Procedural vs. Functional Programming: Which One to Focus On?
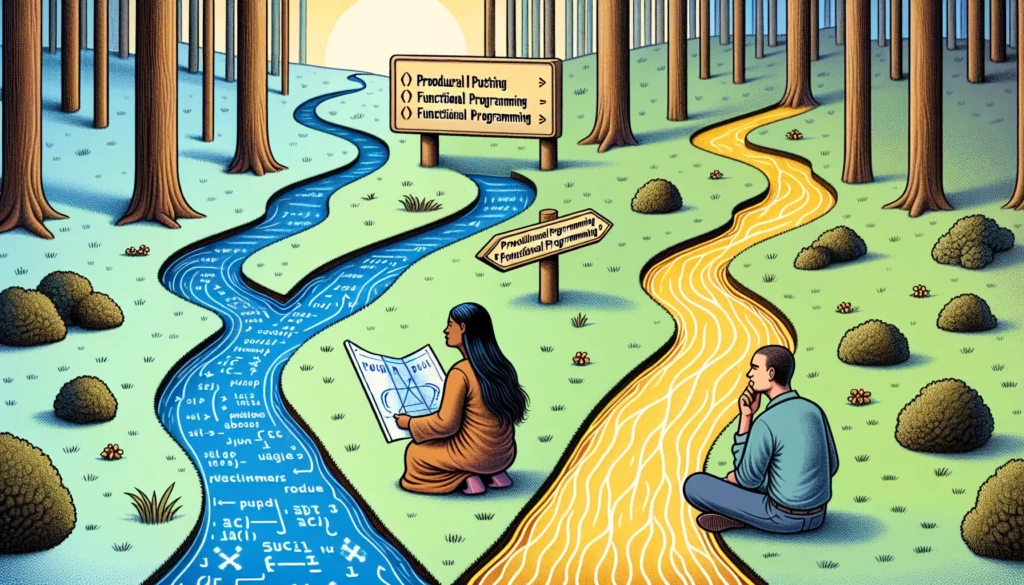
In the ever-evolving world of programming, developers are constantly faced with choices about which paradigms and approaches to adopt. Two prominent programming paradigms that often come up in discussions are procedural programming and functional programming. As aspiring programmers or those looking to expand their skills, it’s crucial to understand these paradigms and determine which one to focus on. In this comprehensive guide, we’ll explore the intricacies of procedural and functional programming, compare their strengths and weaknesses, and help you decide which one aligns best with your goals and the demands of the industry.
Understanding Procedural Programming
Procedural programming is one of the oldest and most widely used programming paradigms. It’s based on the concept of procedure calls, where the program is divided into procedures (also known as routines or functions) that perform specific tasks.
Key Characteristics of Procedural Programming:
- Sequential Execution: Programs are executed in a step-by-step manner, following a predetermined sequence of instructions.
- Modularity: Code is organized into procedures or functions, promoting reusability and easier maintenance.
- Use of Variables: Data is stored in variables that can be modified throughout the program’s execution.
- Top-Down Approach: Problems are broken down into smaller, manageable sub-problems.
- Imperative Nature: Programs explicitly state how to achieve a goal through a series of commands.
Advantages of Procedural Programming:
- Simplicity: Procedural programming is often easier for beginners to grasp due to its straightforward, step-by-step approach.
- Efficiency: It can be highly efficient for solving certain types of problems, especially those that follow a linear sequence of steps.
- Memory Management: Procedural languages often provide more direct control over memory allocation and deallocation.
- Wide Adoption: Many popular languages support procedural programming, making it a versatile skill to have.
Disadvantages of Procedural Programming:
- Limited Abstraction: It can be challenging to represent complex real-world entities and relationships.
- Code Reusability: While procedures promote some reusability, it’s not as extensive as in object-oriented or functional programming.
- Scalability Issues: As programs grow larger, maintaining and debugging procedural code can become more difficult.
- Side Effects: Functions can modify global variables, potentially leading to unexpected behavior and harder-to-track bugs.
Example of Procedural Programming:
Let’s look at a simple example of procedural programming in Python:
def calculate_area(length, width):
return length * width
def calculate_perimeter(length, width):
return 2 * (length + width)
def main():
length = 5
width = 3
area = calculate_area(length, width)
perimeter = calculate_perimeter(length, width)
print(f"Area: {area}")
print(f"Perimeter: {perimeter}")
if __name__ == "__main__":
main()
In this example, we have separate functions for calculating area and perimeter, and a main function that uses these procedures to compute and display the results.
Understanding Functional Programming
Functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It emphasizes the application of functions to inputs to produce outputs without modifying state.
Key Characteristics of Functional Programming:
- Immutability: Data is immutable, meaning once it’s created, it cannot be changed.
- Pure Functions: Functions always produce the same output for the same input and have no side effects.
- First-Class and Higher-Order Functions: Functions can be assigned to variables, passed as arguments, and returned from other functions.
- Recursion: Iteration is typically achieved through recursion rather than loops.
- Declarative Style: Programs focus on what to do rather than how to do it.
Advantages of Functional Programming:
- Predictability: Pure functions and immutability lead to more predictable and easier-to-test code.
- Concurrency: The absence of shared state makes it easier to write concurrent and parallel programs.
- Modularity: Functions are highly modular and composable, promoting code reuse and maintainability.
- Mathematical Rigor: Functional programming is based on mathematical concepts, making it suitable for complex algorithmic problems.
Disadvantages of Functional Programming:
- Learning Curve: The concepts can be challenging for programmers accustomed to imperative styles.
- Performance Overhead: Immutability and recursion can sometimes lead to increased memory usage and slower execution.
- Limited I/O and State Management: Handling I/O operations and maintaining state can be more complex in purely functional languages.
- Less Intuitive for Some Tasks: Certain problems that involve state changes may be more naturally expressed in imperative styles.
Example of Functional Programming:
Here’s an example of functional programming in Python:
from functools import reduce
# Pure function to calculate the sum of squares
def sum_of_squares(numbers):
return sum(map(lambda x: x**2, numbers))
# Pure function to filter even numbers
def filter_even(numbers):
return list(filter(lambda x: x % 2 == 0, numbers))
# Higher-order function to apply a function twice
def apply_twice(f, x):
return f(f(x))
# Main function using functional concepts
def main():
numbers = [1, 2, 3, 4, 5]
# Using map and lambda
squared_numbers = list(map(lambda x: x**2, numbers))
print("Squared numbers:", squared_numbers)
# Using filter
even_numbers = filter_even(numbers)
print("Even numbers:", even_numbers)
# Using reduce
product = reduce(lambda x, y: x * y, numbers)
print("Product of numbers:", product)
# Using higher-order function
double_square = apply_twice(lambda x: x**2, 2)
print("Double square of 2:", double_square)
if __name__ == "__main__":
main()
This example demonstrates various functional programming concepts such as pure functions, higher-order functions, and the use of map, filter, and reduce operations.
Comparing Procedural and Functional Programming
Now that we’ve explored both paradigms, let’s compare them across several key aspects:
1. Code Organization
Procedural: Organizes code into procedures or functions that operate on data.
Functional: Organizes code around the evaluation of functions and the avoidance of state changes.
2. State Management
Procedural: Relies on changing state and mutable data.
Functional: Emphasizes immutability and avoids changing state.
3. Control Flow
Procedural: Uses loops and conditional statements for control flow.
Functional: Relies more on recursion and higher-order functions.
4. Side Effects
Procedural: Functions can have side effects, modifying variables outside their scope.
Functional: Emphasizes pure functions without side effects.
5. Ease of Learning
Procedural: Generally easier for beginners to grasp due to its straightforward nature.
Functional: Can have a steeper learning curve, especially for those used to imperative styles.
6. Performance
Procedural: Often provides good performance, especially for linear, step-by-step problems.
Functional: Can have performance overhead due to immutability and recursion, but enables easier parallelization.
7. Debugging and Testing
Procedural: Debugging can become complex in large programs due to state changes.
Functional: Easier to debug and test due to pure functions and immutability.
8. Concurrency
Procedural: Handling concurrency can be challenging due to shared state.
Functional: Better suited for concurrent programming due to the absence of shared mutable state.
Which One Should You Focus On?
The choice between procedural and functional programming isn’t always a matter of picking one over the other. In many cases, the best approach is to understand both paradigms and use them where they’re most appropriate. However, if you’re looking to prioritize your learning, consider the following factors:
1. Your Background and Learning Style
If you’re new to programming or come from a background in mathematics, you might find functional programming more intuitive. On the other hand, if you prefer a step-by-step approach to problem-solving, procedural programming might be a better starting point.
2. Project Requirements
Consider the types of projects you’ll be working on. Procedural programming might be more suitable for straightforward, linear tasks, while functional programming excels in handling complex data transformations and parallel processing.
3. Industry Trends
While procedural programming is still widely used, there’s a growing trend towards functional programming in many areas of software development, particularly in big data processing, AI, and web development.
4. Language Ecosystem
Look at the programming languages you’re interested in or required to use. Some languages, like C, are primarily procedural, while others, like Haskell, are purely functional. Many modern languages, such as Python, JavaScript, and Scala, support both paradigms.
5. Career Goals
If you’re aiming for a career in data science, AI, or backend development for scalable systems, focusing on functional programming might give you an edge. For system programming or embedded systems, procedural programming might be more relevant.
6. Personal Interest
Ultimately, your personal interest and enjoyment should play a role. If you find one paradigm more engaging or satisfying to work with, it might be worth focusing on that one first.
Practical Approach: Learning Both
In practice, many successful programmers are proficient in both procedural and functional programming. Here’s a suggested approach to learning both:
- Start with Procedural: Begin with procedural programming as it’s often easier to grasp and is fundamental to understanding how computers execute instructions.
- Introduce Functional Concepts: Gradually introduce functional programming concepts, such as pure functions and immutability.
- Practice in a Multi-Paradigm Language: Use a language like Python or JavaScript that supports both paradigms to practice applying each approach to different problems.
- Study Functional Programming in Depth: Once comfortable with basic programming concepts, dive deeper into functional programming to understand its benefits and use cases.
- Apply Both in Projects: Work on projects that allow you to use both paradigms, learning when each is most appropriate.
Conclusion
Both procedural and functional programming have their strengths and are valuable skills in a programmer’s toolkit. Procedural programming offers simplicity and efficiency for many common programming tasks, while functional programming provides powerful tools for managing complexity, ensuring code correctness, and tackling certain types of problems more elegantly.
In the context of coding education and preparing for technical interviews, especially for major tech companies, it’s beneficial to have a strong foundation in both paradigms. Procedural programming will help you understand fundamental programming concepts and solve many algorithmic problems efficiently. Functional programming, on the other hand, will enhance your ability to write clean, maintainable code and tackle complex data processing tasks.
As you progress in your coding journey, strive to understand the principles behind both paradigms and learn to apply them judiciously based on the problem at hand. This versatility will not only make you a more effective programmer but also prepare you for the diverse challenges you’ll face in your career, whether you’re building large-scale applications, working on data-intensive projects, or solving intricate algorithmic problems in technical interviews.
Remember, the goal is not to choose one paradigm exclusively, but to expand your programming repertoire and problem-solving skills. By mastering both procedural and functional programming, you’ll be well-equipped to tackle a wide range of programming challenges and adapt to the ever-changing landscape of software development.