Prim’s Algorithm: A Comprehensive Guide to Minimum Spanning Trees
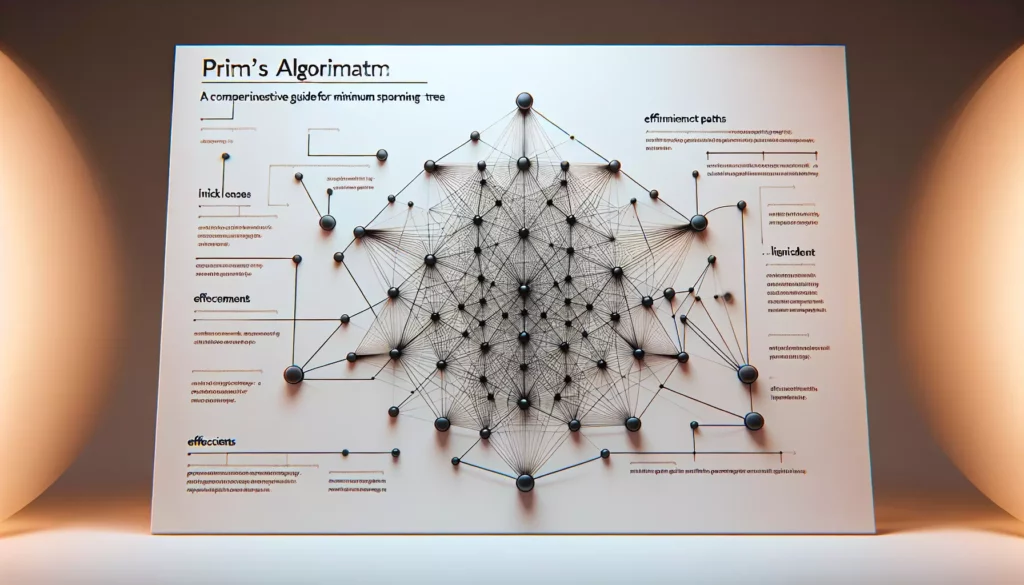
In the world of computer science and graph theory, efficient algorithms play a crucial role in solving complex problems. One such algorithm that has stood the test of time is Prim’s algorithm, a powerful tool for finding the minimum spanning tree (MST) of a weighted, undirected graph. In this comprehensive guide, we’ll dive deep into Prim’s algorithm, exploring its concepts, implementation, and real-world applications.
Table of Contents
- Introduction to Minimum Spanning Trees
- Understanding Prim’s Algorithm
- How Prim’s Algorithm Works
- Implementing Prim’s Algorithm
- Time Complexity Analysis
- Comparison with Kruskal’s Algorithm
- Real-world Applications
- Optimizations and Variations
- Practice Problems and Exercises
- Conclusion
1. Introduction to Minimum Spanning Trees
Before we delve into Prim’s algorithm, it’s essential to understand the concept of a minimum spanning tree (MST). A spanning tree of a graph is a subgraph that includes all vertices of the original graph and is a tree (i.e., it has no cycles). A minimum spanning tree is a spanning tree with the minimum possible total edge weight.
Key properties of a minimum spanning tree:
- It connects all vertices in the graph.
- It contains no cycles.
- It has the minimum total edge weight among all possible spanning trees.
Minimum spanning trees have numerous applications in various fields, including network design, clustering algorithms, and approximation algorithms for NP-hard problems.
2. Understanding Prim’s Algorithm
Prim’s algorithm, developed by computer scientist Robert C. Prim in 1957, is a greedy algorithm that finds a minimum spanning tree for a weighted, undirected graph. The algorithm operates by building the MST one vertex at a time, starting from an arbitrary root node and at each step adding the lowest-weight edge that connects a vertex in the growing MST to a vertex outside it.
Key characteristics of Prim’s algorithm:
- It is a greedy algorithm, making locally optimal choices at each step.
- It grows a single tree from a starting vertex, expanding it until all vertices are included.
- It maintains a set of vertices already included in the MST and a set of edges that cross the cut between included and excluded vertices.
3. How Prim’s Algorithm Works
Let’s break down the steps of Prim’s algorithm to understand its inner workings:
- Initialization:
- Choose an arbitrary starting vertex and add it to the MST.
- Initialize a priority queue to store edges and their weights.
- Add all edges connected to the starting vertex to the priority queue.
- Main Loop:
- While the priority queue is not empty and the MST doesn’t include all vertices:
- Extract the edge with the minimum weight from the priority queue.
- If the edge connects to a vertex not yet in the MST:
- Add the edge to the MST.
- Add the new vertex to the MST.
- Add all edges connected to the new vertex to the priority queue (if their other endpoint is not in the MST).
- Termination:
- The algorithm terminates when all vertices are included in the MST or the priority queue is empty.
This process ensures that at each step, we add the lowest-weight edge that connects the growing MST to a new vertex, ultimately resulting in the minimum spanning tree for the entire graph.
4. Implementing Prim’s Algorithm
Now that we understand how Prim’s algorithm works, let’s implement it in Python. We’ll use a priority queue to efficiently manage the edges and their weights.
import heapq
def prims_algorithm(graph):
# Initialize variables
mst = []
visited = set()
start_vertex = list(graph.keys())[0]
edges = [(weight, start_vertex, to) for to, weight in graph[start_vertex].items()]
heapq.heapify(edges)
while edges:
weight, frm, to = heapq.heappop(edges)
if to not in visited:
visited.add(to)
mst.append((frm, to, weight))
for next_to, next_weight in graph[to].items():
if next_to not in visited:
heapq.heappush(edges, (next_weight, to, next_to))
return mst
# Example usage
graph = {
'A': {'B': 2, 'C': 3},
'B': {'A': 2, 'C': 1, 'D': 1},
'C': {'A': 3, 'B': 1, 'D': 4},
'D': {'B': 1, 'C': 4}
}
minimum_spanning_tree = prims_algorithm(graph)
print("Minimum Spanning Tree:", minimum_spanning_tree)
In this implementation:
- We use a min-heap (priority queue) to efficiently extract the edge with the minimum weight.
- The
visited
set keeps track of vertices already included in the MST. - We start with an arbitrary vertex and add all its edges to the priority queue.
- In each iteration, we extract the minimum-weight edge and add it to the MST if it connects to an unvisited vertex.
- We then add all edges from the newly added vertex to the priority queue.
- The process continues until all vertices are visited or the priority queue is empty.
5. Time Complexity Analysis
The time complexity of Prim’s algorithm depends on the data structures used for implementation. Let’s analyze the time complexity for different implementations:
Using an Array
If we use an array to store vertices and perform a linear search to find the minimum-weight edge, the time complexity would be O(V^2), where V is the number of vertices. This implementation is suitable for dense graphs.
Using a Binary Heap
With a binary heap (priority queue), as in our implementation, the time complexity improves to O((V + E) log V), where E is the number of edges. This is because:
- Each vertex is added and removed from the heap once: O(V log V)
- Each edge may cause an update in the heap: O(E log V)
Using a Fibonacci Heap
For very large graphs, using a Fibonacci heap can further improve the time complexity to O(E + V log V). However, Fibonacci heaps are complex to implement and may not provide significant practical benefits for most applications.
The space complexity of Prim’s algorithm is O(V), as we need to store information for each vertex in the graph.
6. Comparison with Kruskal’s Algorithm
Prim’s algorithm is not the only method for finding a minimum spanning tree. Another popular algorithm is Kruskal’s algorithm. Let’s compare these two approaches:
Prim’s Algorithm:
- Builds the MST by adding vertices.
- Starts with a single vertex and grows the tree.
- Efficient for dense graphs.
- Time complexity: O((V + E) log V) with a binary heap.
Kruskal’s Algorithm:
- Builds the MST by adding edges.
- Sorts all edges and adds them in order of increasing weight.
- Efficient for sparse graphs.
- Time complexity: O(E log E) or O(E log V).
The choice between Prim’s and Kruskal’s algorithms often depends on the graph’s characteristics and the specific requirements of the problem at hand.
7. Real-world Applications
Prim’s algorithm and minimum spanning trees have numerous practical applications across various domains:
Network Design
In designing computer networks, telecommunication networks, or transportation systems, MSTs can help minimize the total cost of connections while ensuring all nodes are reachable.
Cluster Analysis
MSTs can be used in clustering algorithms, such as the Single-linkage clustering method, to group similar data points together.
Image Segmentation
In computer vision, MSTs can be applied to segment images by treating pixels as vertices and using color or intensity differences as edge weights.
Approximation Algorithms
MSTs are used in approximation algorithms for NP-hard problems, such as the Traveling Salesman Problem and the Steiner Tree Problem.
Circuit Design
In VLSI circuit design, MSTs can help optimize the layout of components to minimize wire length and power consumption.
8. Optimizations and Variations
While the basic Prim’s algorithm is powerful, several optimizations and variations can enhance its performance or adapt it to specific scenarios:
Lazy Prim’s Algorithm
This variation reduces the number of decrease-key operations in the priority queue, potentially improving performance for sparse graphs.
Borůvka’s Algorithm
This algorithm can be seen as a parallel version of Prim’s algorithm, growing multiple trees simultaneously. It’s particularly useful for distributed computing environments.
Chazelle’s Algorithm
This sophisticated variation achieves a near-linear time complexity of O(E α(E, V)), where α is the inverse Ackermann function, which grows extremely slowly.
Randomized Algorithms
Randomized versions of Prim’s algorithm can provide good average-case performance and simplify the implementation.
9. Practice Problems and Exercises
To solidify your understanding of Prim’s algorithm and minimum spanning trees, try solving these practice problems:
- Basic Implementation: Implement Prim’s algorithm from scratch without using any libraries.
- Visualization: Create a program that visualizes the step-by-step process of Prim’s algorithm on a given graph.
- Weighted Graph Generator: Develop a function that generates random weighted graphs and test your Prim’s algorithm implementation on them.
- Maximum Spanning Tree: Modify Prim’s algorithm to find the maximum spanning tree instead of the minimum.
- K-MST Problem: Implement a variation of Prim’s algorithm to find the minimum spanning tree that includes exactly K vertices.
- Prim vs. Kruskal: Implement both Prim’s and Kruskal’s algorithms and compare their performance on various types of graphs.
- Distributed Prim’s Algorithm: Design a distributed version of Prim’s algorithm that can work across multiple machines.
These exercises will help you gain a deeper understanding of the algorithm and its applications in various scenarios.
10. Conclusion
Prim’s algorithm is a fundamental tool in graph theory and computer science, offering an elegant solution to the minimum spanning tree problem. Its simplicity, efficiency, and wide range of applications make it an essential algorithm for any programmer or computer scientist to master.
As we’ve explored in this comprehensive guide, Prim’s algorithm showcases the power of greedy approaches in solving complex problems. By understanding its mechanics, implementation details, and various optimizations, you’re now equipped to tackle a wide range of graph-related challenges.
Remember that while Prim’s algorithm is powerful, it’s just one tool in the vast toolkit of graph algorithms. Depending on the specific problem and graph characteristics, other algorithms like Kruskal’s or more specialized variations might be more suitable. The key is to understand the strengths and limitations of each approach and choose the right tool for the job.
As you continue your journey in algorithm design and problem-solving, keep exploring and practicing with different graph problems. The insights gained from understanding Prim’s algorithm will serve you well in tackling more advanced topics in computer science and preparing for technical interviews at top tech companies.
Happy coding, and may your spanning trees always be minimal!