Preparing for SQL and Database Questions in Interviews: A Comprehensive Guide
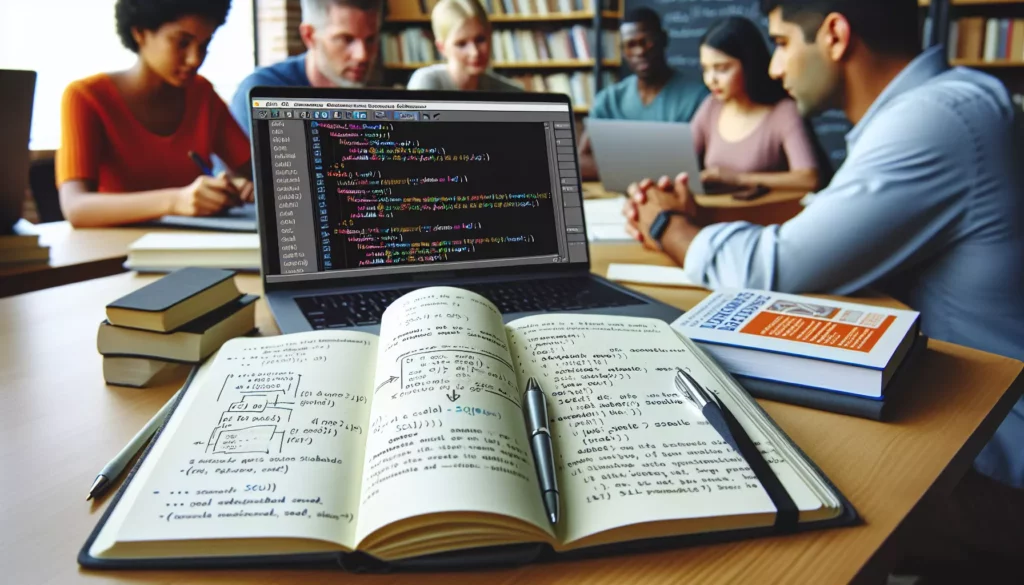
In today’s tech-driven world, mastering SQL and database concepts is crucial for aspiring developers and data professionals. Whether you’re aiming for a position at a FAANG company or any other tech organization, being well-prepared for SQL and database questions can significantly boost your chances of success in technical interviews. This comprehensive guide will walk you through the essential aspects of SQL and database preparation, providing you with the knowledge and confidence needed to excel in your interviews.
1. Understanding the Importance of SQL and Database Knowledge
Before diving into the preparation strategies, it’s essential to grasp why SQL and database skills are so valuable in the tech industry:
- Data-Driven Decision Making: Companies rely heavily on data to make informed decisions, and SQL is the primary language for querying and manipulating this data.
- Scalability: As businesses grow, their data needs expand, making efficient database management crucial for maintaining performance and reliability.
- Integration: Many applications and systems interact with databases, making SQL knowledge essential for developers across various domains.
- Analytics: SQL is a fundamental tool for data analysis, helping businesses extract insights from their vast data repositories.
2. Core SQL Concepts to Master
To prepare effectively for SQL interview questions, focus on mastering these core concepts:
2.1. Basic SQL Queries
Ensure you’re comfortable with fundamental SQL operations:
- SELECT statements
- WHERE clauses
- ORDER BY and GROUP BY
- JOINs (INNER, LEFT, RIGHT, FULL OUTER)
- Aggregate functions (COUNT, SUM, AVG, MAX, MIN)
Example query:
SELECT department, AVG(salary) as avg_salary
FROM employees
JOIN departments ON employees.dept_id = departments.id
GROUP BY department
HAVING AVG(salary) > 50000
ORDER BY avg_salary DESC;
2.2. Subqueries and Nested Queries
Understanding how to use subqueries can help you solve complex problems efficiently:
SELECT employee_name
FROM employees
WHERE salary > (SELECT AVG(salary) FROM employees);
2.3. Window Functions
Window functions are powerful tools for performing calculations across sets of rows:
SELECT
employee_name,
department,
salary,
RANK() OVER (PARTITION BY department ORDER BY salary DESC) as salary_rank
FROM employees;
2.4. Common Table Expressions (CTEs)
CTEs can help you write more readable and maintainable queries:
WITH high_earners AS (
SELECT * FROM employees WHERE salary > 100000
)
SELECT department, COUNT(*) as high_earner_count
FROM high_earners
GROUP BY department;
2.5. ACID Properties
Understand the ACID properties of database transactions:
- Atomicity: Transactions are all-or-nothing operations.
- Consistency: Transactions bring the database from one valid state to another.
- Isolation: Concurrent transactions should not interfere with each other.
- Durability: Once a transaction is committed, it remains so.
3. Database Design Principles
Interviewers often assess candidates’ understanding of database design concepts:
3.1. Normalization
Be familiar with the normal forms (1NF, 2NF, 3NF, BCNF) and their purposes in reducing data redundancy and improving data integrity.
3.2. Entity-Relationship Diagrams (ERDs)
Practice creating and interpreting ERDs to represent database structures visually.
3.3. Indexing
Understand the benefits and trade-offs of using indexes to improve query performance.
3.4. Constraints
Know how to use various constraints (PRIMARY KEY, FOREIGN KEY, UNIQUE, CHECK) to enforce data integrity.
4. Performance Optimization
Optimizing database performance is a critical skill that interviewers often evaluate:
4.1. Query Optimization
Learn techniques for improving query performance:
- Using appropriate indexes
- Avoiding wildcard characters at the beginning of LIKE patterns
- Utilizing EXPLAIN to analyze query execution plans
4.2. Denormalization
Understand when and how to denormalize data for performance gains, weighing the trade-offs against data integrity.
4.3. Caching Strategies
Familiarize yourself with caching mechanisms to reduce database load and improve response times.
5. NoSQL Databases
While SQL databases are prevalent, knowledge of NoSQL databases is increasingly valuable:
5.1. Types of NoSQL Databases
- Document stores (e.g., MongoDB)
- Key-value stores (e.g., Redis)
- Column-family stores (e.g., Cassandra)
- Graph databases (e.g., Neo4j)
5.2. CAP Theorem
Understand the CAP theorem and its implications for distributed database systems:
- Consistency: All nodes see the same data at the same time.
- Availability: Every request receives a response.
- Partition tolerance: The system continues to operate despite network partitions.
6. Practical Interview Preparation Strategies
To excel in SQL and database interviews, incorporate these practical strategies into your preparation:
6.1. Solve Real-World Problems
Practice solving realistic database scenarios. Websites like LeetCode, HackerRank, and SQLZoo offer a wide range of SQL problems to tackle.
6.2. Create Your Own Database Projects
Design and implement a database for a hypothetical application. This hands-on experience will deepen your understanding of database concepts and provide talking points during interviews.
6.3. Study Database Systems
Familiarize yourself with popular database management systems like MySQL, PostgreSQL, or Oracle. Understand their unique features and how they differ from one another.
6.4. Review Company-Specific Technologies
Research the database technologies used by the companies you’re interviewing with. This knowledge can help you tailor your preparation and demonstrate your genuine interest in the role.
6.5. Practice Explaining Your Thought Process
When solving SQL problems, practice verbalizing your thought process. This skill is crucial during interviews, as interviewers are often more interested in your problem-solving approach than the final solution itself.
7. Common SQL Interview Questions and How to Approach Them
Here are some frequently asked SQL interview questions and tips on how to tackle them:
7.1. “Write a query to find the second highest salary in the employees table.”
Approach: This question tests your understanding of subqueries and ranking functions. You could use a subquery with LIMIT and OFFSET, or a window function like DENSE_RANK().
SELECT DISTINCT salary
FROM (
SELECT salary, DENSE_RANK() OVER (ORDER BY salary DESC) as rank
FROM employees
) ranked_salaries
WHERE rank = 2;
7.2. “How would you delete duplicate records from a table?”
Approach: This question assesses your ability to handle data cleaning tasks. You could use a CTE or a subquery to identify and remove duplicates.
WITH duplicates AS (
SELECT *, ROW_NUMBER() OVER (PARTITION BY column1, column2 ORDER BY id) as row_num
FROM table_name
)
DELETE FROM duplicates WHERE row_num > 1;
7.3. “Explain the difference between UNION and UNION ALL.”
Approach: This tests your understanding of set operations. Explain that UNION removes duplicates while UNION ALL doesn’t, making UNION ALL faster but potentially including duplicate rows.
7.4. “How would you optimize a slow-running query?”
Approach: Discuss various optimization techniques such as:
- Analyzing the query execution plan using EXPLAIN
- Adding appropriate indexes
- Rewriting the query to use more efficient joins or subqueries
- Considering partitioning for large tables
8. Advanced Topics to Consider
For more senior positions or specialized roles, you might encounter questions on advanced database topics:
8.1. Database Sharding
Understand the concept of sharding and its implementation strategies for handling large-scale databases.
8.2. Replication and High Availability
Be prepared to discuss various replication models (master-slave, multi-master) and strategies for ensuring database high availability.
8.3. Database Security
Familiarize yourself with database security best practices, including:
- User authentication and authorization
- Encryption of sensitive data
- SQL injection prevention
8.4. Big Data Technologies
For data-intensive roles, have a basic understanding of big data technologies like Hadoop, Spark, and their integration with traditional databases.
9. Behavioral Aspects of Database Interviews
Remember that technical knowledge is just one aspect of a successful interview. Be prepared to discuss:
9.1. Past Projects
Have examples ready of database projects you’ve worked on, challenges you’ve faced, and how you overcame them.
9.2. Teamwork and Communication
Be ready to discuss how you’ve collaborated with team members on database-related tasks and how you communicate complex database concepts to non-technical stakeholders.
9.3. Continuous Learning
Demonstrate your commitment to staying updated with the latest database technologies and best practices.
10. Final Tips for Interview Success
As you prepare for your SQL and database interviews, keep these final tips in mind:
- Stay Calm: Take a deep breath and approach problems methodically.
- Ask Clarifying Questions: If a question is unclear, don’t hesitate to ask for more details or clarification.
- Think Aloud: Share your thought process as you work through problems.
- Practice Whiteboarding: Some interviews may require you to write queries or design schemas on a whiteboard.
- Review Your Basics: Even if you’re experienced, make sure you have a solid grasp of fundamental concepts.
- Be Honest: If you don’t know something, admit it and explain how you would go about finding the answer.
Conclusion
Preparing for SQL and database interviews can be challenging, but with a structured approach and consistent practice, you can significantly improve your chances of success. Remember that interviewers are not just looking for technical proficiency but also for problem-solving skills, the ability to learn quickly, and how well you can explain complex concepts.
By mastering the core SQL concepts, understanding database design principles, and familiarizing yourself with performance optimization techniques, you’ll be well-equipped to handle a wide range of interview questions. Don’t forget to supplement your technical preparation with real-world projects and behavioral interview practice.
As you continue your journey in the world of databases, keep in mind that learning is an ongoing process. The field is constantly evolving, and staying curious and adaptable will serve you well throughout your career. Good luck with your interviews, and may your queries always return the results you expect!