Procedural vs Object-Oriented Programming: Understanding the Key Differences
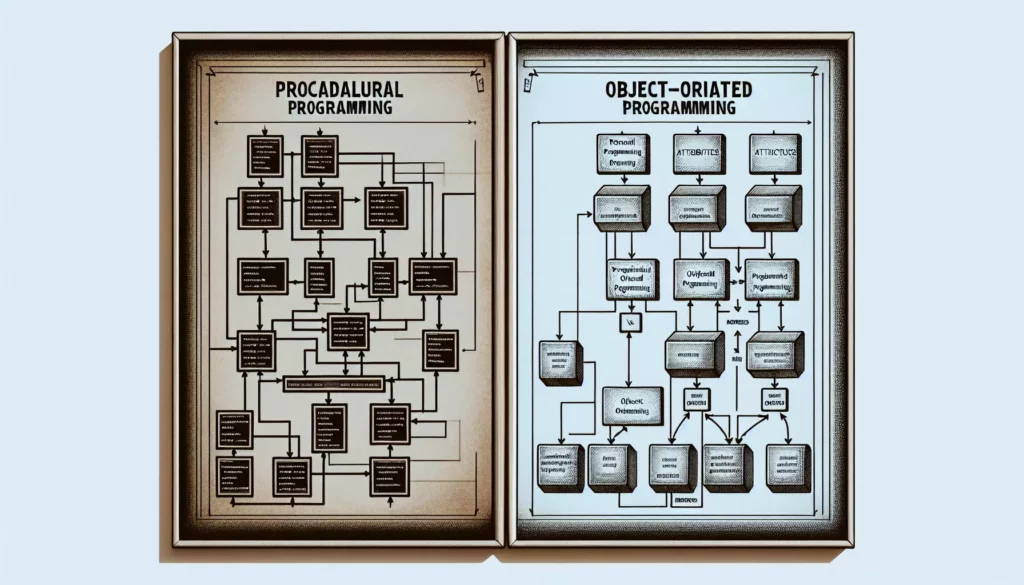
In the world of software development, two major programming paradigms have dominated the landscape for decades: procedural programming and object-oriented programming (OOP). Both approaches offer unique ways to structure and organize code, each with its own strengths and weaknesses. As aspiring programmers or those looking to enhance their coding skills, it’s crucial to understand the differences between these paradigms. This knowledge not only helps in choosing the right approach for a given project but also aids in preparing for technical interviews at major tech companies.
In this comprehensive guide, we’ll explore the key differences between procedural and object-oriented programming, their respective advantages and disadvantages, and when to use each paradigm. We’ll also provide practical examples to illustrate these concepts, helping you grasp the fundamental distinctions between these two programming approaches.
1. Understanding Procedural Programming
Procedural programming, also known as imperative programming, is one of the oldest programming paradigms. It’s based on the concept of procedure calls, where the program is divided into procedures (also known as routines, subroutines, or functions) that perform specific tasks.
Key Characteristics of Procedural Programming:
- Sequential Execution: Programs are executed in a top-down, step-by-step manner.
- Function-Centric: Code is organized into functions that perform specific tasks.
- Data and Procedures are Separate: Data structures and the functions that operate on them are kept separate.
- Global Data: Often relies on global variables that can be accessed and modified by different parts of the program.
Example of Procedural Programming:
Let’s look at a simple example of procedural programming in Python:
# Global variables
students = []
def add_student(name, age, grade):
student = {"name": name, "age": age, "grade": grade}
students.append(student)
def print_students():
for student in students:
print(f"Name: {student['name']}, Age: {student['age']}, Grade: {student['grade']}")
# Main program
add_student("Alice", 20, "A")
add_student("Bob", 22, "B")
print_students()
In this example, we have global data (the students
list) and functions that operate on this data (add_student
and print_students
). The program follows a sequential execution model, calling functions in a specific order.
2. Understanding Object-Oriented Programming (OOP)
Object-Oriented Programming is a programming paradigm based on the concept of “objects,” which can contain data and code. The data is in the form of fields (often known as attributes or properties), and the code is in the form of procedures (often known as methods).
Key Characteristics of Object-Oriented Programming:
- Encapsulation: Bundling of data and the methods that operate on that data within a single unit (object).
- Inheritance: Ability to create new classes based on existing classes, inheriting their properties and methods.
- Polymorphism: Ability of objects to take on multiple forms and behave differently based on the context.
- Abstraction: Hiding complex implementation details and showing only the necessary features of an object.
Example of Object-Oriented Programming:
Here’s the same student management system implemented using OOP in Python:
class Student:
def __init__(self, name, age, grade):
self.name = name
self.age = age
self.grade = grade
def __str__(self):
return f"Name: {self.name}, Age: {self.age}, Grade: {self.grade}"
class StudentManagementSystem:
def __init__(self):
self.students = []
def add_student(self, student):
self.students.append(student)
def print_students(self):
for student in self.students:
print(student)
# Main program
sms = StudentManagementSystem()
sms.add_student(Student("Alice", 20, "A"))
sms.add_student(Student("Bob", 22, "B"))
sms.print_students()
In this OOP example, we have two classes: Student
and StudentManagementSystem
. The Student
class encapsulates the data and behavior of a student, while the StudentManagementSystem
class manages a collection of students.
3. Key Differences Between Procedural and Object-Oriented Programming
Now that we’ve seen examples of both paradigms, let’s dive into the key differences between procedural and object-oriented programming:
3.1. Program Structure
- Procedural: Organized around functions or procedures that operate on data.
- OOP: Organized around objects that contain both data and methods.
3.2. Data Access
- Procedural: Data is typically separate from functions and often global.
- OOP: Data is encapsulated within objects and accessed through methods.
3.3. Code Reusability
- Procedural: Achieves code reuse through functions.
- OOP: Achieves code reuse through inheritance and composition.
3.4. Data Security
- Procedural: Less secure as data is often globally accessible.
- OOP: More secure due to encapsulation and data hiding.
3.5. Modularity
- Procedural: Modularity is achieved through functions but can become complex in large programs.
- OOP: Naturally modular, with objects serving as self-contained units.
3.6. Flexibility and Extensibility
- Procedural: Can be less flexible and harder to extend without modifying existing code.
- OOP: More flexible and easily extensible through inheritance and polymorphism.
3.7. Complexity
- Procedural: Generally simpler and easier to learn for beginners.
- OOP: Can be more complex, with a steeper learning curve for concepts like inheritance and polymorphism.
4. Advantages and Disadvantages
Both procedural and object-oriented programming have their strengths and weaknesses. Let’s explore the advantages and disadvantages of each paradigm:
4.1. Procedural Programming
Advantages:
- Simple and easy to learn for beginners
- Efficient for small to medium-sized programs
- Requires less memory and processing power compared to OOP
- Easier to follow the flow of the program
Disadvantages:
- Difficult to manage and maintain large, complex programs
- Limited code reusability
- Less secure due to global data access
- Harder to model real-world problems and relationships
4.2. Object-Oriented Programming
Advantages:
- Better organization and modularity for large, complex programs
- Improved code reusability through inheritance
- Better data security through encapsulation
- Easier to model real-world problems and relationships
- Supports code extensibility and maintenance
Disadvantages:
- Steeper learning curve, especially for beginners
- Can be overkill for small, simple programs
- May require more memory and processing power
- Can lead to over-engineering if not carefully designed
5. When to Use Each Paradigm
Choosing between procedural and object-oriented programming depends on various factors, including the project’s size, complexity, and requirements. Here are some guidelines on when to use each paradigm:
5.1. Use Procedural Programming When:
- Developing small to medium-sized programs with a clear, linear flow
- Working on projects with limited scope and complexity
- Dealing with straightforward data processing tasks
- Developing scripts or utility programs
- Working with resource-constrained environments
5.2. Use Object-Oriented Programming When:
- Developing large, complex systems
- Modeling real-world objects and their relationships
- Creating reusable and maintainable code
- Working on projects that require frequent updates and extensions
- Developing graphical user interfaces (GUIs)
- Creating frameworks or libraries for other developers to use
6. Real-World Applications and Examples
To further illustrate the differences between procedural and object-oriented programming, let’s look at some real-world applications and examples:
6.1. Procedural Programming Examples
- Data Analysis Scripts: Many data analysis tasks, especially those involving sequential processing of data, are well-suited for procedural programming.
- System Utilities: Command-line tools and system utilities often use procedural programming due to their straightforward nature.
- Embedded Systems: Resource-constrained environments like embedded systems often benefit from the efficiency of procedural programming.
Here’s a simple example of a procedural program for calculating the average of a list of numbers:
def calculate_average(numbers):
total = sum(numbers)
count = len(numbers)
return total / count if count > 0 else 0
# Usage
numbers = [1, 2, 3, 4, 5]
average = calculate_average(numbers)
print(f"The average is: {average}")
6.2. Object-Oriented Programming Examples
- Game Development: Games often use OOP to model game objects, their properties, and behaviors.
- GUI Applications: Graphical user interfaces naturally lend themselves to object-oriented design, with UI elements represented as objects.
- Enterprise Software: Large-scale enterprise applications benefit from the modularity and extensibility of OOP.
Here’s an example of how you might model a simple game character using OOP:
class Character:
def __init__(self, name, health, attack_power):
self.name = name
self.health = health
self.attack_power = attack_power
def attack(self, target):
target.receive_damage(self.attack_power)
print(f"{self.name} attacks {target.name} for {self.attack_power} damage!")
def receive_damage(self, amount):
self.health -= amount
print(f"{self.name} receives {amount} damage. Remaining health: {self.health}")
# Usage
hero = Character("Hero", 100, 20)
enemy = Character("Enemy", 80, 15)
hero.attack(enemy)
enemy.attack(hero)
7. Preparing for Technical Interviews
Understanding the differences between procedural and object-oriented programming is crucial for technical interviews, especially when applying to major tech companies. Here are some tips to help you prepare:
- Know the Fundamentals: Ensure you have a solid grasp of both paradigms, including their key concepts, advantages, and disadvantages.
- Practice Implementation: Be prepared to implement solutions using both paradigms. Practice solving problems using procedural and object-oriented approaches.
- Understand Trade-offs: Be ready to discuss the trade-offs between procedural and OOP in different scenarios. Interviewers often ask about choosing the right approach for specific problems.
- Real-World Examples: Familiarize yourself with real-world applications of both paradigms. Be prepared to discuss how you’ve used them in your projects.
- Design Patterns: For OOP, understand common design patterns and how they solve specific problems.
- Code Organization: Be able to explain how you would organize a large project using either paradigm.
- Performance Considerations: Understand the performance implications of both approaches in different scenarios.
8. Conclusion
Procedural and object-oriented programming are two fundamental paradigms in software development, each with its own strengths and use cases. Procedural programming excels in simplicity and efficiency for smaller, straightforward tasks, while object-oriented programming shines in managing complexity and modeling real-world relationships in larger systems.
As you continue your journey in programming and prepare for technical interviews, it’s essential to be proficient in both paradigms. Understanding when and how to apply each approach will make you a more versatile and effective programmer, capable of choosing the right tool for the job in various scenarios.
Remember, the choice between procedural and object-oriented programming is not always black and white. Many modern programming languages and projects incorporate elements of both paradigms, allowing developers to leverage the strengths of each approach as needed. By mastering both procedural and object-oriented programming, you’ll be well-equipped to tackle a wide range of programming challenges and excel in your coding interviews.