Problem-Solving Strategies for New Programmers: A Comprehensive Guide
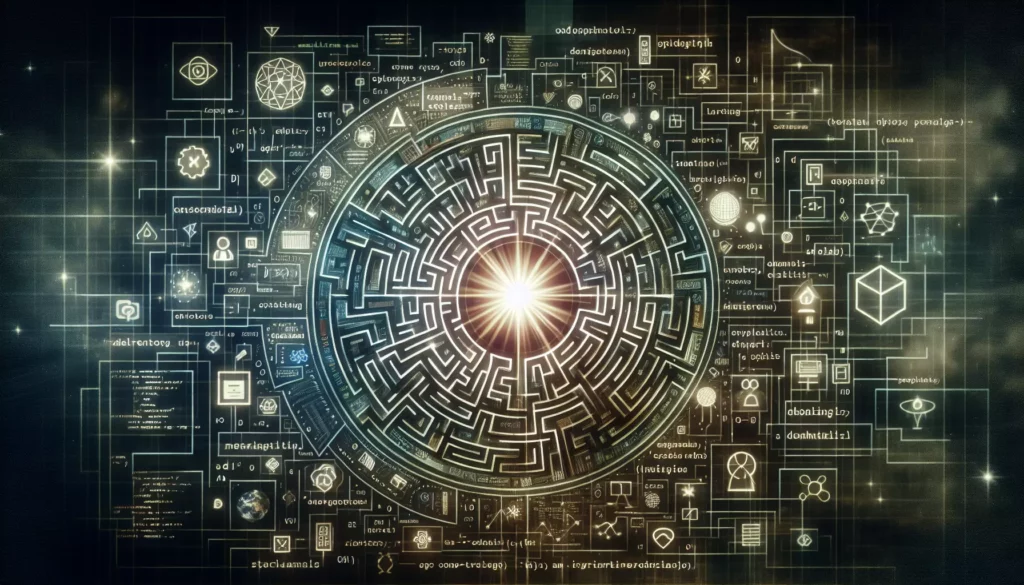
As a new programmer, one of the most crucial skills you’ll need to develop is problem-solving. Whether you’re tackling a coding challenge, debugging an application, or designing a new feature, your ability to approach problems systematically and creatively will be paramount to your success. In this comprehensive guide, we’ll explore various problem-solving strategies that can help you overcome obstacles and become a more efficient and effective programmer.
1. Understanding the Problem
Before diving into any coding, it’s essential to fully understand the problem at hand. This step is often overlooked by eager beginners, but it’s crucial for developing an effective solution.
Key steps for understanding the problem:
- Read the problem statement carefully, multiple times if necessary.
- Identify the inputs and expected outputs.
- List any constraints or special conditions mentioned.
- Restate the problem in your own words to ensure comprehension.
- Ask clarifying questions if anything is unclear.
By thoroughly understanding the problem, you’ll be better equipped to devise an appropriate solution and avoid wasting time on incorrect approaches.
2. Breaking Down the Problem
Large, complex problems can be overwhelming. A key strategy is to break them down into smaller, more manageable sub-problems.
Techniques for breaking down problems:
- Identify the main components or steps required to solve the problem.
- Create a flowchart or diagram to visualize the problem and its parts.
- Use pseudocode to outline the general structure of your solution.
- Tackle one sub-problem at a time, ensuring each part works before moving on.
Breaking down problems not only makes them less daunting but also allows you to focus on solving smaller, more manageable tasks.
3. Planning Your Approach
Once you understand the problem and have broken it down, it’s time to plan your approach. This involves choosing appropriate algorithms, data structures, and coding techniques to solve the problem efficiently.
Steps for planning your approach:
- Consider multiple potential solutions and evaluate their pros and cons.
- Think about the time and space complexity of different approaches.
- Choose the most suitable data structures for the problem (e.g., arrays, linked lists, trees, graphs).
- Decide on the programming paradigm to use (e.g., procedural, object-oriented, functional).
- Outline the main functions or classes you’ll need to implement.
A well-thought-out plan can save you time and frustration during the implementation phase.
4. Implementing the Solution
With a solid plan in place, you can begin implementing your solution. This is where your coding skills come into play.
Best practices for implementation:
- Start with a simple, working solution before optimizing.
- Write clean, readable code with proper indentation and meaningful variable names.
- Comment your code to explain complex logic or important decisions.
- Implement one feature or function at a time, testing as you go.
- Use version control (e.g., Git) to track changes and revert if necessary.
Remember, your first attempt doesn’t need to be perfect. Focus on getting a working solution, then refine and optimize it later.
5. Testing and Debugging
Once you have an initial implementation, it’s crucial to test it thoroughly and debug any issues that arise.
Effective testing and debugging strategies:
- Start with small, simple test cases and gradually increase complexity.
- Use edge cases and boundary conditions to test your solution’s robustness.
- Implement unit tests for individual functions or components.
- Use debugging tools and techniques like print statements, breakpoints, and step-through debugging.
- When encountering bugs, isolate the problem and reproduce it consistently before attempting to fix it.
Thorough testing and effective debugging are essential skills that will save you time and headaches in the long run.
6. Optimizing and Refactoring
After your solution is working correctly, you may want to optimize it for better performance or refactor it for improved readability and maintainability.
Optimization and refactoring techniques:
- Analyze your code for time and space complexity improvements.
- Look for redundant calculations or operations that can be eliminated.
- Consider using more efficient algorithms or data structures.
- Refactor code to improve readability and reduce duplication.
- Apply design patterns where appropriate to enhance code structure.
Remember that premature optimization can lead to unnecessary complexity. Focus on correctness first, then optimize if needed.
7. Learning from Your Solution
After solving a problem, take time to reflect on your approach and learn from the experience.
Reflection questions to ask yourself:
- What worked well in your solution?
- What challenges did you face, and how did you overcome them?
- Are there alternative approaches you could have taken?
- What new concepts or techniques did you learn while solving this problem?
- How can you apply what you’ve learned to future problems?
By reflecting on your problem-solving process, you’ll continually improve your skills and become a more effective programmer.
8. Utilizing Resources and Seeking Help
As a new programmer, it’s important to recognize that you don’t need to solve every problem entirely on your own. Knowing how to effectively use resources and seek help is a valuable skill in itself.
Strategies for utilizing resources and getting help:
- Consult documentation and official language or library references.
- Search for similar problems and solutions online (e.g., Stack Overflow, GitHub).
- Join coding communities and forums to ask questions and share knowledge.
- Pair program with a more experienced developer to learn new techniques.
- Use AI-powered coding assistants for suggestions and explanations.
Remember, seeking help is not a sign of weakness but a smart way to learn and grow as a programmer.
9. Practicing Regularly
Like any skill, problem-solving in programming improves with practice. Regular, consistent practice is key to becoming a proficient problem-solver.
Ways to practice problem-solving:
- Solve coding challenges on platforms like LeetCode, HackerRank, or CodeWars.
- Participate in coding competitions or hackathons.
- Work on personal projects that challenge you to solve real-world problems.
- Contribute to open-source projects to tackle diverse challenges.
- Recreate features from existing applications to understand how they work.
Consistent practice will help you build a repertoire of problem-solving techniques and improve your ability to tackle new challenges.
10. Developing a Growth Mindset
Finally, cultivating a growth mindset is crucial for long-term success in programming and problem-solving.
Key aspects of a growth mindset for programmers:
- Embrace challenges as opportunities to learn and grow.
- View mistakes and failures as valuable learning experiences.
- Persist in the face of setbacks and difficulties.
- Be open to feedback and constructive criticism.
- Celebrate the success of others and learn from their approaches.
With a growth mindset, you’ll be better equipped to handle the ups and downs of programming and continue improving your problem-solving skills.
Practical Example: Solving a Coding Problem
Let’s walk through a simple coding problem to illustrate how these problem-solving strategies can be applied in practice.
Problem Statement:
Write a function that takes an array of integers and returns the two numbers that add up to a given target sum. If no such pair exists, return an empty array.
1. Understanding the Problem:
- Input: An array of integers and a target sum
- Output: An array containing two numbers that add up to the target sum, or an empty array if no such pair exists
- Constraints: The array may contain positive, negative, or zero values
2. Breaking Down the Problem:
- Iterate through the array
- For each number, check if there’s another number that adds up to the target sum
- If found, return the pair
- If not found after checking all pairs, return an empty array
3. Planning the Approach:
We can use a hash table to store the complement of each number as we iterate through the array. This will allow us to find pairs in O(n) time complexity.
4. Implementing the Solution:
Here’s a Python implementation of the solution:
def find_pair_sum(arr, target_sum):
complement_dict = {}
for num in arr:
complement = target_sum - num
if complement in complement_dict:
return [complement, num]
complement_dict[num] = True
return []
# Test the function
print(find_pair_sum([3, 5, 2, -4, 8, 11], 7)) # Expected output: [5, 2]
print(find_pair_sum([1, 2, 3, 4], 10)) # Expected output: []
5. Testing and Debugging:
We’ve included two test cases in the implementation. You should test with more cases, including edge cases like an empty array, arrays with negative numbers, and cases where no pair exists.
6. Optimizing and Refactoring:
The current solution is already optimized for time complexity (O(n)). However, we could potentially optimize for space by using a two-pointer approach if the array is sorted.
7. Learning from the Solution:
This problem demonstrates the usefulness of hash tables for efficient lookups and how we can trade space complexity for improved time complexity.
Conclusion
Problem-solving is a fundamental skill for programmers, and it’s one that improves with practice and experience. By following these strategies – understanding the problem, breaking it down, planning your approach, implementing a solution, testing and debugging, optimizing and refactoring, learning from your experiences, utilizing resources, practicing regularly, and maintaining a growth mindset – you’ll be well-equipped to tackle a wide range of programming challenges.
Remember that becoming a proficient problem-solver takes time and patience. Don’t get discouraged if you encounter difficult problems; each challenge is an opportunity to learn and grow. Keep practicing, stay curious, and never stop learning. With persistence and the right approach, you’ll continually improve your problem-solving skills and become a more confident and capable programmer.
As you continue your journey in programming, consider using platforms like AlgoCademy that provide interactive coding tutorials, resources for learners, and tools to help you progress from beginner-level coding to preparing for technical interviews. These platforms can offer valuable guidance and practice in algorithmic thinking and problem-solving, which are essential skills for success in the tech industry.
Happy coding, and may your problem-solving skills continue to grow and flourish!