Project-Based Learning vs. Structured Tutorials: Which is Better for Coding Education?
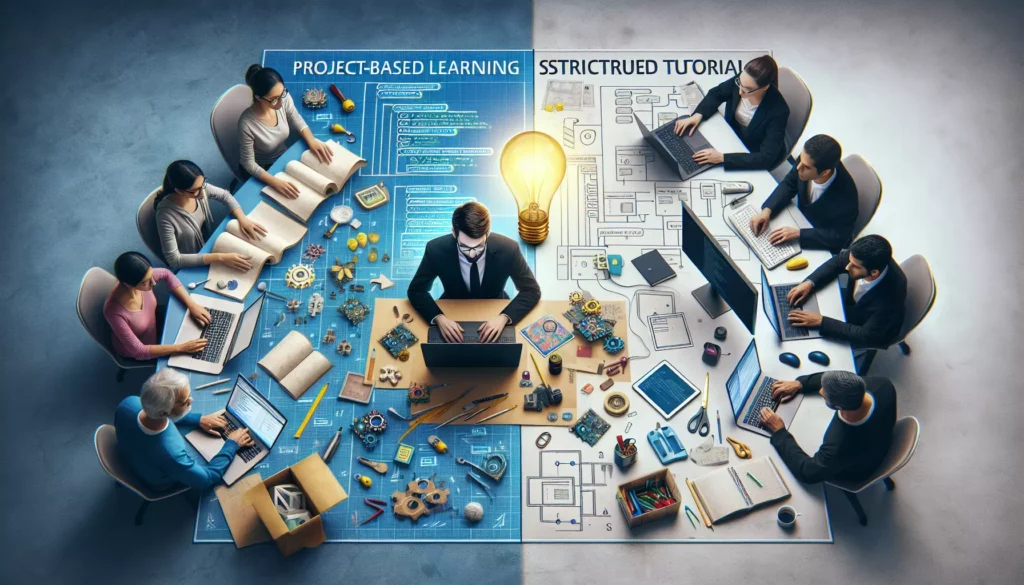
In the ever-evolving landscape of coding education, aspiring programmers often find themselves at a crossroads: should they dive into project-based learning or follow structured tutorials? This question is particularly relevant in the context of platforms like AlgoCademy, which aims to guide learners from beginner-level coding to technical interview preparation for major tech companies. In this comprehensive exploration, we’ll delve into the merits and drawbacks of both approaches, helping you determine which method might be more effective for your learning journey.
Understanding Project-Based Learning in Coding
Project-based learning (PBL) is an educational approach where students learn by actively engaging in real-world and personally meaningful projects. In the context of coding, this might involve building a web application, creating a mobile app, or developing a data analysis tool from scratch.
Advantages of Project-Based Learning
- Practical Application: PBL allows learners to apply theoretical knowledge to real-world scenarios, bridging the gap between concept and practice.
- Motivation and Engagement: Working on a tangible project can be more motivating than abstract exercises, keeping learners engaged throughout the process.
- Problem-Solving Skills: Projects often present unexpected challenges, fostering critical thinking and problem-solving abilities.
- Portfolio Building: Completed projects serve as excellent additions to a programmer’s portfolio, showcasing practical skills to potential employers.
- Holistic Learning: Projects typically involve multiple aspects of programming, providing a well-rounded learning experience.
Challenges of Project-Based Learning
- Lack of Structure: Without proper guidance, beginners might feel overwhelmed or unsure where to start.
- Knowledge Gaps: Learners might miss fundamental concepts that aren’t directly applicable to their chosen project.
- Time-Intensive: Projects can be time-consuming, potentially leading to frustration if progress is slow.
- Difficulty in Assessing Progress: It can be challenging to measure learning outcomes objectively in project-based approaches.
The Role of Structured Tutorials in Coding Education
Structured tutorials provide a systematic, step-by-step approach to learning coding concepts and techniques. These often come in the form of online courses, textbooks, or guided learning paths on platforms like AlgoCademy.
Benefits of Structured Tutorials
- Clear Learning Path: Tutorials offer a well-defined curriculum, ensuring learners cover all necessary topics in a logical order.
- Foundational Knowledge: They excel at teaching fundamental concepts and best practices, which are crucial for long-term success in programming.
- Consistency: Structured learning helps maintain a steady pace and ensures consistent progress.
- Immediate Feedback: Many tutorials include quizzes or coding exercises with automated feedback, allowing learners to quickly identify and correct mistakes.
- Efficiency: Well-designed tutorials can convey information more efficiently than the trial-and-error approach often associated with projects.
Limitations of Structured Tutorials
- Lack of Real-World Context: Tutorials may not always reflect the complexities of real-world programming scenarios.
- Passive Learning: There’s a risk of learners becoming passive consumers of information rather than active problem-solvers.
- Limited Creativity: Following predefined steps may not encourage creative thinking or innovative problem-solving.
- Potential for Boredom: Some learners may find the structured approach less engaging than hands-on project work.
Combining the Best of Both Worlds
The debate between project-based learning and structured tutorials isn’t necessarily an either-or proposition. In fact, the most effective coding education often combines elements of both approaches. Here’s how you can leverage the strengths of each method:
1. Start with Structured Fundamentals
Begin your coding journey with structured tutorials to build a solid foundation. Platforms like AlgoCademy offer comprehensive courses that cover essential programming concepts, algorithms, and data structures. This phase ensures you have the necessary knowledge base to tackle more complex challenges.
2. Integrate Mini-Projects into Tutorials
As you progress through structured learning, incorporate small projects that apply the concepts you’ve just learned. For example, after a tutorial on loops and arrays, you might create a simple program that processes a list of numbers.
3. Gradually Increase Project Complexity
As your skills improve, start working on more substantial projects. These could be guided projects suggested by your learning platform or personal ideas you’re passionate about. The key is to choose projects that stretch your abilities without being overwhelming.
4. Use Tutorials for Specific Skills
When you encounter challenges in your projects, turn to targeted tutorials to fill knowledge gaps. For instance, if your project requires a specific algorithm you’re unfamiliar with, find a tutorial that explains it in depth.
5. Participate in Coding Challenges
Platforms like AlgoCademy often offer coding challenges or algorithmic problems. These serve as a middle ground between tutorials and full-fledged projects, allowing you to apply your skills in a structured yet challenging environment.
6. Reflect and Review
After completing a project, review the concepts you’ve applied and identify areas where you need more practice. This self-reflection can guide you back to specific tutorials or towards new learning resources.
Tailoring Your Learning Approach
The effectiveness of project-based learning versus structured tutorials can vary depending on several factors:
Learning Style
Some individuals thrive on the structure and guidance of tutorials, while others learn best through hands-on experimentation. Reflect on your past learning experiences to identify which approach resonates more with you.
Experience Level
Beginners often benefit more from structured tutorials to build a strong foundation. As you gain experience, you may find project-based learning more beneficial for advancing your skills.
Learning Goals
If your goal is to quickly acquire specific skills for a job or project, focused tutorials might be more efficient. For broader skill development or career preparation, a mix of tutorials and projects is often ideal.
Time Constraints
Consider your available time for learning. Structured tutorials can be more time-efficient, while projects often require a larger time investment but may lead to deeper understanding.
Leveraging AlgoCademy’s Approach
AlgoCademy’s platform is designed to bridge the gap between structured learning and practical application. Here’s how you can make the most of its features:
1. Interactive Coding Tutorials
Start with AlgoCademy’s interactive tutorials to build a strong foundation in programming concepts, algorithms, and data structures. These structured lessons provide immediate feedback and help you develop good coding habits.
2. AI-Powered Assistance
As you work through tutorials or personal projects, take advantage of AlgoCademy’s AI-powered assistance. This feature can help you overcome roadblocks, explain complex concepts, and suggest improvements to your code.
3. Problem-Solving Exercises
Engage with AlgoCademy’s problem-solving exercises, which serve as mini-projects to apply your learning. These exercises are designed to mimic real-world coding challenges and technical interview questions.
4. Algorithmic Thinking Challenges
Participate in algorithmic thinking challenges to develop your problem-solving skills. These challenges combine elements of structured learning with the creative thinking required in project-based approaches.
5. Interview Preparation
As you progress, use AlgoCademy’s interview preparation resources. These often include a mix of structured lessons on common interview topics and practical coding exercises that simulate real interview scenarios.
Practical Tips for Effective Learning
Regardless of whether you lean towards project-based learning or structured tutorials, here are some tips to enhance your coding education:
1. Set Clear Goals
Define what you want to achieve with your coding education. Whether it’s landing a job at a major tech company or building a specific type of application, clear goals will help you choose the most appropriate learning methods.
2. Create a Learning Schedule
Consistency is key in coding education. Set aside regular time for learning, whether it’s working through tutorials or advancing your projects.
3. Join a Community
Engage with other learners through coding forums, local meetups, or online communities. This can provide motivation, support, and opportunities for collaborative learning.
4. Practice Active Recall
After completing a tutorial or project milestone, try to explain the concepts you’ve learned without referring to notes. This reinforces your understanding and helps identify areas that need more attention.
5. Embrace Mistakes
Don’t be discouraged by errors or bugs in your code. These are valuable learning opportunities that contribute to your growth as a programmer.
6. Stay Updated
The field of programming is constantly evolving. Make a habit of staying informed about new technologies, best practices, and industry trends.
Code Examples: Bridging Tutorials and Projects
To illustrate how concepts from tutorials can be applied in project-based learning, let’s look at a simple example. Suppose you’ve completed a tutorial on basic data structures and algorithms, focusing on arrays and sorting. Here’s how you might apply this knowledge in a small project:
Tutorial Concept: Bubble Sort Algorithm
First, let’s review the bubble sort algorithm as you might learn it in a structured tutorial:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list)
print("Sorted array:", sorted_list)
Project Application: Book Sorting System
Now, let’s apply this concept to a small project: a book sorting system for a library. This project will incorporate the bubble sort algorithm while adding real-world context and additional features.
class Book:
def __init__(self, title, author, publication_year):
self.title = title
self.author = author
self.publication_year = publication_year
def __str__(self):
return f"{self.title} by {self.author} ({self.publication_year})"
def sort_books(books, key='title'):
n = len(books)
for i in range(n):
for j in range(0, n - i - 1):
if getattr(books[j], key) > getattr(books[j + 1], key):
books[j], books[j + 1] = books[j + 1], books[j]
return books
# Create a list of books
library = [
Book("To Kill a Mockingbird", "Harper Lee", 1960),
Book("1984", "George Orwell", 1949),
Book("Pride and Prejudice", "Jane Austen", 1813),
Book("The Great Gatsby", "F. Scott Fitzgerald", 1925)
]
# Sort books by title
sorted_by_title = sort_books(library, 'title')
print("Books sorted by title:")
for book in sorted_by_title:
print(book)
# Sort books by publication year
sorted_by_year = sort_books(library, 'publication_year')
print("\nBooks sorted by publication year:")
for book in sorted_by_year:
print(book)
This project example demonstrates how you can take a basic algorithm learned through a tutorial and apply it to a more complex, real-world scenario. It incorporates object-oriented programming, sorting based on different attributes, and handling a collection of custom objects.
Conclusion: Finding Your Optimal Learning Path
The journey of learning to code is highly personal, and the most effective approach often involves a combination of structured tutorials and project-based learning. Platforms like AlgoCademy offer a balanced approach, providing structured content while encouraging practical application and problem-solving skills.
As you progress in your coding education, remember that flexibility is key. Be prepared to switch between tutorials and projects as needed, always focusing on what helps you learn and retain information best. Whether you’re aiming to ace technical interviews at top tech companies or build your own innovative applications, the key is to stay curious, persistent, and engaged with your learning process.
Ultimately, the “better” method between project-based learning and structured tutorials is the one that keeps you motivated, challenges you appropriately, and aligns with your learning style and goals. By thoughtfully combining both approaches and leveraging resources like AlgoCademy, you can create a robust, effective learning strategy that prepares you for success in the dynamic world of programming.