Performance Measurement in Software Development: A Comprehensive Guide
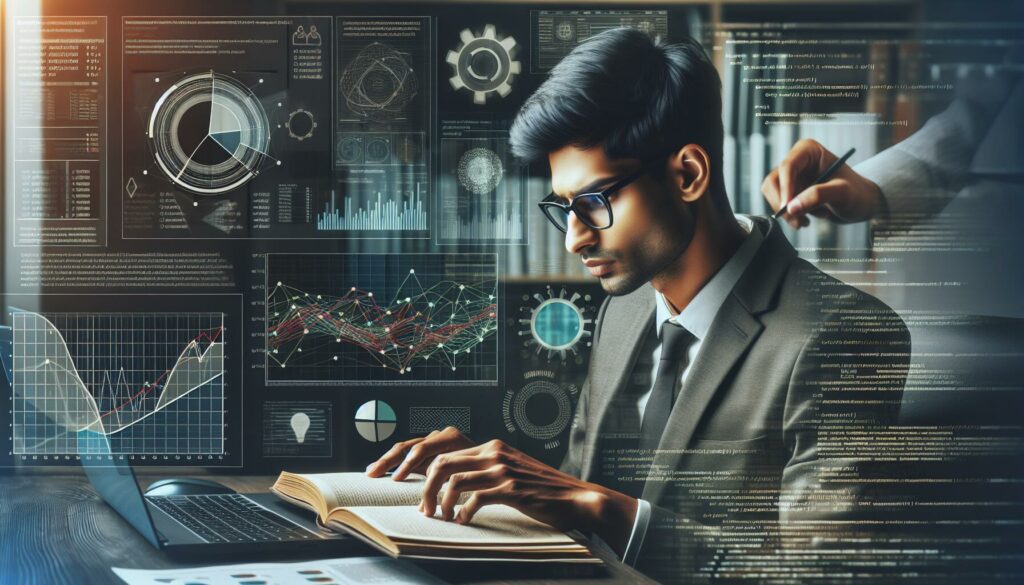
In the fast-paced world of software development, performance measurement plays a crucial role in ensuring the success and efficiency of projects. As coding education platforms like AlgoCademy focus on developing programming skills and preparing individuals for technical interviews, understanding performance measurement becomes increasingly important. This comprehensive guide will explore various aspects of performance measurement in software development, providing valuable insights for both beginners and experienced programmers.
Table of Contents
- Introduction to Performance Measurement
- The Importance of Performance Measurement
- Key Performance Metrics in Software Development
- Tools and Techniques for Performance Measurement
- Best Practices for Implementing Performance Measurement
- Challenges in Performance Measurement
- Case Studies: Real-world Examples of Performance Measurement
- The Future of Performance Measurement in Software Development
- Conclusion
1. Introduction to Performance Measurement
Performance measurement in software development refers to the process of collecting, analyzing, and reporting information about the performance of an individual, group, or organization. In the context of coding and software engineering, it involves evaluating various aspects of code efficiency, system performance, and development processes.
As platforms like AlgoCademy focus on helping individuals progress from beginner-level coding to preparing for technical interviews at major tech companies, understanding performance measurement becomes crucial. It not only helps in writing efficient code but also in assessing one’s progress and readiness for challenging technical roles.
2. The Importance of Performance Measurement
Performance measurement is essential in software development for several reasons:
- Identifying Bottlenecks: It helps in identifying performance bottlenecks in code or systems, allowing developers to optimize and improve efficiency.
- Ensuring Quality: By measuring performance, developers can ensure that their code meets quality standards and performs as expected.
- Guiding Optimization: Performance metrics guide optimization efforts, helping developers focus on areas that will yield the most significant improvements.
- Tracking Progress: For learners using platforms like AlgoCademy, performance measurement provides a way to track progress and identify areas for improvement.
- Meeting Requirements: In professional settings, performance measurement ensures that software meets specified performance requirements and service level agreements (SLAs).
3. Key Performance Metrics in Software Development
Several key metrics are commonly used to measure performance in software development:
3.1 Code-level Metrics
- Time Complexity: Measures how the execution time of an algorithm increases with the input size, often expressed using Big O notation.
- Space Complexity: Assesses the amount of memory an algorithm uses relative to the input size.
- Cyclomatic Complexity: Measures the number of linearly independent paths through a program’s source code.
- Code Coverage: Indicates the percentage of code that is executed during testing.
3.2 System-level Metrics
- Response Time: Measures the time taken for a system to respond to a user request.
- Throughput: Indicates the number of tasks or operations a system can handle in a given time period.
- Latency: Measures the delay between a user action and the system’s response.
- Resource Utilization: Tracks the usage of system resources like CPU, memory, and disk I/O.
3.3 Process Metrics
- Velocity: Measures the amount of work completed in a given time period, often used in Agile methodologies.
- Defect Density: Calculates the number of defects per unit of code.
- Lead Time: Measures the time from the start of development to delivery.
- Cycle Time: Tracks the time from when work begins on a task to when it’s completed.
4. Tools and Techniques for Performance Measurement
A variety of tools and techniques are available for performance measurement in software development:
4.1 Profiling Tools
Profiling tools help analyze the runtime behavior of code, identifying performance bottlenecks and resource usage. Some popular profiling tools include:
- Java VisualVM: A visual tool for profiling Java applications.
- Python cProfile: A built-in profiling module for Python.
- Valgrind: A tool suite for debugging and profiling Linux programs.
Example of using Python’s cProfile:
import cProfile
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
cProfile.run('fibonacci(30)')
4.2 Benchmarking
Benchmarking involves comparing the performance of different algorithms or implementations. Tools like Apache JMeter for web applications or language-specific benchmarking libraries can be used for this purpose.
4.3 Monitoring Tools
Monitoring tools help track system-level metrics in real-time. Examples include:
- Prometheus: An open-source monitoring and alerting toolkit.
- Grafana: A platform for monitoring and observability.
- New Relic: A cloud-based observability platform.
4.4 Code Analysis Tools
Static code analysis tools can help identify potential performance issues before runtime. Examples include:
- SonarQube: An open-source platform for continuous inspection of code quality.
- ESLint: A static code analysis tool for identifying problematic patterns in JavaScript code.
5. Best Practices for Implementing Performance Measurement
To effectively implement performance measurement in software development, consider the following best practices:
5.1 Establish Baseline Metrics
Before making any optimizations, establish baseline performance metrics. This provides a point of comparison for future improvements.
5.2 Set Clear Performance Goals
Define clear, measurable performance goals based on user requirements and business objectives.
5.3 Measure Regularly
Implement continuous performance measurement throughout the development lifecycle, not just at the end.
5.4 Use Appropriate Tools
Choose performance measurement tools that are appropriate for your technology stack and project requirements.
5.5 Focus on Critical Paths
Prioritize performance measurement and optimization efforts on critical paths and frequently used components of your application.
5.6 Consider Different Environments
Measure performance in different environments (development, staging, production) to account for variations.
5.7 Automate Where Possible
Automate performance testing and measurement processes to ensure consistency and save time.
6. Challenges in Performance Measurement
While performance measurement is crucial, it comes with its own set of challenges:
6.1 Complexity of Modern Systems
Modern software systems are often complex and distributed, making it challenging to measure performance comprehensively.
6.2 Variability in Test Environments
Performance can vary significantly between different environments, making it difficult to replicate production conditions in test environments.
6.3 Overhead of Measurement Tools
Some performance measurement tools can introduce their own overhead, potentially skewing results.
6.4 Interpreting Results
Interpreting performance data and translating it into actionable insights can be challenging, especially for complex systems.
6.5 Balancing Performance and Other Factors
Optimizing for performance may sometimes conflict with other important factors like maintainability, readability, or development speed.
7. Case Studies: Real-world Examples of Performance Measurement
7.1 Case Study 1: Netflix’s Performance Measurement
Netflix, a leading streaming service, places a high emphasis on performance measurement to ensure a smooth user experience. They use a variety of tools and techniques:
- Chaos Engineering: Netflix developed the Chaos Monkey tool to test the resilience of their infrastructure by randomly terminating instances in production.
- Real-time Monitoring: They use Atlas, their in-house time series database, for real-time operational insights.
- A/B Testing: Netflix extensively uses A/B testing to measure the performance impact of new features or changes.
By focusing on performance measurement, Netflix has been able to handle millions of concurrent streams while maintaining a high-quality user experience.
7.2 Case Study 2: Google’s PageSpeed Insights
Google’s PageSpeed Insights is a tool that measures the performance of web pages. It provides both lab data (collected in a controlled environment) and field data (from real user experiences). The tool measures various metrics including:
- First Contentful Paint (FCP)
- Largest Contentful Paint (LCP)
- First Input Delay (FID)
- Cumulative Layout Shift (CLS)
By providing these metrics along with suggestions for improvement, Google has helped countless developers optimize their websites for better performance.
7.3 Case Study 3: AlgoCademy’s Performance Tracking
While AlgoCademy is a hypothetical platform, let’s consider how a coding education platform might implement performance measurement:
- Code Execution Time: Measuring the execution time of user-submitted code against predefined test cases.
- Learning Progress Metrics: Tracking metrics like problems solved, time spent on exercises, and improvement over time.
- System Performance: Monitoring server response times and resource utilization to ensure a smooth learning experience for users.
- A/B Testing: Comparing different teaching methods or UI designs to optimize the learning experience.
By implementing these performance measurement strategies, a platform like AlgoCademy could continuously improve its effectiveness in helping users develop their coding skills.
8. The Future of Performance Measurement in Software Development
As software systems continue to evolve, so too will the approaches to performance measurement. Here are some trends and predictions for the future:
8.1 AI and Machine Learning in Performance Measurement
Artificial Intelligence (AI) and Machine Learning (ML) are increasingly being used to analyze performance data, predict potential issues, and even suggest optimizations. These technologies can process vast amounts of performance data and identify patterns that might be missed by human analysts.
8.2 Focus on User-Centric Metrics
There’s a growing emphasis on metrics that directly reflect the user experience, such as Google’s Core Web Vitals. This trend is likely to continue, with performance measurement becoming more closely aligned with actual user perception of performance.
8.3 Increased Automation
As systems become more complex, manual performance measurement becomes increasingly challenging. We can expect to see more automated tools that can continuously monitor, analyze, and even optimize performance with minimal human intervention.
8.4 Performance in Serverless and Edge Computing
With the rise of serverless computing and edge computing, new approaches to performance measurement will be needed. These architectures present unique challenges and opportunities for performance optimization.
8.5 Integration with DevOps Practices
Performance measurement is likely to become more tightly integrated with DevOps practices, with performance metrics being a key part of the continuous integration and deployment pipeline.
9. Conclusion
Performance measurement is a critical aspect of software development that impacts everything from user satisfaction to business success. As we’ve explored in this comprehensive guide, it encompasses a wide range of metrics, tools, and practices.
For learners using platforms like AlgoCademy, understanding performance measurement is crucial for developing efficient algorithms and preparing for technical interviews. It’s not just about writing code that works, but writing code that works efficiently and scales well.
As software systems continue to evolve and become more complex, the importance of effective performance measurement will only grow. By staying informed about the latest trends and best practices in performance measurement, developers can ensure they’re creating high-quality, efficient software that meets the needs of users and businesses alike.
Remember, performance measurement is not a one-time activity but an ongoing process. Continuous monitoring, analysis, and optimization are key to maintaining high-performing software systems. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for a FAANG interview, mastering the art and science of performance measurement will serve you well throughout your career in software development.