Programming Habits That Are Holding You Back
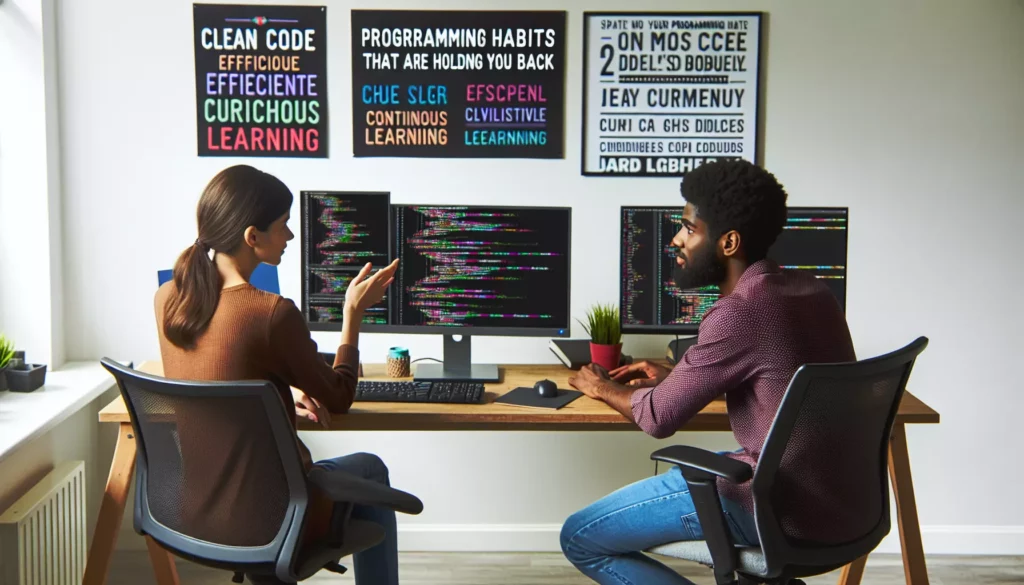
As aspiring developers and seasoned programmers alike, we’re always striving to improve our coding skills and become more efficient in our work. However, sometimes the very habits we’ve developed over time can actually hinder our progress and prevent us from reaching our full potential. In this comprehensive guide, we’ll explore some common programming habits that might be holding you back and provide actionable advice on how to overcome them.
1. Neglecting Version Control
One of the most crucial tools in a developer’s arsenal is version control, yet many programmers underutilize or completely ignore it. Version control systems like Git allow you to track changes, collaborate with others, and maintain a history of your project. Neglecting version control can lead to lost work, difficulty in collaboration, and challenges in managing different versions of your code.
How to improve:
- Make Git a fundamental part of your workflow
- Commit frequently with meaningful messages
- Use branches for new features or experiments
- Learn to use advanced Git features like rebasing and cherry-picking
2. Avoiding Code Reviews
Code reviews are an essential part of the development process, yet many programmers shy away from them. Whether it’s fear of criticism or a desire to work independently, avoiding code reviews can lead to lower code quality, missed bugs, and a lack of knowledge sharing within your team.
How to improve:
- Embrace code reviews as a learning opportunity
- Actively seek feedback from peers and senior developers
- Offer to review others’ code to gain different perspectives
- Use code review tools to streamline the process
3. Ignoring Code Documentation
Writing clear and concise documentation is often overlooked, especially when deadlines are looming. However, neglecting documentation can lead to confusion, wasted time, and difficulties in maintaining or expanding your codebase.
How to improve:
- Make documentation a part of your coding process, not an afterthought
- Use clear and descriptive variable and function names
- Write meaningful comments for complex logic
- Maintain up-to-date README files for your projects
4. Overengineering Solutions
While it’s important to write scalable and maintainable code, overengineering can lead to unnecessary complexity and wasted time. Many programmers fall into the trap of trying to account for every possible future scenario, resulting in overly complicated solutions to simple problems.
How to improve:
- Follow the YAGNI (You Ain’t Gonna Need It) principle
- Start with the simplest solution that solves the current problem
- Refactor and expand your code as new requirements arise
- Regularly review your code for unnecessary complexity
5. Neglecting Testing
Writing tests for your code is crucial for maintaining quality and preventing regressions. However, many developers view testing as a chore or an unnecessary step, leading to bugs slipping through and difficulties in refactoring code later on.
How to improve:
- Adopt a test-driven development (TDD) approach
- Write unit tests for individual functions and components
- Implement integration tests for larger system interactions
- Use continuous integration tools to automate testing
6. Copying and Pasting Code Without Understanding
In the age of Stack Overflow and countless code repositories, it’s tempting to simply copy and paste solutions to problems. While this can be a quick fix, it often leads to a lack of understanding and potential issues down the line.
How to improve:
- Take the time to understand code before using it in your project
- Modify and adapt copied code to fit your specific needs
- Use copied code as a learning opportunity to explore new concepts
- Always give credit and adhere to licensing requirements when using others’ code
7. Ignoring Performance Considerations
While premature optimization can be a pitfall, completely ignoring performance considerations can lead to slow and inefficient code. Many developers focus solely on functionality without considering the impact of their code on system resources.
How to improve:
- Learn and apply basic algorithmic complexity concepts (Big O notation)
- Use profiling tools to identify performance bottlenecks
- Optimize critical paths in your code
- Consider trade-offs between readability and performance
8. Not Staying Up-to-Date with New Technologies
The tech industry moves at a rapid pace, and failing to keep up with new technologies and best practices can leave you behind. Many developers become comfortable with their current stack and resist learning new tools or languages.
How to improve:
- Set aside time regularly to explore new technologies
- Attend conferences, webinars, or local meetups
- Follow industry leaders and tech blogs
- Experiment with new tools in side projects
9. Neglecting Soft Skills
While technical skills are crucial, many programmers underestimate the importance of soft skills in their career development. Communication, teamwork, and problem-solving abilities are just as important as coding skills in most professional environments.
How to improve:
- Practice explaining technical concepts to non-technical people
- Actively participate in team discussions and meetings
- Develop your writing skills for documentation and emails
- Take courses or read books on communication and leadership
10. Not Taking Breaks
Programming can be intense and mentally taxing. Many developers fall into the habit of working long hours without breaks, leading to burnout and decreased productivity. Regular breaks are essential for maintaining focus and creativity.
How to improve:
- Use techniques like the Pomodoro method to structure your work
- Take short breaks to stretch, walk, or rest your eyes
- Step away from difficult problems and return with a fresh perspective
- Maintain a healthy work-life balance
11. Failing to Plan Before Coding
Jumping straight into coding without proper planning can lead to poorly structured code, missed requirements, and wasted time. Many developers underestimate the importance of the planning phase in the development process.
How to improve:
- Start with a clear understanding of the problem and requirements
- Create flowcharts or diagrams to visualize the solution
- Break down large tasks into smaller, manageable components
- Consider potential edge cases and error scenarios
12. Reinventing the Wheel
While it’s important to understand how things work under the hood, constantly reinventing existing solutions can be a waste of time and effort. Many programmers fall into the trap of writing everything from scratch instead of leveraging existing libraries and frameworks.
How to improve:
- Research existing solutions before starting a new project
- Learn to evaluate and choose appropriate libraries and frameworks
- Contribute to open-source projects instead of building everything yourself
- Focus on solving unique problems specific to your project
13. Ignoring Code Smells
Code smells are indicators of potential problems in your codebase. Ignoring these warning signs can lead to technical debt and make your code harder to maintain over time. Many developers overlook code smells in favor of quick fixes or meeting deadlines.
How to improve:
- Familiarize yourself with common code smells
- Use static analysis tools to identify potential issues
- Regularly refactor your code to address smells
- Encourage code quality discussions within your team
14. Not Using Proper Debugging Techniques
Effective debugging is a crucial skill for any programmer. However, many developers rely on inefficient methods like excessive print statements or trial-and-error approaches instead of using proper debugging tools and techniques.
How to improve:
- Learn to use debugging tools in your IDE or development environment
- Practice using breakpoints and step-through debugging
- Understand how to read and interpret stack traces
- Use logging effectively for production debugging
15. Neglecting Security Considerations
In today’s interconnected world, security is more important than ever. Many developers treat security as an afterthought or assume it’s someone else’s responsibility, leading to vulnerable applications and potential data breaches.
How to improve:
- Learn about common security vulnerabilities (e.g., OWASP Top 10)
- Implement security best practices from the start of your projects
- Use security tools and static analysis to identify potential vulnerabilities
- Stay informed about security updates for the libraries and frameworks you use
Practical Examples
Let’s look at some practical examples of how these habits can manifest in code and how to improve them:
1. Neglecting Version Control
Bad habit:
// project_v1.py
// project_v2.py
// project_final.py
// project_final_for_real.py
// project_final_final.py
Improved approach:
$ git init
$ git add .
$ git commit -m "Initial commit"
$ git branch feature/new-functionality
$ git checkout feature/new-functionality
// Make changes
$ git commit -m "Add new functionality"
$ git checkout main
$ git merge feature/new-functionality
2. Ignoring Code Documentation
Bad habit:
def process_data(x):
y = x * 2
z = y + 3
return z * 4
Improved approach:
def process_data(input_value):
"""
Process the input value through a series of calculations.
Args:
input_value (int): The initial value to be processed.
Returns:
int: The result of the calculations.
"""
doubled = input_value * 2
intermediate = doubled + 3
result = intermediate * 4
return result
3. Overengineering Solutions
Bad habit:
class DataProcessor:
def __init__(self):
self.data = []
def add_data(self, item):
self.data.append(item)
def process_data(self):
return [self._complex_calculation(item) for item in self.data]
def _complex_calculation(self, item):
# Overly complex calculation for a simple task
return item * 2 + 3
processor = DataProcessor()
processor.add_data(5)
result = processor.process_data()[0]
Improved approach:
def process_data(item):
return item * 2 + 3
result = process_data(5)
4. Neglecting Testing
Bad habit: Not writing tests at all.
Improved approach:
def add_numbers(a, b):
return a + b
# Test file: test_add_numbers.py
import unittest
from my_math import add_numbers
class TestAddNumbers(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-2, -3), -5)
def test_add_mixed_numbers(self):
self.assertEqual(add_numbers(-2, 3), 1)
if __name__ == '__main__':
unittest.main()
Conclusion
Recognizing and addressing these common programming habits is crucial for your growth as a developer. By actively working to improve in these areas, you’ll not only become a more efficient and effective programmer but also a more valuable team member and professional in the tech industry.
Remember, breaking bad habits and forming new ones takes time and consistent effort. Be patient with yourself and focus on gradual improvement. Regularly reflect on your coding practices and seek feedback from peers and mentors. With dedication and practice, you’ll be able to overcome these habits and unlock your full potential as a programmer.
As you continue your journey in software development, platforms like AlgoCademy can be invaluable resources for honing your skills, practicing algorithmic thinking, and preparing for technical interviews. By combining the habits of successful programmers with structured learning and practice, you’ll be well-equipped to tackle any coding challenge that comes your way.
Happy coding, and may your journey to becoming a better programmer be filled with growth, learning, and exciting discoveries!