Public Key Infrastructure (PKI): The Backbone of Secure Digital Communication
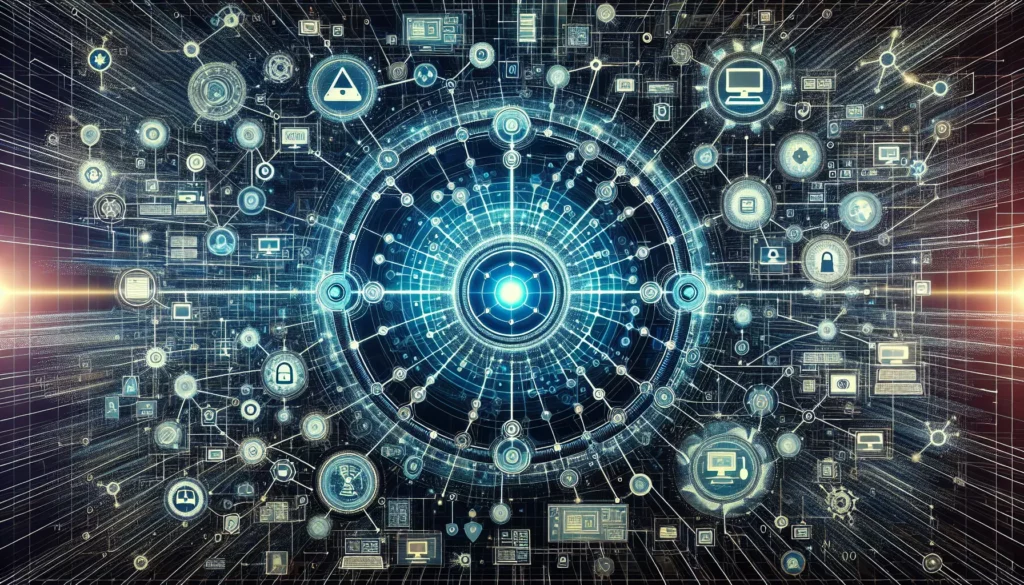
In today’s interconnected digital world, securing our online communications and transactions has become more critical than ever. One of the fundamental technologies that enables this security is Public Key Infrastructure (PKI). This powerful framework forms the backbone of secure digital communication, allowing us to exchange information safely across the internet. In this comprehensive guide, we’ll explore PKI, its components, how it works, and its significance in modern cybersecurity.
What is Public Key Infrastructure (PKI)?
Public Key Infrastructure (PKI) is a set of roles, policies, hardware, software, and procedures needed to create, manage, distribute, use, store, and revoke digital certificates and manage public-key encryption. It’s the foundation that enables secure electronic transfer of information for various network activities, such as e-commerce, internet banking, and confidential email communication.
At its core, PKI is built on the concept of public key cryptography, a system that uses a pair of keys: a public key that can be shared widely, and a private key that is known only to the owner. This system allows for secure communication and authentication without the need to share secret keys over insecure channels.
Key Components of PKI
To understand PKI better, let’s break down its main components:
1. Digital Certificates
Digital certificates are electronic documents used to prove the ownership of a public key. They contain information about the key, the identity of its owner, and the digital signature of the entity that verified the certificate’s contents. The most common standard for digital certificates is X.509.
2. Certificate Authority (CA)
A Certificate Authority is a trusted entity that issues digital certificates. It acts as a trusted third party that verifies the identity of entities requesting certificates and digitally signs the certificates it issues.
3. Registration Authority (RA)
The Registration Authority is responsible for verifying the identity of entities requesting their digital certificates before the CA issues them. The RA acts as the intermediary between the CA and the certificate applicant.
4. Certificate Repository
This is a database of active digital certificates. Certificate repositories can be publicly accessible, allowing anyone to retrieve the public key of an entity that has been issued a certificate.
5. Certificate Revocation List (CRL)
The CRL is a list of certificates that have been revoked before their scheduled expiration date. Reasons for revocation can include compromise of the private key or changes in the certificate holder’s affiliation.
How PKI Works
Now that we’ve covered the main components, let’s walk through how PKI typically functions:
- Certificate Request: An entity (individual or organization) requests a digital certificate from a CA.
- Verification: The RA verifies the identity of the requester.
- Certificate Issuance: If verification is successful, the CA issues a digital certificate to the entity.
- Certificate Usage: The entity can now use the certificate for various purposes, such as securing communications or signing documents.
- Certificate Verification: When another party receives the certificate, they can verify its authenticity using the CA’s public key.
- Revocation: If necessary, certificates can be revoked and added to the CRL.
The Importance of PKI in Cybersecurity
PKI plays a crucial role in modern cybersecurity for several reasons:
1. Secure Communication
PKI enables secure communication over insecure networks like the internet. It forms the basis of protocols like HTTPS, which secures website connections.
2. Digital Signatures
PKI allows for the creation of digital signatures, which provide non-repudiation and integrity for digital documents and transactions.
3. Identity Verification
By providing a framework for verifying identities, PKI helps prevent impersonation and enhances trust in digital interactions.
4. Data Integrity
PKI ensures that data hasn’t been tampered with during transmission, maintaining its integrity.
5. Compliance
Many industries require the use of PKI to comply with regulations regarding data protection and privacy.
PKI in Practice: Real-World Applications
PKI is used in a wide variety of applications in our daily digital lives. Let’s explore some common use cases:
1. Secure Web Browsing (HTTPS)
When you visit a website that uses HTTPS, your browser uses PKI to verify the site’s identity and establish a secure connection. This is indicated by the padlock icon in your browser’s address bar.
2. Email Security
PKI is used in email security protocols like S/MIME (Secure/Multipurpose Internet Mail Extensions) to provide email encryption and digital signatures.
3. Virtual Private Networks (VPNs)
VPNs often use PKI for authentication and to establish secure tunnels for data transmission.
4. Internet of Things (IoT)
As IoT devices become more prevalent, PKI is increasingly used to secure communications between these devices and ensure their authenticity.
5. Code Signing
Software developers use PKI to digitally sign their code, assuring users that the software hasn’t been tampered with and comes from a verified source.
Implementing PKI: Best Practices
If you’re considering implementing PKI in your organization, here are some best practices to keep in mind:
1. Robust Key Management
Implement strong procedures for generating, storing, and managing cryptographic keys. This is crucial for maintaining the security of your PKI.
2. Regular Audits
Conduct regular audits of your PKI to ensure it’s functioning correctly and securely. This includes checking for expired or revoked certificates.
3. Use of Hardware Security Modules (HSMs)
Consider using HSMs to generate and store private keys. These specialized devices provide an extra layer of security for critical cryptographic operations.
4. Clear Certificate Policies
Establish clear policies regarding certificate issuance, usage, and revocation. This helps maintain the integrity of your PKI.
5. Automation
Where possible, automate PKI processes to reduce the risk of human error and ensure timely certificate renewals and revocations.
Challenges and Future of PKI
While PKI has proven to be a robust and effective security framework, it’s not without its challenges:
1. Scalability
As the number of devices and services requiring certificates grows, managing PKI at scale becomes increasingly complex.
2. Quantum Computing
The advent of quantum computing poses a potential threat to current cryptographic algorithms used in PKI. Research is ongoing into quantum-resistant algorithms.
3. Certificate Authority Compromises
If a CA is compromised, it can have far-reaching consequences. Improving CA security and developing alternative trust models are ongoing areas of research.
4. User Education
Many users don’t fully understand PKI, which can lead to security vulnerabilities. Ongoing education is crucial.
Despite these challenges, PKI continues to evolve. Future developments may include:
- Integration with blockchain technology for decentralized trust models
- Improved automation and management tools
- Enhanced support for IoT and mobile devices
- Development of post-quantum cryptography standards
PKI and Coding: Implementing PKI in Your Applications
As a developer, understanding PKI is crucial for building secure applications. Let’s look at how you might implement PKI concepts in your code using Python as an example.
Generating a Key Pair
Here’s a simple example of generating an RSA key pair using Python’s cryptography library:
from cryptography.hazmat.primitives.asymmetric import rsa
from cryptography.hazmat.primitives import serialization
# Generate a private key
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
# Get the public key
public_key = private_key.public_key()
# Serialize the private key
pem = private_key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.PKCS8,
encryption_algorithm=serialization.NoEncryption()
)
# Serialize the public key
public_pem = public_key.public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo
)
print(pem.decode())
print(public_pem.decode())
Creating a Self-Signed Certificate
Here’s how you might create a self-signed certificate:
from cryptography import x509
from cryptography.x509.oid import NameOID
from cryptography.hazmat.primitives import hashes
import datetime
# Assume we have a private_key from the previous example
# Create a self-signed certificate
subject = issuer = x509.Name([
x509.NameAttribute(NameOID.COUNTRY_NAME, u"US"),
x509.NameAttribute(NameOID.STATE_OR_PROVINCE_NAME, u"California"),
x509.NameAttribute(NameOID.LOCALITY_NAME, u"San Francisco"),
x509.NameAttribute(NameOID.ORGANIZATION_NAME, u"My Company"),
x509.NameAttribute(NameOID.COMMON_NAME, u"mysite.com"),
])
cert = x509.CertificateBuilder().subject_name(
subject
).issuer_name(
issuer
).public_key(
private_key.public_key()
).serial_number(
x509.random_serial_number()
).not_valid_before(
datetime.datetime.utcnow()
).not_valid_after(
datetime.datetime.utcnow() + datetime.timedelta(days=365)
).add_extension(
x509.SubjectAlternativeName([x509.DNSName(u"mysite.com")]),
critical=False,
).sign(private_key, hashes.SHA256())
# Serialize the certificate
cert_pem = cert.public_bytes(encoding=serialization.Encoding.PEM)
print(cert_pem.decode())
These examples demonstrate basic PKI operations, but remember that in a production environment, you’d need to consider many additional factors, including secure key storage, certificate chain validation, and proper error handling.
Conclusion
Public Key Infrastructure is a cornerstone of modern digital security. It provides the framework necessary for secure communication, authentication, and data integrity in our increasingly connected world. As a developer, understanding PKI is crucial for building secure applications and systems.
While PKI comes with its challenges, ongoing research and development continue to improve its robustness and applicability. As we move into an era of quantum computing and ever-more connected devices, PKI will undoubtedly continue to evolve to meet new security challenges.
By mastering PKI concepts and implementation, you’ll be well-equipped to tackle complex security challenges in your software development projects. Whether you’re securing web applications, implementing secure communication protocols, or developing IoT solutions, a solid understanding of PKI will be invaluable in your journey as a developer.