Practical Guide to Implementing Graph Algorithms
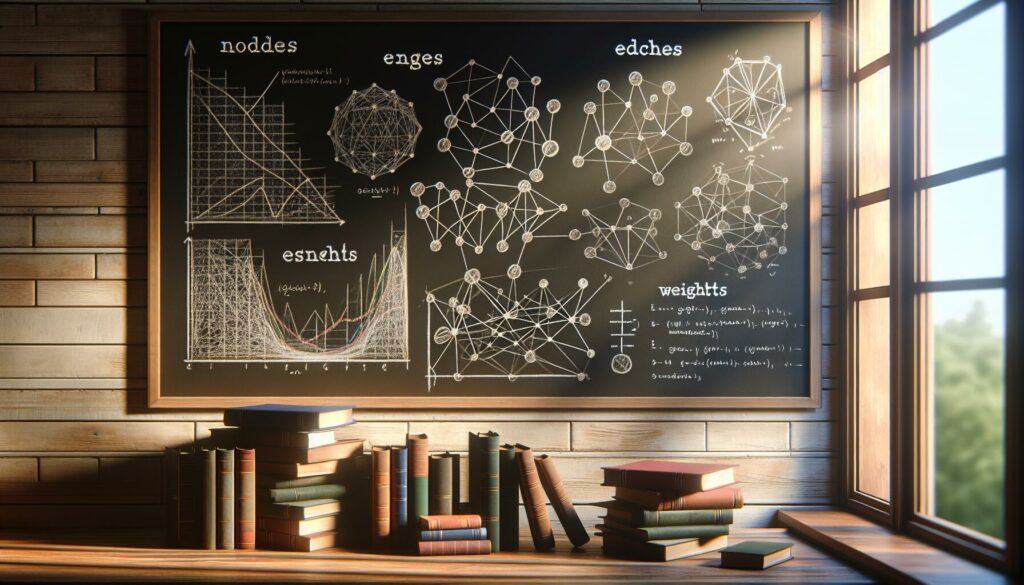
Graph algorithms are fundamental tools in computer science and are widely used in various applications, from social network analysis to route planning in navigation systems. This comprehensive guide will walk you through the practical implementation of key graph algorithms, providing you with the knowledge and skills to tackle complex problems efficiently.
Table of Contents
- Introduction to Graph Algorithms
- Graph Representation
- Graph Traversal Algorithms
- Shortest Path Algorithms
- Minimum Spanning Tree Algorithms
- Topological Sorting
- Strongly Connected Components
- Advanced Graph Algorithms
- Practical Applications
- Optimization Techniques
- Conclusion
1. Introduction to Graph Algorithms
Graph algorithms are a set of instructions designed to perform operations on graph data structures. These algorithms are crucial in solving various real-world problems that can be modeled as graphs. Before diving into specific algorithms, let’s review some basic graph concepts:
- Vertex (Node): A fundamental unit of a graph representing an entity.
- Edge: A connection between two vertices, which can be directed or undirected.
- Weighted Graph: A graph where each edge has an associated weight or cost.
- Directed Graph (Digraph): A graph where edges have a direction, indicating a one-way relationship.
- Undirected Graph: A graph where edges have no direction, representing a two-way relationship.
Understanding these concepts is crucial for implementing and working with graph algorithms effectively.
2. Graph Representation
Before implementing any graph algorithm, we need to choose an appropriate way to represent the graph in code. The two most common representations are:
Adjacency Matrix
An adjacency matrix is a 2D array where the cell at position (i, j) represents the edge between vertices i and j. This representation is efficient for dense graphs and quick edge lookup but can be memory-intensive for sparse graphs.
class Graph:
def __init__(self, num_vertices):
self.num_vertices = num_vertices
self.adj_matrix = [[0] * num_vertices for _ in range(num_vertices)]
def add_edge(self, u, v, weight=1):
self.adj_matrix[u][v] = weight
self.adj_matrix[v][u] = weight # For undirected graph
Adjacency List
An adjacency list uses a list or dictionary to store the neighbors of each vertex. This representation is memory-efficient for sparse graphs and is generally preferred for most graph algorithms.
from collections import defaultdict
class Graph:
def __init__(self):
self.adj_list = defaultdict(list)
def add_edge(self, u, v, weight=1):
self.adj_list[u].append((v, weight))
self.adj_list[v].append((u, weight)) # For undirected graph
Choosing the right representation depends on the specific problem and the operations you need to perform on the graph.
3. Graph Traversal Algorithms
Graph traversal algorithms are fundamental for exploring and analyzing graphs. The two primary traversal methods are Depth-First Search (DFS) and Breadth-First Search (BFS).
Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking. It’s implemented using recursion or a stack.
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
Breadth-First Search (BFS)
BFS explores all the neighbor nodes at the present depth before moving to nodes at the next depth level. It’s implemented using a queue.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
Both DFS and BFS have a time complexity of O(V + E), where V is the number of vertices and E is the number of edges.
4. Shortest Path Algorithms
Shortest path algorithms are used to find the most efficient route between nodes in a graph. Two popular algorithms for this purpose are Dijkstra’s algorithm and the Bellman-Ford algorithm.
Dijkstra’s Algorithm
Dijkstra’s algorithm finds the shortest path from a starting node to all other nodes in a weighted graph with non-negative edge weights.
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
Bellman-Ford Algorithm
The Bellman-Ford algorithm can handle graphs with negative edge weights and detect negative cycles.
def bellman_ford(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
for _ in range(len(graph) - 1):
for node in graph:
for neighbor, weight in graph[node].items():
if distances[node] + weight < distances[neighbor]:
distances[neighbor] = distances[node] + weight
# Check for negative cycles
for node in graph:
for neighbor, weight in graph[node].items():
if distances[node] + weight < distances[neighbor]:
print("Graph contains a negative cycle")
return None
return distances
Dijkstra’s algorithm has a time complexity of O((V + E) log V) with a binary heap, while Bellman-Ford has a time complexity of O(VE).
5. Minimum Spanning Tree Algorithms
A Minimum Spanning Tree (MST) is a subset of edges in a weighted, undirected graph that connects all vertices with the minimum total edge weight. Two popular algorithms for finding MSTs are Kruskal’s algorithm and Prim’s algorithm.
Kruskal’s Algorithm
Kruskal’s algorithm builds the MST by adding edges in order of increasing weight, skipping edges that would create a cycle.
class DisjointSet:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
def kruskal(graph):
edges = [(weight, u, v) for u in graph for v, weight in graph[u].items()]
edges.sort()
vertices = list(graph.keys())
ds = DisjointSet(vertices)
mst = []
for weight, u, v in edges:
if ds.find(u) != ds.find(v):
ds.union(u, v)
mst.append((u, v, weight))
return mst
Prim’s Algorithm
Prim’s algorithm starts with a single vertex and grows the MST by adding the cheapest edge that connects a vertex in the MST to a vertex outside the MST.
import heapq
def prim(graph):
start_vertex = next(iter(graph))
mst = []
visited = set([start_vertex])
edges = [(weight, start_vertex, to) for to, weight in graph[start_vertex].items()]
heapq.heapify(edges)
while edges:
weight, frm, to = heapq.heappop(edges)
if to not in visited:
visited.add(to)
mst.append((frm, to, weight))
for next_to, next_weight in graph[to].items():
if next_to not in visited:
heapq.heappush(edges, (next_weight, to, next_to))
return mst
Both Kruskal’s and Prim’s algorithms have a time complexity of O(E log E) or O(E log V), depending on the implementation.
6. Topological Sorting
Topological sorting is used to linearly order the vertices of a Directed Acyclic Graph (DAG) such that for every directed edge (u, v), vertex u comes before v in the ordering. This is particularly useful in scheduling tasks with dependencies.
from collections import defaultdict
def topological_sort(graph):
def dfs(node):
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs(neighbor)
stack.append(node)
visited = set()
stack = []
for node in graph:
if node not in visited:
dfs(node)
return stack[::-1]
# Example usage
graph = defaultdict(list)
graph[0].extend([1, 2])
graph[1].append(3)
graph[2].append(3)
graph[3].append(4)
graph[4] = []
print(topological_sort(graph))
The time complexity of topological sorting is O(V + E), where V is the number of vertices and E is the number of edges in the graph.
7. Strongly Connected Components
A Strongly Connected Component (SCC) in a directed graph is a subset of vertices where every vertex is reachable from every other vertex in the subset. Kosaraju’s algorithm is an efficient method to find all SCCs in a graph.
from collections import defaultdict
def kosaraju(graph):
def dfs(node, visited, stack):
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs(neighbor, visited, stack)
stack.append(node)
def reverse_graph(graph):
reversed_graph = defaultdict(list)
for node in graph:
for neighbor in graph[node]:
reversed_graph[neighbor].append(node)
return reversed_graph
def dfs_scc(node, visited, scc):
visited.add(node)
scc.append(node)
for neighbor in reversed_graph[node]:
if neighbor not in visited:
dfs_scc(neighbor, visited, scc)
stack = []
visited = set()
for node in graph:
if node not in visited:
dfs(node, visited, stack)
reversed_graph = reverse_graph(graph)
visited.clear()
sccs = []
while stack:
node = stack.pop()
if node not in visited:
scc = []
dfs_scc(node, visited, scc)
sccs.append(scc)
return sccs
# Example usage
graph = defaultdict(list)
graph[0].extend([1, 3])
graph[1].extend([2])
graph[2].extend([0])
graph[3].extend([4])
graph[4] = []
print(kosaraju(graph))
Kosaraju’s algorithm has a time complexity of O(V + E), making it efficient for large graphs.
8. Advanced Graph Algorithms
As you become more proficient with basic graph algorithms, you can explore more advanced techniques to solve complex problems. Some advanced graph algorithms include:
A* Search Algorithm
A* is a best-first search algorithm that finds the least-cost path from a start node to a goal node. It uses a heuristic function to estimate the cost from any node to the goal, making it more efficient than Dijkstra’s algorithm for many problems.
import heapq
def heuristic(a, b):
# Manhattan distance on a square grid
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def a_star(graph, start, goal):
open_set = []
heapq.heappush(open_set, (0, start))
came_from = {}
g_score = {start: 0}
f_score = {start: heuristic(start, goal)}
while open_set:
current = heapq.heappop(open_set)[1]
if current == goal:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.append(start)
return path[::-1]
for neighbor in graph[current]:
tentative_g_score = g_score[current] + graph[current][neighbor]
if neighbor not in g_score or tentative_g_score < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = g_score[neighbor] + heuristic(neighbor, goal)
heapq.heappush(open_set, (f_score[neighbor], neighbor))
return None
Floyd-Warshall Algorithm
The Floyd-Warshall algorithm finds the shortest paths between all pairs of vertices in a weighted graph. It can handle negative edge weights but not negative cycles.
def floyd_warshall(graph):
dist = {node: {node: float('inf') for node in graph} for node in graph}
for node in graph:
dist[node][node] = 0
for neighbor, weight in graph[node].items():
dist[node][neighbor] = weight
for k in graph:
for i in graph:
for j in graph:
dist[i][j] = min(dist[i][j], dist[i][k] + dist[k][j])
return dist
Maximum Flow Algorithms
Maximum flow algorithms, such as Ford-Fulkerson or Edmonds-Karp, are used to find the maximum flow in a flow network. These algorithms have applications in network optimization, bipartite matching, and more.
Implementing these advanced algorithms will significantly enhance your problem-solving skills and prepare you for tackling complex graph-related challenges in technical interviews and real-world applications.
9. Practical Applications
Graph algorithms have numerous real-world applications across various domains. Understanding these applications can help you appreciate the importance of mastering these algorithms:
Social Network Analysis
- Identifying influential users (using centrality measures)
- Detecting communities (using clustering algorithms)
- Recommending friends or connections (using similarity measures)
Transportation and Logistics
- Finding optimal routes (using shortest path algorithms)
- Optimizing delivery networks (using minimum spanning tree algorithms)
- Solving the traveling salesman problem (using approximation algorithms)
Computer Networks
- Routing protocols (using shortest path algorithms)
- Network flow optimization (using maximum flow algorithms)
- Detecting network vulnerabilities (using connectivity algorithms)
Bioinformatics
- Protein-protein interaction networks
- Gene regulatory networks
- Phylogenetic tree construction
Recommendation Systems
- Collaborative filtering
- Content-based recommendation
- Hybrid recommendation systems
By understanding these applications, you can better contextualize the graph algorithms you’re learning and see how they can be applied to solve real-world problems.
10. Optimization Techniques
As you work with larger and more complex graphs, optimizing your implementations becomes crucial. Here are some techniques to improve the performance of your graph algorithms:
Data Structure Selection
Choose the right data structures for your specific use case. For example:
- Use adjacency lists for sparse graphs and adjacency matrices for dense graphs
- Consider using bit vectors for representing sets in algorithms like DFS or BFS
- Use efficient priority queues (e.g., Fibonacci heaps) for algorithms like Dijkstra’s
Algorithmic Improvements
Implement optimized versions of algorithms when possible:
- Use bidirectional search for faster pathfinding
- Implement iterative deepening for memory-constrained DFS
- Use Johnson’s algorithm for all-pairs shortest paths in sparse graphs
Parallelization
For large-scale graphs, consider parallelizing your algorithms:
- Use multi-threading for independent computations
- Implement distributed versions of algorithms for processing massive graphs
- Utilize GPU acceleration for graph algorithms that can be parallelized
Caching and Memoization
Store and reuse intermediate results to avoid redundant computations:
- Cache shortest paths in multi-source shortest path problems
- Use dynamic programming techniques in applicable algorithms
Graph Compression
For very large graphs, consider using compression techniques:
- Implement graph compression algorithms like WebGraph
- Use succinct data structures for space-efficient graph representation
By applying these optimization techniques, you can significantly improve the performance and scalability of your graph algorithm implementations.
11. Conclusion
Mastering graph algorithms is a crucial skill for any software engineer or computer scientist. This guide has provided you with a comprehensive overview of essential graph algorithms, their implementations, and practical applications. By understanding and implementing these algorithms, you’ll be well-equipped to tackle a wide range of problems in various domains.
Remember that becoming proficient in graph algorithms requires practice and experience. Continue to work on diverse problems, participate in coding challenges, and apply these algorithms to real-world scenarios. As you progress, you’ll develop a deeper intuition for when and how to use specific algorithms, and you’ll be able to optimize and adapt them to suit your needs.
Key takeaways from this guide include:
- Understanding different graph representations and their trade-offs
- Implementing fundamental graph traversal algorithms (DFS and BFS)
- Mastering shortest path algorithms like Dijkstra’s and Bellman-Ford
- Applying minimum spanning tree algorithms (Kruskal’s and Prim’s)
- Utilizing topological sorting for dependency resolution
- Identifying strongly connected components in directed graphs
- Exploring advanced algorithms like A* search and maximum flow
- Recognizing practical applications of graph algorithms
- Optimizing implementations for better performance
As you continue your journey in mastering graph algorithms, remember to stay curious, explore new techniques, and always look for opportunities to apply your knowledge to solve real-world problems. With dedication and practice, you’ll become proficient in implementing and optimizing graph algorithms, making you a valuable asset in any software development or data science team.