except ZeroDivisionError:
print("Error: Division by zero!")
finally:
print("This code always runs.")
2. List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists or other iterable objects:
numbers = [1, 2, 3, 4, 5]
squares = [x**2 for x in numbers]
print(squares) # Output: [1, 4, 9, 16, 25]
3. Lambda Functions
Lambda functions are small, anonymous functions that can be used for simple operations:
multiply = lambda x, y: x * y
result = multiply(5, 3)
print(result) # Output: 15
4. Decorators
Decorators allow you to modify or enhance functions without changing their source code:
def uppercase_decorator(func):
def wrapper():
result = func()
return result.upper()
return wrapper
@uppercase_decorator
def greet():
return "hello, world!"
print(greet()) # Output: HELLO, WORLD!
Python Scripting for Automation
One of the most powerful applications of Python scripting is automation. Here are some common areas where Python excels in automating tasks:
1. File and Folder Operations
Python’s os and shutil modules provide functions for file and folder manipulation:
import os
import shutil
# Create a new directory
os.mkdir("new_folder")
# Copy a file
shutil.copy("source.txt", "destination.txt")
# Move a file
shutil.move("old_location.txt", "new_location.txt")
# Delete a file
os.remove("unwanted_file.txt")
2. Web Scraping
Python’s requests and BeautifulSoup libraries make it easy to extract data from websites:
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
# Extract all paragraph texts
paragraphs = soup.find_all("p")
for p in paragraphs:
print(p.text)
3. Data Processing
Python’s pandas library is excellent for data manipulation and analysis:
import pandas as pd
# Read CSV file
df = pd.read_csv("data.csv")
# Perform data operations
filtered_df = df[df["age"] > 30]
average_salary = df["salary"].mean()
# Write results to a new CSV file
filtered_df.to_csv("filtered_data.csv", index=False)
4. Task Scheduling
The schedule library allows you to run Python scripts at specified intervals:
import schedule
import time
def job():
print("Running scheduled task...")
schedule.every(10).minutes.do(job)
schedule.every().hour.do(job)
schedule.every().day.at("10:30").do(job)
while True:
schedule.run_pending()
time.sleep(1)
Python Scripting for Technical Interviews
As you prepare for technical interviews, particularly at major tech companies, Python scripting skills can be a significant asset. Here are some areas to focus on:
1. Data Structures and Algorithms
Implement common data structures and algorithms in Python:
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
current = self.head
while current.next:
current = current.next
current.next = new_node
# Usage
ll = LinkedList()
ll.append(1)
ll.append(2)
ll.append(3)
2. Problem-Solving
Practice solving coding challenges using Python. Here’s an example of a function to check if a string is a palindrome:
def is_palindrome(s):
# Remove non-alphanumeric characters and convert to lowercase
s = ''.join(c.lower() for c in s if c.isalnum())
return s == s[::-1]
# Test the function
print(is_palindrome("A man, a plan, a canal: Panama")) # Output: True
print(is_palindrome("race a car")) # Output: False
3. Time and Space Complexity Analysis
Understand how to analyze the efficiency of your Python scripts:
def find_duplicate(nums):
# Time Complexity: O(n), Space Complexity: O(n)
seen = set()
for num in nums:
if num in seen:
return num
seen.add(num)
return None
# Test the function
print(find_duplicate([1, 3, 4, 2, 2])) # Output: 2
4. System Design
While system design often involves high-level concepts, you can use Python to prototype or demonstrate certain aspects of your design. For example, here’s a simple cache implementation:
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = OrderedDict()
def get(self, key):
if key not in self.cache:
return -1
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key, value):
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = value
if len(self.cache) > self.capacity:
self.cache.popitem(last=False)
# Usage
cache = LRUCache(2)
cache.put(1, 1)
cache.put(2, 2)
print(cache.get(1)) # Output: 1
cache.put(3, 3)
print(cache.get(2)) # Output: -1 (evicted)
Best Practices for Python Scripting
To write efficient and maintainable Python scripts, consider the following best practices:
1. Follow PEP 8 Guidelines
PEP 8 is the style guide for Python code. It covers naming conventions, indentation, and other formatting rules. Use tools like pylint or flake8 to check your code for PEP 8 compliance.
2. Write Docstrings
Document your functions and classes using docstrings:
def calculate_area(length, width):
"""
Calculate the area of a rectangle.
Args:
length (float): The length of the rectangle.
width (float): The width of the rectangle.
Returns:
float: The area of the rectangle.
"""
return length * width
3. Use Virtual Environments
Virtual environments help manage dependencies for different projects. Use the venv module to create isolated Python environments:
python -m venv myenv
source myenv/bin/activate # On Windows, use: myenv\Scripts\activate
4. Implement Logging
Use Python’s logging module for better debugging and monitoring:
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def divide(a, b):
try:
result = a / b
logger.info(f"Division result: {result}")
return result
except ZeroDivisionError:
logger.error("Division by zero attempted")
return None
divide(10, 2)
divide(5, 0)
5. Write Unit Tests
Use Python’s unittest module to create and run tests for your scripts:
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
if __name__ == "__main__":
unittest.main()
Conclusion
Python scripting is a powerful skill that can significantly enhance your programming abilities and prepare you for technical interviews at major tech companies. By mastering the fundamentals, exploring advanced techniques, and applying best practices, you’ll be well-equipped to tackle a wide range of scripting tasks and coding challenges.
Remember that the key to improving your Python scripting skills is consistent practice. Take on small projects, contribute to open-source initiatives, and challenge yourself with coding puzzles. As you gain experience, you’ll find that Python scripting becomes an invaluable tool in your programming toolkit, enabling you to automate tasks, analyze data, and solve complex problems efficiently.
Whether you’re preparing for a career in software development, data science, or any tech-related field, proficiency in Python scripting will undoubtedly give you a competitive edge. So, keep coding, keep learning, and embrace the versatility and power of Python scripting in your journey towards becoming a skilled programmer.