Poetic Programming: Expressing Algorithms Through Haiku and Sonnets
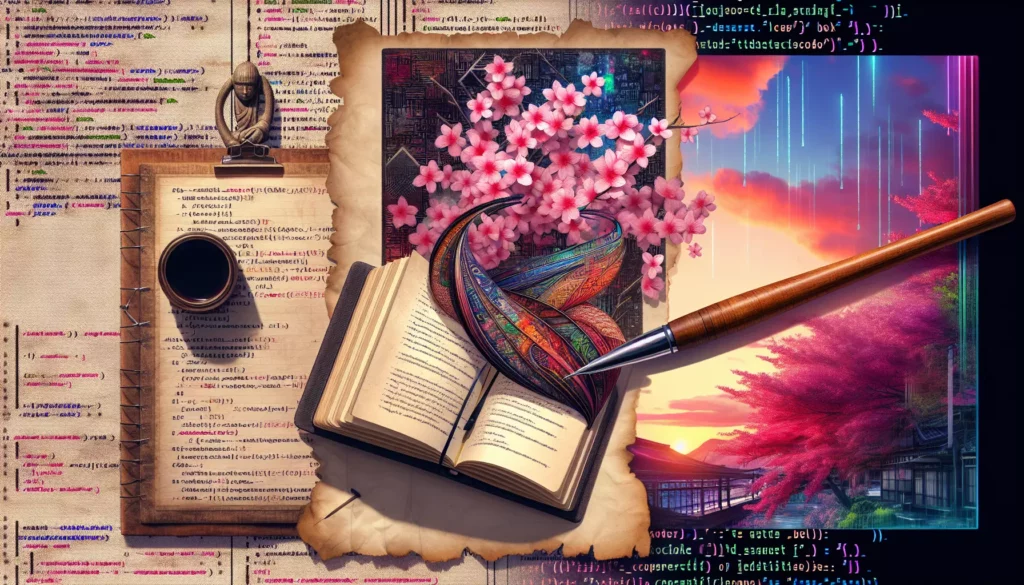
In the realm of coding and computer science, we often think of algorithms as purely logical constructs, devoid of artistic expression. However, what if we could bridge the gap between the structured world of programming and the lyrical beauty of poetry? Welcome to the fascinating intersection of code and verse, where algorithms dance with haiku and sonnets paint pictures of computational processes. This unique fusion not only offers a fresh perspective on programming concepts but also provides an innovative approach to learning and remembering complex algorithms.
The Art of Algorithmic Poetry
Algorithmic poetry, or code poetry, is an emerging form of creative expression that combines the precision of programming with the elegance of poetic structure. By encapsulating algorithmic concepts within the constraints of poetic forms like haiku and sonnets, we can create a memorable and aesthetically pleasing way to understand and recall key programming principles.
Haiku: Capturing Algorithms in 17 Syllables
Haiku, a traditional Japanese poetic form, consists of three lines with a 5-7-5 syllable structure. This concise format challenges us to distill complex algorithms into their essence, focusing on the core concepts and steps. Let’s explore some examples of algorithmic haiku:
Binary Search Haiku
Divide and conquer
Mid-point splits the sorted list
Left or right, repeat
This haiku encapsulates the fundamental principle of the binary search algorithm. It highlights the divide-and-conquer approach, the importance of a sorted list, and the iterative process of narrowing down the search space.
Quicksort Haiku
Choose pivot, divide
Less left, greater right, recurse
Merge for sorted bliss
Here, we’ve captured the essence of the Quicksort algorithm in just 17 syllables. The haiku touches on key concepts like selecting a pivot, partitioning the array, and the recursive nature of the algorithm.
Sonnets: Eloquent Expressions of Algorithmic Complexity
While haiku offer a concise snapshot of an algorithm, sonnets provide more room for elaboration. With 14 lines and a specific rhyme scheme, sonnets allow us to delve deeper into the intricacies of complex algorithms. Let’s examine a sonnet dedicated to the famous Dijkstra’s algorithm:
Dijkstra’s Algorithm Sonnet
In graph of nodes, a path we seek to find,
From source to all, the shortest route to trace.
With weighted edges, distances refined,
We start our journey at the starting place.
Priority queue, our faithful guide and friend,
Keeps track of vertices we've yet to see.
While queue's not empty, onwards we'll extend,
Updating distances relentlessly.
For each new node, we check its neighbors true,
If shorter path exists, we update fast.
The process continues, our search renews,
Until the queue is empty at the last.
When algorithm ends, its work complete,
The shortest paths to all nodes we can greet.
This sonnet beautifully captures the essence of Dijkstra’s algorithm, from its purpose of finding the shortest path in a weighted graph to the key components like the priority queue and the process of updating distances. The rhyme and meter of the sonnet form add a melodic quality to the description, making it more engaging and memorable.
The Benefits of Poetic Programming
Expressing algorithms through poetry offers several advantages for both learners and experienced programmers:
- Enhanced Memorization: The rhythmic and structured nature of poetry makes it easier to remember complex algorithmic concepts.
- Creative Problem-Solving: Combining coding with poetry encourages out-of-the-box thinking and can lead to novel approaches to problem-solving.
- Improved Communication: Poetic representations of algorithms can serve as effective communication tools, making it easier to explain concepts to non-technical audiences.
- Interdisciplinary Learning: This approach bridges the gap between STEM and the humanities, fostering a more well-rounded educational experience.
- Engagement and Fun: Adding a creative twist to coding can make learning more enjoyable and engaging, especially for those who might be intimidated by traditional programming instruction.
Implementing Poetic Programming in Education
Integrating poetic programming into coding education can be a powerful tool for enhancing learning experiences. Here are some ways to incorporate this concept into educational settings:
1. Algorithm Poetry Workshops
Organize workshops where students learn to express algorithms through various poetic forms. This can be a collaborative exercise where groups work together to create haiku or sonnets that accurately represent specific algorithms.
2. Coding Challenges with a Poetic Twist
Create coding challenges that require students to not only implement an algorithm but also describe it in a poetic form. This dual approach reinforces understanding and encourages creativity.
3. Algorithmic Poetry Slams
Host events where students can perform their algorithmic poems, fostering a fun and interactive learning environment while also practicing public speaking skills.
4. Interdisciplinary Projects
Collaborate with literature or creative writing departments to create projects that blend coding concepts with poetic expression, encouraging students from different disciplines to work together.
5. Mnemonic Devices
Use poetic representations of algorithms as mnemonic devices to help students remember key concepts, especially when preparing for exams or technical interviews.
Advanced Poetic Programming: Beyond Haiku and Sonnets
While haiku and sonnets provide excellent starting points for poetic programming, the concept can be extended to other poetic forms and even more complex programming concepts. Let’s explore some advanced applications:
Villanelles for Design Patterns
The villanelle, with its intricate structure of repeating lines, can be an excellent format for expressing design patterns in object-oriented programming. Here’s an example of a villanelle describing the Singleton pattern:
A single instance, forever alone,
Global access, a constant guide,
In Singleton, we find our cornerstone.
Constructor private, no clones are grown,
Instance created once, inside,
A single instance, forever alone.
Static method, its presence known,
Returns the instance, verified,
In Singleton, we find our cornerstone.
Thread-safe and lazy, or eagerly shown,
Implementation details may decide,
A single instance, forever alone.
Across the codebase, widely flown,
Shared state and behavior applied,
In Singleton, we find our cornerstone.
From logging to configs, its use has grown,
In patterns of design, it's a trusted ride,
A single instance, forever alone,
In Singleton, we find our cornerstone.
This villanelle captures the key aspects of the Singleton pattern, including its purpose, implementation details, and common use cases, all while adhering to the strict form of the villanelle.
Epic Poetry for System Architecture
For more complex topics like system architecture, we might turn to longer poetic forms such as epic poetry. Imagine describing a microservices architecture in the style of an epic poem, with each service represented as a character in a grand narrative.
Code Comments as Poetry
Another interesting application of poetic programming is the use of poetic forms in code comments. This can make documentation more engaging and memorable. For example:
// Merge Sort Haiku
// Divide the array
// Sort each half recursively
// Merge sorted halves
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
In this example, the haiku serves as a concise summary of the Merge Sort algorithm, providing a quick reference for developers reviewing the code.
The Future of Poetic Programming
As we continue to explore the intersection of coding and poetry, several exciting possibilities emerge:
1. AI-Generated Algorithmic Poetry
With advancements in natural language processing and AI, we could see the development of tools that automatically generate poetic representations of algorithms. This could be particularly useful for creating educational materials or documentation.
2. Visual Programming Poetry
Combining visual programming concepts with poetic structures could lead to new forms of representation that are both visually appealing and informationally rich.
3. Musical Algorithms
Extending the concept of poetic programming to music, we could create musical representations of algorithms, where different aspects of the algorithm are represented by various musical elements like rhythm, melody, and harmony.
4. Virtual Reality Poetic Coding Experiences
Imagine a virtual reality environment where learners can interact with poetic representations of algorithms, manipulating code structures in a 3D space while experiencing the rhythm and flow of the associated poetry.
Challenges and Considerations
While poetic programming offers many benefits, it’s important to consider some potential challenges:
- Accuracy vs. Artistry: Balancing the need for technical accuracy with poetic expression can be challenging. It’s crucial not to sacrifice correctness for the sake of adhering to a poetic form.
- Accessibility: Poetic representations might be more difficult for some learners, particularly those who struggle with language or have different learning styles.
- Scalability: While effective for certain concepts, poetic programming might be less suitable for extremely complex or abstract programming ideas.
- Cultural Considerations: Poetic forms and styles vary across cultures, so it’s important to consider diverse perspectives when implementing this approach globally.
Conclusion: The Symphony of Code and Verse
Poetic programming represents a harmonious blend of logic and creativity, offering a unique approach to understanding and expressing algorithmic concepts. By encapsulating the essence of algorithms in the rhythmic structures of haiku, sonnets, and other poetic forms, we create a bridge between the world of coding and the realm of artistic expression.
This innovative approach not only enhances memorization and understanding but also fosters creativity, improves communication skills, and makes the learning process more engaging and enjoyable. As we continue to explore and expand the boundaries of poetic programming, we open up new possibilities for interdisciplinary learning and creative problem-solving in the field of computer science.
Whether you’re a seasoned programmer looking for a fresh perspective or a beginner seeking a more accessible entry point into the world of coding, consider exploring the lyrical side of algorithms. Who knows? You might just find yourself composing sonnets about sorting algorithms or haiku about hash tables, all while deepening your understanding of fundamental programming concepts.
In the grand symphony of technology and art, poetic programming strikes a beautiful chord, reminding us that even in the most logical of disciplines, there’s always room for a touch of poetic inspiration. So, let your code sing, let your algorithms dance, and embrace the poetic programmer within!