Preparing for Interviews as a Self-Taught Programmer: A Comprehensive Guide
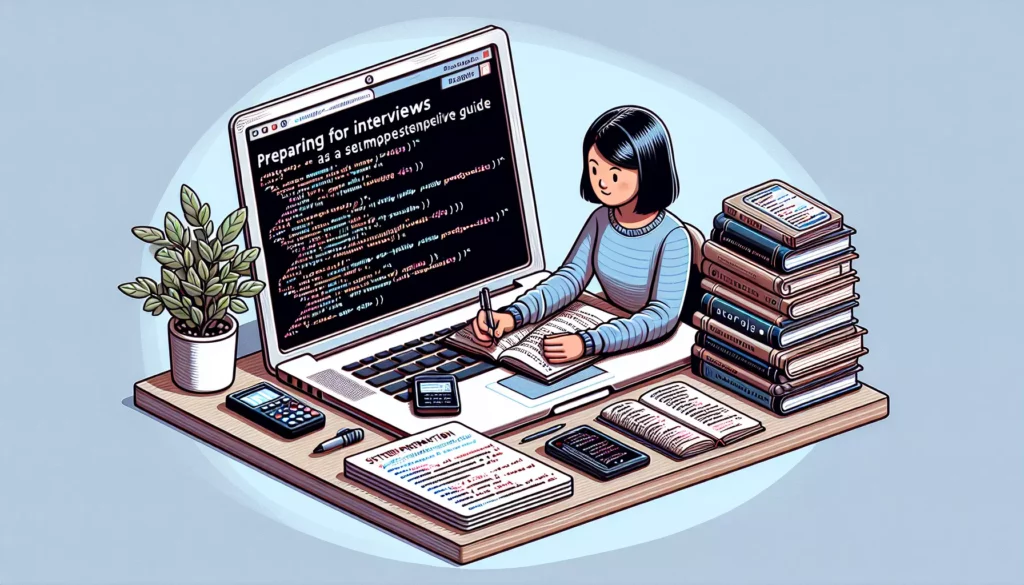
Breaking into the tech industry as a self-taught programmer can be both exciting and daunting. While you may have honed your coding skills through countless hours of practice and personal projects, the prospect of facing technical interviews at top companies like FAANG (Facebook, Amazon, Apple, Netflix, Google) can still be intimidating. However, with the right preparation and mindset, you can level the playing field and showcase your abilities effectively. This comprehensive guide will walk you through the essential steps and strategies for preparing for interviews as a self-taught programmer.
1. Understanding the Interview Process
Before diving into preparation tactics, it’s crucial to understand what you’re up against. Technical interviews at major tech companies typically consist of several stages:
- Initial phone screen or online assessment
- One or more technical phone/video interviews
- On-site interviews (which may be virtual due to current circumstances)
Each stage is designed to assess different aspects of your skills and knowledge. The process usually evaluates your:
- Problem-solving abilities
- Coding proficiency
- Algorithm and data structure knowledge
- System design understanding (for more senior roles)
- Communication skills
- Cultural fit
2. Strengthening Your Foundation
As a self-taught programmer, you may have gaps in your knowledge that formal education typically covers. It’s essential to identify and address these gaps:
2.1. Master the Fundamentals
Ensure you have a solid understanding of:
- Data Structures (arrays, linked lists, stacks, queues, trees, graphs, hash tables)
- Algorithms (sorting, searching, recursion, dynamic programming)
- Time and space complexity analysis (Big O notation)
- Object-Oriented Programming concepts
- Basic design patterns
2.2. Choose a Primary Programming Language
While it’s beneficial to be versatile, focus on mastering one language for interviews. Popular choices include:
- Python
- Java
- C++
- JavaScript
Understand the language’s syntax, standard libraries, and idiomatic practices thoroughly.
3. Developing a Study Plan
Creating a structured study plan is crucial for effective preparation:
3.1. Set Clear Goals
Define what you want to achieve and by when. For example:
- Solve 200 LeetCode problems in 3 months
- Complete a data structures and algorithms course in 6 weeks
- Build 3 significant projects to showcase your skills
3.2. Allocate Time Wisely
Balance your time between:
- Studying theoretical concepts
- Practicing coding problems
- Working on personal projects
- Mock interviews and soft skills development
3.3. Use Quality Resources
Leverage a mix of resources to cover all bases:
- Books: “Cracking the Coding Interview” by Gayle Laakmann McDowell, “Introduction to Algorithms” by Cormen et al.
- Online platforms: LeetCode, HackerRank, AlgoCademy
- MOOCs: Coursera, edX, Udacity
- YouTube channels: MIT OpenCourseWare, mycodeschool, CS Dojo
4. Mastering Problem-Solving Techniques
Developing strong problem-solving skills is crucial for acing technical interviews:
4.1. Understand the Problem-Solving Framework
- Clarify the problem and requirements
- Analyze different approaches
- Design a solution
- Implement the code
- Test and optimize
4.2. Practice Common Patterns
Familiarize yourself with recurring problem-solving patterns:
- Two-pointer technique
- Sliding window
- Binary search
- Depth-First Search (DFS) and Breadth-First Search (BFS)
- Dynamic programming
- Greedy algorithms
4.3. Solve Problems Regularly
Consistent practice is key. Aim to solve:
- 1-2 easy problems daily
- 2-3 medium problems weekly
- 1 hard problem weekly
Gradually increase the difficulty as you improve.
5. Building a Strong Portfolio
As a self-taught programmer, your portfolio is crucial in demonstrating your skills:
5.1. Develop Personal Projects
Create projects that:
- Solve real-world problems
- Demonstrate your proficiency in relevant technologies
- Showcase your ability to write clean, maintainable code
5.2. Contribute to Open Source
Contributing to open-source projects can:
- Enhance your collaboration skills
- Expose you to large codebases and industry practices
- Build your network within the developer community
5.3. Document Your Work
Maintain a well-organized GitHub profile and consider starting a technical blog to document your learning journey and project insights.
6. Honing Your Soft Skills
Technical skills alone aren’t enough. Soft skills play a crucial role in interviews:
6.1. Improve Communication
Practice explaining your thought process clearly. This includes:
- Articulating your approach to problem-solving
- Explaining technical concepts in simple terms
- Asking clarifying questions when needed
6.2. Develop Collaboration Skills
Even in individual interviews, showcase your ability to work in a team:
- Be receptive to feedback
- Demonstrate how you handle disagreements constructively
- Show enthusiasm for learning from others
6.3. Cultivate a Growth Mindset
Emphasize your passion for continuous learning and adaptability to new technologies and challenges.
7. Mastering the Art of Whiteboard Coding
Many technical interviews involve coding on a whiteboard or a shared online editor:
7.1. Practice Without an IDE
Get comfortable coding without the crutch of auto-completion and syntax highlighting:
- Use a simple text editor for practice
- Write code on paper or a personal whiteboard
7.2. Think Aloud
Verbalize your thought process as you code. This helps the interviewer understand your approach and can lead to valuable hints or guidance.
7.3. Handle Edge Cases
Always consider and address edge cases in your solutions. This demonstrates thoroughness and attention to detail.
8. Preparing for System Design Interviews
For more senior roles or at companies like FAANG, system design interviews are common:
8.1. Understand Key Concepts
Familiarize yourself with:
- Scalability principles
- Load balancing
- Caching mechanisms
- Database sharding
- Microservices architecture
8.2. Study Real-World Systems
Analyze the architecture of popular systems and services:
- How does Twitter handle millions of tweets?
- How does Google Maps provide real-time navigation?
- How does Netflix stream video to millions of users simultaneously?
8.3. Practice Designing Systems
Work through common system design interview questions:
- Design a URL shortener
- Create a social media feed
- Build a distributed cache
9. Leveraging Online Coding Platforms
Online platforms can significantly enhance your interview preparation:
9.1. AlgoCademy
AlgoCademy offers interactive coding tutorials and AI-powered assistance, making it an excellent resource for self-taught programmers. It provides:
- Step-by-step guidance on solving algorithmic problems
- A structured curriculum to progress from basics to advanced topics
- Real-time feedback on your code
9.2. LeetCode
LeetCode is a go-to platform for coding interview preparation:
- Vast collection of coding problems
- Company-specific problem sets
- Discussion forums for learning from others’ solutions
9.3. HackerRank
HackerRank offers a mix of practice problems and skill certifications:
- Language-specific practice tracks
- Coding challenges and contests
- Interview preparation kits
10. Conducting Mock Interviews
Simulating the interview experience is crucial for building confidence:
10.1. Use Online Platforms
Websites like Pramp or InterviewBit allow you to practice with peers in a simulated interview environment.
10.2. Practice with Friends or Mentors
If possible, ask experienced developers to conduct mock interviews and provide feedback.
10.3. Record Yourself
Record your mock interviews to review your performance, body language, and communication style.
11. Staying Updated with Industry Trends
As a self-taught programmer, it’s crucial to stay informed about the latest developments in the tech industry:
11.1. Follow Tech News
Stay updated with industry news through sources like:
- Hacker News
- TechCrunch
- ArsTechnica
11.2. Engage in Developer Communities
Participate in online forums and communities:
- Stack Overflow
- Reddit (r/programming, r/cscareerquestions)
- Dev.to
11.3. Attend Tech Meetups and Conferences
Even if virtual, these events can provide valuable insights and networking opportunities.
12. Tailoring Your Preparation to Specific Companies
Different companies have different interview styles and focus areas:
12.1. Research Company-Specific Interview Processes
Use resources like Glassdoor and LeetCode’s company sections to understand what to expect.
12.2. Focus on Relevant Technologies
If a company primarily uses a specific tech stack, ensure you’re comfortable with it.
12.3. Understand the Company’s Products and Culture
Familiarize yourself with the company’s products, recent news, and cultural values.
13. Managing Interview Anxiety
Nervousness is natural, but it’s important to manage it effectively:
13.1. Practice Relaxation Techniques
Deep breathing exercises or meditation can help calm nerves before an interview.
13.2. Prepare a Pre-Interview Routine
Develop a routine that helps you feel confident and focused, such as reviewing key concepts or doing light coding exercises.
13.3. Reframe Your Mindset
View the interview as an opportunity to learn and showcase your skills, rather than a test.
14. Handling Technical Questions During the Interview
When faced with a technical question in the interview:
14.1. Listen Carefully and Ask Questions
Ensure you fully understand the problem before starting to solve it. Don’t hesitate to ask for clarification.
14.2. Think Out Loud
Share your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach.
14.3. Start with a Brute Force Solution
If you’re stuck, begin with a simple, inefficient solution and then work on optimizing it.
14.4. Test Your Solution
Before declaring you’re done, walk through your solution with a few test cases to catch any errors.
15. Dealing with Rejection Constructively
Rejection is a part of the job search process, especially in competitive fields like tech:
15.1. Ask for Feedback
If possible, request feedback on your performance to understand areas for improvement.
15.2. Reflect and Improve
Use each interview experience, successful or not, as a learning opportunity.
15.3. Keep Persevering
Remember that many successful programmers faced multiple rejections before landing their dream job.
16. Negotiating Job Offers
If you receive an offer, it’s important to negotiate effectively:
16.1. Research Market Rates
Use sites like Glassdoor, PayScale, and levels.fyi to understand the typical compensation for your role and experience level.
16.2. Consider the Entire Package
Look beyond just the salary. Consider factors like equity, bonuses, benefits, and growth opportunities.
16.3. Practice Your Negotiation
Role-play negotiation scenarios with a friend to build confidence in discussing compensation.
17. Continuing Education After Landing the Job
Your learning journey doesn’t end with getting hired:
17.1. Set Learning Goals
Regularly set new learning objectives to keep growing your skills.
17.2. Take Advantage of Company Resources
Many companies offer learning stipends or internal training programs. Make the most of these opportunities.
17.3. Mentor Others
Teaching is an excellent way to solidify your own knowledge and give back to the community.
Conclusion
Preparing for interviews as a self-taught programmer requires dedication, strategic planning, and consistent effort. By following this comprehensive guide, you can develop the skills and confidence needed to succeed in technical interviews, even at top tech companies. Remember, your unique journey as a self-taught programmer is an asset – it demonstrates your passion, self-motivation, and ability to learn independently. Embrace your background, showcase your projects and skills effectively, and approach each interview as an opportunity to learn and grow. With persistence and the right preparation, you can turn your self-taught programming skills into a successful career in tech.
As you embark on this challenging but rewarding journey, leverage resources like AlgoCademy to structure your learning, practice coding problems, and receive guidance. Remember that every experienced programmer was once a beginner, and many successful tech professionals are self-taught. Your dedication to learning and problem-solving is your greatest asset. Good luck with your interview preparation, and may your self-taught journey lead you to the tech career of your dreams!