Practical Guide to Modular Exponentiation: Efficient Power Calculation in Cryptography
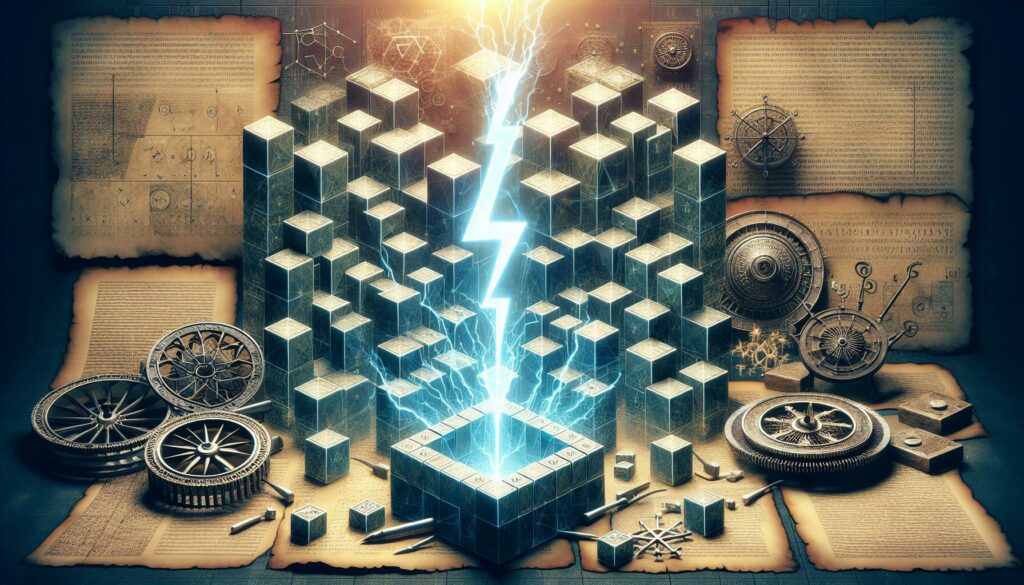
Welcome to AlgoCademy’s comprehensive guide on modular exponentiation! If you’re preparing for technical interviews at top tech companies or looking to enhance your algorithmic skills, understanding this crucial concept is essential. Modular exponentiation is a fundamental operation in various cryptographic algorithms and has wide-ranging applications in computer science. In this article, we’ll dive deep into the topic, exploring its importance, implementation, and optimization techniques.
Table of Contents
- Introduction to Modular Exponentiation
- Importance in Cryptography and Computer Science
- Naive Approach to Modular Exponentiation
- Efficient Algorithm: Square and Multiply
- Implementation in Various Programming Languages
- Optimization Techniques
- Real-world Applications
- Interview Tips and Common Questions
- Conclusion
1. Introduction to Modular Exponentiation
Modular exponentiation is the operation of calculating the remainder when a number (base) is raised to a power (exponent) and then divided by a modulus. Mathematically, it’s represented as:
result = (base^exponent) mod modulus
For example, if we want to calculate 3^7 mod 10, the result would be 7 because 3^7 = 2187, and 2187 mod 10 = 7.
While this may seem simple for small numbers, it becomes challenging when dealing with large values, which is often the case in cryptographic applications. The naive approach of first calculating the full exponentiation and then taking the modulus is inefficient and can lead to integer overflow for large numbers.
2. Importance in Cryptography and Computer Science
Modular exponentiation is a cornerstone of many cryptographic algorithms and protocols. Its importance stems from several key factors:
- Security: It forms the basis of public-key cryptography systems like RSA, where the security relies on the difficulty of factoring large numbers.
- Efficiency: It allows for computations with very large numbers without causing overflow, which is crucial in cryptography.
- Diffie-Hellman Key Exchange: This protocol uses modular exponentiation to securely exchange cryptographic keys over a public channel.
- Digital Signatures: Many digital signature algorithms rely on modular exponentiation for their security properties.
- Primality Testing: Some primality tests, like the Miller-Rabin test, use modular exponentiation as a core operation.
Beyond cryptography, modular exponentiation finds applications in various areas of computer science, including:
- Hash functions
- Random number generation
- Coding theory
- Computer algebra systems
3. Naive Approach to Modular Exponentiation
Before we dive into efficient algorithms, let’s look at the naive approach to understand why it’s inadequate for large numbers:
def naive_mod_exp(base, exponent, modulus):
result = 1
for _ in range(exponent):
result = (result * base) % modulus
return result
This approach has a time complexity of O(exponent), which becomes impractical for large exponents. Moreover, it doesn’t handle the potential overflow that can occur when dealing with large numbers in cryptographic applications.
4. Efficient Algorithm: Square and Multiply
The efficient approach to modular exponentiation is the “Square and Multiply” algorithm, also known as the “Binary Exponentiation” method. This algorithm reduces the time complexity to O(log(exponent)), making it suitable for large numbers.
The key idea is to use the binary representation of the exponent and perform a series of squaring and multiplying operations. Here’s how it works:
- Convert the exponent to its binary representation.
- Initialize the result to 1.
- For each bit in the binary representation of the exponent (from left to right):
- Square the result.
- If the current bit is 1, multiply the result by the base.
- Apply the modulus operation after each step to keep the numbers manageable.
Here’s a Python implementation of the Square and Multiply algorithm:
def mod_exp(base, exponent, modulus):
result = 1
base = base % modulus
while exponent > 0:
if exponent % 2 == 1:
result = (result * base) % modulus
exponent = exponent >> 1
base = (base * base) % modulus
return result
This algorithm is much more efficient and can handle large numbers without overflow issues.
5. Implementation in Various Programming Languages
Let’s look at implementations of the Square and Multiply algorithm in different programming languages:
C++
#include <iostream>
long long mod_exp(long long base, long long exponent, long long modulus) {
long long result = 1;
base = base % modulus;
while (exponent > 0) {
if (exponent % 2 == 1)
result = (result * base) % modulus;
exponent = exponent >> 1;
base = (base * base) % modulus;
}
return result;
}
int main() {
std::cout << mod_exp(3, 7, 10) << std::endl; // Output: 7
return 0;
}
Java
public class ModularExponentiation {
public static long modExp(long base, long exponent, long modulus) {
long result = 1;
base = base % modulus;
while (exponent > 0) {
if (exponent % 2 == 1)
result = (result * base) % modulus;
exponent = exponent >> 1;
base = (base * base) % modulus;
}
return result;
}
public static void main(String[] args) {
System.out.println(modExp(3, 7, 10)); // Output: 7
}
}
JavaScript
function modExp(base, exponent, modulus) {
let result = 1n;
base = base % modulus;
while (exponent > 0) {
if (exponent % 2n === 1n)
result = (result * base) % modulus;
exponent = exponent >> 1n;
base = (base * base) % modulus;
}
return result;
}
console.log(modExp(3n, 7n, 10n)); // Output: 7n
Note: In JavaScript, we use BigInt (denoted by the ‘n’ suffix) to handle large integers accurately.
6. Optimization Techniques
While the Square and Multiply algorithm is efficient, there are several optimization techniques that can further improve its performance:
6.1 Montgomery Reduction
Montgomery reduction is a technique that replaces expensive division operations with cheaper multiplication and bit-shift operations. It’s particularly useful when performing many modular multiplications with the same modulus.
6.2 Chinese Remainder Theorem (CRT)
When the modulus is a product of coprime factors (as in RSA), the Chinese Remainder Theorem can be used to perform the exponentiation in smaller subgroups and then combine the results. This can significantly speed up the computation.
6.3 Sliding Window Exponentiation
This is an extension of the Square and Multiply method that processes multiple bits of the exponent at once, reducing the number of multiplications required.
6.4 Precomputation
If the base and modulus are fixed and multiple exponentiations are needed, precomputing and storing some powers of the base can speed up subsequent calculations.
7. Real-world Applications
Modular exponentiation is used in various real-world applications:
7.1 RSA Encryption
RSA, one of the first public-key cryptosystems, heavily relies on modular exponentiation. The encryption process involves computing:
ciphertext = (message^e) mod n
Where ‘e’ is the public exponent and ‘n’ is the modulus.
7.2 Diffie-Hellman Key Exchange
This protocol allows two parties to establish a shared secret over an insecure channel. It uses modular exponentiation to compute the shared secret:
shared_secret = (other_party_public_key^private_key) mod p
7.3 ElGamal Encryption
Another public-key cryptosystem that uses modular exponentiation for both encryption and decryption.
7.4 Digital Signature Algorithm (DSA)
DSA uses modular exponentiation in the process of generating and verifying digital signatures.
8. Interview Tips and Common Questions
When preparing for technical interviews, especially for FAANG companies, it’s crucial to have a solid understanding of modular exponentiation. Here are some tips and common questions:
8.1 Time and Space Complexity
Be prepared to discuss the time and space complexity of your implementation. The Square and Multiply algorithm has a time complexity of O(log n) and space complexity of O(1).
8.2 Handling Large Numbers
Interviewers might ask how you’d handle numbers that are too large for standard integer types. Discuss using libraries for arbitrary-precision arithmetic or implementing your own big integer class.
8.3 Optimization Techniques
Be familiar with optimization techniques like Montgomery reduction or the Chinese Remainder Theorem. Even if you don’t implement them, being able to discuss these optimizations shows depth of knowledge.
8.4 Common Interview Questions
- “Implement a function to calculate (x^n) % m efficiently.”
- “How would you optimize modular exponentiation if you need to perform it multiple times with the same base and modulus?”
- “Explain how modular exponentiation is used in the RSA algorithm.”
- “What’s the difference between (a * b) % m and ((a % m) * (b % m)) % m? When would you use each?”
9. Conclusion
Modular exponentiation is a fundamental concept in cryptography and computer science. Its efficient implementation is crucial for the security and performance of many cryptographic systems. By understanding the Square and Multiply algorithm and various optimization techniques, you’ll be well-prepared to tackle related problems in technical interviews and real-world applications.
Remember, practice is key. Implement the algorithms yourself, experiment with different optimizations, and solve related problems to solidify your understanding. With dedication and consistent practice, you’ll master this important concept and be well on your way to excelling in your coding interviews and career.
Stay curious, keep coding, and best of luck in your journey with AlgoCademy!