Push to GitHub: A Comprehensive Guide for Beginner Developers
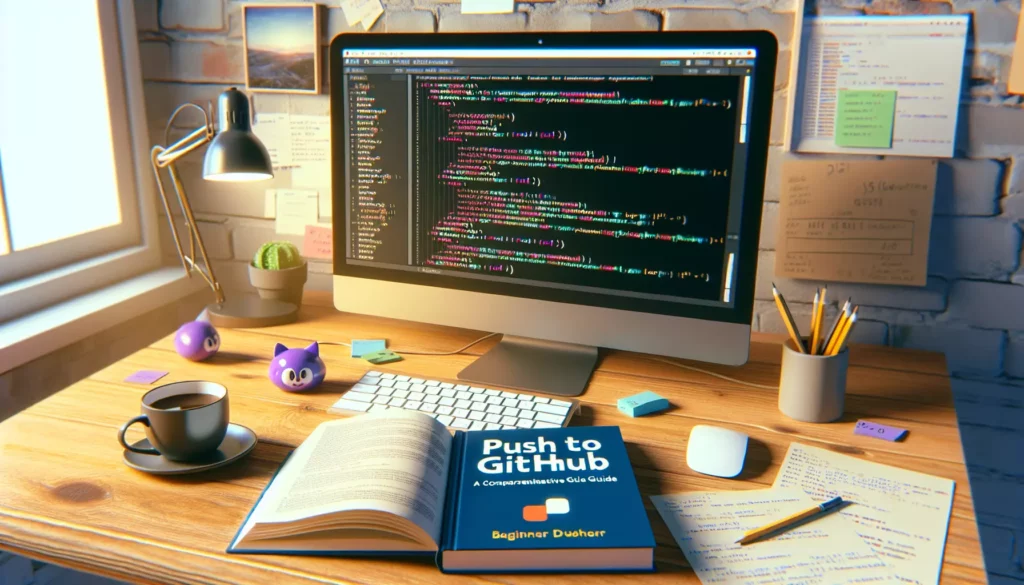
As a budding developer, one of the most crucial skills you’ll need to master is version control. Among the various version control systems available, Git stands out as the most widely used, and GitHub has become the go-to platform for hosting and sharing code. In this comprehensive guide, we’ll walk you through the process of pushing your code to GitHub, from setting up your repository to making your first commit and push.
Table of Contents
- What is GitHub?
- Setting Up GitHub
- Creating a Repository
- Initializing Git in Your Local Project
- Adding Files to Your Repository
- Making Commits
- Pushing to GitHub
- Best Practices for GitHub
- Troubleshooting Common Issues
- Advanced GitHub Features
- Conclusion
1. What is GitHub?
Before we dive into the technical aspects of pushing code to GitHub, let’s briefly discuss what GitHub is and why it’s so important in the world of software development.
GitHub is a web-based platform that uses Git for version control. It provides a centralized location for developers to store, manage, and collaborate on their code. Some key features of GitHub include:
- Repository hosting
- Version control
- Collaboration tools (pull requests, issues, etc.)
- Project management features
- Code review capabilities
- Integration with various development tools
For beginners, GitHub serves as an excellent platform to showcase your projects, contribute to open-source software, and learn from other developers’ code. As you progress in your coding journey, you’ll find that many employers and clients consider a strong GitHub profile as a valuable asset.
2. Setting Up GitHub
Before you can push your code to GitHub, you need to set up an account and configure your local environment. Here’s how to get started:
2.1. Create a GitHub Account
- Go to github.com
- Click on the “Sign up” button
- Follow the prompts to create your account, choosing a username, email, and password
- Verify your email address
2.2. Install Git
If you haven’t already installed Git on your local machine, you’ll need to do so:
- For Windows: Download and install Git from git-scm.com
- For macOS: Install Git using Homebrew by running
brew install git
in the terminal - For Linux: Use your distribution’s package manager (e.g.,
sudo apt-get install git
for Ubuntu)
2.3. Configure Git
After installing Git, you need to configure it with your name and email address. Open a terminal or command prompt and run the following commands:
git config --global user.name "Your Name"
git config --global user.email "your_email@example.com"
Replace “Your Name” and “your_email@example.com” with your actual name and email address.
3. Creating a Repository
Now that you have a GitHub account and Git installed, it’s time to create a repository for your project.
3.1. Create a New Repository on GitHub
- Log in to your GitHub account
- Click the “+” icon in the top-right corner and select “New repository”
- Choose a name for your repository
- Optionally, add a description
- Choose whether the repository should be public or private
- Click “Create repository”
3.2. Clone the Repository (Optional)
If you’re starting a new project, you might want to clone the empty repository to your local machine:
git clone https://github.com/your-username/your-repository.git
Replace “your-username” and “your-repository” with your GitHub username and the name of your repository.
4. Initializing Git in Your Local Project
If you have an existing project on your local machine that you want to push to GitHub, you’ll need to initialize Git in that project directory.
- Open a terminal or command prompt
- Navigate to your project directory:
cd path/to/your/project
- Initialize Git in the directory:
git init
5. Adding Files to Your Repository
Once you have Git initialized in your project directory, you can start adding files to your repository.
5.1. Check the Status of Your Repository
To see which files are ready to be added to your repository, use the following command:
git status
This will show you a list of untracked files and changes that haven’t been committed yet.
5.2. Add Files to the Staging Area
To add files to the staging area (preparing them for commit), use the git add
command:
git add filename.ext
To add all files in the current directory and its subdirectories, use:
git add .
5.3. Create a .gitignore File
It’s a good practice to create a .gitignore
file to specify which files or directories should be ignored by Git. This is especially useful for excluding build artifacts, temporary files, or sensitive information.
touch .gitignore
Edit the .gitignore
file to include patterns for files or directories you want to ignore. For example:
# Ignore node_modules directory
node_modules/
# Ignore log files
*.log
# Ignore .env files containing sensitive information
.env
6. Making Commits
After adding files to the staging area, you’re ready to make a commit. A commit is like a snapshot of your project at a specific point in time.
6.1. Create a Commit
To create a commit, use the following command:
git commit -m "Your commit message here"
Replace “Your commit message here” with a brief, descriptive message about the changes you’re committing.
6.2. Best Practices for Commit Messages
- Keep messages concise and descriptive
- Use the imperative mood (e.g., “Add feature” instead of “Added feature”)
- Limit the first line to 50 characters
- For longer explanations, add a blank line after the first line and then provide more details
7. Pushing to GitHub
Now that you’ve made commits to your local repository, it’s time to push those changes to GitHub.
7.1. Add a Remote Repository
If you didn’t clone the repository from GitHub, you’ll need to add a remote repository:
git remote add origin https://github.com/your-username/your-repository.git
Replace “your-username” and “your-repository” with your GitHub username and repository name.
7.2. Push Your Changes
To push your commits to GitHub, use the following command:
git push -u origin main
This pushes your changes to the “main” branch of your GitHub repository. The -u
flag sets up tracking, so in future pushes, you can simply use git push
.
Note: If you’re using an older version of Git or GitHub, you might need to use “master” instead of “main” as the default branch name.
8. Best Practices for GitHub
To make the most of GitHub and maintain a professional coding presence, consider these best practices:
8.1. Use Meaningful Repository Names
Choose repository names that clearly indicate the purpose or content of the project. This makes it easier for others to understand what your project is about at a glance.
8.2. Write Detailed README Files
Include a README.md file in your repository that explains:
- What the project does
- How to set it up and run it
- Any dependencies or prerequisites
- How to contribute (if it’s an open-source project)
- Licensing information
8.3. Use Branches for New Features or Bug Fixes
Instead of working directly on the main branch, create separate branches for new features or bug fixes. This helps keep your main branch stable and makes it easier to manage multiple changes.
git checkout -b feature-branch-name
8.4. Make Regular, Small Commits
Commit your changes frequently and in small, logical units. This makes it easier to track changes and revert if necessary.
8.5. Use Pull Requests
When working on a team or contributing to open-source projects, use pull requests to propose changes. This allows for code review and discussion before merging changes into the main branch.
8.6. Keep Your Fork Updated
If you’ve forked a repository, keep it updated with the original repository to stay current with any changes:
git remote add upstream https://github.com/original-owner/original-repository.git
git fetch upstream
git merge upstream/main
9. Troubleshooting Common Issues
Even experienced developers encounter issues when working with Git and GitHub. Here are some common problems and their solutions:
9.1. Authentication Failed
If you receive an “Authentication failed” error when pushing to GitHub, ensure that:
- Your GitHub username and password are correct
- You have the necessary permissions for the repository
- You’re using a personal access token if you have two-factor authentication enabled
9.2. Merge Conflicts
Merge conflicts occur when Git can’t automatically merge changes. To resolve them:
- Open the conflicting files and look for the conflict markers (
<<<<<<<
,=======
, and>>>>>>>
) - Manually edit the files to resolve the conflicts
- Add the resolved files using
git add
- Complete the merge by creating a new commit
9.3. Undoing Changes
If you need to undo changes, you have several options:
- To discard changes in your working directory:
git checkout -- filename
- To unstage files:
git reset HEAD filename
- To undo the last commit while keeping the changes:
git reset --soft HEAD~1
10. Advanced GitHub Features
As you become more comfortable with GitHub, you may want to explore some of its advanced features:
10.1. GitHub Actions
GitHub Actions allow you to automate your software development workflows. You can set up continuous integration, run tests, and deploy your applications directly from GitHub.
10.2. GitHub Pages
GitHub Pages lets you host static websites directly from your GitHub repository. This is great for project documentation, personal portfolios, or small web applications.
10.3. GitHub Packages
GitHub Packages is a package hosting service that allows you to host your packages privately or publicly and use packages as dependencies in your projects.
10.4. GitHub Codespaces
Codespaces provides a complete, configurable development environment in the cloud, allowing you to code, build, test, and debug from any device with a web browser.
11. Conclusion
Pushing your code to GitHub is an essential skill for any developer. It not only provides a backup of your work but also enables collaboration and showcases your projects to potential employers or clients. By following this guide, you should now have a solid understanding of how to push your code to GitHub, from setting up your account to making commits and resolving common issues.
Remember that mastering Git and GitHub takes practice. Don’t be afraid to experiment with different commands and workflows. As you continue to use these tools, you’ll become more comfortable with version control and discover how it can significantly improve your development process.
Keep coding, keep pushing, and watch your GitHub profile grow into a testament to your skills and dedication as a developer. Happy coding!